20 Javascript Check Null Or Undefined
javascript check if undefined or null or empty string Jan 27, 2021 - In contrast, JavaScript has two such non-values: undefined and null. In this blog post, we examine how they differ and how to best use or avoid them.
What S The Difference Between Null And Undefined In Javascript
February 3, 2021 June 25, 2021 amine.kouis 0 Comments check if value is null javascript, check if variable is undefined javascript, how to check undefined in jquery, javascript check for null or empty string, javascript check if not undefined, javascript check if variable is defined, javascript check undefined or null or empty, jquery check if ...
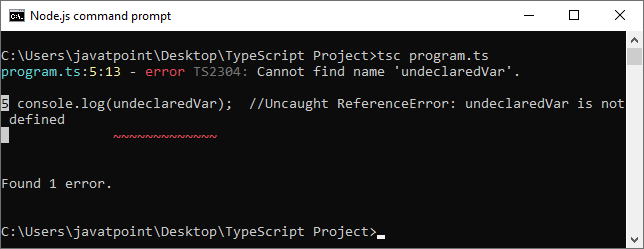
Javascript check null or undefined. Nov 19, 2020 - How to Check for Undefined in JavaScript. The typeof keyword returning "undefined" can mean one of two things: the variable is the primitive undefined, or the variable has not been…. Jan 07, 2021 - The typeof undefined is what you ... an object or a null value; you can’t have an undefined value. And whether you check object !== null or object != null ends up being your personal preference when type checking JavaScript objects.... Nov 12, 2019 - In your own functions, you can avoid creating null or undefined values to begin with. There are a couple ways to do that built into JavaScript that spring to mind. See below. ... I never explicitly create null values in JavaScript, because I never really saw the point of having two different ...
3. Validate the undefined, null and length of an array. There can be cases where the array doesn't exist at all or remains uninitialized. We can have a check over such cases also and verify for the length of arrays. We will check the array for undefined or nullwith the help of typeof an operator. If these check pass then we can check for the ... javascript check if undefined or null or empty string Oct 23, 2019 - javascript check if undefined or null or empty string
Finally, the standard way to check for null and undefined is to compare the variable with null or undefined using the equality operator (==). This would work since null == undefined is true in JavaScript. null !== undefined But, and this may surprise you, null loosely equals undefined. Non-null and non-undefined type guards may use the ==, !=, ===, or !== operator to compare to null or undefined, as in x != null or x === undefined. The effects on subject variable types accurately reflect JavaScript semantics (e.g. double-equals operators check for both values no matter which ...
A function, test (val) that tests for null or undefined should have the following characteristics: test (null) => true test (undefined) => true test (0) => false test (1) => false test (true) => false test (false) => false test ('s') => false test ([]) => false Let's see which of the ideas here actually pass our test. In JavaScript, checking if a variable is undefined can be a bit tricky since a null variable can pass a check for undefined if not written properly. As a result, this allows for undefined values to slip through and vice versa. Make sure you use strict equality === to check if a value is equal to undefined. Example 1: Check undefined or null // program to check if a variable is undefined or null function checkVariable(variable) { if(variable == null) { console.log('The variable is undefined or null'); } else { console.log('The variable is neither undefined nor null'); } } let newVariable; checkVariable(5); checkVariable('hello'); checkVariable(null); checkVariable(newVariable);
The value null represents the intentional absence of any object value. It is one of JavaScript's primitive values and is treated as falsy for boolean operations. To check for null SPECIFICALLYyou would use this: if (variable === null) This test will ONLYpass for nulland will not pass for "", undefined, false, 0, or NaN. Additionally, I've provided absolute checks for each "false-like" value (one that would return true for !variable). // simple check do the job if (myVar) { // comes here either myVar is not null, // or myVar is not undefined, // or myVar is not '' (empty string).
2 weeks ago - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. There are few simple methods in JavaScript using which you can check or determine if a variable is undefined or null. At the end we see that even though null and undefined are considered equal, they are not the same identity (equal without type conversion).As discussed, this is because they are of different types behind the scenes: null being an object and undefined being an undefined type. With that out of the way we can start to understand why trying to access a property of null or undefined may fail.
So when it comes to comparing null, undefined, and "": null == undefined in the sense that they both have a "nothing" value. null !== undefined because one is an object while the other is undefined. The empty string is the "odd one" that will not match with null and undefined. The undefined value is derived from the null value undefined: declares a variable but is not initialized. The value of this variable is automatically assigned the undefined value. So no matter what th... ... History When JavaScript was born in 1995, it was originally set up like ... In simple words you can say a null value means no value or absence of a value, and undefined means a variable that has been declared but no yet assigned a value. To check if a variable is undefined or null you can use the equality operator == or strict equality operator === (also called identity operator).
