35 Javascript Datetime From String
Split Date-Time column into Date and Time variables in R. 02, Jun 21. How to check the input date is equal to today's date or not using JavaScript ? 29, Jan 20. ... How to convert image into base64 string using JavaScript ? 09, Apr 21. How to convert a string into a integer without using parseInt() function in JavaScript ? Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
C Datetime Is Generated As String In Javascript
You can get the current date and time in JavaScript using the Date object. // the following gives the current date and time object new Date() Using the date object you can print the current date and time. let current_datetime = new Date() console.log(current_datetime.toString())
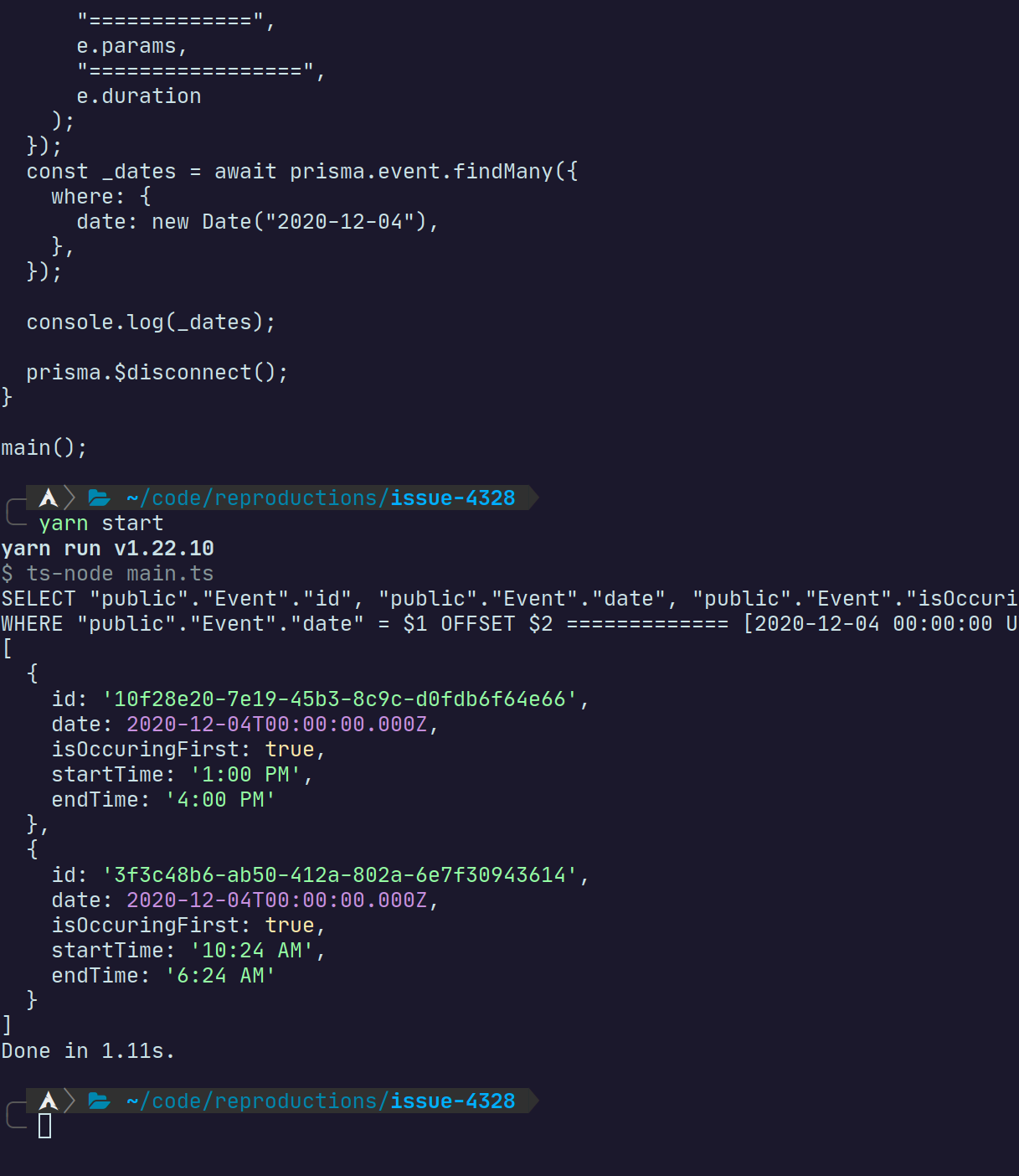
Javascript datetime from string. Get a date and time from a string with Moment.js. Parsing a date from a string with Moment.js is easy, and the library accepts strings in the ISO 8601 or RFC 2822 Date Time format, along with any string accepted by the JavaScript Date object. ISO 8601 strings are recommended since it is a widely accepted format. Here are some examples: From ISO strings. const date = new Date('2018-04-20T12:00:00Z') Note that JavaScript doesn’t “store” timezones in a date object. All these date objects, when expressed via .toString () or similar, will show the local timezone of the browser, regardless if you parsed UTC dates. Aug 25, 2020 - javascript new date() Expected String with value "Fri Aug 14 2020 14:05:58 GMT+0200 (heure d’été d’Europe centrale)", got Date
10/4/2011 · If you want to convert from the format "dd/MM/yyyy". Here is an example: var pattern = /^ (\d {1,2})\/ (\d {1,2})\/ (\d {4})$/; var arrayDate = stringDate.match (pattern); var dt = new Date (arrayDate [3], arrayDate [2] - 1, arrayDate [1]); This solution works in IE versions less than 9. Share. Apr 03, 2021 - This tutorial lists out various ways in Javascript to convert a string into a date. May 23, 2013 - Still don't know why (perhaps the replacement string is just a place holder for a separator). The 'g' means all occurrences. The pattern you are thinking of is /[-]+/g – Gerard ONeill Dec 21 '16 at 17:40 ... You made my day. I've been searching for days for a simple way to bypass the horrible TimeZone handling in Javascript...
