33 Javascript Mysql Query Example
10/6/2010 · Below is the example of PHP file. <?php $con = mysql_connect('localhost:3306', 'dbusername', 'dbpsw'); mysql_select_db("(dbname)", $con); $sql="SELECT * FROM table_name"; $result = mysql_query($sql); echo " <table border='1'> <tr> <th>Header of Table name</th> </tr>"; while($row = mysql_fetch_array($result)) { echo "<tr>"; echo "<td>" . $row['(database_column_name)'] . "</td>"; echo "<td>" . $row['database_column_name'] . "</td>"; echo "</tr>"; } echo "</table>"; mysql… 24/4/2018 · All the functions used for DreamFactory 2.0 REST API requests are extended in the file functions.js, and the code examples show how to use them. Table of Contents. Getting Started. Step 1: Import the application package. Step 2: Configure CORS. JavaScript-MySQL Code Examples. Logging in.
How To Connect To A Mysql Database With Javascript
// Node.js MySQL SELECT FROM query Example // include mysql module var mysql = require('mysql'); // create a connection variable with the required details var con = mysql.createConnection({ host: "localhost", // ip address of server running mysql user: "arjun", // user name to your mysql database password: "password", // corresponding password database: "studentsDB" // use the specified database }); // make to connection to …
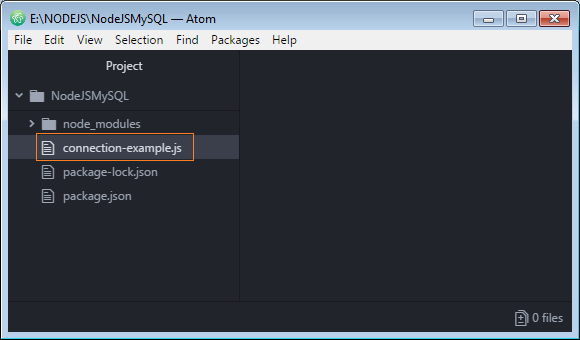
Javascript mysql query example. 5.4 Using the MySQL JavaScript Connector: Examples. This section contains a number of examples performing basic database operations such as retrieving, inserting, or deleting rows from a table. The source for these files ca also be found in share/nodejs/samples, under the NDB Cluster installation directory. The page on the server called by the JavaScript above is a PHP file called "getuser.php". The source code in "getuser.php" runs a query against a MySQL database, and returns the result in an HTML table: The client created by the configuration initializes a connection pool, using the tarn.js library. This connection pool has a default setting of a min: 2, max: 10 for the MySQL and PG libraries, and a single connection for sqlite3 (due to issues with utilizing multiple connections on a single file). To change the config settings for the pool, pass a pool option as one of the keys in the ...
Summary: in this tutorial, you will learn how to query data from a table in MySQL from a node.js application. The steps for querying data in the MySQL database from a node.js application are as follows: Establish a connection to the MySQL database server. Execute a SELECT statement and process the result set. Close the database connection. To fill a table in MySQL, use the "INSERT INTO" statement. Example. ... con.query(sql, function (err, result) ... JavaScript Tutorial How To Tutorial SQL Tutorial Python Tutorial W3.CSS Tutorial Bootstrap Tutorial PHP Tutorial Java Tutorial C++ Tutorial jQuery Tutorial. Top References Increase your knowledge of Node.js with our tutorial on MySQL events. Learn the basics of stored procedures, NPM, Express Routes & more.
