25 Javascript Check Key In Object
May 06, 2021 - Learn more about How to Check If a Key Exists in a JavaScript Object? from DevelopIntelligence. Your trusted developer training partner. Get a customized quote today: (877) 629-5631. Unlike the in operator, this method does not check for the specified property in the object's prototype chain. The method can be called on most JavaScript objects, because most objects descend from Object, and hence inherit its methods. For example Array is an Object, so you can use hasOwnProperty() method to check whether an index exists:
8 Ways To Check If An Object Is Empty Or Not In Javascript
17/6/2019 · We can use 'in' operator on object to check its properties. The 'in' operator also looks about inherited property if it does not find any actual properties of the object. In the following example when 'toString' is checked whether present or not, the 'in' operator scrutinizes through the properties of the object.
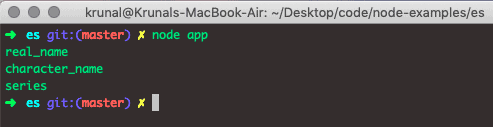
Javascript check key in object. How to check if a key exists in a JavaScript object, Method 1: Using 'in' operator: The in operator returns a boolean value if the specified property is in the object. Syntax: propertyName in object. There exist several ways of checking if a key exists in the object. The in operator matches all object keys, including those in the object's prototype chain. Use myObj.hasOwnProperty('key') to check an object's own keys and will only return true if key is available on myObj directly: myObj.hasOwnProperty('key') Check if Key Exists in JavaScript Object Using hasOwnProperty () In the below program, the hasOwnProperty () method is used to check if a key exists in an object. The hasOwnProperty () method returns true if the specified key is in the object, otherwise, it returns false.
Apr 28, 2021 - That’s all about checking if a key exists in a JavaScript object. The Object.keys () method accepts one parameter: the name of the Object whose keys you want to retrieve. This method returns the names of all keys in the Object you have specified, stored as a JavaScript list. Notice that the method itself is called Object.keys (). This is because keys () is a method of Object. Use myObj.hasOwnProperty('key') to check an object's own keys and will only return true if key is available on myObj directly: myObj.hasOwnProperty('key') Unless you have a specific reason to use the in operator, using myObj.hasOwnProperty('key') produces the result most code is looking for.
Problem: I just jump into the JavaScript learning process. I am grabbing the language day by day gradually. I want to declare an object or an array in my code and check whether the key is available or not in it. So, let me put my question this way, in javascript check if key exists in array? I’d really appreciate your effort and help. Mar 01, 2020 - "key" in obj // true, regardless of the actual value If you want to check if a key doesn't exist, remember to use parenthesis: !("key" in obj) // true if "key" doesn't exist in object !"key" in obj // ERROR! Equivalent to "false in obj" Or, if you want to particularly test for properties of ... Nov 16, 2020 - The 3 ways to check if an object has a property in JavaScript: hasOwnProperty() method, in operator, comparing with undefined.
JavaScript Check if Key Exists in Deeply Nested Object or Array of Objects, Without Knowing the Path The following JavaScript code recursively goes through the whole object, array of objects or a collection of both to verify if the given key exists somewhere down the object. It does not require path to the key in advance. JavaScript Array keys() ... Definition and Usage. The keys() method returns an Array Iterator object with the keys of an array. keys() does not change the original array. Browser Support. The numbers in the table specify the first browser version that fully supports the method: Chrome 38: Edge 12: 20/2/2020 · To avoid iterating over prototype properties while looping an object, you need to explicitly check if the property belongs to the object by using the hasOwnProperty () method: for (const key in user) { if ( user.hasOwnProperty( key)) { console.log(`$ {key}: $ {user[key]}`); } }
Check for the non-existing key, is key exists > false Check for the existing key, is key exists > true The Other style to check for the key by direct access is using the object style. In the following example, we can see how to check for that key existence in JavaScript. Object.keys() returns an array whose elements are strings corresponding to the enumerable properties found directly upon object.The ordering of the properties is the same as that given by looping over the properties of the object manually. JavaScript | Check if a key exists inside a JSON object Last Updated : 29 May, 2019 Given a JSON Object, the task is to check whether a key exists in Object or not using JavaScript. We're going to discuss few methods.
Get code examples like "check if object key exists javascript" instantly right from your google search results with the Grepper Chrome Extension. 6/2/2021 · In this article, we’ll explore how to check if a JavaScript Object has any keys on it. First, let’s quickly demonstrate the “old way” of doing things, which would involve using a for loop: const item = { id : ' 🥽 ' , name : ' Goggles ' , price : 1499 }; let hasKeys = false ; // oldschool approach for ( const key in item ) { if ( item . hasOwnProperty ( key )) { // a key exists at this point, for sure! hasKeys = true ; break ; // break when found } } 31/5/2020 · Given a JavaScript object, you can check if a property key exists inside its properties using the in operator. Say you have a car object: const car = { color: 'blue' } We can check if the color property exists using this statement, that results to true: 'color' in car We can use this in a conditional: if ('color' in car) { } Another way is to use the hasOwnProperty() method of the object:
The key exists. In the above program, the hasOwnProperty () method is used to check if a key exists in an object. The hasOwnProperty () method returns true if the specified key is in the object, otherwise it returns false. 11/8/2020 · Object.keys(hero) returns the list ['name', 'city'], which, as expected, are the keys of hero object. 1.1 Keys in practice: detect if object is empty. If you’d like to quickly check if an object is empty (has no own properties), then a good approach is to check whether the keys list is empty. To check if the object is empty, all you need to do is verify the length property of the array returned by Object.keys(object): Object.values () Method. Object.values () method is used to return an array whose elements are the enumerable property values found on the object. The ordering of the properties is the same as that given by the object manually is a loop is applied to the properties. Object.values () takes the object as an argument of which the enumerable own ...
