30 Javascript Get Value Of Input
Can't Pass an Input Value Into a JavaScript Variable. Imagine the following scenario - you have a simple input and a button. When a user types into the input and presses the button, the text from the input should be logged to the console. Here's what you have so far: But when you load the page you see Uncaught ReferenceError: test is not ... Nov 18, 2020 - Input fields enable us to receive data from users. There are many types of input fields, and while getting their value is done similarly in each
Get Input Element Value On Click Using Javascript
The value property sets or returns the value of the value attribute of the radio button. For radio buttons, the contents of the value property do not appear in the user interface. The value property only has meaning when submitting a form. If a radio button is in checked state when the form is submitted, the name of the radio button is sent ...
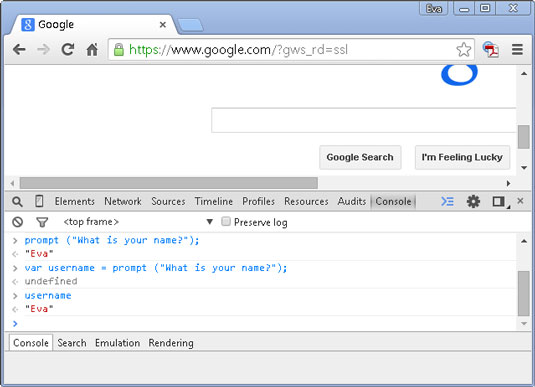
Javascript get value of input. If not, the value must be discarded and shows a message to tell users that input must be integer. After some searches, I found that parseInt() and isNaN() can be used to do the job. The return value of text input field is string. First try parseInt() to convert the value to integer. The value property sets or returns the value of the value attribute of a text field. The value property contains the default value OR the value a user types in (or a value set by a script). Get the single value from a single select and an array of values from a multiple select and display their values. ... Find the value of an input box.
1 week ago - The HTMLFormElement property elements returns an HTMLFormControlsCollection listing all the form controls contained in the element. You can set the initial value with the value attribute, but the value property contains the actual value of the control. If you want to get or set the initial value in JavaScript, use the defaultValue property. For the input:checkbox and the input:radio elements, the contents of the value property ... The value property contains the default value, the value a user types or a value set by a script. Syntax: Return the value property: textObject.value. Set the value property: textObject.value = text. Property Values: text: It specifies the value of input text field. attributeValue: This parameter is required.
HTMLElement: input event. The input event fires when the value of an <input>, <select>, or <textarea> element has been changed. The event also applies to elements with contenteditable enabled, and to any element when designMode is turned on. In the case of contenteditable and designMode, the event target is the editing host. Answer: Use the value Property You can simply use the value property of the DOM input element to get the value of text input field. The following example will display the entered text in the input field on button click using JavaScript. 8/9/2017 · The first one holds a code which will return the value of the property. JavaScript get value of input: textObject.value. The second example can be used for setting the value of the property. As you can see, it contains a value called text, indicating the value of the text input field: textObject.value = text.
3 weeks ago - The HTMLInputElement.select() method selects all the text in a element or in an element that includes a text field. Apr 16, 2020 - <!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title>Get Text Input Field Value in JavaScript</title> </head> <body> <input type="text" placeholder="Type something..." id="myInput"> <button type="button" onclick="getInputValue();">Get Value</button> <script> function ... To get the value of selected radio button, a user-defined function can be created that gets all the radio buttons with the name attribute and finds the radio button selected using the checked property. The checked property returns True if the radio button is selected and False otherwise.
The value of the textarea is reset only the first time the textarea field gets focus, by setting its value attribute to the empty string. Download the code. Setting the value of the text input element through JavaScript. In order to set the value of a text input field element in a form, we can use the following code: Jun 30, 2021 - <!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title>Get Text Input Field Value in JavaScript</title> </head> <body> <input type="text" placeholder="Type something..." id="myInput"> <button type="button" onclick="getInputValue();">Get Value</button> <script> function ... 15/4/2019 · We can get the value of text input field using various methods in script. There is a text value property which can set and return the value of the value attribute of a text field. Also we can use jquery val() method inside script to get or set the value of text input field. Using text value property: Syntax:
Use document.getElementsByClassName ('class_name') to Get Input Value in JavaScript Use document.querySelector ('selector') to Get Input Value in JavaScript We can get the value of input without wrapping it inside a form element in JavaScript by selecting the DOM input element and use the value property. May 31, 2021 - You can retrieve this using the ... in JavaScript. ... If no validation constraints are in place for the input (see Validation for more details), the value can be any text string or an empty string (""). ... In addition to the attributes that operate on all <input> elements regardless of their type, ... JavaScript - How to Get an Input’s Value with JavaScript Input fields enable us to receive data from users. There are many types of input fields, and while getting their value is done similarly in each case, it requires some thought to do well. Get the value of a text input. Here is a basic example. It creates a text input field, then prints the contents to the console. HTML <input type="text" placeholder="Enter …
The val () method returns or sets the value attribute of the selected elements. When used to return value: This method returns the value of the value attribute of the FIRST matched element. When used to set value: This method sets the value of the value attribute for ALL matched elements. Note: The val () method is mostly used with HTML form ... - JavaScript - Get selected value from dropdown list. About Mkyong . Mkyong is providing Java and Spring tutorials and code snippets since 2008. Apr 16, 2020 - This JSX tag's 'children' prop expects a single child of type 'Element', but multiple children were provided. ... If para1 is the DOM object for a paragraph, what is the correct syntax to change the text within the paragraph? ... Showing results for div id javascript id selector combine with ...
