29 Create A Todo List App Javascript
Grab the value which is contained in the input field. Assuming that the input field isn't empty, we'll create a new object literal which will represent the task. Each task will have a unique id, a name, and be active (not completed) by default. Add this task to the tasks array. Store the array in local storage. How to build a Todo List App with JavaScript. Some people claim building a todo list app is a boring activity since so many exist already but I think it remains a great exercise for learning useful concepts that are widely applicable in many programming contexts. JavaScript projects for beginners (8 part series)
Build A Simple Todo App With React By Wilstaley
the to-do list we're going to create has a beautiful UI, the user can add a to-do by filling the input and hit ENTER, after that he can rather check the to-do when it's done, or remove it using the delete button. The user's to-do list is stored in the local storage, so when he refreshes the page, he can always find the list there.
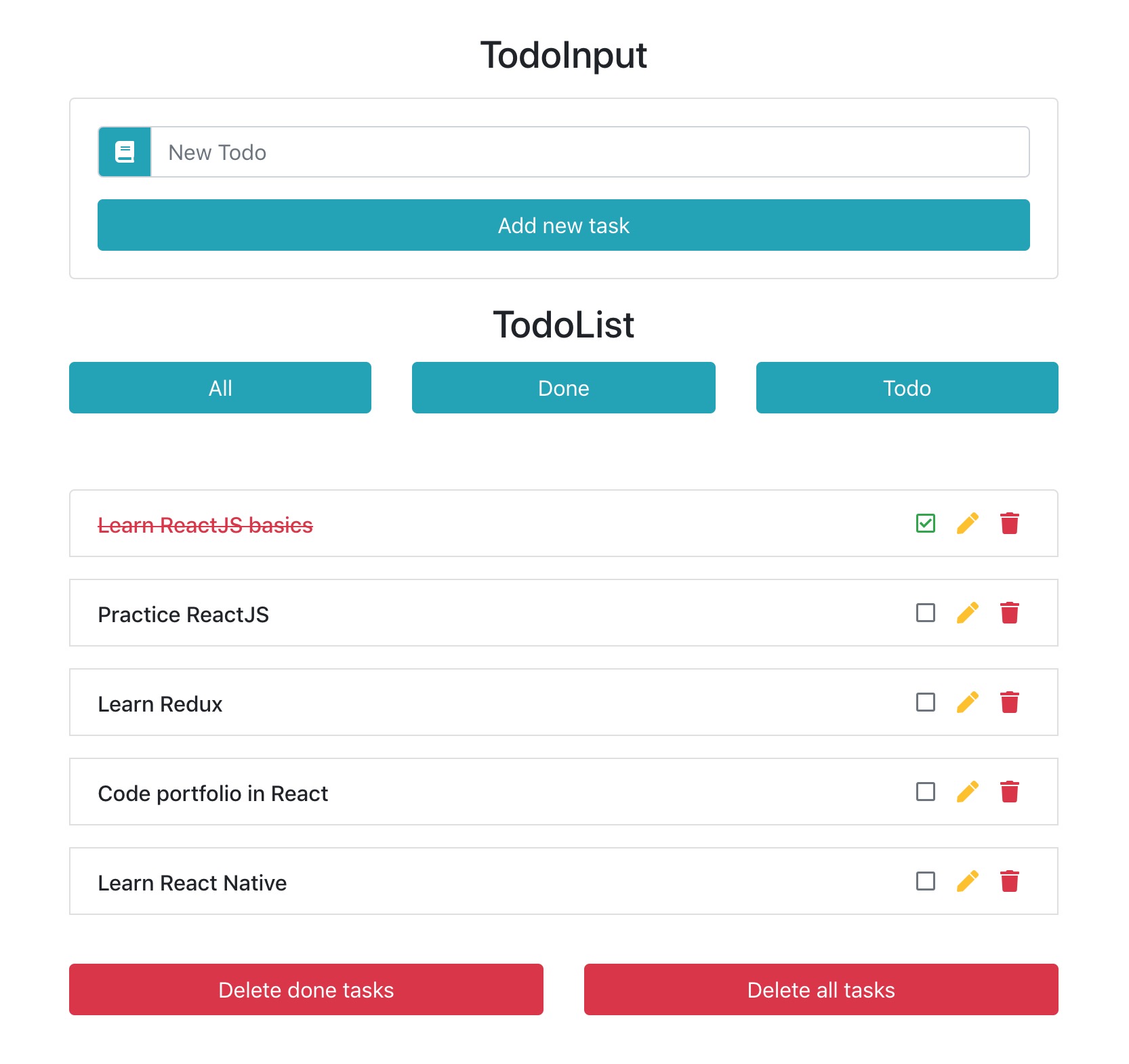
Create a todo list app javascript. Just like when adding a todo to the list, removing one will also require some steps to handle the data flow. First thing first, we need a function in app.js which removes a todo from the list. We create one and use the filter() function. The function returns a new list with all the elements that pass the test we add to the function. -----------------------------------------------------------------------------------------------------| BOOST YOUR PRODUCTIVITY - AFFILIATE ... Get the list values from database (or file) and read into an array (an array is like a train where each boxcar is a variable name containing data). Then, loop through the array to create the HTML to display your current list. (4) After creating HTML for the list, add a bit of HTML (perhaps a button with a label) to allow creating a new list item.
