26 Compare Empty Object Javascript
Jan 14, 2020 - I'll show you how to check if an object is empty in JavaScript. Checking if an object is empty is not as simple as checking if an array is empty. "Thankfully since null isn't really an object, it's the only 'object' that is a falsy value, empty objects are truthy." — Casey Morris in Daily JS. A nother method of checking for null is based on the fact that null is falsy, but empty objects are truthy, so null is the only falsy object.
In Javascript Why Does Object Getprototypeof 1 2 Returns
JavaScript provides 3 ways to compare values: The strict equality operator ===. The loose equality operator ==. Object.is () function. When comparing objects using any of the above, the comparison evaluates to true only if the compared values reference the same object instance. This is the referential equality.
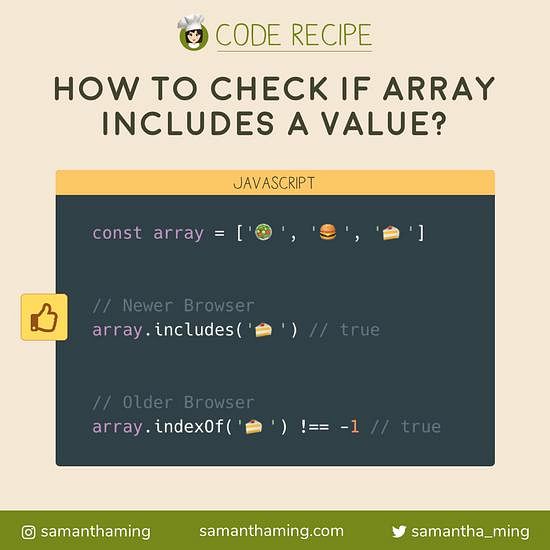
Compare empty object javascript. Object Equality in JavaScript. Originally published in the A Drip of JavaScript newsletter. Equality is one of the most initially confusing aspects of JavaScript. The behavior of == versus ===, the order of type coercions, etc. all serve to complicate the subject. Today we'll be looking at another facet: how object equality works. Morioh is the place to create a Great Personal Brand, connect with Developers around the World and Grow your Career! JavaScript automatically converts primitives to String objects, so that it's possible to use String object methods for primitive strings. In contexts where a method is to be invoked on a primitive string or a property lookup occurs, JavaScript will automatically wrap the string primitive and call the method or perform the property lookup.
JavaScript provides the typeof operator to check the value data type. The operator returns a string of the value data type. For example, for an object, it will return "object". However, for arrays and null, "object" is returned, and for NaN/Infinity, "number" is returned. It is somehow difficult to check if the value is exactly a real object. May 13, 2020 - Sometimes it’s the basics that get you, so here are 10 ways to check if an object is empty in Javascript. Objects are not like arrays or strings. So simply comparing by using "===" or "==" is not possible. Here to compare we have to first stringify the object and then using equality operators it is possible to compare the objects. In the following example, objects were stringified () at first and then compared with each other.
Jul 24, 2019 - Check if a given object is empty or not. ... ExpressionChangedAfterItHasBeenCheckedError: Expression has changed after it was checked. Previous value: 'ngIf: [object Object]'. Current value: 'ngIf: true'. JSON.stringify() is often used to check if an object is empty. Yet, I wouldn't recommend using it this way. I will explain the reasons behind that, but first, let's see how it works. JSON.stringify() converts an object to it's JSON string representation. If the object is empty we'll always get a "{}" JSON string as a result. If the object is not empty, the JSON string will contain all ... 18/8/2019 · The Object.keys() method is probably the best way to check if an object is empty because it is supported by almost all browsers including IE9+. It returns an array of a given object's own property names. So we can simply check the length of the array afterward: Object. keys ({}). length === 0; // true Object. keys ({name: 'Atta'}). length === 0; // false
Sep 01, 2016 - How to check Object is empty or not in Jquery/JavaScript ? By Hardik Savani January 9, 2016 Category : PHP Javascript jQuery · you can check your JavaScript OR jQuery object is empty or not, because we need to check many place our jQuery object is empty, null or undefined etc., So usually, ... Apr 28, 2021 - This post will discuss how to check for an empty object in JavaScript... The idea is to use the `Object.entries()` method, which returns an array object's own [key, value] pairs. Jun 08, 2020 - Object.keys will return an Array, which contains the property names of the object. If the length of the array is 0, then we know that the object is empty. In the above code, we will loop through…
How to check if an object is empty in JavaScript Find out how to see if a variable is equivalent to an empty object. Published Sep 10, 2019. Say you want to check if a value you have is equal to the empty object, which can be created using the object literal syntax: const emptyObject = {} How can you do so? The object that will be checked to see if it's empty. As of jQuery 1.4 this method checks both properties on the object itself and properties inherited from prototypes (in that it doesn't use hasOwnProperty). The argument should always be a plain JavaScript Object as other types of ... In Javascript, to compare two arrays we need to check that the length of both arrays should be same, the objects present in it are of the same type and each item in one array is equal to the counterpart in another array. By doing this we can conclude both arrays are the same or not.
