23 Javascript New Regexp Global
Using test () on a regex with the "global" flag When a regex has the global flag set, test () will advance the lastIndex of the regex. (RegExp.prototype.exec () also advances the lastIndex property.) Further calls to test (str) will resume searching str starting from lastIndex. The exec () method is a RegExp expression method. It searches a string for a specified pattern, and returns the found text as an object. If no match is found, it returns an empty (null) object. The following example searches a string for the character "e": Example.
Regexp Archives Teachucomp Inc
Jun 29, 2017 - I wasn't sure if regex in Javascript could return an array like it does in PHP, this confirms it. – Joseph Kreifels II Mar 30 at 13:13 ... The global search flag makes the RegExp search for a pattern throughout the string, creating an array of all occurrences it can find matching the given ...
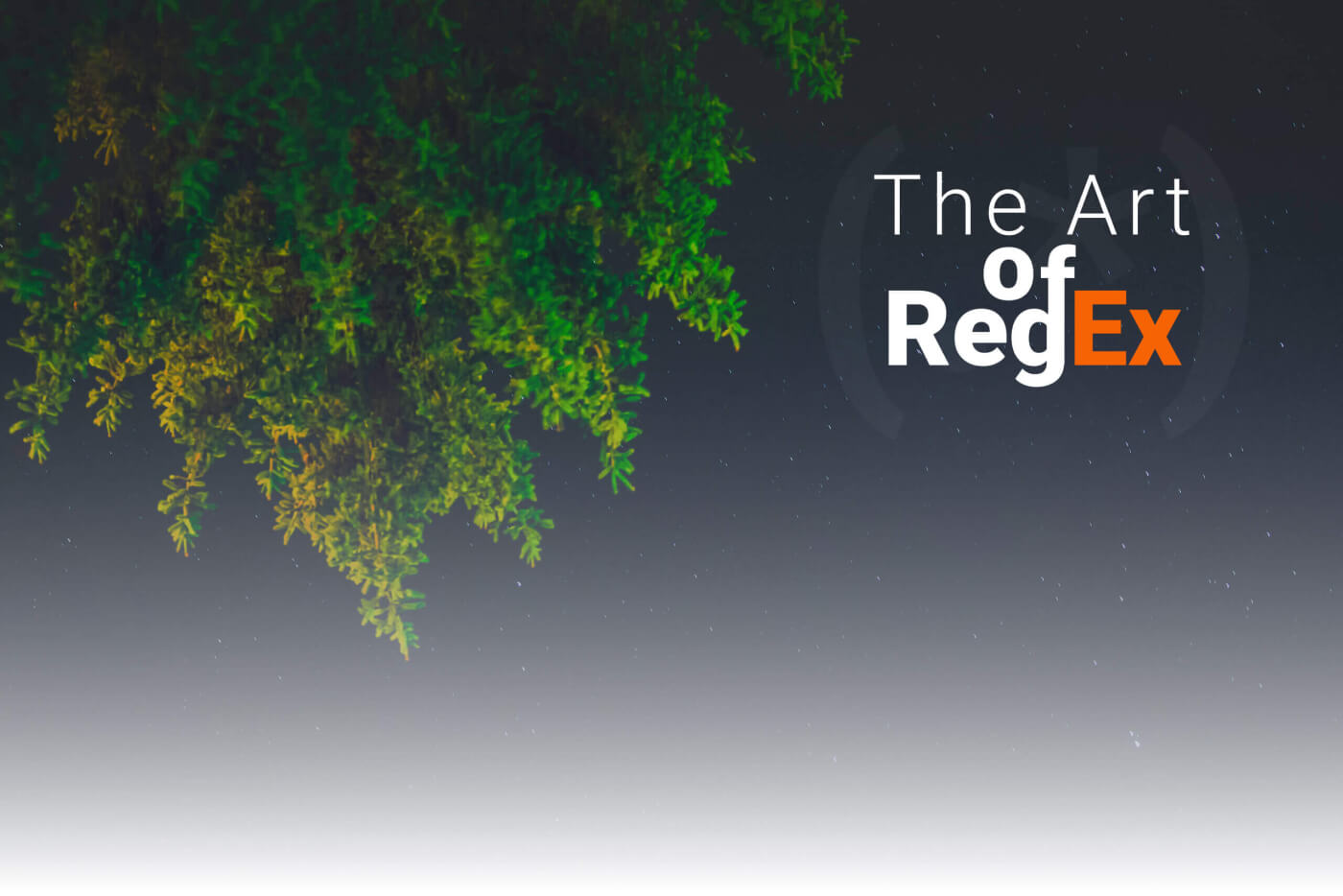
Javascript new regexp global. The RegExp constructor creates a regular expression object for matching text with a pattern. For an introduction to regular expressions, read the Regular Expressions chapter in the JavaScript Guide. /w3schools/i is a regular expression. w3schools is a pattern (to be used in a search). i is a modifier (modifies the search to be case-insensitive). For a tutorial about Regular Expressions, read our JavaScript RegExp Tutorial. It helps if you are familiar with ES5 regular expression features and Unicode. Consult the following two chapters of “Speaking JavaScript” if necessary: ... The new flag /y (sticky) anchors each match of a regular expression to the end of the previous match.