Displaying Date & Time as per the Localization. The toLocaleString() method of the Date object return a date-time string as per the localization. It accepts 2 parameters which can be used to change the format of the date-time string : locales: This parameter accepts a language code of the form "en-US", "en-GB", "ja-JP" etc. For example if ... JavaScript Date instances inherit from Date.prototype. You can modify the constructor's prototype object to affect properties and methods inherited by JavaScript Date instances You can make use of the Date prototype object to create a new method which will return today's date and time. JavaScript doesn't have a date literal, and for this reason JSON serializes dates as strings rather than real JavaScript dates. In this post I show how JSON date serialization works, a few approaches how you can manage dates and how to automate the process of converting JSON dates to 'real' dates more easily.
Jul 20, 2021 - The toString() method returns a string representing the specified Date object. JavaScript Date: Create, Convert, Compare Dates in JavaScript. JavaScript provides Date object to work with date & time, including days, months, years, hours, minutes, seconds, and milliseconds. Use the Date () function to get the string representation of the current date and time in JavaScript. Use the new keyword in JavaScript to get the Date ... Epoch time was chosen as a standard for computers to measure time by in earlier days of programming, and it is the method that JavaScript uses. It is important to understand the concept of both the timestamp and the date string, as both may be used depending on the settings and purpose of an application.
In JavaScript, a time stamp is the number of milliseconds that have passed since January 1, 1970. If you don't intend to support <IE8, you can use Date.now() to directly get the time stamp without having to create a new Date object. Parsing a Date. Converting a string to a JavaScript date object is done in different ways. JavaScript only has a Date object, which is misnamed since it is really a date+time. Which means it cannot parse a time string HH:mm:ss without a date, but it can parse a date string. There are a few options. Convert time to a datetime string and parse using Date (). Use Momentjs String + Format parsing function. Sep 07, 2014 - Not the answer you're looking for? Browse other questions tagged javascript string date datetime type-conversion or ask your own question.
19 hours ago - Display Format Time from now Time from X Time to now Time to X Calendar Time Difference Unix Timestamp (milliseconds) Unix Timestamp (seconds) Days in Month As Javascript Date As Array As JSON As ISO 8601 String As Object As String Inspect Aug 03, 2020 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. The parse() method takes a date string (such as "2011-10-10T14:48:00") and returns the number of milliseconds since January 1, 1970, 00:00:00 UTC. This function is useful for setting date values based on string values, for example in conjunction with the setTime() method and the Date object.
The best format for string parsing is the date ISO format with the JavaScript Date object constructor. But strings are sometimes parsed as UTC and sometimes as local time, which is based on browser vendor and version. It is recommended is to store dates as UTC and make computations as UTC. To parse a date as UTC, you should append a Z: 1 week ago - Interactive API reference for the JavaScript Date Object. An object that represents a date and time. Internally the time is stored as the number of milliseconds since 01 January, 1970 UTC. Use new Da Date and time in JavaScript are represented with the Date object. We can't create "only date" or "only time": Date objects always carry both. Months are counted from zero (yes, January is a zero month). Days of week in getDay () are also counted from zero (that's Sunday).
The Date object is used to work with dates and times. Date objects are created with new Date (). There are four ways of instantiating a date: var d = new Date (); getDate() - Provides the day of the month values 1-31. getMonth() - Provides the current month with 0-11 values (0 for Jan and 11 for Dec). You should add +1 to get result. getFullYear() - Provides the current year. Here's the full code: For this format (assuming datepart has the format dd-mm-yyyy) in plain javascript use dateString2Date. [ Edit] Added an ES6 utility method to parse a date string using a format string parameter (format) to inform the method about the position of date/month/year in the input string.
Nov 09, 2011 - The querist asked for specifying a format string. ... Highly active question. Earn 10 reputation (not counting the association bonus) in order to answer this question. The reputation requirement helps protect this question from spam and non-answer activity. Not the answer you're looking for? Browse other questions tagged javascript datetime ... How can I convert a string to a date time object in javascript by specifying a format string? I am looking for something like: If you want a datetime string set to some other date & time (not the current time), pass an appopriate parameter to the Date object. See Several ways to create a Date object for more. 3) Combine both DateTime and Timezone Offset Concatenate datetime and timezone offset to get an ISO-8601 datetime string.
