30 Javascript Addition Not Concatenation
1 week ago - The addition assignment operator (+=) adds the value of the right operand to a variable and assigns the result to the variable. The types of the two operands determine the behavior of the addition assignment operator. Addition or concatenation is possible. The JavaScript Arithmetic Operators include operators like Addition, Subtraction, Multiplication, Division, and Modulus. All these operators are binary operators, which means they operate on two operands. The below table shows the Arithmetic Operators in JavaScript with examples. 10 % 2 = 0 (Here remainder is zero).
Why Does Javascript Handle The Plus And Minus Operators
Nov 25, 2011 - Strings being immutable, most string operations whose results are strings produce new strings. Therefore languages such as C# or Java whose string handling is similar to JavaScript’s have special classes that help with concatenating strings. For example, C# calls this class StringBuilder.
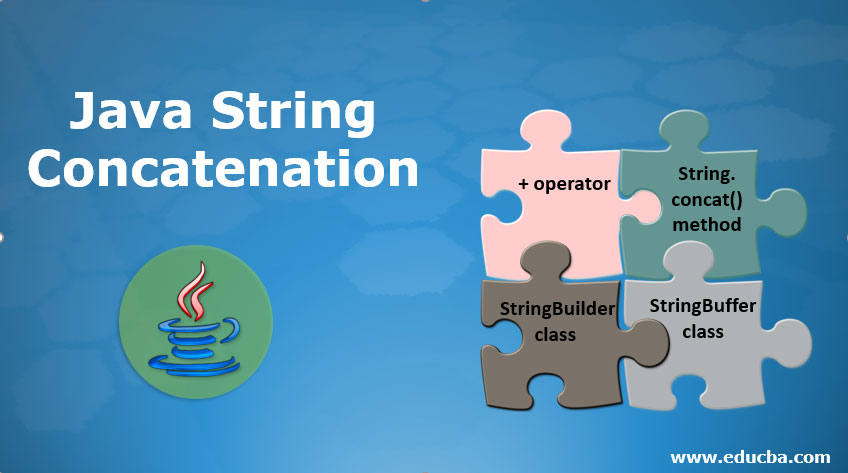
Javascript addition not concatenation. How To Calculate Two Numbers In JavaScript : sum, minus, duplication, divisionSource Code: http://1bestcsharp.blogspot /2017/01/javascript-addition-subtra... Sep 02, 2015 - Forgot your password · By Choeeey, February 9, 2015 in Phaser 2 JavaScript addition in javascript, divide in javascript, eval javascript example, multiply in javascript, school college javascript, simple javascript tutorials. agurchand. Technologist, software engineer, blogger with experience in Web development and the Media. More Posts Twitter Facebook LinkedIn.
JavaScript Program to find Matrix Addition: In the following example, we will add the two given matrices (two-dimensional arrays). In Javascript, you have to initialize the two dimensional array before using it, because it is technically impossible to create a 2d array in javascript. An arrow function expression is a compact alternative to a traditional function expression, but is limited and can't be used in all situations.. Differences & Limitations: Does not have its own bindings to this or super, and should not be used as methods. Does not have new.target keyword.; Not suitable for call, apply and bind methods, which generally rely on establishing a scope. Save Your Code. If you click the save button, your code will be saved, and you get a URL you can share with others.
This page lists over 100 JavaScript projects for beginners! There are no frameworks and each completed project includes the description, my summary, and the source files to download. We've compiled the list with over 100 JavaScript projects for beginners because the key to becoming a great JavaScript Developer is to practice, practice, practice. Basic math in JavaScript — numbers and operators. At this point in the course we discuss math in JavaScript — how we can use operators and other features to successfully manipulate numbers to do our bidding. Basic computer literacy, a basic understanding of HTML and CSS, an understanding of what JavaScript is. Sep 03, 2020 - The + sign concatenates because you haven’t used parseInt(). The values from the textbox are string values, therefore you need to use parseInt() to parse the value · After selecting the value from text box, you need to parse that value. Following is the code −
Javascript Tutorial: Addition, Subtraction, Multiplication, Division. Inventory Management System Source Code Using VB.NET And MySQL Database in this vb project demo we will see how to use our visu... To add 2 numbers in Javascript, get the values from the fields and parse them into integers before adding: var first = document.getElementById ("FIRST").value; var second = document.getElementById ("SECOND").value; var added = parseInt (first) + parseInt (second); That covers the basics, but let us walk through a few more examples in this guide. Sep 01, 2020 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
How to add JavaScript to html with javascript tutorial, introduction, javascript oops, application of javascript, loop, variable, objects, map, typedarray etc. It looks like you are new here. If you want to get involved, click on Join below · How to force addition instead of concatenation Javascript calculator with basic arithmetic operations. This is a simple javascript that performs basic arithemetic operations like Addition, Subtraction, Division, Multipication and Modulus. Just copy paste the below script in to an html file and run on any browser to see the working. Check out Advance Calculator With Memory Function Using ...
