20 How To Get Browser Size In Javascript
How to get window width and height using JavaScript # The dimensions of the browser window can determined using the followng width-height peoperties on the window object. window.innerWidth - width of the area within which the webpage is displayed window.innerHeight - height of the area within which the webpage is displayed Get viewport/window size (width and height) with javascript. While finding out monitor resolution with javascript can be useful for statistics and certain other applications, often the need is to determine how much space is available within the browser window. Space within the browser window is known as the 'viewport' affected by the monitor ...
19 Hidden Tricks Inside Apple S Safari Browser Pcmag
Feb 22, 2018 - If you wanted to have JavaScript that worked differently depending on how wide the viewport was, how would you handle it? There are a bunch of reasons why you might need to do this: JavaScript enhancements that are only applied on smaller viewports (or bigger ones), values that vary based on ...
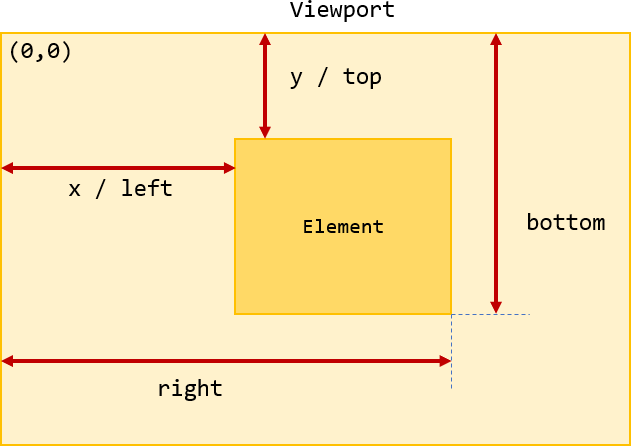
How to get browser size in javascript. Get the two different types of the window size. Get the window outer size; Get the window inner size; 1. Get the window outer size. window.outerWidth Gets the width of the outside of the browser window. window.outerHeight Gets the height of the outside of the browser window. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Jun 17, 2021 - The read-only innerHeight property of the Window interface returns the interior height of the window in pixels, including the height of the horizontal scroll bar, if present.
Jan 15, 2012 - Directly using .clientWidth is faster and equally compatible. The tables below compare these live against the inner/outer methods to determine the most accurate method: verge wins because it normalizes browser nuances to accurately match @media breakpoints. Device: How to get device size. The window resize event occurs whenever the size of the browser window gets changed. We can listen to the resize event in two ways: Using onresize event. Using Resize Observer API. Method 1: Using resize event: We can add an event listener to the body element which fires every time when the window size is resized. For width, you would probably want to look into the clientWidth method like document.body.clientWidth, here's a great resource: document.body and doctype switching. For height you may want to look into the clientHeight method, see the link above.. The values returned from the clientHeight and clientWidth methods will dynamically change as the browser is resized.
in this video, you will learn how to get screen size, window size, content size using javascript. I have used chrome browser in tutorial so results might var... This page requires JavaScript, sorry. ... Calculating... ... Try viewing the page in different browsers to see how each handles viewport size. Specifically, try it out in Internet Explorer 9, Firefox, Chrome for Windows, Safari for Windows, and Opera. Answer: Use the window.screen Object You can simply use the width and height property of the window.screen object to get the resolution of the screen (i.e. width and height of the screen). The following example will display your screen resolution on click of the button.
I'm trying to detect the browser's current size (width and height). I know it's super easy in jQuery with $(document).width and $(document).height , but I don't want to add the size of the jQuery lib to the project, so I'd rather just use built in JavaScript. 1 week ago - The read-only Window property innerWidth returns the interior width of the window in pixels. This includes the width of the vertical scroll bar, if one is present. In this tutorial, you'll learn how to get the user's screen (or browser) size using JavaScript. Get my free 32 page eBook of JavaScript HowTos 👉https://bit....
JavaScript provided on this page allows you to determine the size of the user's browser window, AKA the viewport dimensions. Two sets of properties are available for obtaining the width and height of the browser window: innerWidth and innerHeight, or clientWidth and clientHeight. Fortunately, you cannot move or resize a browser window your JavaScript code did not create. Imagine the chaos that could ensue if any random function could shrink your browser window to the size of a quarter. However, you can use built-in JavaScript functions to resize and reposition browser windows instantly. Example. <script>. /* Get the element you want displayed in fullscreen mode (a video in this example): */. var elem = document.getElementById("myvideo"); /* When the openFullscreen () function is executed, open the video in fullscreen. Note that we must include prefixes for different browsers, as they don't support the requestFullscreen method ...
Theoretically, as the root document element is document.documentElement, and it encloses all the content, we could measure the document's full size as document.documentElement.scrollWidth/scrollHeight. But on that element, for the whole page, these properties do not work as intended. Get dimensions of the entire browser window. Get dimensions of section of the browser where pages are displayed. Detect a change in window size. window.innerWidth & window.innerHeight gives the width & height of the viewport window. Jul 01, 2013 - While CSS if often enough, sometimes JavaScript is required. Currently, there are several outdated and incorrect ways to get the viewport size using JavaScript. Many people use the innerWidth of the browser window, while others use the clientWidth of the documentElement.
