35 Javascript Currency To Number
In JavaScript, the Intl.NumberFormat () method is used to specify a currency we want a number to represent. The method is a constructor that can be used to format currency in its style property. This article states how we use the Intl.NumberFormat () to achieve this, as well as show some example code. Sep 01, 2020 - I would like to format a price in JavaScript. I'd like a function which takes a float as an argument and returns a string formatted like this: "$ 2,500.00" What's the best way to do this?
Handle Money With Js Dev Community
The Number () function converts the object argument to a number that represents the object's value. If the value cannot be converted to a legal number, NaN is returned. Note: If the parameter is a Date object, the Number () function returns the number of milliseconds since midnight January 1, 1970 UTC.
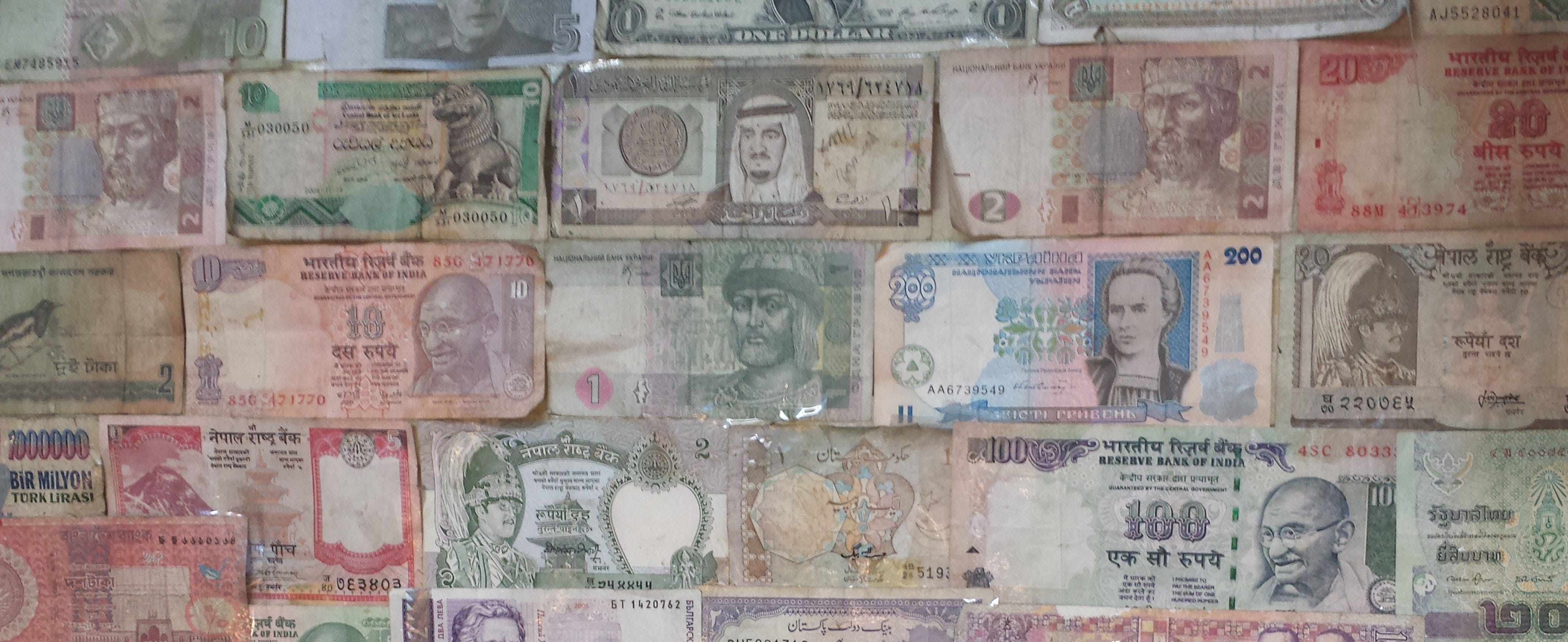
Javascript currency to number. Given a positive integer n representing the amount of cents you have, return the formatted currency amount. For example, given n = 123456, return "1,234.56" how to convert number to format in euro javascript The Intl.NumberFormat object can be used to format a number as a currency in Javascript. Various options can be set to customize format of currency code, comma / decimal separators etc. A number in Javascript can be displayed in currency format using the Intl.NumberFormat object. Furthermore currency is formatted as per the required localization. A number from 0 to 20 (default is 3) maximumSignificantDigits: A number from 1 to 21 (default is 21) minimumFractionDigits: A number from 0 to 20 (default is 3) minimumIntegerDigits: A number from 1 to 21 (default is 1) minimumSignificantDigits: A number from 1 to 21 (default is 21) style: Legal values: "currency" "decimal" (default) "percent ...
