31 Javascript Create Iframe Element
As you can see, there is a small inline JavaScript snippet that sets the iframe's document.domain to the domain of its parent. This solution does fix the accessibility issue in IE. However, it creates a new problem: the creation of the iframe adds a new entry to the browser's history. ... Using this solution, here's how the final IFrame ... 2) Define the main page, for example "main.html" (in the same folder on the server), in which write the code to include the IFrame and the JavaScript that will retrieve and modify the content of the IFrame.
The HTML <iframe> tag specifies an inline frame. The src attribute defines the URL of the page to embed. Always include a title attribute (for screen readers) The height and width attributes specifies the size of the iframe. Use border:none; to remove the border around the iframe.
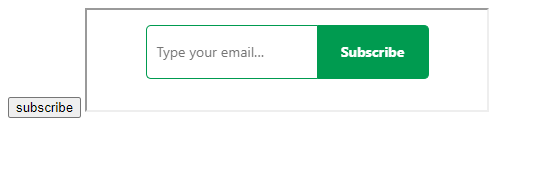
Javascript create iframe element. Given an HTML document containing an <iframe> element and the task is to change the height of the <iframe> element to 100% with the help of JavaScript. There are two methods to change the height of the iframe which are discussed below: Method 1: This method uses id attribute of iframe with height property to change the height of <iframe> element. In this tutorial, we'll show you how to use JavaScript to inject CSS into iFrame. In the snippet below, once everything was loaded, we'll get the iFrame element by ID. Then get the contentDocument and inject our styles into iFrame using innerHTML. window.onload = function() {. let myiFrame = document.getElementById("myiFrame"); The iframe in HTML stands for Inline Frame. The " iframe " tag defines a rectangular region within the document in which the browser can display a separate document, including scrollbars and borders. An inline frame is used to embed another document within the current HTML document. Iframe is basically used to show a webpage inside the ...
2. Anything within the iframe can be access by accessing the iframe's contentWindow property, but only if the iframe's content is from the same origin as the parent window. Once you're at contentWindow, access the element just like you would normally ( document.getElementBy...) Share. Improve this answer. In the code, firstly we identify our iframe element by id and assign it to a variable called myIframe, this later helps us to work with the iframe with ease. Then, we need to construct the url using our parameter values that are size (width x height) and geo in this example. Finally assign, the url to iframe object using the src property of iframe. HTMLIFrameElement.contentWindow. The contentWindow property returns the Window object of an HTMLIFrameElement. You can use this Window object to access the iframe's document and its internal DOM. This attribute is read-only, but its properties can be manipulated like the global Window object.
3) Creating a script element example. Sometimes, you may want to load a JavaScript file dynamically. To do this, you can use the document.createElement() to create the script element and add it to the document. The following example illustrates how to create a new script element and loads the /lib.js file to the document: Javascript Element How to - Create iframe and load its content. Back to iframe ↑ ... Definition and Usage. The <iframe> tag specifies an inline frame.. An inline frame is used to embed another document within the current HTML document. Tip: Use CSS to style the <iframe> (see example below). Tip: It is a good practice to always include a title attribute for the <iframe>.This is used by screen readers to read out what the content of the <iframe> is.
If your iframe is in the same domain as your parent page you can access the elements using window.frames collection. // replace myIFrame with your iFrame id // replace myIFrameElemId with your iFrame's element id // you can work on window.frames['myIFrame'].document like you are working on // normal document object in JS window.frames['myIFrame'].document.getElementById('myIFrameElemId') HTMLIFrameElement.src. Is a DOMString that reflects the src HTML attribute, containing the address of the content to be embedded. Note that programmatically removing an <iframe> 's src attribute (e.g. via Element.removeAttribute ()) causes about:blank to be loaded in the frame in Firefox (from version 65), Chromium-based browsers, and Safari/iOS. In the JavaScript code above, we have first used the document.createElement () function to create an iframe element. Then we have used the appendChild () function to append it to some DOM element on our HTML page, in this case a <div> tag with id attribute as myDIV.