We will create a basic todo app to understand the basics of JavaScript. In this web app, one can create a todo list and can remove specific elements from the list. Features or Functionalities to implement: Interactive and Responsive design Just like Mead's Note App Project, this todo app project can be found in Andrew Mead's Modern JavaScript course. As I stated previously, this is great course that I first completed in March of this year, but I wanted to go through it again so I could complete all 3 of his projects, again. This todo application project is the project he builds ... 31/7/2020 · 2. Adding Event Listeners to elements. Now we will add click event listeners to our Buttons and Dropdown Filter. //event listeners todoButton.addEventListener("click", addTodo) todoList.addEventListener("click", deleteCheck) filterOption.addEventListener("click", filterTodo) The addEventListener() method attaches an event handler to the specified element.
6/7/2020 · // function to add todo function addTodo (item) {// if item is not empty if (item !== '') {// make a todo object, which has id, name, and completed properties const todo = {id: Date. now (), name: item, completed: false}; // then add it to todos array todos. push (todo); renderTodos (todos); // then renders them between <ul> // finally clear the input box value todoInput. value = '';}} 29/1/2021 · Introduction: TODO List are the lists that we generally use to maintain our day to day tasks or list of everything that we have to do, with the most important tasks at the top of the list, and the least important tasks at the bottom. It is helpful in planning our daily schedules. We can add more tasks any time and delete a task which is completed. The To Do List. Use CSS and JavaScript to create a "to-do list" to organize and prioritize your tasks. Try it Yourself » ...
Hey friends, today in this blog you'll learn how to create Todo List App using HTML CSS & JavaScript. In the previous blog, I've also shared how to create Todo App in JavaScript but in that program when you refresh your webpage, the lists or tasks that you've added are also removed. So today I came with another blog where I create Todo App and the lists or tasks that you add won't be removed ... In your editor/terminal create the following files: test/todo-app.test.js; lib/todo-app.js; index.html; These file names should be self-explanatory, but if unclear, todo-app.test.js is where we will write the tests for our Todo List App. todo-app.js is where all the JSDOCs and functions for our Todo List App will be written. Test Setup Your todo list should have projects or separate lists of todos. When a user first opens the app, there should be some sort of 'default' project to which all of their todos are put. Users should be able to create new projects and choose which project their todos go into.
React is an easy to use JavaScript framework that lets us create front end apps. In this article, we'll look at how to create a to-do list app with React and JavaScript. Create the Project. We can create the React project with Create React App. To install it, we run: npx create-react-app todo-list. with NPM to create our React project. The starter code will contain three files: index.html, scripts.js, and styles.css. Open the index.html file in a web browser to see the basic design of the ToDo List app, as shown in the preceding screenshot. The JavaScript file will be empty, in which we are going to write scripts to create the application. JavaScript Tutorials | Coding a Todo List in HTML & CSS & JavaScript (Using ES6)In this video, I will show how to code a Todo List using HTML & CSS & JavaScr...
