33 How To Convert To String Javascript
How to convert Set to String with spaces in javascript. Multiple ways we can convert Set to String with spaces. Array.from with join function. First , convert set into Array using Array.from() function. Array.from() method accepts set or any iterated array of objects and returns an array. Next, Iterate resulted array lements and use join ... Nov 06, 2017 - In JavaScript, you can represent a number is an actual number (ex. 42), or as a string (ex. '42'). If you were to use a strict comparison to compare the two, it would fail because they’re two different types of objects.
Es6 Convert String To Number Code Example
Can you use the string "number" as a variable name? Well, it is possible, and here in this simple tutorial, I am going to show you how to convert any string into a variable in JavaScript. To do this task, we will use the JavaScript eval() function. Well, this is the function that will play the main role to create variable name from our string.
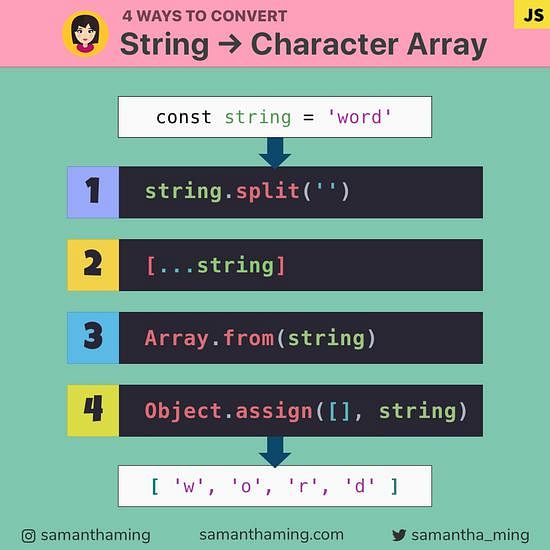
How to convert to string javascript. Nov 03, 2019 - Managing data is one of the fundamental concepts of programming. Converting a Number to a String is a common and simple operation. The same goes for the other... You can use the simple javascript JSON.stringify () method to simply convert a JavaScript object a JSON string. javascript string to json You can use the JSON.parse () method in JavaScript, to convert a JSON string into a JSON object. Convert String to JSON Using json.stringify () How to Convert a Number to a String in JavaScript¶ The template strings can put a number inside a String which is a valid way of parsing an Integer or Float data type: let num = 10 ; let flt = 10.502 ; let string = ` ${num} ` ; // '10' console .log(string); let floatString = ` ${flt} ` ; // '10.502' console …
Sep 13, 2020 - There are two main ways to convert a string to a number in javascript. One way is to parse it and the other way is to change its type to a Number. All of the tricks in the other answers (e.g. unary plus) involve implicitly coercing the type of the string to a number. Jul 20, 2021 - The toString() method returns a string representing the specified object. 10/5/2016 · Without converting any of the strings in your array into integers or operators you could write. eval(myArray[0] + myArray[1] + myArray[2]); which will translate to . eval("225" + "+" + "15"); which will translate to. eval("225 + 15"); which will return 240, the value of adding 225 to 15. Or even more generic: eval(myArray.join(' '));
Converting objects to string Both approaches first convert an object to a primitive, before converting that primitive to string. However, + uses the internal ToPrimitive(Number) operation (except for dates ), while String() uses ToPrimitive(String). ToPrimitive(Number): To convert an object obj to a primitive, invoke obj.valueOf().If the result is primitive, return that result. How to convert a date object to string with format hh:mm:ss in JavaScript? Convert object to a Map - JavaScript. Convert Java String Object to Boolean Object. Sep 22, 2020 - The toString() method returns a string representing the object.
The toString() method is also called when you need to convert the object into a string: var obj = { siteName : "W3Docs" , bookName : "Javascript" , booksCount : 5 }; function objToString ( object ) { var str = '' ; for ( var k in object) { if (object.hasOwnProperty(k)) { str += k + '::' + object[k] + '\n' ; } } console .log(str); return str; } objToString(obj); Using join() method to convert an array to string in javascript. If you want to form the string of the array values with custom separator then join(separator) is the method you are looking for. Dec 02, 2015 - You can call Number object and then call toString(). ... You may use this trick for another javascript native objects. ... Just come across this recently, method 3 and 4 are not appropriate because how the strings are copied and then put together. For a small program this problem is insignificant, ...
