25 Javascript Alert Array To String
6/9/2019 · First take the values in a variable (lets arr). Pass the array name in the alert () . We can directly use the array name because arrayName automatically converted to arrayName.toString () Example 1: This example follows the approach discussed above. <!DOCTYPE HTML>. You can add elements with string indexes to JavaScript arrays, but does that mean JavaScript supports associative arrays? Subject explored with demos.
Convert String With Commas To Array Stack Overflow
Dec 16, 2010 - (Russian Version is here) What is an Array in JavaScript? A numerically indexed map of values. Traditionally an array reserves a continuous allocation of memory of predefined length. In JavaScript this is not the case. A JavaScript array is simply a glorified object with a unique constructor ...
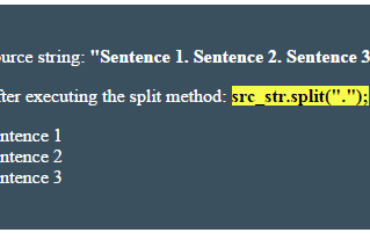
Javascript alert array to string. javascript string to json Use the JSON.stringify() Method. You can use the simple javascript JSON.stringify() method to simply convert a JavaScript object a May 19, 2016 - Simple function to alert contents of an object or an array . Call this function with an array or string or an object it alerts the contents. JavaScript does not support associative arrays. You should use objects when you want the element names to be strings (text). You should use arrays when you want the element names to be numbers. JavaScript new Array ()
Internally, the Array.prototype.includes() uses the same value zero algorithm to search for the presence of the element value in the array. Examples of JavaScript Array Contain. Let us consider a few examples of how the includes() method can be used to determine the presence of particular value in the array that is specified. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. To access the array elements, use the square bracket notation, with each set of brackets used to address each level of the array. In the following code, the array contents are printed out via an alert window, after being converted to a string first, if necessary:
May 13, 2021 - There exists a special data structure named Array, to store ordered collections. ... Almost all the time, the second syntax is used. We can supply initial elements in the brackets: ... Array elements are numbered, starting with zero. We can get an element by its number in square brackets: let fruits = ["Apple", "Orange", "Plum"]; alert... JavaScript calls the toString method automatically when an array is to be represented as a text value or when an array is referred to in a string concatenation. ECMAScript 5 semantics Starting in JavaScript 1.8.5 (Firefox 4), and consistent with ECMAScript 5th edition semantics, the toString () method is generic and can be used with any object. alert("Value of x" + x); JavaScript is a dynamic language. The conversion is done automatically for you. When you do something like "var x = string + int" Update. The reason your alert is failing now is because you start the alert with a double quotation mark and end the string piece of the alert with a single quote.
Sep 27, 2020 - Make a program that filters a list of strings and returns a list with only your friends name in it.javascript ... Write a function called lucky_sevens which takes an array of integers and returns true if any three consecutive elements sum to 7. by js We can use the + operator of javascript to convert array to string. Whenever we add an array to a string using the plus operator, javascript internally converts the objects that are being concatenated to string format. Similarly, if we add an array while doing the same, it gets converted to a string. JavaScript Array toString () Method. The toString () method is used for converting and representing an array into string form. It returns the string containing the specified array elements. Commas separate these elements, and the string does not affect the original array.
The JavaScript method toString () converts an array to a string of (comma separated) array values. Feb 26, 2020 - JavaScript exercises, practice and solution: Write a JavaScript program which prints the elements of the following array. The another way to check if a string is in array is by using indexof () function.
In vanilla JavaScript, you can use the Array.join () method to convert an array into a human-readable string. This method takes an array as input and returns the array as a string. By default, the elements are joined together by using a comma (,) as a separator: You can also specify a custom separator — dashes, spaces, or empty strings — as ... Accessing characters as numeric properties of a string is non-standard prior to ECMAScript 5 and doesn't work in all browsers (for example, it doesn't work in IE 6 or 7). You should use myString.charAt(0) instead when your code has to work in non-ECMAScript 5 environments. Alternatively, if you're going to be accessing a lot of characters in the string then you can turn a string into an array ... Jul 20, 2021 - The split() method divides a String into an ordered list of substrings, puts these substrings into an array, and returns the array. The division is done by searching for a pattern; where the pattern is provided as the first parameter in the method's call.
1 month ago - The join() method creates and returns a new string by concatenating all of the elements in an array (or an array-like object), separated by commas or a specified separator string. If the array has only one item, then that item will be returned without using the separator. Converting an Array to string in JavaScript Last updated on July 31, 2021 by Yogesh Singh Sometimes required to convert an Array to string format for displaying on the page, send to AJAX request, or further processing. Mainly it is done by traversing on the Array and concat value to a variable with a separator for string conversion. JavaScript String slice () method. You have to pass the start and end parameter value in the slice () method. A slice method will return the extracted parts in a new string. Where a first character has position will be 0 and the second has position 1, and so on. If you want string from the end then use negative number values.
