24 How To Use A Class In Javascript
If you're looking for JavaScript has class or JavaScript hasclass then there's a high probability that you used to work with jQuery in the past.. It's great news that you don't need a library anymore to check if an element has a class or not, because you can now simply do it with a call to classList.contains("class-name"). Here's an example. JavaScript classes initialize instances with constructors, define fields and methods. You can attach fields and methods even on the class itself using the static keyword. Inheritance is achieved using extends keyword: you can easily create a child class from a parent. super keyword is used to access the parent class from a child class.
What Are Javascript Classes And How To Use Them
Adding a CSS class to an element using JavaScript. Now, let's add the CSS class "newClass" to the DIV element "intro". For the purpose of this example, I have added a delay using the setTimeout () method so that you can see the style changing:
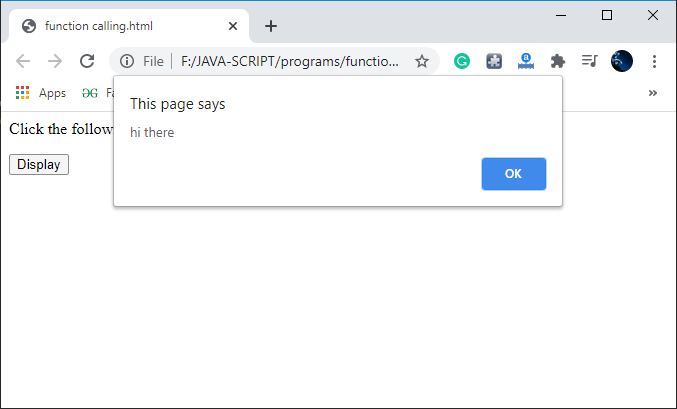
How to use a class in javascript. A class in JavaScript is created with the special word: function , using this syntax: className = function () { // code of the className class } A class can contain public and private variables (called also properties) and functions (also called methods). The private variables, and functions are defined with the keyword " var ". Array class in JavaScript: class Array {. constructor () {. this.length = 0; this.data = {}; } } In the above example, create a class Array which contains two properties i.e. length and data, where length will store the length of an array and data is an object which is used to store elements. Function in Array: There are many functions in array ... A JavaScript class will execute naturally if a constructor is not provided (the class will simply create an empty constructor during execution). However, if a JavaScript class is created with constructors and other functions but no class keyword is used, this class will not be executable.
Class methods are non-enumerable. A class definition sets enumerable flag to false for all methods in the "prototype". That's good, because if we for..in over an object, we usually don't want its class methods. Classes always use strict. All code inside the class construct is automatically in strict mode. In this article, we will learn how we can access an element (s) based on its class. GetElementsByClassName () method is used to retrieve a collection or array of all the HTML elements that are child nodes of the element on which this method is called and have the class as mentioned in the parameter of this method. Code language: JavaScript (javascript) The querySelectorAll() method returns a static NodeList of elements that match the CSS selector. If no element found, it returns an empty NodeList. Note that the NodeList is an array-like object, not an array object. However, in modern web browsers, you can use the forEach() method like the one in the ...
A JavaScript class is a type of function. Classes are declared with the class keyword. We will use function expression syntax to initialize a function and class expression syntax to initialize a class. const x = function() {} In JavaScript, there are some approaches to add a class to an element. We can use the.className property or the.add () method to add a class name to the particular element. Now, let's discuss the approaches to add a class to an element. In JavaScript, the class keyword is used to create a class. Classes in JavaScript use a method named constructor (). It automatically executes when creating a new object. It initializes the properties of the object.
The element's classList property returns the live collection of CSS classes of the element. Use add () and remove () to add CSS classes to and remove CSS classes from the class list of an element. Use replace () method to replace an existing class with a new one. In the other programming languages like Java or C#, a class can have one or more constructors. In JavaScript, a function can have one or more parameters. So, a function with one or more parameters can be used like a constructor where you can pass parameter values at the time or creating an object with new keyword. ES6 Class and Subclass Syntax. The above code has 2 JavaScript classes namely Animal and Gorilla.. The Gorilla class is a subclass or child class of Animal and it uses the extends keyword to set ...
