22 Javascript Get Random Array Element
Lesson Code: http://www.developphp /video/JavaScript/Get-or-Remove-Random-Array-Elements-TutorialSince JavaScript does not have built in methods for selec... Feb 26, 2020 - See the Pen JavaScript - Get a random item from an array - array-ex- 35 by w3resource (@w3resource) on CodePen. Improve this sample solution and post your code through Disqus · Previous: Write a JavaScript function to get nth largest element from an unsorted array.
Retrieve Random Item From An Array In Javascript By Arpit
Jul 25, 2021 - Choose a random element from a multidimensional array · Most of the time, we work with 2d or 3d arrays in Python. ... numpy.random.choice() function to generate the random choices and samples from a NumPy multidimensional array. Using this function we can get single or multiple random numbers ...
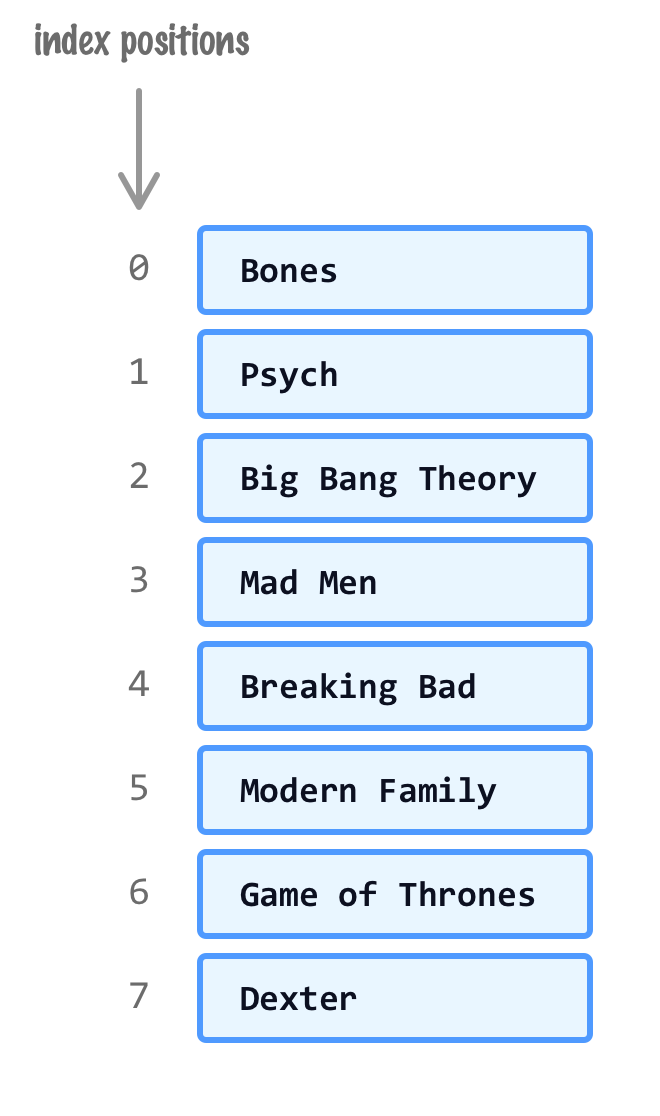
Javascript get random array element. generating a random number and checking in which segment it lands. STEP 1. make a prefix sum array for the probability array, each value in it will signify where its corresponding section ends. For example: If we have probabilities: 60% (0.6), 30%, 5%, 3%, 2%. the prefix sum array will be: [0.6,0.9,0.95,0.98,1] Write a JavaScript program to get a random element from an array. Use Math.random () to generate a random number. Multiply it by Array.prototype.length and round it off to the nearest whole number using Math.floor (). This method also works with strings. Jul 06, 2020 - Finally, it picks the first n items of the (randomly ordered) array of items (line 6). For a better understanding, read the documentation of functions like map and sort, like in developer.mozilla /en-US/docs/Web/JavaScript/Reference/…. ... Gets one or n random elements at unique keys from ...