Node mysql: This is a node.js driver for mysql. It is written in JavaScript, does not require compiling. It provides all most all connection/query from MySQL. Node-mysql is probably one of the best modules used for working with MySQL database which is actively maintained and well documented. To select data from a table in MySQL, use the "SELECT" statement. Example. Select all records from the "customers" table, and display the result object: var mysql = require ('mysql'); var con = mysql.createConnection( {. host: "localhost", user: "yourusername", … Aug 04, 2019 - Node.js and MySQL are some of the necessary binding needed for any web application. MySQL is one of the most popular open-source databases in the world and
JavaScript query - 13 examples found. These are the top rated real world JavaScript examples of mysql.query extracted from open source projects. You can rate examples to help us improve the quality of examples. Escaping Query Values. When query values are variables provided by the user, you should escape the values. This is to prevent SQL injections, which is a common web hacking technique to destroy or misuse your database. The MySQL module has methods to escape query values: MySQL- An open-source relational database management system (RDBMS), MySQL is backed by the Oracle Corporation and is free to use. Furthermore, the source code can be easily customized as per user requirements. After knowing these tools, you will be able to decode the Node.js MySQL Example with the utmost ease. Available Data Types In Sequelize
Node.js Tutorial - Learn Node.js MySQL SELECT FROM query to access rows of a table and also access the field information with Node.js example programs. } else { connection.query(sql, values, (err, rows) =>Packs CommonJs/AMD modules for the browser. Allows to split your codebase into multiple bundles, which can be loaded on demand. I know how to create a query with node.js, I just don't know a method to get the results I want.I don't know how to make a proper query. javascript mysql node.js Share
HERE "SELECT * FROM tableName" is the standard SELECT statement "WHERE" is the keyword that restricts our select query result set and "condition" is the filter to be applied on the results. The filter could be a range, single value or sub query. Let's now look at a practical example.. Suppose we want to get a member's personal details from members table given the membership ... MySQL workbench falls in the category of "Query by Example" QBE tools. It's intended to help generate SQL statements faster to increase the user productivity. Learning the SQL SELECT command can enable you to create complex queries that cannot be easily generated using Query by Example utilities such as MySQL workbench. 20/1/2020 · const mysql = require ('mysql'); // First you need to create a connection to the database // Be sure to replace 'user' and 'password' with the correct values const con = mysql. createConnection ...
It's just different syntax for achieving the same thing. The differences become more obvious when you try to do something more complex. Limitations of callbacks and promises. In my earlier article, Node.js, MySQL and promises, I gave an example of executing a few queries, one after another, and subsequently closing the connection. 6. Javascript cannot run MySQL Queries itself; however, you can use ajax to make a call to the server to retrieve the data. I like to use jQuery's ajax () for my ajax needs. Here is an example of how jquery's ajax () method works: $.ajax ( { url: "pathToServerFile", type: "POST", data: yourParams, dataType: "json" }); MySQL is one of the most popular open-source databases in the world and efficient as well. Almost every popular programming language like Java and PHP provides drivers to access and perform operations with MySQL. In this Node js and MySQL tutorial, we are going to learn how to connect Node js server with MySQL database. We will also learn how ...
First, navigate to your project folder in the command line, and install the database module: D:\YOUR\PROJECT> npm install mysql. For the sake of simplicity, we are just going to reuse the above dummy database table and MySQL. If you are not planning to use MySQL, there are also several other Node Database Modules: MSSQL. MongoDB. Setting an array for a mysql query with php 1 ; How to synchronize a file upload with an insert in MySQL through Javascript-AJAX-PHP? 12 ; How to create rss.php file 5 ; MySQL query works on page but not when used as a function. 3 ; using For loop to get Mysql Query Output 3 ; Creating a Cookie with a Counter 2 ; MySQL query generator - best ... Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
/** * Query MySQL as a promise * @param {String} q MySQL Query * @param {Object} data Data needed by the query * @returns ... Popular in JavaScript. request. Simplified HTTP request client. fs; express. Fast, unopinionated, minimalist web framework. ms. Tiny millisecond conversion utility. MySQL Queries. A list of commonly used MySQL queries to create database, use database, create table, insert record, update record, delete record, select record, truncate table and drop table are given below. 1) MySQL Create Database. MySQL create database is used to create database. For example constructor () { super (); mysqlPool. query ( 'SELECT 1 + 1 AS two', (error) => { if (error) { console.log (chalk.bgYellow.bold ( 'Warning:') + ' Cannot connect to the MySQL server. Error Code: ' + error.code); return ; } this .init (); }); } var sql_depend = function (ret, did, lid, uid, callback) { var cmd = …
May 22, 2017 - Javascript cannot run MySQL Queries itself; however, you can use ajax to make a call to the server to retrieve the data. I like to use jQuery's ajax() for my ajax needs. Here is an example of how jquery's ajax() method works: jQuery AJAX example with php MySQL. jQuery and Ajax are buzzwords now a days in web development community and it is a must have skill for a web developer. In this tutorial we are going to explore jQuery AJAX example with php MySQL and how we can send an AJAX get request using jQuery to fetch data from MySQL database server. Run example ». Notice the WHERE clause in the UPDATE syntax: The WHERE clause specifies which record or records that should be updated. If you omit the WHERE clause, all records will be updated! Save the code above in a file called "demo_db_update.js" and run the file: Run "demo_db_update.js". C:\Users\ Your Name >node demo_db_update.js.