Use parseFloat () function, which purses a string and returns a floating point number. The argument of parseFloat must be a string or a string expression. The result of parseFloat is the number whose decimal representation was contained in that string (or the number found at the beginning of the string). In the currency variable, dots need to get eliminated and commas turned into floating points. I need to cater for cases when currency and views variables do not have a number in their string or do have a dash or a blank in them. Would you say this script can be optimsed to be more efficient? ku4js-kernel has a sweet $.money class for this. You can just $.money.parse("$101.82") and then do what you want. You could get the value() and use as a number or even better, just keep as money and do your operations. You can check out the documentation here.
Jun 15, 2020 - Convert a String to a Number! ... Write a function that accepts an array of 10 integers (between 0 and 9), that returns a string of those numbers in the form of a phone number. ... Write a javascript program to find roots of quadratic equation. Intl.NumberFormat.prototype.format () Getter function that formats a number according to the locale and formatting options of this Intl.NumberFormat object. Intl.NumberFormat.prototype.formatToParts () Returns an Array of objects representing the number string in parts that can be used for custom locale-aware formatting. A number, represented as monetary value, creates an impact and becomes much more readable, and that's the reason behind formatting a number as currency. For example, a number, let's say 100000 when represented as $100,000.00 it becomes pretty much understand that it represents a monetary value, and the currency in which it is formatted is USD .
You can use the NumberFormat instance to format any number into a currency value... For currency formatting, I used Number.prototype.toFixed() to convert a number to string to have always two decimal digits.. If you need to format currency for a different country / locale, you would need to add some modifications to the currencyFormat method. // program to format numbers as currency string const number = 1234.5678; const result = '$ ' + number.toFixed(2); console.log(result); Output. $ 1234.57. In the above example, the toFixed(2) method is used to round up the number to two decimal values. '$' is added to the number to convert it into a currency string.
accounting.js accounting.jsis a tiny JavaScript library by Open Exchange Rates, providing simple and advanced number, money and currency formatting. Features custom output formats, parsing/unformatting of numbers, easy localisation and spreadsheet-style column formatting (to line up symbols and decimals). The minimum number of fraction digits to use. Possible values are from 0 to 20; the default for plain number and percent formatting is 0; the default for currency formatting is the number of minor unit digits provided by the ISO 4217 currency code list (2 if the list doesn't provide that information). Displaying a Number as Currency in JavaScript. JavaScript provides a number of options -- from simple to complex -- that allows you to format and display numbers as currency. Regardless of which you use, however, the process is not as simple as it may first appear.
13/5/2018 · Say you have a number like 10, and it represents the price of something. You want to transform it to $10,00. If the number has more than 3 digits however it should be displayed differently, for example 1000 should be displayed as $1,000.00. This is in USD, however. Different countries have different conventions to display values. For more details on why Javascript has issues with floating point numbers, there's an excellent talk by Bartek Szopka on everything you never wanted to know about Javascript numbers explaining why Javascript and other IEEE 754 implementations have floating point issues. A javascript library for formatting and manipulating numbers. Set. Set the value of your numeral object. var number = numeral(); number.set(1000); var value = number.value(); // 1000
17/6/2019 · There are two popular ways to convert currency string into float string using different JavaScript inbuilt libraries. Method 1: This is a simple approach in which we will match character one by one from starting using the loop and if any character comes out to be of integer type or numeric type then we will use substring() method to take out substring from original string. Learn how to format any given number to a currency in javascript. Many times you get value for something in numbers 5000 and you want to display them as a currency in your locale "₹ 5,000" . This is how your value would be displayed in INR . Solution: See this JavaScript Currency Converter With API and CSS Styling, JS Currency Calculator Program. Previously I have shared a simple calculator using JavaScript, but this is a currency calculator with third-party API. There is almost all currency available, you can check the exchange rate of any currency comparing to others.
JavaScript only has the Number type, which can be used as an integer or a double precision float. Because it's a binary representation of a base 10 system, you end up with inaccurate results when you try to do math. 0.1 + 0.2 // returns 0.30000000000000004 😧 Using floats to store monetary values is a bad idea. In JavaScript, the easiest and most popular way to format numbers as currency strings is via the Intl.NumberFormat () method. This approach lets you format numbers using custom locale parameters - and in this article, we'll focus on currencies. The Intl.NumberFormat () constructor accepts two arguments, the first being a locale string, with ... Paolo Ferretti. Mar 10, 2016 · 1 min read. To format numbers with locale formatting conditions, you can use toLocaleString () method of Javascript Number object. So, for example: var data ...
