34 How To Use Includes In Javascript
The includes() method determines whether an array includes a certain value among its entries, returning true or false as appropriate. Syntax includes ( searchElement ) includes ( searchElement , fromIndex ) Code language: JavaScript (javascript) In this example, the colors array doesn't contain 'green' but 'GREEN'. First, the map() method makes every element in the colors array lowercase and returns a new array. Then, the includes() method returns true because the result array contains the element 'green'. 2) Check if an array contains a number
You Are Not Permitted To Use Any Javascript Built In Chegg Com
Array.includes compares by object identity just like obj === obj2, so sadly this doesn't work unless the two items are references to the same object. You can often use Array.prototype.some () instead which takes a function: const arr = [ {a: 'b'}] console.log (arr.some (item => item.a === 'b')) But of course you then need to write a small ...
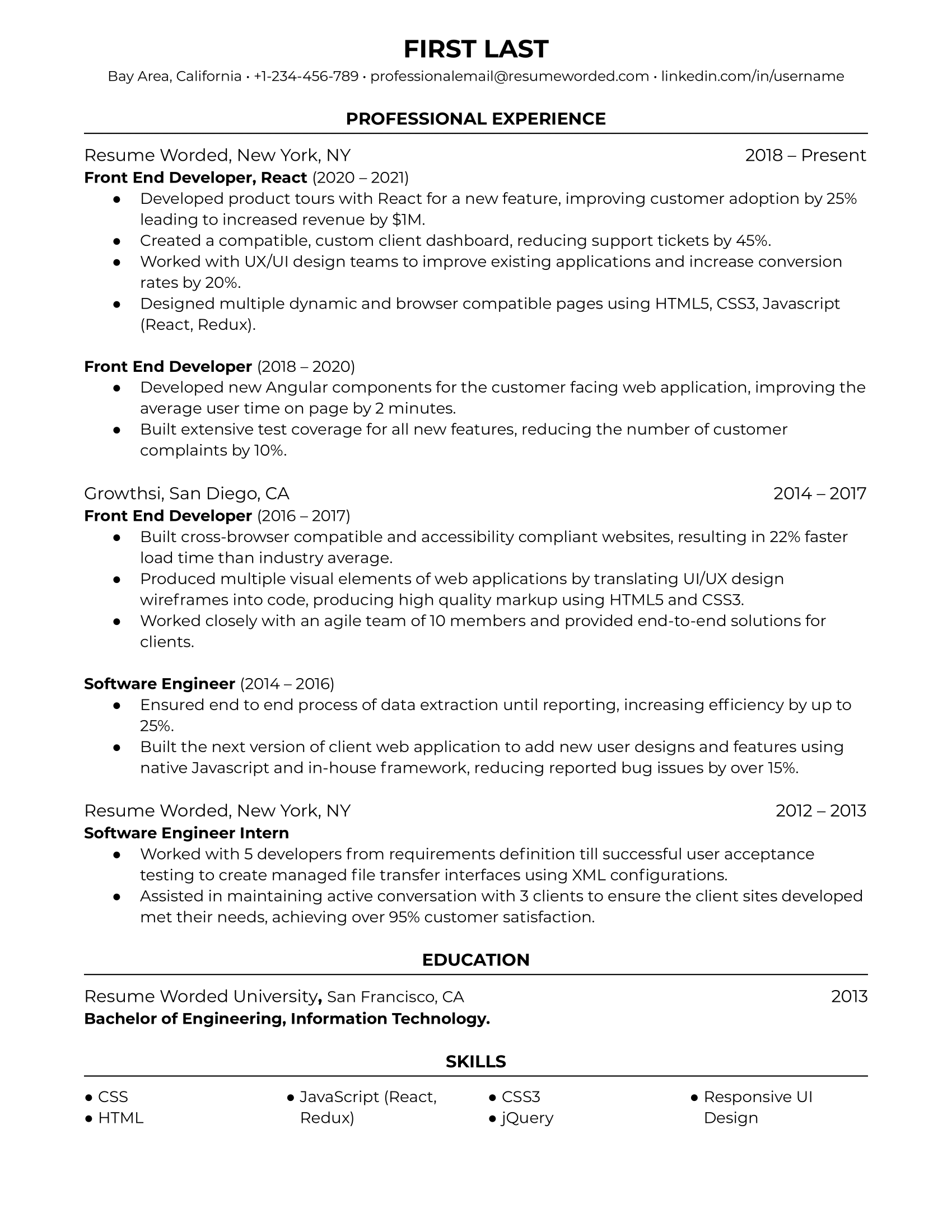
How to use includes in javascript. The includes () is a built-in method of JavaScript that is used to discover whether an element is present in an array or not. It can also be used to find substrings in a string. The returning value of the includes () method is a boolean, which means it either returns true or false. External JavaScript file only contains JavaScript code and nothing else, even the <script>.... </script>tag are also not used in it. The HTML noscript Element. The <nosscript> element provides us an alternate way to create content for the users that either have browsers that don?t support the JavaScript or have disabled JavaScript in the browser. In JavaScript, includes () method determines whether a string contains the given characters within it or not. This method returns true if the string contains the characters, otherwise, it returns false. Note: The includes () method is case sensitive i.e, it will treat the Uppercase characters and Lowercase characters differently.