tagName A string that specifies the type of element to be created. The nodeName of the created element is initialized with the value of tagName.Don't use qualified names (like "html:a") with this method. When called on an HTML document, createElement() converts tagName to lower case before creating the element. In Firefox, Opera, and Chrome, createElement(null) works like createElement("null"). The ultimate guide to iframes. January 23, 2020 10 min read 3014. The iframe element (short for inline frame) is probably among the oldest HTML tags and was introduced in 1997 with HTML 4.01 by Microsoft Internet Explorer. Even though all modern browsers support them, many developers write endless articles advising against using them. To get the element in an iframe, first we need access the <iframe> element inside the JavaScript using the document.getElementById () method by passing iframe id as an argument. const iframe = document.getElementById("myIframe");
var var_name = parent.iframe_name.element; - element - can be an object, a variable, or function in the " iframe_name ". Here's an example (explanations are in the code). 1) First, create a file named "ifr1.htm", that will represent the page included in the first IFrame. Approach 2: Create an empty image instance using new Image (). Then set its attributes like (src, height, width, alt, title etc). Finally, insert it to the document. Example 2: This example implements the above approach. <!DOCTYPE HTML>. The first line will create a reference to the select element that we will be using, for simplicity we doing it by ID in this tutorial.. The second line fetches the value of the currently selected option element, which will then be handed over to the iframe in the final and third line of code.. How to block framing of your own content
The <iframe> tag specifies an inline frame. It allows us to load a separate HTML file into an existing document. Code snippet: function getIframeContent(frameId) { var frameObj = document.getElementById(frameId); var frameContent = frameObj. JavaScript Create Element. Creating DOM elements using js, adding text to the new element and appending the child to the Html body is not a hard task, it can be done in just a couple of lines of code. Let's see in the next example: var div = document.createElement ('div'); //creating element div.textContent = "Hello, World"; //adding text on ... This element includes the global attributes. allow Specifies a feature policy for the <iframe>. The policy defines what features are available to the <iframe> based on the origin of the request (e.g. access to the microphone, camera, battery, web-share API, etc.). For more information and examples see: Using Feature Policy > The iframe allow ...
Click the button to hide the first H1 element in the iframe (another document). Hide H1 Element Creating an Iframe¶. The HTML <iframe> tag creates an inline frame for embedding third-party content (media, applets, etc.).. In this snippet, you can learn how to create a responsive iframe with CSS.First, let's see how a simple iframe looks like and then, we'll show how to make it responsive. Creating an IFRAME using JavaScript. Ask Question Asked 9 years, 7 months ago. Active 4 months ago. Viewed 151k times 41 11. I have a webpage hosted online and I would like it to be possible that I could insert an IFRAME onto another webpage using some JavaScript. ... How to add an iframe element into html dynamically? 1. Post login information ...
Lines 5 and 6 create a new paragraph element with some simple content, and then lines 8-12 handle inserting the new paragraph into the new document. Line 16 pulls the contentDocument of the frame; this is the document into which we'll be injecting the new content. The next two lines handle importing the contents of our new document into the ... The createElement () method creates an Element Node with the specified name. Tip: After the element is created, use the element.appendChild () or element.insertBefore () method to insert it to the document. Tip: You can also access an <iframe> element by using the window.frames property. Create an IFrame Object. You can create an <iframe> element by using the document.createElement() method:
Embedding An Uploaded Html Page In An Iframe Instructure
Working With Iframes In Cypress
100 Working Code Html Css Iframe Css Example How To
Using The Adoptnode Method With Embedded Iframes
How To Handle Iframes In Selenium Webdriver Switchto
How To Render A React Component Styled With Emotion In An
Using Testproject With Iframes Testproject Documentation
Github Videsk Iframex Iframe Generator With Dynamic
How To Embed Bubble In Wordpress Using An Iframe
Tracking Iframes How To Track Conversions In Iframes With Google Tag Manager
What Is An Iframe Or Inline Frame Rcs
Best Way To Use Iframe On Any Website Dev Community
How To Handle Iframes In Selenium Webdriver Switchto
It Doesn T Work Html And Javascript And Iframe Stack Overflow
Add Iframe To Div Using Javascript Dev Practical
How To Pass Url Parameters To Iframe In Javascript Step By Step
Embeds Video Audio And Iframe Elements Ilovecoding
How Can My Custom Ckeditor Plugin Properly Insert Special
Resize An Iframe Based On The Content Geeksforgeeks
How To Interact With Iframe Elements Learn With Examples
Tracking Iframes How To Track Conversions Through Iframes
Velo Working With The Html Iframe Element Help Center
Embedding Iframes Static Third Party And Dynamic Options
8 16 Iframe Tool Building And Running An Open Edx Course
Iframe In Action Center Form Html Element Content Forms
Html Tips And Tricks In Outsystems By Negin Nafissi Itnext
Dynamic Iframe Generator In Pure Javascript Iframex Css
Js Html Or Iframe Embed Code For Forms 123formbuilder
Securing Drift On Your Site With An Iframe
0 Response to "31 Javascript Create Iframe Element"
Post a Comment