JavaScript » Objects » RegExp. Syntax: new RegExp ("pattern" [, "flags"]) The RegExp object contains the pattern of a regular expression, and is used to match strings using its methods and properties. The predefined RegExp object has static properties set whenever a regular expression is used, as well as user-defined ones for individual objects. With RegExp () syntax - every time new object gets created See, for instance, Stoyan Stefanov's JavaScript Patterns book: Another distinction between the regular expression literal and the constructor is that the literal creates an object only once during parse time. // A RegExp literal to match "and/or": var re3 = /and\/or/i; // Create the same object using RegExp() : var re4 = new RegExp("and/or", "i"); Note that with the regex literal syntax, the forward slash must be escaped (preceded with a single backslash) because with a regex literal, the forward slash has special meaning (it is a special ...
Aug 08, 2013 - Then JavaScript abuses the regular expression as an iterator, as a pointer into the sequence of results. That causes problems: · The above loop is infinite, because a new regular expression is created for each loop iteration, which restarts the iteration over the results. The \b character in a regex matches a word boundary, while the g flag after the regex symbol indicates a global search. The regex library of matching characters has been growing in Javascript for a... If we apply the same global regexp to different inputs, it may lead to wrong result, because regexp.test call advances regexp.lastIndex property, so the search in another string may start from non-zero position. For instance, here we call regexp.test twice on the same text, and the second time fails:
To make the method replace() replace all occurrences of the pattern you have to enable the global flag on the regular expression: Append g after at the end of regular expression literal: /search/g; Or when using a regular expression constructor, add 'g' to the second argument: new RegExp('search', 'g') Let's replace all occurrences of ... Sep 06, 2017 - This is strange because I would expect a new RegExp to be allocated each time the function is called and not shared across multiple invocations of my function. ... Then I don't get the lastIndex holdover effect. It works as I would expect it to. ... Not the answer you're looking for? Browse other questions tagged javascript ... Detailed description of the capabilities of the JavaScript RegExp Object, defined in the ECMA-262 standard.
Definition and Usage. The g modifier is used to perform a global match (find all matches rather than stopping after the first match). Tip: To perform a global, case-insensitive search, use this modifier together with the "i" modifier. Tip: Use the global property to specify whether or not the g modifier is set. Regular expressions are used with several String methods of JavaScript such as match(), replace(), search(), split(). RegExp objects sport built-in properties, methods and special characters that you can explore on this page. var regex = /pattern/; var regex = new RegExp("pattern"); Javascript Regex. A Practical Guide To Regular Expressions Regex In. Javascript Regular Expressions Example Tutorial. Regexp Definition And Modifiers Tutorial Teachucomp Inc. What Are Regular Expressions In Javascript And Are Their. Learn Regex A Beginner S Guide Sitepoint. New Regex Features With Examples Included 4 New Regular.
Regular expressions are implemented in JavaScript in two ways: Literal syntax: //match all 7 digit numbers. var phonenumber= /\d {7}/. Dynamically, with the RegExp () constructor: //match all 7 digit numbers (note how "\d" is defined as "\\d") var phonenumber=new RegExp ("\\d {7}", "g") A pattern defined inside RegExp () should be enclosed in ... Starting with ECMAScript 6, new RegExp (/ab+c/, 'i') no longer throws a TypeError ("can't supply flags when constructing one RegExp from another") when the first argument is a RegExp and the second flags argument is present. A new RegExp from the arguments is created instead. Interactive API reference for the JavaScript RegExp Object. RegExp represents a regular expression that can be used for searching and extracting parts of Strings.