In an earlier article, we looked at how to convert an array to string in vanilla JavaScript. Today, let us look at how to do the opposite: convert a string back to an array.. String.split() Method The String.split() method converts a string into an array of substrings by using a separator and returns a new array. It splits the string every time it matches against the separator you pass in as ... The toString () method is a built-in method of the JavaScript Number object that allows you to convert any number type value into its string type representation. How to Use the toString Method in JavaScript To use the toString () method, you simply need to call the method on a number value. Another way of dealing with string numbers is using the parseInt () method of JavaScript. parseInt () takes two arguments, one is the string that needs to be converted, and the other one is the radix (means the base). Most numbers that we deal with in our day-to-day life are usually in the base of 10, which signifies a decimal value.
5 Answers5. eval () This will convert string to javascript code. eval ("console.log ('Alive! Woo!')"); eval and new Function let you parse and execute JavaScript code from strings. In general, avoid executing code from strings. The toString() method converts a number to a string.
There is only one native Javascript function to turn an object into a string - the JSON.stringify() function. So if you are looking for alternative ways to convert an object into a string, the only option is to create a custom "to string" function. In vanilla JavaScript, you can use the Array.join () method to convert an array into a human-readable string. This method takes an array as input and returns the array as a string. By default, the elements are joined together by using a comma (,) as a separator: You can also specify a custom separator — dashes, spaces, or empty strings — as ... Jul 28, 2021 - Parsing JSON (converting strings to JavaScript objects)
// program to convert an object to a string const person = { name: 'Jack', age: 27 } const result = JSON.stringify(person); console.log(result); console.log(typeof result); Output {"name":"Jack","age":27} string. In the above example, the JSON.stringify() method is used to convert an object to a string. Jul 17, 2014 - Note: Only the first number in the string is returned! Note: Leading and trailing spaces are allowed. Note: If the first character cannot be converted to a number, parseInt() returns NaN. One of the easiest ways to convert a string to lowercase is by using Javascript's toLowerCase () method. It's quite simple. The syntax is as follows: string.toLowerCase () The above syntax will produce a lowercase version of the given string. Let's look at an example of how to do implement this function.
May 05, 2020 - There are also cases when we need to explicitly convert a value to the expected type. ... In this chapter, we won’t cover objects. For now we’ll just be talking about primitives. Later, after we learn about objects, in the chapter Object to primitive conversion we’ll see how objects fit in. ... String ... Automatic Type Conversion. When JavaScript tries to operate on a "wrong" data type, it will try to convert the value to a "right" type. The result is not always what you expect: 5 + null // returns 5 because null is converted to 0. "5" + null // returns "5null" because null is converted to "null". "5" + 2 // returns "52" because 2 is converted ... 18/4/2019 · By concatenating with empty string "". This the most simplest method which can be used to convert a int to string in javascript. As javascript is loosely typed language when we concatenate a number with string it converts the number to string. As you can see we can also convert floating point and exponential numbers.
5 Ways to Convert a Value to String in JavaScript. Samantha Ming. Follow. ... So the battle really comes down to toString() and String() when you want to convert a value to a string. This one does ... Apr 26, 2013 - Hey, Im trying to convert specific javascript objects to a String. So far I'm working with json2.js. As soon as my Object contain functions, those functions are stripped. I need a way to convert Aug 23, 2019 - This function takes a string and converts it into an integer. If there is no integer present in the string, NaN will be the output.
