21 Function In Javascript With Parameters
This video will focus on arguments and parameters.. In the calculateBill() function from the previous video, we hardcoded the tax amount.. A best practice in JavaScript is to keep your code DRY, which stands for Don't Repeat Yourself.. calculateBill would not be useful if it could only calculate the value assuming the bill is $100 and a 15% tax rate, so we need to modify those hardcoded values. JavaScript function basics. A JavaScript (Node.js) function is an exported function that executes when triggered (triggers are configured in function.json). The first argument passed to every function is a context object, which is used for receiving and sending binding data, logging, and communicating with the runtime. Folder structure
Beginner Javascript Tutorial 7 Using Parameters With Functions
Example 3: Add Two Numbers. // program to add two numbers using a function // declaring a function function add(a, b) { console.log (a + b); } // calling functions add (3,4); add (2,9); Output. 7 11. In the above program, the add function is used to find the sum of two numbers. The function is declared with two parameters a and b.
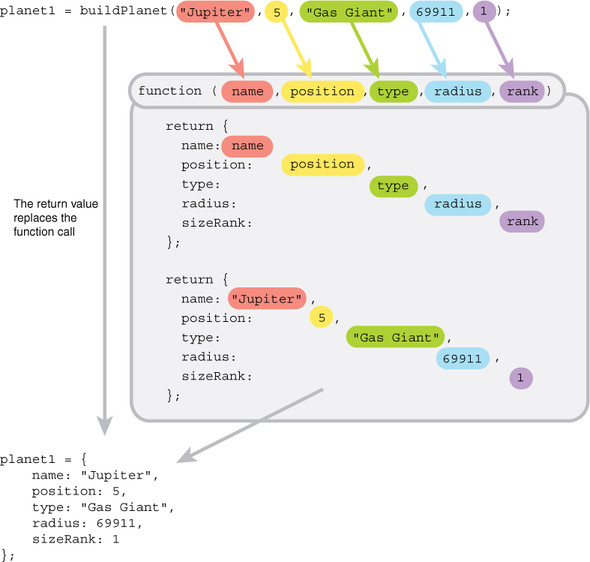
Function in javascript with parameters. Jul 20, 2021 - Conversely, non-strict functions that are passed rest, default, or destructured parameters will not sync new values assigned to argument variables in the function body with the arguments object. Instead, the arguments object in non-strict functions with complex parameters will always reflect ... Function parameters — For all other functions, the default return value is undefined . The parameters of a function call are the function's arguments. JavaScript Function with Parameters or Arguments. A function with parameters or arguments accepts some values when it is called. Basically arguments are the values that you pass to a function, which has corresponding parameters to store them. Note - Arguments are passed while calling a function.
Parameters & Arguments in JavaScript. Javascript Web Development Object Oriented Programming. Function parameters are the names of variables present in the function definition. Function arguments are the real values that are passed to the function and received by them. Following is the code showing parameters and arguments in JavaScript −. While parameters are used to pass one or more values into a JavaScript function, you can use a return value to pass a value out of the function as well. The function can use the return statement to exit and pass some value back to the caller when it's completed execution. If you click the save button, your code will be saved, and you get a URL you can share with others · By clicking the "Save" button you agree to our terms and conditions
7/11/2012 · function ToBeCalled(){ alert("I was called"); } function iNeedParameter( paramFunc) { //it is a good idea to check if the parameter is actually not null //and that it is a function if (paramFunc && (typeof paramFunc == "function")) { paramFunc(); } } //this calls iNeedParameter and sends the other function to it iNeedParameter(ToBeCalled); Name *. Email *. Website. Save my name, email, and website in this browser for the next time I comment. Get the CSS-Tricks newsletter. Copy and paste this code: micuno * 22 Aug 2021 — The rest parameter syntax allows a function to accept an indefinite number of arguments as an array, providing a way to represent variadic ...
Jul 07, 2020 - In JavaScript function is a block of code to perform a particular task. A function is group of reusable code that eliminates the… JavaScript Function and Function Expressions Example: Function as Parameter // program to pass a function as a parameter function greet() { return 'Hello'; } // passing function greet() as a parameter function name(user, func) { // accessing passed function const message = func(); console.log(`${message} ${user}`); } name('John', greet); name('Jack', greet); name('Sara', greet); Functions are values that can be called. One way of defining a function is called a function declaration. For example, the following code defines the function id that has a single parameter, x:. function id(x) {return x;}. The return statement returns a value from id. You can call a function by mentioning its name, followed by arguments in parentheses:
Using arguments variable JavaScript functions have a built-in object called arguments. It contains an array of parameters. The length of this array gives the number of parameters passed. A conditional statement is used to check the number of parameters passed and pass default values in place of the undefined parameters. Learn the basics of JavaScript Function Parameters. What if you have an unique object with parameters values in it? Once upon a time, if we had to pass an object of options to a function, in order to have default values of those options if one of them was not defined, you had to add a little bit of code inside the function: 7/3/2019 · Defaults Parameter: The default parameters is used to initialized the named parameters with default values in case of when no value or undefined is passed. Syntax: function Name(paramet1 = value1, paramet2 = value2 .. .) { // statements } Example: This example use default parameters and perform multiplication of numbers.
8/5/2020 · JavaScript Function Parameters. Pass different parameters while calling a function. These passed parameters can be captured inside the function and any manipulation can be done over those parameters. A function can take multiple parameters separated by comma. Following is the code for implementing function parameters in JavaScript −. Rest parameters are used to create functions that accept any number of arguments. The spread syntax is used to pass an array to functions that normally require a list of many arguments. Together they help to travel between a list and an array of parameters with ease. JavaScript allows us to write our own functions as well. This section explains how to write your own functions in JavaScript. Function Definition. Before we use a function, we need to define it. The most common way to define a function in JavaScript is by using the function keyword, followed by a unique function name, a list of parameters (that ...