The random () function does this work for you. It is a powerful function that can generate multiple random unique numbers. The random () function generates a float number between o and 1. Including only 1. So, we will use this random number to find random elements of the JavaScript array. Method 3: Using Random Class function. nextInt () method of Random class can be used to generate a random value between 0 and the size of ArrayList. Now use this number as an index of the ArrayList. Use get () method to return a random element from the ArrayList using number generated from nextInt () method. Definition and Usage. The find() method returns the value of the array element that passes a test (provided by a function).. The method executes the function once for each element present in the array: If it finds an array element where the function returns a true value, find() returns the value of that array element (and does not check the remaining values)
How to get multiple, random, unique elements from a JavaScript array. By Alvin Alexander. Last updated: April 18, 2018. As a note to self, this JavaScript code gets multiple, random, unique elements from a JavaScript array and displays three of those elements in the second div: Jul 21, 2021 - Get access to ad-free content, doubt assistance and more! ... Explain the differences between for(..in) and for(..of) statement in JavaScript. ... Ways of iterating over a array in JavaScript. How to ignore loop in else condition using JavaScript ? ... The task is to select the random element from ... We can use the Array.filter() method to find elements in an array that meet a certain condition. For instance, if we want to get all items in an array of numbers that are greater than 10, we can do this: const array = [10, 11, 3, 20, 5]; const greaterThanTen = array.filter(element => element > 10); console.log(greaterThanTen) //[11, 20]
Nov 07, 2020 - Retrieve a random item from an array is a common task and fairly easy to implement. There are many ways to achieve that and I am going to discuss a few of them In the normal method, we are using… You want to randomly select an index of the array. Arrays are zero-indexed, so you can review Generate Random Whole Numbers with JavaScriptfor exactly how to do this. Once you have a random index, you can simply returnthe element of data (passed to the function) which has the index of this random index you created. 17/2/2019 · We can get a particular item from an array just by passing the index number just like we can see below: myArr[3] // Output: media To take the random value from our JavaScript array, we are passing a random index number of the array within the range instead of sending a fixed index number to get a random item.
Create an array that contains the indexes of the array used to hold the data you want to randomly select from. Then randomly pick an item from this index array and use its stored value to retrieve the item from the data array. Then remove the index item so that the array of indexes continues to get smaller. You can get a random element from the Array in JS by using Math.random () function to get the random number then Multiply it by the array length. After it, you will get numbers between zero to array length. Last use Math.floor () to get the index number from 0 to arrayLength-1. JS get a random element from the Array Example 13/9/2020 · Sometimes we need to get a random element from an array in JavaScript. An array is a list of indexed elements so in order to get a random element, we need to pick one of the indexes at random. Getting a Random Index Number. Array indexes start at 0 and count up until the final element. Therefore we need to pick a random number between 0 and the length of the array minus 1.
Jul 26, 2019 - Given a string S and a character C, return an array of integers representing the shortest distance from the character C in the string. javascript ... Learn how to use Js export and import. ... The value associated with each key will be an array consisting of all the elements that resulted in ... Apr 19, 2020 - Is it possible to use Math.random to return a random value in an array? Does that value be a string and still work? java ... javascript create variable containing an object that will contain three properties that store the length of each side of the box This random value is the index of input array which is not yet accessed. Then we remove this value from alreadyDone array and return corresponding value at index from myArray. Live Demo. This demo will show you the working of our code example of accessing javascript random array element no repeat.
Write a function called lucky_sevens which takes an array of integers and returns true if any three consecutive elements sum to 7. by js · exchange value between 2 items in array javascript Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. In this video i will show you how you can easily get a element/item from an Array list in simple and easy steps using Javascript.Random item selection from a...
javascript how to get a random element from an array · javascript by Helpless Hornet on Mar 19 2020 Comment // program to get a random item from an array function getRandomItem(arr) { // get random index value const randomIndex = Math.floor(Math.random() * arr.length); // get random item const item = arr[randomIndex]; return item; } const array = [1, 'hello', 5, 8]; const result = getRandomItem(array); console.log(result); Jul 26, 2019 - javascript how to get a random element from an array · javascript by Helpless Hornet on Mar 19 2020 Donate
May 19, 2020 - This is a short tutorial on how to get a random value from a JavaScript array. To do this, we will have to generate a random index number that represents an element in the array. This function is ran for every element in the array. You can pass 2 elements of the array, like this: list.sort((a, b) => Math.random() - 0.5) but in this case we're not using them. If the result of this operation is < 0, the element a is put to an index lower than b, and the opposite if the result is > 0.. You can read all the details on Array.sort() here. Jul 17, 2021 - Of course, you can use this function to select any random value from an array, not just an array of colors. We could stop here and call it a day, but let's take a closer look at the randomColor function and see what it does, bit by bit.