Mar 21, 2021 - Learn how to format a number as a currency string in JavaScript. Use the toLocaleString () Method to Format a Number as a Currency String in JavaScript Use the Numeral.js Library to Format a Number as a Currency String in JavaScript A number is represented in many ways, date/time, currency, number, and much more. When it is represented as a monetary value, its impact increases and becomes much more readable. In this article, you will learn how to format numbers as a currency string in Javascript. Let's say you have a number variable 'a' with value 12345.60. var a = 12345.60; In order to format numbers as a currency, you can use the Intl.NumberFormat()method. In this example, you will be formatting a number into a currency of type USD.
7/6/2021 · In Javascript applications, You can store data as numbers and display the data in currency strings. For example, In commerce web application, Each product is displayed with price. Suppose, Application shows dollar as currency to US users and display it in Rupees to Indian users. JavaScript HTML CSS Result Visual: Light Dark Embed snippet Prefer iframe?: No autoresizing to fit the code. Render blocking of the parent page. jinglesthula Fiddle meta Private fiddle Extra. Groups Extra. Resources URL cdnjs 0. Paste a direct CSS/JS URL; Type a library name to fetch from CDNJS; Async requests ... Here is an example of how to output rounded currency formatted numbers using the decimal option with the toLocaleString function: 1. var outNumber = (Number (enteredNumber) ).toLocaleString ('en-US', { style: 'decimal', maximumFractionDigits : 2, minimumFractionDigits : 2 }); This behaves exactly like the currency option, but the decimal option ...
Take a look at the JavaScript Number object and see if it can help you. toLocaleString () will format a number using location specific thousands separator. toFixed () will round the number to a specific number of decimal places. To use these at the same time the value must have its type changed back to a number because they both output a string. Given a positive integer n representing the amount of cents you have, return the formatted currency amount. For example, given n = 123456, return "1,234.56" how to convert number to format in euro javascript JavaScript to Convert Numbers Into Words. If you want to be able to do these conversions on your site, you will need a JavaScript code that can do the conversion for you. The simplest way to do this is to use the code below; just select the code and copy it into a file called toword.js. // Convert numbers to words.
iven a positive integer n representing ... currency amount. For example, given n = 123456, return "1,234.56". ... Write a function that accepts an array of 10 integers (between 0 and 9), that returns a string of those numbers in the form of a phone number. ... Write a javascript program to find roots ... Learn how to convert a string to a number using JavaScript. This takes care of the decimals as well. Number is a wrapper object that can perform many operations. If we use the constructor (new Number("1234")) it returns us a Number object instead of a number value, so pay attention.Watch out for separators between digits:
Best Free Currency In Javascript Amp Css Css Script
Formatting Numbers As Currencies In Javascript By Ilarion
Set Number Date And Currency Formats Thoughtspot
The Dollar The World S Currency Council On Foreign Relations
International Number And Currency Formatting With Js
Currency Number Javascript Internationalization Tutorial
Number Currency To Word Currency Indian Currency On
Angularjs Filter Example Lowercase Uppercase Amp Json
Convert Numbers Into A Currency Format Using Javascript
Auto Format Currency Money With Jquery Simple Money
Simple Javascript Currency Converter Currency Converter
Convert Currency String To Number Javascript Javascript
How To Add Separator Comma Inside A Formula Microsoft
Convert Integer Values To Money Javascript Code Example
15 Javascript Libraries For Formatting Number Currency Time
Javascript Format Currency Intl Numberformat Career Karma
How To Format Numbers As Currency String In Javascript
How To Format Currency In Es6 Samanthaming Com
Outputting A Currency Symbol Using Javascript Javascript
How To Change Currency And Currency Symbol Using Javascript
How To Handle Monetary Values In Javascript Frontstuff
Changing Currency Field Display Format
The Currency Converter In Javascript Stack Overflow
How To Format A Number As A Currency Value In Javascript
Natively Format Javascript Numbers
How To Format Numbers As A Currency String In Javascript
Convert Number To Words In Codeigniter Pakainfo
Javascript Currency Format With Decimal Recognition Stack
Central Bank Digital Currency Risks Stifling Innovation
Enable Commas Within The Currency Fields To Work With
15 Javascript Libraries For Formatting Number Currency Time
Html5 Input For Money Currency Stack Overflow
Number Amp Currency Tolocalestring Js Tutorial 2020
0 Response to "35 Javascript Currency To Number"
Post a Comment