35 Javascript Add Class To Input
If you run the JavaScript above, you will see that "newClass" is added to "intro" after five seconds. It is important to note that the classList.add approach will not work in Internet Explorer version 9 and below. If you do need to support older browsers, then you can use the following approach instead: JavaScript is especially useful when you want to take user information and process it without sending the data back to the server. JavaScript is much faster than sending everything to the server to process, but you must be able to read user input and use the right syntax to work with that input.
Manage Tags Amp Attributes Word To Html
1 week ago - The className property of the Element interface gets and sets the value of the class attribute of the specified element.
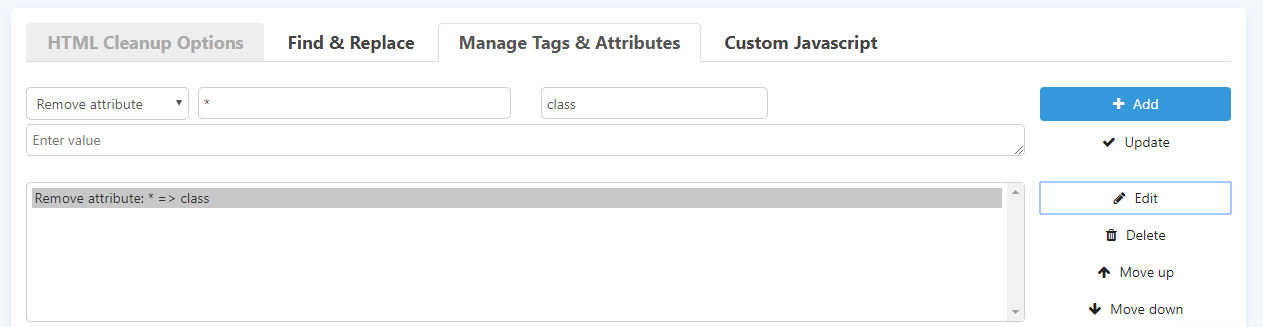
Javascript add class to input. Aug 26, 2009 - A function returning one or more space-separated class names to be added to the existing class name(s). Receives the index position of the element in the set and the existing class name(s) as arguments. Within the function, this refers to the current element in the set. The addEventListener () method allows you to add event listeners on any HTML DOM object such as HTML elements, the HTML document, the window object, or other objects that support events, like the xmlHttpRequest object. Example. Add an event listener that fires when a user resizes the window: window.addEventListener("resize", function() {. The constructor () method is a special method for creating and initializing objects created within a class. The constructor () method is called automatically when a class is initiated, and it has to have the exact name "constructor", in fact, if you do not have a constructor method, JavaScript will add an invisible and empty constructor method.
class Car {. constructor (name, year) {. this.name = name; this.year = year; } } The example above creates a class named "Car". The class has two initial properties: "name" and "year". A JavaScript class is not an object. The className property In HTML we can just say <span class='foo'>, but in JavaScript the word "class" is a reserved word, so it has to be called className instead: const element = document.getElementById('foo') element. className = 'my-class' Syntax: var elementVar = document.getElementById ("element_id"); elementVar.setAttribute ("attribute", "value"); So what basically we are doing is initializing the element in JavaScript by getting its id and then using setAttribute () to modify its attribute. Example: Below is the implementation of above approach. <!DOCTYPE html>.
Both the add() and remove() methods discussed above can be used for adding and removing multiple classes at a time. The below statement adds three classes — thorn , bud and sepal — to the <div/> element. May 01, 2013 - Javascript: Add class to required input from jQuery validate - gist:5494865 In modern JavaScript, it makes no sense to load the complete jQuery library just to do some simple DOM manipulations. In this article, you'll learn how to add, remove, and toggle CSS classes in vanilla JavaScript without jQuery. Using className Property. The simplest way to get as well as set CSS classes in JavaScript is by using the className ...