JavaScript will (by default) output dates in full text string format: Try it Yourself » When you display a date object in HTML, it is automatically converted to a string, with the toString() method. The toString() method converts a Date object to a string. Note: This method is automatically called by JavaScript whenever a Date object needs to be displayed as a string. 10/12/2018 · export class DateExample { date: string ="2018-12-04T12:02:56"; } In simple format, the date is 12/04/18 12:02:56 PM. The intended timezone is PST. At the server side, .NET serializes the value into a DateTime object with the timezone (System.DateTime.TimeKind) as unspecified.
5/2/2020 · The Intl object in JavaScript is the home for methods and constructors of the ECMAScript Internationalization API. This API has a number of convenient features for converting strings, numbers, and dates. Intl.DateTimeFormat is part of Intl and in this post we'll see how it can help with date formatting. A quick introduction to ISO strings Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. The toLocaleDateString() method returns a string with a language sensitive representation of the date portion of this date. The new locales and options arguments let applications specify the language whose formatting conventions should be used and allow to customize the behavior of the function. In older implementations, which ignore the locales and options arguments, the locale used and the ...
Sep 04, 2020 - Date is weird in JavaScript. It gets on our nerves so much that we reach for libraries (like Date-fns and Moment) the moment (ha!) we need to work with The.format () method constructs a string of tokens that refer to a particular component of date (like day, month, minute, or am/pm). Date.prototype.toISOString () The toISOString () method returns a string in simplified extended ISO format ( ISO 8601 ), which is always 24 or 27 characters long ( YYYY - MM - DD T HH: mm: ss.sss Z or ±YYYYYY - MM - DD T HH: mm: ss.sss Z , respectively). The timezone is always zero UTC offset, as denoted by the suffix " Z ".
Formatting time. The toLocaleTimeString() method also accepts two arguments, locales and options. These are some available options. timeZone : Iana time zones List hour12 : true or false.(true is 12 hour time ,false is 24hr time) hour: numeric, 2-digit. minute: numeric, 2-digit. second: numeric, 2-digit. Date.prototype.toLocaleTimeString () The toLocaleTimeString () method returns a string with a language-sensitive representation of the time portion of the date. The newer locales and options arguments let applications specify the language formatting conventions to use. These arguments can also customize the behavior of the function. Oct 05, 2019 - For custom-delimited date formats, you have to pull out the date (or time) components from a DateTimeFormat object (which is part of the ECMAScript Internationalization API), and then manually create a string with the delimiters you want.
Returns a string with a locality-sensitive representation of the time portion of this date, based on system settings. Date.prototype.toString() Returns a string representing the specified Date object. Overrides the Object.prototype.toString() method. Date.prototype.toTimeString() Returns the "time" portion of the Date as a human-readable string. Omitting T or Z in a date-time string can give different results in different browsers. Time Zones When setting a date, without specifying the time zone, JavaScript will use the browser's time zone.
Error Provided String Expected Datetime Or
How To Convert String Array To Datetime Array Help
Dart Flutter Convert String To Datetime Woolha
How To Convert Time String Into 24 Hrs Format Studio
Simple Date And Time Selector Using Pure Javascript Dtsel
How To Convert A String In Mm Dd Yyyy Into Dd Mm Yyyy In
3 2 3 6 Datetime Control Documentation Processmaker
Input Type Datetime Local Gt Html Hypertext Markup
Convert String Into Date Using Javascript Geeksforgeeks
How To Convert Javascript Datetime To Mysql Datetime
Pandas To Datetime String To Date Pd To Datetime Data
Create Date From Mysql Datetime Format In Javascript By
How Do You Display Javascript Datetime In 12 Hour Am Pm
Parse And Format Datetime Strings Using Php Datetime Formats
Setting Default Value To Datetime Field In Lightning Sfdc Panda
Convert Date Objects Into Iso 8601 Utc Strings Isoformat
How To Convert Date To Iso String In Javascript Code Example
Learn How To Get Current Date Amp Time In Javascript
Converting A String To A Date In Javascript Stack Overflow
Convert String To Datetime Var Help Uipath Community Forum
Using Python Datetime To Work With Dates And Times Real Python
Sql Server Functions For Converting A String To A Date
Jquery Date Format Plugins Jquery Script
3 2 3 6 Datetime Control Documentation Processmaker
Convert Datetime To Yyyy Mm Dd Js Code Example
Javascript Extract Date From String Code Example
How To Pass Map Date Object From Javascript To Datetime
Understanding About Rfc 3339 For Datetime And Timezone
How To Change String In To Datetime Type Import Export
Formatting Dates In Javascript With Intl Datetimeformat
Convert Datetime To String Js Code Example
How To Convert Utc Date Time Into Local Date Time Using
C Datetime Is Generated As String In Javascript
0 Response to "35 Javascript Datetime From String"
Post a Comment