the empty string "" (equivalent to `` or '') Values not on the list of falsy values in JavaScript are called truthy values and include the empty array [] or the empty object {} . The JSON.stringify method is used to convert a JavaScript object to a JSON string. So we can use it to convert an object to a string, and we can compare the result with {} to check if the given object is empty. Let's go through the following example. JavaScript: Empty Object Comparison The following is my summary of empty object comparsion. They used in node command line. They are slightly difference. For prototype inheritance, always use var o…
Use the code above when you are comparing objects and arrays. If the class of the object is important to you (for dates for example) compare the classes too using the instanceof operator in a separate test case. Happy testing :). Update 1: Improved assertObjectEqual to support an array of objects as well as just an array of primitives. Apr 02, 2019 - Learn the easiest ways to check if a specific object in JavaScript is empty or not. When comparing a string with a number, JavaScript will convert the string to a number when doing the comparison. An empty string converts to 0. A non-numeric string converts to NaN which is always false. When comparing two strings, "2" will be greater than "12", because (alphabetically) 1 is less than 2.
Apr 30, 2019 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. In Example 4, Object.is(obj1,obj2); would return true. So even if two objects contain the same data, === and Object.is() will return false, unless the variables contain a reference to the same object. Comparing object keys and values is more complex. Possibly Simple Solution. One possible simple solution is to JSON.stringify the objects and ... 4 weeks ago - A protip by kyleross about objects, prototype, javascript, and isempty.
24/3/2009 · var myObject = {}; // Object is empty var isEmpty = isObjectEmpty(myObject); // will return true; // populating the object myObject = {"name":"John Smith","Address":"Kochi, Kerala"}; // check if the object is empty isEmpty = isObjectEmpty(myObject); // will return false; from here. Update. OR. you can use the jQuery implementation of isEmptyObject # ES6 Way for comparing 2 objects. This is a solution suggested by @mustafauzun0. Few things to note though, it won't work with nested objects and the order of the keys are important. The idea behind this is similar to the stringify way. It coverts the object into a string and compare if the strings are a match. In javascript, we can check if an object is empty or not by using. JSON.stringify. Object.keys (ECMA 5+) Object.entries (ECMA 7+) And if you are using any third party libraries like jquery, lodash, Underscore etc you can use their existing methods for checking javascript empty object.