The nullish coalescing operator (??) can be used to give default values to variables in Javascript if they are either null or undefined. A common practice in programming is to give default values to variables if they are either undefined or null. The nullish coalescing operator has been introduced in Javascript for these cases. In JavaScript null is an object (try typeof null in a JavaScript console if you don't believe me), which means that null is a value (in fact even undefined is a value). An Easy and better way to check for null and undefined values in JavaScript. Published July 15, 2020 . Photo byLaya Clode. In many cases, we have null or undefined values in our variables. We can conditionally show other relevant value using the new ?? (Nullish coalescing) operator instead of just showing null or undefined values.
Aug 02, 2020 - Most of the modern languages like Ruby, Python, or Java have a single null value (nil or null), which seems a reasonable approach. But JavaScript is different. null, but also undefined, represent in JavaScript empty values. So what’s the exact difference between them? This snippet will guide you in finding the ways of checking whether the string is empty, undefined, or null. Here we go. If you want to check whether the string is empty/null/undefined, use the following code: let undefinedStr; if (!undefinedStr) { console .log ( "String is undefined" ); } let emptyStr = "" ; if (!emptyStr) { console … In JavaScript, null is treated as an object. You can check this using the typeof operator. The typeof operator determines the type of variables and values. For example, const a = null; console.log (typeof a); // object. When the typeof operator is used to determine the undefined value, it returns undefined.
Feb 17, 2021 - All other values in Javascript ... when Javascript is first created. ¯\_(ツ)_/¯ ... (I never knew these are their real names haha when we are so used to calling these “strictly” or “loosely” equal to each other.) When we are checking for null and undefined , it is quite ... If we create one variable and don’t assign any value to it, JavaScript assigns undefined automatically. null is an assignment value, we need to assign a variable null to make it null. If you don’t know the content of a variable in JavaScript, it is always a good idea to check if it is undefined or null. null is a special value in JavaScript that represents a missing object. The strict equality operator determines whether a variable is null: variable === null. typoef operator is useful to determine the type of a variable (number, string, boolean). However, typeof is misleading in case of null: typeof null evaluates to 'object'.
Null: It is the intentional absence of the value. It is one of the primitive values of JavaScript. Undefined: It means the value does not exist in the compiler. It is the global object. You can see refer to "==" vs "===" article. It means null is equal to undefined but not identical. When we define a variable to undefined then we are ... The explicit approach, when we are checking if a variable is undefined or null separately, should be applied in this case, and my contribution to the topic (27 non-negative answers for now!) is to use void 0 as both short and safe way to perform check for undefined. Feb 17, 2021 - Next, I explore using the == or === equality operators to check for null. ... “Despite the fact that null is [falsy], it isn't considered loosely equal to any of the other falsy values in JavaScript. In fact, the only values that null is loosely equal to are undefined and itself.” —Josh ...
I often see JavaScript code which checks for undefined parameters etc. this way: if (typeof input !== "undefined") { // do stuff } This seems kind of wasteful, since it involves both a type lookup and a string comparison, not to mention its verbosity. It's needed because undefined could be renamed, though. null and undefined in JavaScript As we have seen in the variable section that we can assign any primitive or non-primitive type of value to a variable. JavaScript includes two additional primitive type values - null and undefined, that can be assigned to a variable that has special meaning. To check a null value in JavaScript, use the equality operator (==). The equality operator (==) in JavaScript checks whether its two operands are equal, returning a Boolean result. Unlike the strict equality operator, it attempts to convert and compare operands of different data types.
By using the ?. operator instead of just., JavaScript knows to implicitly check to be sure obj.first is not null or undefined before attempting to access obj.first.second. If obj.first is null or undefined, the expression automatically short-circuits, returning undefined. Nullish coalescing operator (??) The nullish coalescing operator (??) is a logical operator that returns its right-hand side operand when its left-hand side operand is null or undefined, and otherwise returns its left-hand side operand. Null vs. Undefined ... Fact is you will need to deal with both. Interestingly in JavaScript with ==
To check if a string is null or empty or undefined use the following code snippet if(!emptyString){ // String is empty }
How To Check If A Variable Is Undefined Or Null In Javascript
Difference Between Null And Undefined Javatpoint
Javascript Fundamentals Checking For Null And Undefined
Check If Javascript Variable Is Null Or Undefined Phpcoder Tech
Null Vs 0 Vs Undefined While Dev
Check If Javascript Variable Is Null Or Undefined Phpcoder Tech
How To Check If A Javascript Array Is Empty Or Not With Length
How To Check If A Variable In Javascript Is Undefined Or Null
Strictly Check For Null And Undefined Values In Typescript
Javascript Array Remove Null 0 Blank False Undefined And
How To Check Empty Undefined Null String In Javascript
How To Check For An Object In Javascript Object Null Check
What Is The Difference Between Null And Undefined In Javascript
Non Nullable Types In Typescript Marius Schulz
Undefined And Null In Javascript
Youvcode Knowledge Shared 2 X Knowledgehow To Determine
Javascript Null Vs Undefined Understanding The Difference
String Is Empty Javascript Design Corral
Javascript Check Null Or Undefined Best Practice Mad
0 Response to "20 Javascript Check Null Or Undefined"
Post a Comment