The above example REST API for popular programming languages serves as a good starting point. We now have a functioning API server with Node.js and MySQL. In this tutorial, we learned how to set up MySQL on a free service, and how to create an Express.js server that can handle various HTTP methods in connection to how it translates to SQL queries. Apr 11, 2020 - In this tutorial, you will learn how to query data from a table in the MySQL database from a node.js application using the mysql module. Install MySQL Driver. Once you have MySQL up and running on your computer, you can access it by using Node.js. To access a MySQL database with Node.js, you need a MySQL driver. This tutorial will use the "mysql" module, downloaded from NPM. To download and install the "mysql" module, open the Command Terminal and execute the following:
Sep 21, 2015 - The reason is that while loading the page, javascript will be executed first, after that PHP script will be executed. hmmmm....sorry i didnot understood. if you dont mind how to use javascrit variables in php mysql query. is there any chance to workout like this. why because i have to change ... Using javascript only it's not possible because javascript by itself cannot connect to MySQL. Take a look at jQuery.ajax() - jQuery API If you want the script to wait to get the results and ...
Getting Data In Realtime From Mysql With Java
How To Create A Search Function For Your Website Using
Node Js Mysql Update Record Javatpoint
Node Js Mysql Update Query Example
First Crud Node Express Js Mysql Example
How To Display Data From Mysql Database Table In Node Js
Node Js Mysql Create Database Javatpoint
A Guide To Mysql With Node Js Part 1 Enabling Async And
Node Js Mysql Tutorial How To Build A Crud Application Edureka
Node Js Mysql Delete Query Example
Node Js Mysql Simple Api For Authentication Registration
How To Use An Aws Sql Database With Node Js And Mysql
Select In Mysql Output Results In Html Table
Knex Js A Sql Query Builder For Javascript
Loizenai Mobile Amp Web Programming Tutorials
First Crud Node Express Js Mysql Example
Build Node Js Rest Apis With Express Amp Mysql Bezkoder
1 How To Connect A Mysql Database With Node Js Tutorialswebsite
Github Nodeadmin Nodeadmin A Fantastically Elegant
How To Build A Crud Application Using Node Js And Mysql By
Mysql Saving Query Result Stack Overflow
Node Js Mysql Tutorial How To Build A Crud Application Edureka
Search Your Server Side Mysql Database From Node Js Website
Basic Login System With Node Js Express And Mysql
How To Use Mysql With Node Js And Docker By Catalin S Tech
Node Js Rest Apis Example With Express Sequelize Amp Mysql
Docker Step By Step Nodejs And Mysql App With React I 2020
Connect To Mysql Database In Nodejs
Nodejs Connect Mysql With Node App Geeksforgeeks
Crud Operations With Nodejs And Mysql W3jar Com
Node Js Mysql Tutorial How To Build A Crud Application Edureka
0 Response to "33 Javascript Mysql Query Example"
Post a Comment