To convert a number to a string, we need to use the method in JavaScript. Here is an example: Similarly, we can also do it by adding an… The String() function converts the value of an object to a string. Note: The String() function returns the same value as toString() of the individual objects. Browser Support Dec 02, 2015 - I found three ways to cast a variable to String in JavaScript. I searched for those three options in the jQuery source code, and they are all in use. I would like to know if there are any differences
Convert array to string, elements will be separated by commas. Click Here: ARRAY.JOIN(SEPARATOR) Convert array to string, elements will be separated by defined SEPARATOR. Click Here: ARRAY.flat(LEVEL) Flatten the array to the specified LEVEL. Click Here: JSON.stringify(ARRAY) JSON encode an array or object into a string. Click Here + The add ... Which method converts an array to string in JavaScript. There are two methods we can use to convert an array to string in JavaScript, either the Array.prototype.toString method, or the Array.prototype.join method. Using toString keeps things nice and simple, but using the join method gives us much more control. To convert Javascript int to string, use the toString() method. The toString() method converts the number to a string. Syntax number.toString(base) Parameters. The toString() function accepts a single optional parameter base. The base parameter defines the base in which the integer is represented in the string.
There are 5 ways to convert a value to a string.They are . Concatenating empty strings. Template strings . JSON. stringify . toString() String() Example. In the following example, all the above-mentioned methods were used to convert a value to a string and the final result was displayed as shown in the output. Apr 28, 2021 - The value to parse. If the string argument is not a string, then it is converted to a string (using the ToString abstract operation). Leading whitespace in the string argument is ignored.’= radix An integer between 2 and 36 that represents the radix (the base in mathematical numeral systems) ... 22/5/2019 · Last Updated : 23 May, 2019. Below are the methods to convert different objects to string. Method 1: Using the function String () The String () function converts the value of an object to a string. Syntax: String (object) Parameter: JavaScript Object. Example :
To convert array to string in JavaScript, use the toString () method. The array.toString () is a built-in JavaScript method that returns a string with all array values separated by commas. The toString () method does not modify the original array. Converting Number to String. There are some built-in methods in JavaScript that provide conversion from an number data type to a String, which only differ in performance and readability. These are:.toString() String() Template Strings; Concatenating an Empty String.toString() Next, we parse the string using JSON.parse. Lastly, we output the the value for keyOne, which looks like this: String to JSON: Value one; Conclusion. Converting an array of bytes to a string with JavaScript is very easy when we use the fromCharCode function, however, if you string have special characters you might want to look at a different ...
Jul 20, 2021 - The toString() method in Javascript is used with a number and converts the number to a string. It is used to return a string representing the specified Number object.
How To Convert Integer Array To String Array Using Javascript
How To Convert Js Object To Json String In Jquery Javascript
4 Ways To Convert A String To An Array In Javascript Dev
Converting An Object To A String Stack Overflow
How To Convert String To Number In Javascript Or Typescript
C Java Php Programming Source Code Javascript Convert
How To Avoid Javascript Type Conversions By Viduni
5 Ways To Convert A Value To String In Javascript
Convert Array To String In Javascript Wisetut
Javascript Convert A String To Title Case W3resource
How To Convert Comma Separated String Into An Array In
How To Convert Comma Separated String Into An Array In
Automatically Convert String To Upper Case With Javascript
Beginner Goodies Convert A Javascript Object To Json String
Javascript Convert String Array To Array
How To Convert An Array To A String In Javascript
Convert String To Real Number Knime Analytics Platform
How To Convert Char Into Int In Javascript Code Example
How To Convert String To Float Number In Javascript
4 Ways To Convert String To Character Array In Javascript
9 Ways To Convert Strings Into Numbers In Javascript By
Convert Comma Separated String To Array Using Javascript
Convert Javascript String To Ascii Array Example Code
How To Convert A String To An Integer In Javascript Stack
Javascript Parse String How Javascript Parse String Work
3 Ways To Convert String To Object In Javascript
Convert A String To A Number In Javascript Clue Mediator
How To Convert A String To An Integer In Javascript Stack
9 Ways To Convert Strings Into Numbers In Javascript By
Javascript Convert String To Number Convert String To Array
Javascript Numbers Get Skilled In The Implementation Of Its
Convert Javascript String To Int Javascript Parseint
0 Response to "33 How To Convert To String Javascript"
Post a Comment