May 31, 2021 - The value attribute is a DOMString that contains the current value of the text entered into the text field. You can retrieve this using the HTMLInputElement value property in JavaScript. ... If no validation constraints are in place for the input (see Validation for more details), the value ... The prompt () method displays a dialog box that prompts the visitor for input. A prompt box is often used if you want the user to input a value before entering a page. Note: When a prompt box pops up, the user will have to click either "OK" or "Cancel" to proceed after entering an input value. Do not overuse this method, as it prevents the user ... Javascript access the dom elements by id, class, name, tag, attribute and it's valued. Here you will learn how to get HTML elements values, attributes by getElementById (), getElementsByClassName (), getElementByName (), getElementsByTagName (). Selecting Elements in Document
You can use onkeyup when you have more input field. Suppose you have four or input.then document.getElementById ('something').value is annoying. we need to write 4 lines to fetch value of input field. So, you can create a function that store value in object on keyup or keydown event. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. How to get value from an input control on a View (MVC) Ask Question Asked 4 years, 2 months ago. ... And when View loads I tried to get a value so I wrote this: ... How do I check if an array includes a value in JavaScript? 2315. How to get the children of the $(this) selector?
There are several methods are used to get an input textbox value without wrapping the input element inside a form element. Let’s show you each of them separately and point the differences. The first method uses document.getElementById('textboxId').value to get the value of the box: Hi I basically am trying to disable another input field if the day is set to today from another input field (date). I can't seem to force an onchange onto an input field and grab that value into a variable to make this work. In this article we demonstrate the use of JavaScript for accessing the values of form elements. Later, we will demonstrate all the concepts using a real world example. Text input element. To obtain a reference to a text input element, here is some sample code:
If you want to get the values of all checkboxes using jQuery, this might help you. This will parse the list and depending on the desired result, you can execute other code. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. This problem can be solved by using input tags having the same "name" attribute value that can group multiple values stored under one name which could later be accessed by using that name. To access all the values entered in input fields use the following method: var input = document.getElementsByName('array[]');
5 days ago - The HTMLInputElement interface provides special properties and methods for manipulating the options, layout, and presentation of elements. If the radio input does not have the same name attribute it will be independently selectable. In the example below only one of the following radio buttons can be selected because they all have the name attribute of day: ... To get the value of a radio button use the value JavaScript property: ... Well organized and easy to understand Web bulding tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, PHP, and XML.
The document.getElementById () method returns the element of specified id. In the previous page, we have used document.form1.name.value to get the value of the input value. Instead of this, we can use document.getElementById () method to get value of the input text. But we need to define id for the input field. How to get all checked checkbox value in JavaScript? A checkbox is a selection box that allows the users to make the binary choice (true or false) by checking and unchecking it. Basically, a checkbox is an icon, which is frequently used in GUI forms and application to get one or more inputs from the user. How do I get the current date in JavaScript? 2400. Get selected text from a drop-down list (select box) using jQuery. 2226. Get the size of the screen, current web page and browser window ... Get the value in an input text box. 1031. How do I get the value of text input field using JavaScript? 1708. Can't bind to 'ngModel' since it isn't a ...
Try and test HTML code online in a simple and easy way using our free HTML editor and see the results in real-time. Jul 30, 2021 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
C Java Php Programming Source Code Javascript Get
Get Value Of An Input Field From Web Form Iframe Studio
How To Prompt The User For Input In Javascript Dummies
Where Is My Input Current Value Stored Stack Overflow
How To Get The Value Of Text Input Field Using Javascript
Javascript Get Form Data Code Example
How To Set Value For Date Time Field By Javascript In Crm
Sum Html Textbox Values Using Jquery Javascript Summing
How To Get An Input Field Value In Javascript Reactgo
Get Value From Html Table Input Cell With Javascript Stack
How Get Total Sum From Input Box Values Using Javascript
How To Get An Input Value Using Refs In React Bootstrap
Modified Java Script Value Hitachi Vantara Lumada And
Get Value Of An Input Field From Web Form Iframe Studio
Angular 4 Get Input Value Stack Overflow
How To Get And Set Input Text Value In Javascript
How To Get Old Default Value Of An Input Field After Changed Html Javascript
Selecting Clearing And Focusing On Input In Javascript
How To Get The Value From Input Checkbox With Id Attribute
How To Get Html Textbox Value In Asp Net Dotnettec
Jquery Set The Value Of An Input Text Field Geeksforgeeks
Adf Faces And Client Side Value With Innerhtml By Andrej
Verify Input Value In Javascript Code Example
Get Input Element Value On Click Using Javascript
Javascript And It S Forms Getting And Setting Input Text
How To Prompt The User For Input In Javascript Dummies
How To Get Values From Html5 Input Type Range Using Javascript
How To Pass Variables From Javascript To Php Wikihow
0 Response to "30 Javascript Get Value Of Input"
Post a Comment