33 Reverse String In Javascript W3schools
slice () extracts a part of a string and returns the extracted part in a new string. The method takes 2 parameters: the start position, and the end position (end not included). This example slices out a portion of a string from position 7 to position 12 (13-1): Remember: JavaScript counts positions from zero. First position is 0. Definition and Usage. The lastIndexOf () method returns the position of the last occurrence of a specified value in a string. lastIndexOf () searches the string from the end to the beginning, but returns the index s from the beginning, starting at position 0. lastIndexOf () returns -1 if the value is not found. lastIndexOf () is case sensitive.
Web Basics Contents Q How The Web Works
Reverse a String in C - Reversing a string means the string that will be given by the user to your program in a specific sequence will get entirely reversed when the reverse of a string algorithm gets implemented in that particular input string. In the below-mentioned example, two approaches have been used to reverse a string in C language.
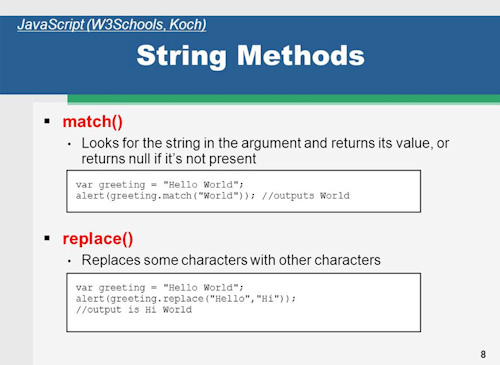
Reverse string in javascript w3schools. See the Pen JavaScript - Reverse a string - basic-ex-48 by w3resource (@w3resource) on CodePen. Improve this sample solution and post your code through Disqus Previous: Write a JavaScript program to check if a number in the range 40..10000 presents in two number (in same range). Reversing a String in JavaScript is a small and simple algorithm that can be asked on a technical phone screening or a technical interview. You could take the short route in solving this problem, or take the approach by solving it with recursion or even more complex solutions. I hope you found this helpful. Aug 08, 2018 - Quora is a place to gain and share knowledge. It's a platform to ask questions and connect with people who contribute unique insights and quality answers.
javascript string reverse w3schools; javascript code to reverse a string; reverse a string with no method in js; reverse string.js; how do you reverse a given string in place in javascript; string backwards javascript\ best way to reverse string in javascript; reverse string javascript Do you know; how to reverse a string in javascript w3schools JS String JS RegExp JS Functions JS Events DOM Objects DOM Window DOM Navigator DOM Screen DOM History ... JavaScript reverse() Method. Complete Array Object Reference. ... W3Schools is for training only. We do not warrant the correctness of its content. The risk from using it lies entirely with the user. StringBuilder reverse() in java with example program code : It (public StringBuilder reverse()) replace the string builder's character sequence by reverse character sequence.
Oct 31, 2011 - Multiple ways of completing a popular interview question. Here, the string that is given as input will be buffered using the StringBuffer, and it will be reversed using the reverse() method. Once the buffer is reversed, it will be converted to a string with the help of the toString() method. Converting String into Bytes: getBytes() method is used to convert the input string into bytes[]. Method: 1. Create a temporary byte[] of length equal to the length of the input string. 2. Store the bytes (which we get by using getBytes() method) in reverse order into the temporary byte[] .
string reverse in javascript w3schools code example JavaScript Program to Check Whether a String is Palindrome or Not In this example, you will learn to write a JavaScript program that checks if the string is palindrome or not. To understand this example, you should have the knowledge of the following JavaScript programming topics: Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
TypedArray reverse() JavaScript example program code : The Javascript TypedArray reverse() method reverses an array based on the index. Syntax: array.reverse() In JavaScript, there is no built-in method to reverse a string. There is however, a built-in method to reverse an array. So the first step is convert our string into an array. Split our string into an array of letters. The String to Reverse. txt = "Hello World" [::-1] print(txt) Create a slice that starts at the end of the string, and moves backwards. In this particular example, the slice statement [::-1] means start at the end of the string and end at position 0, move with the step -1, negative one, which means one step backwards.