A new regular expression is created as specified via pattern. If flags is missing, the empty string '' is used. new RegExp(regExp : RegExp, flags = regExp.flags) [ES6] regExp is cloned. If flags is provided, then it determines the flags of the clone. The second variant is useful for cloning regular expressions, optionally while modifying them. For regex, new RegExp(stringtofind, 'g');. BUT. If 'find' contains characters that are special in regex, they will have their regexy meaning. So if you tried to replace the '.' in 'abc.def' with 'x', you'd get 'xxxxxxx' — whoops. If all you want is a simple string replacement, there is no need for regular expressions! The RegExp constructor creates a regular expression object for matching text with a pattern. For an introduction on what regular expressions are, read the Regular Expressions chapter in the JavaScript Guide. Constructor. Literal and constructor notations are possible: /pattern/flags new RegExp(pattern[, flags]) Parameters pattern
JavaScript RegExp - global, global is a read-only boolean property of RegExp objects. It specifies whether a particular regular expression performs global matching, i.e., whether it was cr Creating JavaScript RegExp Object. Let's see how we can create a RegExp object in JavaScript: // Object Syntax let regExp = new RegExp("pattern", "flag"); // Literal Syntax let regExp = /pattern/flag; Where the pattern is the text of regular expression, the flag makes the regular expression search for a specific pattern. Jan 01, 2020 - function countMatches(regExp, str) { if (!regExp.global) { throw new Error('Flag /g of regExp must be set'); } if (regExp.lastIndex !== 0) { throw new Error('regExp.lastIndex must be zero'); } let count = 0; while (regExp.test(str)) { count++; } return count; }
The RegExp object is used for matching text with a pattern. The flag g stands for global, more specifically, global searching. It serves to make an expression look for all its matches, rather than stopping at the first one. By default, when a regex engine finds the first match for a given pattern in a given test string, it terminates and prevents any further searching. The Javascript string match () method allows us to collect values matched by regular expression patterns. The match () method is a bit funky is that it appears to behave differently depending on whether or not your regular expression contains the global flag, "g". When we call the match () method without a global regular expression, it returns ...
A regular expression is a string that describes a pattern e.g., email addresses and phone numbers. In JavaScript, regular expressions are objects. JavaScript provides the built-in RegExp type that allows you to work with regular expressions effectively. Regular expressions are useful for searching and replacing strings that match a pattern. Apr 22, 2019 - The RegExp g Modifier in JavaScript ... performs global match. ... Example 1: This example searches the word “geeks” in the whole string. ... Example 2: This example searches the word “portal” in the whole string and replaces it with “PORTAL”. ... JavaScript | RegExp ... RegExp.prototype.global The global property indicates whether or not the " g " flag is used with the regular expression. global is a read-only property of an individual regular expression instance.
true if the RegExp was created with the 's' flag. DotAll RegExps will match any character for '.' including new lines ('\r' or '\n') which do not match '.' by default. Another option is to replace '.' with an empty inverted character class match '[^]' for systems that do not support ECMAScript 2018. See also multiline. global is a read-only boolean property of RegExp objects. It specifies whether a particular regular expression performs global matching, i.e., whether it was created with the "g" attribute. JavaScript RegExp objects are stateful when they have the global or sticky flags set (e.g. /foo/g or /foo/y). They store a lastIndex from the previous match.
The following regular expression flags are available in JavaScript (tbl. 21 provides a compact overview): /g (.global): fundamentally changes how the following methods work. JavaScript regular expressions automatically use an enhanced regex engine, which provides improved performance and supports all behaviors of standard regular expressions as defined by Mozilla JavaScript. The enhanced regex engine supports using Java syntax in regular expressions. The enhanced regex
Script To Perform Multiple Regular Expressions Rename
Javascript And Al The Regular Expressions Example
What Are Regex Javascript Regular Expressions In 5 Minutes
How Javascript Works Regular Expressions Regexp By
A Practical Guide To Regular Expressions Regexp Properties
Easily Digestible Introduction To Regex In Javascript
How Javascript Works Regular Expressions Regexp By
Summary Of Javascript Regular Expressions Regex Tutorial By
Javascript Regexp Exec With Global Flag
The Simplest Way To Master Regex In Javascript
Introduction To The Use Of 10 Regular Expressions In
Javascript Regular Expression How To Create Amp Write Them In
Learn Regex A Beginner S Guide Sitepoint
Regular Expressions In Javascript With Examples
Understanding Regular Expressions In Javascript Weekly Webtips
Global Find And Replace Rules Copado Solutions Documentation
How You Can Learn Enough Regex In Javascript To Be Dangerous
Javascript Regular Expressions
Webkit Regexp Exploit Addrof Walk Through
Powering Your Javascript With New Regex Capabilities
How Javascript Works Regular Expressions Regexp By
0 Response to "23 Javascript New Regexp Global"
Post a Comment