Class Methods. Class methods are created with the same syntax as object methods. Use the keyword class to create a class. Always add a constructor() method. Then add any number of methods. Classes in JavaScript are a type of function only, but instead of using the keyword " function ", the keyword " class " is used to declare a class. Its syntax looks like below: class classname { //variables and methods which need to as part of an object } Tip: Also see How To Toggle A Class. Tip: Also see How To Remove A Class. Tip: Learn more about the classList property in our JavaScript Reference. Tip: Learn more about the className property in our JavaScript Reference.
To extend a class: class Child extends Parent: That means Child.prototype.__proto__ will be Parent.prototype, so methods are inherited. When overriding a constructor: We must call parent constructor as super() in Child constructor before using this. When overriding another method: We can use super.method() in a Child method to call Parent method. Classes in JavaScript As of ecmascript 2015 (es6), class es were added to the JavaScript language. However it's very important to understand that underneath the syntax, class es use functions and... The static keyword defines a static method or property for a class. Neither static methods nor static properties can be called on instances of the class. Instead, they're called on the class itself. Static methods are often utility functions, such as functions to create or clone objects, whereas static properties are useful for caches, fixed-configuration, or any other data you don't need to ...
A class can be defined by using a class declaration. A class keyword is used to declare a class with any particular name. According to JavaScript naming conventions, the name of the class always starts with an uppercase letter. JavaScript is not a class-based object-oriented language. But it still has ways of using object oriented programming (OOP). In this tutorial, I'll explain OOP and show you how to use it. According to Wikipedia, class-based programming is a style of Object-oriented programming (OOP) in which inheritance occurs via defining classes Toggling the class means if there is no class name assigned to the element, then a class name can be assigned to it dynamically or if a certain class is already present, then it can be removed dynamically by just using the toggle() or by using contains(), add(), remove() methods of DOMTokenList object within JavaScript.. Properties of HTML elements being used:
ES6 brought classes to JavaScript, which are nothing but a new way of writing constructor functions by utilizing the prototype functionality. If you want to learn more about objects, here is an in-depth MDN guide that explains how to use objects, properties, and methods. Define a class in Javascript One way to define the class is by using the class declaration. If we want to declare a class, you use the class keyword with the name of the class ("Employee" here). See the below code. One way to define a class is using a class declaration. To declare a class, you use the class keyword with the name of the class ("Rectangle" here). class Rectangle { constructor ( height , width ) { this . height = height ; this . width = width ; } }
As you can see, I have used constructor method to set property as the time of class instantiate. Create Class Using class expression keyword. We can also create class using js class expression.We will use let keyword and store class into this variable.The class expression can be named or unnamed. you have put the call to the private method inside the constructor of the javascript class. in that point the functions are not yet initialized. but if you initialize the object like so: var test = new MyObject(); and then do this: test.myMethod(); it will work.
How To Load A Class Dynamically In Javascript Es6 Stack
How To Call Javascript Function In Html Javatpoint
Closest Ancestor Element That Has A Specific Class In
Basic Accounting Formulas Calculator In Javascript Classes
Learn Javascript All Levels Start Strong In Js
Javascript Call Class Method Code Example
The Complete Guide To Javascript Classes
Why Does The Javascript Class Keyword Display As A Error In
A Simple Explanation Of Javascript Classes By Raji Ayinla
Example Of Class In Javascript Es6 Learn Web Tutorials
How Classes Work In Javascript Dev Community
Javascript Object Methods Geeksforgeeks
A Quick Guide To Get Started With Javascript Classes
Details Of The Object Model Javascript Mdn
Javascript Code Cleanup How You Can Refactor To Use Classes
Javascript Class How To Define And Use Class In Javascript
Javascript Class Find Out How Classes Works Dataflair
How Classes Work In Javascript Skptricks
Using Element Classlist To Manipulate Css Classes On The
0 Response to "24 How To Use A Class In Javascript"
Post a Comment