The function keyword goes first, then goes the name of the function, then a list of parameters between the parentheses (comma-separated, empty in the example above, we'll see examples later) and finally the code of the function, also named "the function body", between curly braces. Nov 24, 2016 - From time to time, people ask things ... common with many libraries: $.post({ a: 'lot', of: 'properties', live: 'in', here: 'yep' }) But I would say this is not actually such a good idea. Let’s take a look at the best practices for defining function parameter lists in JavaScript... One interesting feature of the arguments object is that it aliases function parameters in non strict mode. What this means is that changing arguments[0] would change parameter1. Rest Parameters. Rest Parameter is an ES6 addition to JavaScript. To create a rest parameter prefix the last parameter in a function definition with ellipsis(…).
May 28, 2021 - In JavaScript, default function parameters allow you to initialize named parameters with default values if no values or undefined are passed into the function. All the functions can access arguments object by default instead of parameter names. A function can return a literal value or another function. A function can be assigned to a variable with different name. JavaScript allows you to create anonymous functions that must be assigned to a variable. In this tutorial learn about the Function Arguments, Parameters, and Argument Objects in JavaScript. We learned how to create a function in JavaScript. The parameters are the way we pass values to a JavaScript function. We learn the difference between argument and parameters, setting Default Parameters & learn about Argument Object.
Aug 20, 2016 - Using the rest parameter instead of the arguments object improves the readability of the code and avoids optimization issues in JavaScript. Nevertheless, the rest parameter is not without its limitations. For example, it must be the last argument; otherwise, a syntax error will occur: function ... A JavaScript function is defined with the function keyword, followed by a name, followed by parentheses (). Function names can contain letters, digits, underscores, and dollar signs (same rules as variables). The parentheses may include parameter names separated by commas: (parameter1, parameter2, ...) The code to be executed, by the function ... The returned value. The context this when the function is invoked. Named or an anonymous function. The variable that holds the function object. arguments object (or missing in an arrow function) This post teaches you six approaches to declare JavaScript functions: the syntax, examples and common pitfalls.
Function arguments !== function parameters. By now, it probably makes sense that function parameters and arguments are different things. However, this is not what I mean. What I actually mean is this: JavaScript doesn't check the number of parameters you define and the number of arguments you pass. Primitive parameters (such as a number) are passed to functions by value; the value is passed to the function, but if the function changes the value of the parameter, this change is not reflected globally or in the calling function.. If you pass an object (i.e., a non-primitive value, such as Array or a user-defined object) as a parameter and the function changes the object's properties, that ... Feb 02, 2016 - The method of passing in functions as parameters to other functions to use them inside is used in JavaScript libraries almost everywhere · A JavaScript Callback Function is a function that is passed as a parameter to another JavaScript function, and the callback function is run inside of the ...
How to pass an array as a function parameter in JavaScript ? Method 1: Using the apply () method: The apply () method is used to call a function with the given arguments as an array or array-like object. It contains two parameters. The this value provides a call to the function and the arguments array contains the array of arguments to be passed. 22 Aug 2021 — JavaScript Demo: Functions => ... Remove the word "function" and place arrow between the argument and opening body bracket (a) => { return a ... The parameters, in a function call, are the function's arguments. JavaScript arguments are passed by value: The function only gets to know the values, not the argument's locations. If a function changes an argument's value, it does not change the parameter's original value. Changes to arguments are not visible (reflected) outside the function.
Check out my courses and become more creative!https://developedbyed Getting Started With Javascript | Learn Javascript For BeginnersIn this video series w... In order for JavaScript functions to be able to do the same thing with different input, they need a way for programmers to give them input. The difference between parameters and arguments can be confusing at first. Here’s how it works: Parameters are the names you specify in the function ... For example, in the say() function, the message is the parameter. On the other hand, the arguments are values the function receives from each parameter at the time the function is called. In the case of the say() function, the 'Hello' string is the argument. Returning a value. Every function in JavaScript returns undefined, unless otherwise ...
In JavaScript, function parameters default to undefined. However, it's often useful to set a different default value. This is where default parameters can help. In the past, the general strategy for setting defaults was to test parameter values in the function body and assign a value if they are undefined. To write concise and efficient JavaScript code, you have to master the function parameters. In this post, I will explain with interesting examples of all the features that JavaScript has to efficiently work with function parameters. 1. Function parameters. A JavaScript function can have any number of parameters.
Html Call Javascript Function With String Parameter Code Example
Chapter 7 Object Arguments Functions Working With Objects
Declaring Javascript Functions
Simple Explanation Of Javascript Function Parameters Stack
Default Function Parameters In Javascript Es6
Introduction To Javascript Functions Part 3 Prezentaciya
Cssi 2 Javascript Functions Learn Co
Get Url Parameters With Javascript Chuvash Eu
How Do You Compose Javascript Functions With Multiple Parameters
What Are Argument And Parameter In Javascript With Examples
Working With Javascript In Visual Studio Code
Javascript Functions Understanding The Basics By Brandon
Viewing Method Parameter Information Webstorm
Javascript How To Pass An Array And Function As Argument Code
Javascript Function Parameters
Set Type For Function Parameters Stack Overflow
Functions In Javascript Tektutorialshub
0 Response to "21 Function In Javascript With Parameters"
Post a Comment