Sep 18, 2020 - Checking if the Object is empty or not is quite a simple & common task but there are many ways to... Tagged with javascript, beginners. Dec 07, 2017 - Quora is a place to gain and share knowledge. It's a platform to ask questions and connect with people who contribute unique insights and quality answers. 12/4/2021 · EMPTY: USED FOR: Explicitly define “nothing” or “absent”. A variable without an assigned value. An empty string. EXAMPLE: var members = ["Jon", "Joy", null]; var result; var table = ""; DATA TYPE: Object. Undefined. String. COMPARISON: null == undefined null !== undefined null != "" null !== "" undefined == null undefined !== null undefined != "" undefined !== ""
Test if two objects are the same. These two objects are the same. var myObj = { a: 1 }; Object.is(myObj, myObj); //True These two objects are not the same. var myObj = { a: 1 }; var myObj2 = { a: 1 }; Object.is(myObj, myObj2); //False Arrays. This checks to see if array is undefined, null, or empty. let myArray = []; // Array does not exist, is ... testArray is not empty array. Suggested read : JavaScript String Includes and How To Use Examples 4. Check if the object is empty. I will give you the shortest way to check if the javascript object is empty. Here is a readymade helper function to validate any object for nonemptiness. Objects are the reference type in JavaScript and are also named value pairs, where the value may determine a property or behaviour. They are used extensively because nowadays the web development has changed vastly. This article describes how to compare two JavaScript objects in the following formats: Comparing JavaScript Objects based on reference
Comparing two arrays in JavaScript using either the loose or strict equality operators (== or ===) will most often result in false, even if the two arrays contain the same elements in the same order. This is due to the fact that arrays and objects are compared by reference and not by value in JavaScript, which means this solution does not ... Values in Javascript can come from. Object - object reference. String - immutable, 16-bit USC-2. Boolean - true, false. Number - only 64-bit floating point, IEEE 754 (double) null - empty object reference. undefined - unassigned variables and function arguments, missing object properties. Object.is () determines whether two values are the same value. Two values are the same if one of the following holds: both undefined. both null. both true or both false. both strings of the same length with the same characters in the same order. both the same object (meaning both values reference the same object in memory)
If we pass an object to the Object.getOwnPropertyNames () method it returns an array of object properties.so that by using the length of that array we can easily check if the given object is empty or not. Similarly, we can use the Object.keys () or Object.values () method. The Object.keys () method returns an array with object keys. Apr 12, 2016 - 4 thoughts on “Javascript – Is an Object Empty?” Here's a Code Recipe to check if an object is empty or not. For newer browsers, you can use plain vanilla JS and use the new "Object.keys" 🍦 But for older browser support, you can install the Lodash library and use their "isEmpty" method 🤖. const empty = {}; Object.keys(empty).length === 0 && empty.constructor === Object _.isEmpty(empty ...
23/8/2020 · Today I had the need to check if an object was empty. Since in JavaScript objects are compared by reference, we can’t do a simple comparison like this: const obj = {} if (obj === {}) { //no } The solution is to pass the object to the built-in method Object.keys() and to check if the object constructor is Object: const obj = {} Object.keys(obj).length === 0 && obj.constructor === Object It’s … Hi, in this tutorial, we are going to talk about How to compare or find differences between two arrays of objects in Javascript using the key properties. Compare Two Arrays of Objects. I was working on one of my client projects and I want to ingest some data back to the existing ElasticSearch Index. How to check whether a checkbox is checked in jQuery · ExpressionChangedAfterItHasBeenCheckedError: Expression has changed after it was checked. Previous value: 'ngIf: [object Object]'. Current value: 'ngIf: true' · How can I test whether a variable has a value in JavaScript
How Can I Check For An Empty Undefined Null String In
Null Value Is Converted To An Empty Object Issue 1428
How To Check If An Object Is Empty In Javascript
How Do I Test For An Empty Javascript Object Dev Community
Check That An Object Is Empty In Javascript
Nodejs Proto Amp Prototype Pollution Hacktricks
How Do I Test For An Empty Javascript Object Stack Overflow
Grouping Object Shorthand Properties
How To Create Modify And Loop Through Objects In Javascript
Check If An Array Is Empty Or Not In Javascript Geeksforgeeks
Typescript Empty Object For A Typed Variable Stack Overflow
How To Check For Empty Object In Javascript For Beginners
Javascript Object Have Keys But Object Keys Returns Empty
Comparing Objects Why Am I Getting Different Results Using
How To Create An Object From The Given Key Value Pairs Using
Esp32 Espruino Creating Simple Javascript Objects
Spreading A Realm Object Gives In An Empty Object Issue
Javascript Archives Page 2 Of 4 Creatifwerks
How To Check If Array Includes A Value In Javascript
How To Remove Empty Object From Json In Javascript Xpertphp
Nested Object Equals Check Js Code Example
Check If A Javascript Object Is Empty Dev Community
How To Check If Object Is Empty In Javascript Samanthaming Com
Top 10 Javascript Errors From 1000 Projects And How To
Javascript Check If Object Is Empty Pakainfo
0 Response to "26 Compare Empty Object Javascript"
Post a Comment