Sep 30, 2020 - We are required to write a JavaScript function that takes in an array of unique literals and a number n. The function should return an array of n elements all chosen randomly from the input array and no element should appear more than once in the output array. 3/4/2020 · To select a random value from an array in JavaScript, you can use the built-in Math object functions. Let us say we want to create a function that selects a random fruit from an array of fruits. Here is how our fruits array looks like: const fruits = [ "Apple", "Orange", "Mango", "Banana", "Cherry" ]; Mar 19, 2020 - javascript how to get a random element from an array · javascript by Helpless Hornet on Mar 19 2020 Comment
JavaScript has a buit in array constructor new Array (). But you can safely use [] instead. These two different statements both create a new empty array named points: const points = new Array (); Get random item from JavaScript array, I am working on 'how to access elements randomly from an array in javascript'. I found many links regarding this. Like: Get random item from JavaScript array How to select a random element from array in JavaScript ? Use Math.random() function to get the ... Nov 27, 2017 - I am building a program which will change the colour of an element from the click of a button. I want to create a function which will select 6 random elements from an array of 16 elements and return another array of the elements randomly selected. The code below only returns 1 element, not ...
Get or Remove Random Array Elements Tutorial. Since JavaScript does not have built in methods for selecting or removing array elements randomly, we will demonstrate how to extend the Array object to supply it with those types of methods. The first example shows how to get a random array element without affecting the original array, the second ... 30/8/2020 · This plugin will return a random element if given an array, or a value from [0 .. n) given a number, or given anything else, a guaranteed random value! For extra fun, the array return is generated by calling the function recursively based on the array's length :) Working demo at http://jsfiddle /2eyQX/ Select Random Item from an Array. Chris Coyier on Dec 23, 2016. var myArray = [ "Apples", "Bananas", "Pears" ]; var randomItem = myArray [ Math.floor( Math.random()* myArray. length)]; Our Learning Partner. Frontend Masters. Need front-end development training? Frontend Masters is the best place to get it.
Multiply the random number with array.length (to get a number between 0 and array.length); Use Math.floor() on the result to get an index between 0 and array.length - 1; Use the random index on the array to get an element at random. For example, let's suppose you have an array of colors like the following from which you wish to pick a color ... A Proper Random Function. As you can see from the examples above, it might be a good idea to create a proper random function to use for all random integer purposes. This JavaScript function always returns a random number between min (included) and max (excluded):
Picking A Random Item From An Array Kirupa Com
How To Get Random Element From Array In Javascript
How To Select A Random Element From An Array In Javascript
How To Select A Random Element From Array In Javascript
Pick Random Item From Array Javascript Vps And Vpn
How To Select A Random Element From An Array In Javascript
Javascript Generate Random Array Code Example
Random Element Of The Array With Javascript Web Profile
Javascript Generate Random Number And Map It To An Array
Picking A Random Item From An Array Kirupa Com
Javascript Array Get A Random Item From An Array W3resource
Shuffle Json Array Javascript Code Example
How To Build A Random Quote Generator With Javascript And
Picking A Random Item From An Array Javascript Code Example
Pick A Random Element From An Array Dev Community
Random Numbers Ecosystem In Julia The Pseudo Side
How To Select A Random Element From An Array In Java Code Example
Generate A Random Number Between Two Numbers In Javascript
Picking A Random Item From An Array Kirupa Com
How To Select A Random Item From A List In Excel
How To Generate An Array Of Random Numbers In Javascript
0 Response to "22 Javascript Get Random Array Element"
Post a Comment