This is a Java program which is used to find the reverse of a number. So in this program you have to first create a class name FindReverseNumber and within this class you will declare the main() method. Now inside the main() you will take two integer type variables. The following technique (or similar) is commonly used to reverse a string in JavaScript: // Don't use this! var naiveReverse = function (string) { return string.split ('').reverse ().join (''); } In fact, all the answers posted so far are a variation of this pattern. However, there are some problems with this solution. We have seen this implementation along with a recursive style to reverse a string. Recommended Articles. This is a guide to Reverse String in JavaScript. Here we discuss the examples of Reverse String in JavaScript using various loops such as (For Loop, While Loop, do While Loop). You may also have a look at the following articles to learn more -
May 27, 2013 - To answer your initial question — how to [properly] reverse a string in JavaScript —, I’ve written a small JavaScript library that is capable of Unicode-aware string reversal. It doesn’t have any of the issues I just mentioned. The library is called Esrever; its code is on GitHub, and ... W3Schools is optimized for learning and training. Examples might be simplified to improve reading and learning. Tutorials, references, and examples are constantly reviewed to avoid errors, but we cannot warrant full correctness of all content. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
REVERSE() FUNCTION The MySQL REVERSE function is used to reverse the order of the position of the characters in a string. The various versions of MySQL supports the REVERSE function, namely, MySQL 5.7, MySQL 5.6, MySQL 5.5, MySQL 5.1, MySQL 5.0, MySQL 4.1, MySQL 4.0 and MySQL 3.23. The strrev() function reverses a string. ... Get certified by completing a course today! ... If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail: ... Thank You For Helping Us! Your message has been sent to W3Schools. ... HTML Tutorial CSS Tutorial JavaScript ... javascript flip operation string. js function to reverse a string. Try to reverse string using the split, reverse, and join methods. reverse two letters in javascript. javascript array has a reverse method to reverse any string. javascript string has a reverse method to reverse any string.
Java Examples - String Reverse, How to reverse a String? Problem Description. How to reverse a String? Solution. Following example shows how to reverse a String after taking it from command line argument .The program buffers the input String using StringBuffer(String string) method, reverse the buffer and then converts the buffer into a String with the help of toString() method. Well organized and easy to understand Web bulding tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, PHP, and XML. Jul 24, 2020 - Write a function called reverseString that accepts a string and returns a reversed copy of the string. ... Unclosed regular expression. (E015)jshint(E015) javascript remove characters from beginning of string
The String to Reverse. txt = "Hello World" [::-1] print(txt) Create a slice that starts at the end of the string, and moves backwards. In this particular example, the slice statement [::-1] means start at the end of the string and end at position 0, move with the step -1, negative one, which means one step backwards. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. In this video series, Stephen Mayeux (http://stephenmayeux ) walks through the algorithmic challenges at Free Code Camp.Method chaining. `split`, `reverse...
Definition and Usage. The split () method splits a string into an array of substrings, and returns the new array. If an empty string ("") is used as the separator, the string is split between each character. The split () method does not change the original string. The JavaScript Certificate documents your knowledge of JavaScript and HTML DOM. More control flow tools in Python 3. python by Distinct Dragonfly on Jun 11 2020 Donate . Given a number, and we have to calculate its square in Python. Write a Python program to reverse a string. def reverse(i): ... In the above program, the built-in methods are used to reverse a string. First, the string is split into individual array elements using the split () method. str.split ("") gives ["h", "e", "l", "l", "o"]. The string elements are reversed using the reverse () method. arrayStrings.reverse () gives ["o", "l", "l", "e", "h"].
The reverse () method comes then into play and does the main job: it simply reverses the elements contained in the originally array so that what originally was the last character in the -String array, is now the first element (in our case it's a ".", since it's the character that our variable "stringToReverse" was ending on). If the first element within the array matches value, $.inArray returns 0. "js reverse string functions w3schools" Code Answer's javascript reverse a string javascript by Grepper on Aug 05 2019 Donate Comment There are three ways in which you can create a String in TypeScript. Note: It returns the complete string if … The parameter passed to the split method is an empty string with no spaces in it. In the next step, we're reversing that array using JavaScript's native reverse method which reverses the elements in the array. And in the final step, we're joining the array elements i.e. characters of the string using the join method.
Nov 25, 2016 - Reversing a string is one of the most frequently asked JavaScript question in the technical round of interview. Interviewers may ask you to write different ways to reverse a string, or they may ask… Apr 16, 2019 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
How To Reverse A String In Javascript Using For Loop Code Example
Github Vanbumi Javascript Tutorial Belajar Sendiri Javascript
Features Of Javascript Top 10 Characteristics Amp Comments Of
Protecting Legacy Apis With An Asp Net Core Yarp Reverse
2d Array Javascript W3schools Code Example
Three Ways To Reverse A String In Javascript
Reverse String In Javascript 4 Ways To Reverse A String In
Reverse String In Javascript Using Reduce Method Stack Overflow
Pragmatic Python Ii The Building Blocks Of Python By Shuvo
Jquery Validation W3schools Code Example
Javascript Attacks Ethical Hacking And Penetration Testing
How To Reverse A String In Javascript Samanthaming Com
Strings And Regular Expressions Ppt Download
Java Program To Reverse A String Using Recursion
Web Basics How To Capitalize A Word In Javascript Dev
Java Program To Reverse Words In String Reverse Only Words
How To Reverse A String In Javascript Step By Step
3 Interview Solutions To Reverse String In Javascript Codez Up
Advanced Palindrome Interview Question Javascript Hello Dev
Time Counter In Javascript W3schools Code Example
How To Reverse A String In Javascript Samanthaming Com
Python Machine Learning Decision Tree
Logical Interview Questions And Answers In Javascript
Java Script Part 2 1 Arithmetic Operators When
0 Response to "33 Reverse String In Javascript W3schools"
Post a Comment