In an earlier article, we looked at how to convert an array to string in vanilla JavaScript. Today, let us look at how to do the opposite: convert a string back to an array.. String.split() Method The String.split() method converts a string into an array of substrings by using a separator and returns a new array. It splits the string every time it matches against the separator you pass in as ... JavaScript array to string without commas Sometimes you may need to convert your array into a string without the commas. The toString method has no parameter, so you need to use join method instead. join accepts one string parameter which will serve as the separator for your string:
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. JavaScript support various types of array, string array is one of them. String array is nothing but it's all about the array of the string. The array is a variable that stores the multiple values of similar types. In the context of a string array, it stores the string value only. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
28/3/2013 · 3 Answers3. In the line btn.Attributes.Add ("onclick", "javascript:test (str);"); str is a string, not a variable. You can simply call the join method on an array to merge the elements in the array: Where above the , is the delimiter. It seems you have a .NET array you want to encode to javascript. The sort () method sorts the elements of an array. The sort order can be either alphabetic or numeric, and either ascending (up) or descending (down). By default, the sort () method sorts the values as strings in alphabetical and ascending order. This works well for strings ("Apple" comes before "Banana"). How to Convert JavaScript array to string? Using the toString() or join() method you can easily Convert JavaScript array to string. The elements will be separated by a specified separator. It's up to you to choose any separator in a string or not. Note: Using this methods will not change the original (given) array.
If you want to see the array as an array, you can say. alert(JSON.stringify(aCustomers)); instead of all those document.writes. http://jsfiddle /5b2eb/ However, if you want to display them cleanly, one per line, in your popup, do this: alert(aCustomers.join("\n")); http://jsfiddle /5b2eb/1/ An Uint8Array is a typed array that represents an array of 8-bit unsigned integers. The following list of methods , provide a collection of efficient ways to convert an Uint8Array to a regular string in javascript. Little strings Summary: in this tutorial, you will learn how to display an alert dialog by using the JavaScript alert() method. Introduction to JavaScript alert() method. The browser can invoke a system dialog to display information to the user. Click here to show the alert system dialog.
convert an array to a GET param string that can be appended to a url could be done as follows . function encodeGet(array){ return getParams = $.map(array , function(val,index) { var str = index + "=" + escape(val); return str; }).join("&"); } Using join() method to convert an array to string in javascript. If you want to form the string of the array values with custom separator then join(separator) is the method you are looking for. JavaScript Array Displaying elements ... split():Creating array by splitting string variable toString: To join all elements and create a string concat: To join two or more arrays to a single array slice: To return element from an array with starting and ending positions Two Dimensional ...
Quick Reach 1 Alerts in JavaScript 2 Alert box syntax 2.1 An alert example 2.2 An alert with new line example 2.3 The alert with variable example 2.4 JavaScript alert title 3 JavaScript confirm box 4 JavaScript prompt example 5 Related Alerts in JavaScript The alert is the way to […] Javascript Object Notation. Simply put, representing arrays and objects in a flat string format. If you are looking for a way to store or transfer an array as a string - JSON is one of the de-facto industry standards and one that you should know. P.S. JSON.stringify (ARRAY) will turn an array into a string. JavaScript - Array to String in JavaScript JavaScript's toString() method operates on a given object, returning its text representation as a string. Applying the toString() method to an array returns the values in the array as a string. Converting an Array to a String. Let's look at three examples of converting an array to a string ...
How to convert a string into an array of characters in JavaScript? Here are 4 ways using the built-in split and 3 new ES6 methods. Read which is best for different scenarios... Next, we parse the string using JSON.parse. Lastly, we output the the value for keyOne, which looks like this: String to JSON: Value one; Conclusion. Converting an array of bytes to a string with JavaScript is very easy when we use the fromCharCode function, however, if you string have special characters you might want to look at a different ... JavaScript alert displaying stringified objects And that's how you can use the alert () method to display variable values. You can use the alert () method to display multiple variables too, just like a normal string. It's recommended for you to use template string to ember the variables in your alert box as follows:
Javascript Interview Questions
Javascript String Split Tutorial
Top Javascript Interview Questions Amp Answers Of 2021
Javascript Basic Remove A Character At The Specified
For Each Over An Array In Javascript Stack Overflow
Javascript Array Print The Elements Of An Array W3resource
4 Ways To Print In Javascript Wikihow
Restful Web Services By Lojfe Issuu
6 Simple And Beautiful Javascript Alert With Demos And Code
How To Print An Object In An Array To Console Coding
Loop Through Words In A String Javascript Iterate Words In
Javascript Array Tostring Method Javatpoint
How To Pop An Alert Message Box Using Php Geeksforgeeks
Multi Dimensional Array In Javascript Properties Amp Top 8
How To Convert An Array To A String In Javascript
String Array In Javascript Type Of Array In Javascript With
How To Convert Comma Separated String Into An Array In
How To Convert A String To Bytearray Stack Overflow
How To Remove Commas From Array In Javascript
Javascript Join Array Elements Into String Start Index Code
0 Response to "25 Javascript Alert Array To String"
Post a Comment