Nov 20, 2020 - We are required to illustrate the correct way to check whether a particular key exists in an object or not. Before moving on to the correct way let's first ... Object.entries() returns an array whose elements are arrays corresponding to the enumerable string-keyed property [key, value] pairs found directly upon object. The ordering of the properties is the same as that given by looping over the property values of the object manually. If you only want to consider properties attached to the object itself, and not its prototypes, use getOwnPropertyNames () or perform a hasOwnProperty () check (propertyIsEnumerable () can also be used). Alternatively, if you know there won't be any outside code interference, you can extend built-in prototypes with a check method.
3 weeks ago - A protip by steveniseki about jquery and javascript. Here's a Code Recipe to check if an object is empty or not. For newer browsers, you can use plain vanilla JS and use the new "Object.keys" 🍦 But for older browser support, you can install the Lodash library and use their "isEmpty" method 🤖. const empty = {}; Object.keys(empty).length === 0 && empty.constructor === Object _.isEmpty(empty ... Sep 26, 2020 - You are able to create objects that contains key value pairs in JavaScript. They function much like they do in other languages, you are able to look up a value if you provide the key. This is an excellent way of storing values that you want to look up quickly, as you can easily check if an object ...
Jan 11, 2021 - I read somewhere that a for...in loop is up to 10x faster than Object.keys(), but that’s going to depend on the browser implementation — so I doubt modern browsers show such a difference. I’d argue that performance really doesn’t matter here. Why not? Because you’re checking for an ... Problem: Check if key in object javascript. account_circle. Log In Answer: Try the JavaScript in operator. And the inverse. Be careful! The in operator matches all object keys, including those in the object's prototype chain. Use myObj.hasOwnProperty ('key') to check an object's own keys and will only return true if key is available on myObj directly: Unless you have a specific reason to use the in operator ...
Object.keys () method is used to return an array whose elements are strings corresponding to the enumerable properties found directly upon an object. The ordering of the properties is the same as that given by the object manually in a loop is applied to the properties. Object.keys () takes the object as an argument of which the enumerable own ... In case you want to view all the enumerable keys in a JavaScript object, you can use the Object.keys() method, which looks and works as follows: let obj = { objName : "animal" , "data type" : typeof this , logName : function ( ) { console .log( this .objName); }, logDataType : function ( ) { console .log( this [ "data type" ]); } }; obj.logName(); // animal obj.logDataType(); // object 3 weeks ago - A protip by kyleross about objects, prototype, javascript, and isempty.
Conclusion The JavaScript [object Object] is a string representation of an object. To see the contents of an object, you should print the object to the console using console.log () or convert the object to a string. Or, you can use a for…in loop to iterate over the object and see its contents. Related Searches to Checking if a key exists in a javascript object - javascript tutorial check if key exists in json object javascript check if value exists in object javascript javascript check if key exists in map javascript check if value exists in array javascript object contains value javascript check if object exists in array if value in ... Jul 27, 2021 - Object.keys/values/entries ignore symbolic properties · Just like a for..in loop, these methods ignore properties that use Symbol(...) as keys.
Use myObj.hasOwnProperty ('key') to check an object's own keys and will only return true if key is available on myObj directly: myObj.hasOwnProperty ( 'key' ) Unless you have a specific reason to use the in operator, using myObj.hasOwnProperty ('key') produces the result most code is looking for. Source: Stackoverflow by For Your Own Good. There are mainly two methods to check the existence of a key in JavaScript Object. The first one is using "in operator" and the second one is using "hasOwnProperty () method". Method 1: Using 'in' operator: The in operator returns a boolean value if the specified property is in the object. This is the basic object syntax. But there are a few rules to keep in mind when creating JavaScript objects. Object Keys in JavaScript. Each key in your JavaScript object must be a string, symbol, or number. Take a close look at the example below. The key names 1 and 2 are actually coerced into strings.
How to get a key in a JavaScript object by its value ? Method 1: Checking all the object properties to find the value: The values of the object can be found by iterating through its properties. Each of these properties con be checked to see if they match the value provided. The properties of the object are obtained by using a for loop on the ...
How To Get A Key In A Javascript Object By Its Value
How To Remove A Property From A Javascript Object
Javascript Check If A Key Exists Inside A Json Object
How To Select Every Value Inside The Object In Js Code Example
How To Remove A Property From A Javascript Object
Javascript Tracking Key Value Pairs Using Hashmaps By
Javascript Equality Javascript Is Host To A Number Of By
Everything About Javascript Objects By Deepak Gupta
Javascript Object Exists Key Design Corral
How To Check If Object Is Empty In Javascript Samanthaming Com
Javascript Check If Object Key Exists Design Corral
Js Check If Array Of Objects Contains Key
Javascript Typeof How To Check The Type Of A Variable Or
How To Check If A Key Exists In A Javascript Object
Javascript Tip Property S Enumerability Learnjavascript
30 Seconds Of Code How To Rename Multiple Object Keys In
How To Check If The Localstorage Key Exists Or Not In
Converting Object To An Array Samanthaming Com
Object Keys Function In Javascript The Complete Guide
How To Check If A Key Exists In A Javascript Object
How To Check For The Existence Of Nested Javascript Object Key
How To Check If A Key Exists In Javascript Object
How To Check Two Objects Have Same Data Using Javascript
Javascript Check If Object Key Exists Design Corral
0 Response to "25 Javascript Check Key In Object"
Post a Comment