Add, Subtract, Multiply, and Divide in JavaScript. The following JavaScript program find and prints the addition, subtraction, multiplication, and division result of two numbers say 12 and 10 without getting input from user. This is just a demo program, that how these four mathematical operation performs using JavaScript code. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Dev #1 — Addition Function (JavaScript) ... To begin with, I want to say to you that every JavaScript engine that exists in the world already have the function to add two numbers implemented ...
2. I'm going to assume that float addition is sufficient, and if it isn't, then you'll need to dig deeper. function alwaysAddAsNumbers(numberOne, numberTwo){ var parseOne = parseFloat(numberOne), parseTwo = parseFloat(numberTwo); if (isNaN(parseOne)) parseOne = 0; if (isNaN(parseTwo)) parseTwo = 0; return parseOne + parseTwo;} Sum. Numbers in HTML with JavaScript and DOM. Write a JS function that reads two numbers from input fields in a web page and puts their sum in another field when the user clicks on a button. There will be no input/output, your program should instead modify the DOM of the given HTML document. JavaScript Add Two Numbers using Form and TextBox. This is the actual program to add two numbers in JavaScript using form and TextBoxes. As this program allows user to input the data. On the basis of user input, JavaScript code gets in action to output the addition result
JavaScript addition, subtraction, multiplication, division Examples. HTML complete example code for all basic athematic operations in JS (JavaScript). Users can choose or enter and select the option to calculate value dynamically. In the example we used bootstrap and jQuery. Let's have a more detailed view of each of these arithmetic operators in JavaScript. JavaScript Addition (+) permalink. This can be used to add two numbers, an example: const a = 5 + 2; // 7 const b = a + 3; // 10. However, be aware the plus sign is also used to combine strings, so doing something like this might surprise you: const a = '3' + 3 ... May 11, 2011 - It's a fairly common problem and doesn't just happen in JavaScript. The idea is that + can represent both concatenation and addition.
Good evening guys and girls! (At least it's evening time where I am in the world.) I'm pretty good at creating JS scripts that use inline JS however, this is totally wrong, evil and can sometimes ... Well, in the first case, when JavaScript detects a max overflow, it will just assign Number.MAX_VALUE, while in the second case, when JavaScript detects a min overflow, ... If the addition didn't overflow, the following is true: a == c-b and b == c-a. Download the source code from the github repository above. Delete the contents of the app.js file. Implement the JavaScript code in your own app.js file. If you think you need to see a video solution first, be sure to pick up Bluelime's 27 JavaScript Projects Beginner Course . Add a link to your finished project below!
Aug 07, 2011 - It's not bad to use + for both addition and concatenation, as long as you can only add numbers, and only concatenate strings. However, Javascript will automatically convert between numbers and strings as needed, so you have: What is Addition Assignment Operator (+=) in JavaScript? Javascript Web Development Front End Technology. It adds the right operand to the left operand and assigns the result to the left operand. May 22, 2017 - Not the answer you're looking for? Browse other questions tagged jquery scope concatenation addition or ask your own question. ... Total sum using javascript on.change function is showing 00.000.000.00, can anyone explain it and give a solution of it?
The parseInt () converts the numeric string value to an integer value. The for loop is used to find the sum of natural numbers up to the number provided by the user. The value of sum is 0 initially. Then, a for loop is used to iterate from i = 1 to 100. In each iteration, i is added to sum and the value of i is increased by 1. How to force addition instead of concatenation. in Getting Started • 8 years ago. I'm trying to add all of the calorie contents in my javascript like this: total = parseFloat (myInt1 + myInt2 + myInt3); $ ('#response').append (total); Instead of adding the variables they get concatenated. Javascript (+) sign concatenates ... type if not defined in HTML is considered as string. Because of this the Plus "+" operator is concatenating. Use parseInt(i) than the value of "i" will be casted to Integer. Than the "+" operator will work like addition. Object-Oriented JavaScript - Second Edition: ...
Dec 19, 2012 - Instead of adding the variables they get concatenated. I've tried using parseInt, parseFloat, and Number but I still just get concatenation and not addition. Please look at the view source at http://maureenmoore....121912_800.html Basic JavaScript Addition. The following code adds two numbers and stores the result in a variable named "sum": var x = 1; var y = 2; var result = x + y; The result is "3" in this simple example ... Assignment operators assign values to JavaScript variables. The addition assignment operator (+=) adds a value to a variable. ... Assignment operators are fully described in the JS Assignment chapter. ... The + operator can also be used to add (concatenate) strings.