So yes, it contains general information like the browser, operating system, and other software technologies. We can use this for browser detection, and it is as simple as checking if the browser name is stuck somewhere inside the block of text. But take note - Users can choose to hide the user agent, and it is not a totally reliable method. Jan 16, 2012 - I want to provide my visitors the ability to see images in high quality, is there any way I can detect the window size? Or better yet, the viewport size of the browser with JavaScript? See green area here: ... What i do is, set an element usually html to 100% height and get its height. Instructions on how to enable (activate) JavaScript in web browser and why.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title>JavaScript Window Resize Event</title> </head> <body> <div id="result"></div> <script ... Selenium WebDriver supports getting the browser window size, resizing and maximizing window natively from its API, no JavaScript injections like window.resizeTo(X, Y); are necessary any more. Below shows the examples on how to achieve this in Selenium WebDriver C#, Ruby and Python bindings. Dec 19, 2019 - I want to provide my visitors the ability to see images in high quality, is there any way I can detect the window size? Or better yet, the viewport size of the browser with JavaScript? See green area here: ... What i do is, set an element usually html to 100% height and get its height.
I want to know what size browser window visitors to my site use (preferably; failing that, what their monitor resolution is). I want this info so that I can know what sort of resolution to display my photographs at. Any ideas? JavaScript. You can't do this server side.--Ian Collins. Screen resolution detection with Javascript. You can get the outer window size with window.outerWidth and window.outerHeight. You can get the inner window size with window.innerWidth and window.innerHeight. With IE you can get it with document.body.clientWidth and document.body.clientHeight. How to get the screen width and height using JavaScript #. The screen resolution of a device can be determined using the screen object. screen.width returns the width of the screen, while screen.height returns the height of the screen. You may want to determine a user's screen size for various purposes. Eg: extended features for users with ...
Current Screen Size. Use window.innerWidth and window.innerHeight to get the current screen size of a page. This example displays the browser window's height and width (NOT including toolbars/scrollbars): The window outer size consists of the width and height of the entire browser window, including the address bar, tabs bar, and other browser panels. To access the outer window size, you can use the properties outerWidth and outerHeight that are available directly on the window object: To get the browser window size or screen size, we use window.innerHeight and window.innerWidth window object properties. These properties work in most of browsers but not in Internet Explorer versions 5, 6, 7, 8. So for Internet Explorer versions 5, 6, 7, 8 we use these following properties:
Using availHeight and availWidth. We can use the screen.availWidth and screen.availHeight properties to get the screen size of a device in pixels. Example: window.screen.availWidth; window.screen.availWidth; Note: The availWidth, availHeight properties excludes the device taskbar. If you want to get the total width and height of the user's ... You will be redirected to an JavaScript FAQ home page. If this does not happen automatically, feel free to click the above hyperlink In this article, we'll look at how to get the size of the screen, web page, or browser window with JavaScript. Use the window.screen Object to Get a Screen's Dimensions The window.screen object lets us get the height and width of the browser's screen with the height and width properties respectively. For instance, we can write:
screen is the object of window, but sometimes it might not work for all browsers. In that case we utilize the DOM. Using document.body you can get the height and width of the browser window. I have written the following function, which will allow you to get height and width from different browsers. In this tutorial, you will learn how to get the size of an image using JavaScript. I said the size of an image, so this could be a little bit confusing. Don't worry I am explaining. The size here refers to the height and width of an image. the user has too many toolbars in browser I don't want to show some footer image at the bottom of the screen. Simple question: how I determine my browser visible availible size? Unfortunately what I found is only screen.width and screen.height, which give monitor resolution. That's not what I need. Thanks in advance. Vadim
Looking for guides to enable JavaScript in other web browsers? Here are some guides for other web browsers like Chrome or Firefox. ... Get more features and better security. Read more Make a program that filters a list of strings and returns a list with only your friends name in it.javascript ... Allowed memory size of 1610612736 bytes exhausted (tried to allocate 4096 bytes) in phar:///usr/local/Cellar/composer/1.9.1/bin/composer/src/Composer/DependencyResolver/Solver.php ... For a recent project, I wanted to display the browser and version on the screen. I figured that this would be possible in JavaScript, so I went searching around. This led me to the User-Agent, "sniffing", and its wild and crazy history. I also read a bunch of articles on why serving different content to different browsers is a bad idea.
How To Get Screen Browser Size With Javascript
Solved Why Am I Getting This Error Message When Approving
Monitor Javascript Bundle Size Jake Trent
Chrome Edge Firefox Opera Or Safari Which Browser Is
Javascript How To Get The Window Width And Height Stack
Get Image Width And Height Using Javascript And Jquery Qa
How To Get The Height Of Device Screen In Javascript
Detect Device Browser Viewport Information Device Js Css
Javascript Get Image Width And Height Code Example
How To Get Screen Size Using Javascript
How To Get Browser Screen Width Height Size Jquery Forum
How To Get The Image Size Height Amp Width Using Javascript
Get The Size Of The Screen Current Web Page And Browser
Make Element Height Of Browser Window Stack Overflow
How To Get The Screen Window And Web Page Sizes In Javascript
Reduce Unused Javascript Gtmetrix
How Can I Resize My Scene To Be 100 Of The Window S Width
How To Get The Screen Window And Web Page Sizes In Javascript
Check If An Element Is Visible In The Viewport In Javascript
0 Response to "20 How To Get Browser Size In Javascript"
Post a Comment