A function returning one or more space-separated class names to be added to the existing class name (s). Receives the index position of the element in the set and the existing class name (s) as arguments. Within the function, this refers to the current element in the set. version added: 3.3.addClass (function) There are following three ways in which users can add JavaScript to HTML pages. Embedding code; Inline code; External file; We will see three of them step by step. I. Embedding code:-To add the JavaScript code into the HTML pages, we can use the <script>.....</script> tag of the HTML In the example above, the addNewClass() function adds a new class highlight to the DIV element that already has a class box without removing or replacing it using the className property.
Input Text Object Properties. Property. Description. autocomplete. Sets or returns the value of the autocomplete attribute of a text field. autofocus. Sets or returns whether a text field should automatically get focus when the page loads. defaultValue. Sets or returns the default value of a text field. add (class) — applies a new class to the node. remove (class) — removes a class from the node. toggle (class) — removes or adds a class if it's applied or not applied respectively. We can ... Nov 06, 2020 - It demonstrates different ways to get input value in JavaScript
Code language: JavaScript (javascript) The document.createElement() accepts an HTML tag name and returns a new Node with the Element type. 1) Creating a new div example Change the class name of an element ... and add a new class name. ... Get certified by completing a course today! ... If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail: ... Thank You For Helping Us! Your message has been sent to W3Schools. ... HTML Tutorial CSS Tutorial JavaScript Tutorial How ... In this JavaScript program, we are going to learn how to take input from HTML form and print add (sum) of given numbers calculating through JavaScript? Submitted by Ridhima Agarwal, on October 18, 2017 Here, we are taking input from HTML form (using input tags) and printing the sum (addition) on the HTML by calculating it through JavaScript.
The problem with dynamically created elements, is that they aren't born with the same event handlers as the existing elements. Let's say we have a list of items that you could click on to toggle/add a class name, when a new element is created and appended to that same list - it won't work - the event handler attachment is missing. 24/9/2012 · You need a variable for the created element. var input = document.createElement ("input"); input.className = 'class_to_add'; document.forms [0].appendChild (input); JavaScript focus method is used to give focus to a html element. It sets the element as the active element in the current document. It can be applied to one html element at a single time in a current document. The element can either be a button or a text field or a window etc. It is supported by all the browsers. Syntax: HTMLElementObject.focus ...
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, PHP, and XML. Output. Enter the first number 5 Enter the second number 3 The sum of 5 and 3 is: 8. The above program asks the user to enter two numbers. Here, prompt () is used to take inputs from the user. parseInt () is used to convert the user input string to number. const num1 = parseInt(prompt ('Enter the first number ')); const num2 = parseInt(prompt ... 2 days ago - Use getElementsByClassName return a list/array of elements. Pick element through index and set value on input element. You can also iterate..
Learn how to add a class name to an element with JavaScript. Add Class. Click the button to add a class to me! Add Class. Step 1) Add HTML: Add a class name to the div element with id="myDIV" (in this example we use a button to add the class). Example. <button onclick="myFunction()">Try it</button>. <div id="myDIV">. JavaScript function add_feed () is the function which will add Input element dynamically to the working form after clicking in Add new link. Comments inside the function will describe the working of the function. Javascript function validate () is just to show you how you handle the input elements client-side. This property can be used to return the value of the class attribute of an element. We can use this property to add a class to an HTML element without replacing its existing class. To add multiple classes, we have to separate their name with space such as"class1 class2".
Apply class element. ... This JSX tag's 'children' prop expects a single child of type 'Element', but multiple children were provided. ... If para1 is the DOM object for a paragraph, what is the correct syntax to change the text within the paragraph? ... Showing results for div id javascript id ... You can use the URL of any other Pen and it will include the JavaScript from that Pen. ... You can apply a script from anywhere on the web to your Pen. Just put a URL to it here and we'll add it, in the order you have them, before the JavaScript in the Pen itself. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
20/11/2012 · 1. Add an onchange handler to your input. <input type="text" class="input-text required-entry validate-value-eg" id="city_field" name="city" onchange="addClass (this)" onfocus="if (this.value=='e.g. Bristol, Yorkshire') this.value='';" onblur="if (this.value=='') this.value='e.g. Bristol, Yorkshire';" value="e.g. Bristol, Yorkshire"> <script> ... Feb 14, 2019 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. Apply class to an element. Apply class element. ... using javascript when i ' m iterate localstorage current input value my DOM its Add multiple value or perivous value of localstorage ?