1. The sync addition. Because the title of the post mentions an interesting explanation, I'm going to gradually explain async/await in regards to a greedy boss story.. Let's start with a simple (synchronous) function which task is to calculate the salary increase: 29/7/2019 · You can pass non-string parameters to concat(), but you will get a TypeError if str == null. // If `str` is null or not a string, can't use `concat()` const str = 'Values: '; // 'Values: 42 null' str.concat(42, ' ', null); The concat() function is rarely used because it has more error cases than the + operator. The class name attribute can be used by CSS and JavaScript to perform certain tasks for elements with the specified class name. Adding the class name by using JavaScript can be done in many ways. Using .className property: This property is used to add a class name to the selected element. Syntax: element.className += "newClass";
How do I safely host third-party Javascript code in an iframe? Why not offer credit by exam to students who have failed a course? How do I handle the wizard's familiar invalidating exploration, outshining the rogue, at low to no cost? A JavaScript function is defined with the function keyword, followed by a name, followed by parentheses (). Function names can contain letters, digits, underscores, and dollar signs (same rules as variables). The parentheses may include parameter names separated by commas: (parameter1, parameter2, ...) The code to be executed, by the function ... // Number + Number -> addition 1 + 2 // 3 // Boolean + Number -> addition true + 1 // 2 // Boolean + Boolean -> addition false + false // 0 String concatenation // String + String -> concatenation 'foo' + 'bar' // "foobar" // Number + String -> concatenation 5 + 'foo' // "5foo" // String + Boolean -> concatenation 'foo' + false // "foofalse"
Learn JavaScript Tutorial. Our JavaScript Tutorial is designed for beginners and professionals both. JavaScript is used to create client-side dynamic pages. JavaScript is an object-based scripting language which is lightweight and cross-platform.. JavaScript is not a compiled language, but it is a translated language. Feb 05, 2021 - And, while it's easy to concatenate two values of different types in JavaScript, it does not necessarily lead to an expected – or even useful – result. Some confusion stems from the fact that, in JavaScript, the concatenation operator is the same as that for addition, and so it must decide ... Feb 01, 2016 - Addition in JavaScript can sum numbers or concatenate strings. How not to get confused? Study the addition algorithm and follow the examples.
Addition is not working in JavaScript, One or both of the variables is a string instead of a number. This makes the + do string concatenation. '2' + 2 === '22'; // true 2 + 2 === 4; // true. In Javascript the + operator can either perform addition or concatenation depending on the type of its ... Nov 11, 2016 - As I'm sure you all know, in JavaScript the + symbol can be used both for numeric addition and string concatenation, depending on the variables either side. For example, If JavaScript has been disabled within your browser, the content or the functionality of the web page can be limited or unavailable. This article describes the steps for enabling JavaScript in web browsers. More Information Internet Explorer. To allow all websites within the Internet zone to run scripts within Internet Explorer:
How To Concatenate Strings In Javascript With Tabs And
Mysql Concat Function W3resource
How To Use Javascript Operator Code Leaks
Java String Concatenation 4 Useful Ways To Concatenate
Are Backticks Slower Than Other Strings In Javascript
Javascript Lesson 2 Variables Appending Strings Adding Numbers
Javascript Numbers Get Skilled In The Implementation Of Its
How To Concatenate Strings Javascript Code Example
How To Do Text Concatenation In Storyline Javascript Light
Sql Server Concatenate Operations With Sql Plus And Sql
String Interpolation In Javascript
Javascript Concat String How Does Concat Function Work In
String Concatenation Rosetta Code
The Problem Of String Concatenation And Format String
4 Ways To Combine Strings In Javascript Samanthaming Com
Strings In Powershell Replace Compare Concatenate Split
Javascript Concat String Does Not Work As Expected Stack
Applied Sciences Free Full Text Malicious Javascript
Splitting Concatenating And Joining Strings In Python
Add Letter To String Javascript Code Example
Concatenation Functions In Dataweave Apisero
Frd Cnn Object Detection Based On Small Scale Convolutional
Why Gives Object Object Object Object In
Why Is The Result Of B A A A Tolowercase
String Concatenation In Javascript String Concat Method
Python Concatenate Strings Step By Step Guide Career Karma
Concatenate Row Concatenation In Case Of Missing Data
0 Response to "30 Javascript Addition Not Concatenation"
Post a Comment