Demo Download GitHub. A ToDo list app in Angular JS? Coding a todo list in HTML and JavaScript has always been difficult and tricky and a lot of developers struggeled doing it, let alone a responsive but since we're using AngularJS, dealing with "real time" actions is no more a problem and coding a todo list app takes no time and can be done with only a bunch of lines of code? Add items to the todo lit function addItemTodo(text) { // grab the `ul` let list = document.getElementById('todo') // create an `li` let item = document.createElement('li') // set the inside of our `li` the same as the parameter that we passed in the function, which is going to be the value set by the user in the input field item.innerText = text //create container for our buttons remove and ... Great! Now that our app is styled, we can begin using Vue to create a dynamic todo list. Displaying Our Todo List To display our todo list, we'll take advantage of Vue's 2-way data flow. Inside of our script tag, we'll use Vue's data object to create an array that will contain all our todo items.
After retrieving the current list of TODO items, we use the splice method to remove a specific element from the JavaScript array, and then we store the new list back the database. Then, just as in the add function we call the show function to update the list in the browser as well and we return false; to stop the propagation of the 'click' event. Beginning our React todo list. Let's say that we've been tasked with creating a proof-of-concept in React - an app that allows users to add, edit, and delete tasks they want to work on, and also mark tasks as complete without deleting them. This article will walk you through putting the basic App component structure and styling in place ... To make our to-do list usable, we need to write JavaScript. As we can see in the image above, we need to add a new task, make it available to the to-do section, move it to the completed-tasks section if it marked as complete, then delete all the visual display items from the to-do and the complete tasks after the clear button is clicked.
Todo List App in JavaScript [Source Codes] To create this program [Todo List App]. First, you need to create three files, HTML File, CSS File, and JavaScript File. After creating these files just paste the following codes into your files. 22 hours ago · Adding Items to the To-Do List. To add a task to the array, you need to push it to the todoArray and then display it on the webpage. For this to happen, a click event must be triggered on the add button. addTaskButton.addEventListener("click", (e) => {e.preventDefault(); let todo = localStorage.getItem("todo"); Create a todo app in the browser with plain JavaScript, and get familiar with the concepts of MVC (and OOP - object-oriented programming). View demo; View source; Note: Since this app uses the latest JavaScript features (ES2017), it won't work as-is on some browsers like Safari without using Babel to compile to backwards-compatible JavaScript ...
Within the todo-app directory, create a new file named index.js — this is where we will put most of our code. Server Setup : To get our server up and running we need to use Express . Now that we have a way to store state we can build a todo app. Each todo item has three checkboxes to store: whether the todo has been created; whether the todo has been marked as done; whether the todo has been deleted #1 might give you a clue of how the todo app will work. Without JavaScript we have no way to modify the DOM.
A Detailed Guide To Create A Simple Todo List With Plain
How To Build A Todo List With React Hooks
Build A To Do List App In Javascript
How To Build A Todo List App With Javascript
Todo List In Javascript With Source Code Video 2020
Tutorial Create Todolist App With Vue Js 2 For Beginners
How To Create Todo App Using Html Css Js And Bootstrap
Build A Todo App With Node Js Expressjs Mongodb And Vuejs
100 Javascript Projects For Beginners Solutions Provided
How To Build A To Do List With React Inventive Blog Inventive
A Detailed Guide To Create A Simple Todo List With Plain
How To Create Todo App Using Html Css Js And Bootstrap
How To Code Javascript Todo App
Create A To Do List App With Javascript Html And Css Javascript Project For Beginners
How To Build A Real Time Todo App With React Native
Todo List App Javascript By Panshak Solomon On Dribbble
A Detailed Guide To Create A Simple Todo List With Plain
Javascript Project How To Create A To Do List Using
Github Dwyl Javascript Todo List Tutorial A Step By
How To Build A Todo App With React Typescript Nodejs And
How To Build A Todo List App With Javascript
Build A To Do List Jamstack App Cloudflare Workers Docs
React Todo List App Tutorial Beginner React Js Project Using Hooks
Javascript Todo List With Source Code Source Code Amp Projects
Trying To Do A Filter For A To Do List App In Javascript But
Building A To Do List App With Vanilla Javascript By Linda
Github Va2 React Todo List Reactjs Todo List Project
0 Response to "29 Create A Todo List App Javascript"
Post a Comment