20/7/2021 · Adding the class name by using JavaScript can be done in many ways. Using .className property: This property is used to add a class name to the selected element. Syntax: element.className += "newClass"; Example: This example uses .className property to add class name. Now, lets add the javascript code to glue everything together: This method works by attaching an event listener to the textbox input element using jQuery and 'fire' on change, i.e when the user browses and selects a file(s). The addClass () method does not replace the existing class it simply adds or appends the new class within the element if it is not available. If you don't specify any class-name in the removeClass () method then it will remove all classes from the selected element. If you found this tutorial helpful then don't forget to share.
1a-quick-eg.html. First, we give the HTML element a unique id. Then select it with var element = document.getElementById (ID) in Javascript. Finally, take note that innerHTML can be used in two directions. When we do var contents = element.innerHTML, it will get the current contents of element. I have a dynamic form and every time the focus is on a particular field the label text moves above the field and stays there if a value ... This method is often used with .addClass() to switch elements' classes from one to another, like so: Here, the myClass and noClass classes are removed from all paragraphs, while yourClass is added.
Definition and Usage The classList property returns the class name (s) of an element, as a DOMTokenList object. This property is useful to add, remove and toggle CSS classes on an element. The classList property is read-only, however, you can modify it by using the add () and remove () methods. Add /Delete rows in HTML table with JavaScript. The script presented in this page it is a JavaScript object that can be used to Add and Delete dinamically rows with input fields in HTML table. - The new row in table is added directly after the clicked row. - The columns with rows index (ID) must have the class "tbl_id". Mar 24, 2020 - Get code examples like "add class to element by id javascript" instantly right from your google search results with the Grepper Chrome Extension.
Use setAttribute () Method to add the readonly attribute to the form input field using JavaScript. setAttribute () Method: This method adds the defined attribute to an element, and gives it the defined value. If the specified attribute already present, then the value is being set or changed. Syntax: Set the value property: textObject.value = text. Property Values: text: It specifies the value of input text field. attributeValue: This parameter is required. It specifies the value of the attribute to add. setAttribute method. This method adds the specified attribute to an element, and set it's specified value.
How Can I Add A Input Text If A Select Option Is Right
Working With Refs In React Css Tricks
Bootstrap 3 File Upload Component
Php Get Dynamic Add Input Javascript Js Frontier Html Page
How To Submit Ajax Forms With Jquery Digitalocean
Workarounds Manipulating A Survey At Runtime Using
Javascript Shopping List I Ve Beening Learning Javascript
Workarounds Manipulating A Survey At Runtime Using
Javascript Show Value In Input Field Code Example
How To Create A Password Validation Form
Github Jackocnr Intl Tel Input A Javascript Plugin For
Laravel Dynamically Add Or Remove Input Fields Using Jquery
Javascript Adding A Class Name To The Element Geeksforgeeks
Bootstrap Validation Examples Amp Tutorial
Javascript Add Class To Element Dynamically Code Example
How To Convert Html Form Field Values To A Json Object
Css Ready Classes For Gravity Forms Gravity Forms
The 10 Most Common Bootstrap Mistakes That Developers Make
Tutorial Add A Date Picker To A Bootstrap Form Formden Com
Textbox And Textarea Style Change On Focus Using Jquery
How To Build International Phone Number Input In Html And
Github Bytesnz Infocus A Super Simple Javascript To Add A
How To Build International Phone Number Input In Html And
Using Spotfire Text Areas To Increase Usability Of Analytics
Javascript Adding A Class Name To The Element Geeksforgeeks
Styling Amp Customizing File Inputs The Smart Way Codrops
Javascript Project How To Create A To Do List Using
Value Bubbles For Range Inputs Css Tricks
Css Ready Classes For Gravity Forms Gravity Forms
Ngclass How To Assign Css Classes In Angular Malcoded
Karandeep Singh On Twitter Introducing Rjs R In Javascript
0 Response to "35 Javascript Add Class To Input"
Post a Comment