String includes () Method The includes () method was introduced in ES6 and works in all modern browsers except Internet Explorer. Unlike the good old indexOf () method, which returns the starting index of the substring, the includes () method returns true if the string contains the specified substring. Otherwise, it returns false. Javascript array contains To check if a JavaScript array contains a value or not, use the array includes () method. The array includes () method checks whether an array contains the item or specified an element or not. We can also determine if an array consists of an object in JavaScript. In general, if you have any doubt about whether code will run in an environment that supports includes(), you should use indexOf(). The includes() function's syntax is only marginally more concise than indexOf(). Case Insensitive Search. Both String#includes() and String#indexOf() are case sensitive. Neither function supports regular expressions.
Note that if try to find the object inside an array using the indexOf() method like persons.indexOf({name: "Harry"}) it will not work (always return -1). Because, two distinct objects are not equal even if they look the same (i.e. have the same properties and values). In native JavaScript before ES6 Modules 2015 has been introduced had no import, include, or require, functionalities. Before that, we can load a JavaScript file into another JavaScript file using a script tag inside the DOM that script will be downloaded and executed immediately. To achieve all of these objectives and more, JavaScript comes with the built in Date object and related methods. This tutorial will go over how to format and use date and time in JavaScript. The Date Object. The Date object is a built-in object in JavaScript that stores the date and time. It provides a number of built-in methods for formatting ...
includes/copyright.js. Open an HTML editor and open a web page that will display the JavaScript output. Find the location in the HTML where the include file should display, and place the following code there: Add that same code to every relevant page. When the copyright information changes, edit the copyright.js file. The includes() method performs a case-sensitive search to determine whether one string may be found within another string, returning true or false as appropriate. Syntax includes ( searchString ) includes ( searchString , position ) 3 Ways To Include Javascript In HTML - Simple Examples By W.S. Toh / Tips & Tutorials - Javascript / July 20, 2021 July 20, 2021 Welcome to a tutorial on how to include Javascript in HTML.
Definition and Usage The includes () method returns true if a string contains a specified string, otherwise false. includes () is case sensitive. The includes () method is a searching algorithm used to find a substring within a string or to find elements within an array. includes () method returns boolean values (It returns either true or false). So it can be used as the condition for an if statement or a loop. ` JavaScript includes () ECAMScript 6 added the includes method to both the String and Array objects natively. This method returns either true or false if the target string is contained within the source string or array. let sample = "The human body is a remarkably adaptable machine.
3/6/2009 · Maybe you can use this function that I found on this page How do I include a JavaScript file in a JavaScript file? function include(filename) { var head = document.getElementsByTagName('head')[0]; var script = document.createElement('script'); script.src = filename; script.type = 'text/javascript'; head.appendChild(script) } We've included the HTML this time to show you how JavaScript prompts work with HTML elements. The element we're using is the div HTML tag with the id of "welcome." The JavaScript prompt asks the user for his name and then displays the result in the "welcome" div. This tutorial provides an introduction to working with JSON in JavaScript. Some general use cases of JSON include: storing data, generating data from user input, transferring data from server to client and vice versa, configuring and verifying data.
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. The JavaScript includes () method, introduced in ES6, determines whether a string contains the characters you have passed into the method. If the string contains certain characters, the method will return "true." If the specified string does not contain the characters for which you are looking, includes () will return "false." Filter array with filter() and includes() in JavaScript. Javascript Web Development Object Oriented Programming. Let's say following is our array − ...
To include code from another file, you have to use the import statement and import using the file path. For example, // importing codes from module file import { message, number, multiplyNumbers } from './modules.js'; Then, you can use these codes as they are a part of the same file. This helps in writing cleaner, maintainable, and modular code. To use JavaScript from an external file source, you need to write all your JavaScript source code in a simple text file with the extension ".js" and then include that file as shown above. For example, you can keep the following content in filename.js file and then you can use sayHello function in your HTML file after including the filename.js file. 18/4/2009 · in the case you care, here there is a version of include that uses the document object via it's DOM interface: function include(aFilename) { var script = document.createElement('script'); script.src = aFilename; script.type = 'text/javascript'; document.getElementsByTagName('head')[0].appendChild(script)}
The JavaScript includes () method determines whether an array contains a particular value. The includes () method returns true if the specified item is found and false if the specified item array_name.includes (element, start_position); The includes () method accepts two arguments: includes () Method The includes method is part of ES6 that can also be used to determine whether an array contains a specified item. This method returns true if the element exists in the array, and false if not. The includes () method is perfect for finding whether the element exists or not as a simple boolean value. The includes () method determines whether a string contains another string: The includes () method returns true if the searchString found in the string; otherwise false. The optional position parameter specifies the position within the string at which to begin searching for the searchString. The position defaults to 0.
Javascript Properties And Methods String Prototype Includes
Cross Origin Resource Sharing Cors Http Mdn
Solved Use Html And Javascript To Make A Basic Web Site
Includes Front End Javascript Libraries With Known Security
Javascript In Load Testing On Web Load Testing
Javascript Array Contains How To Use Array Includes Function
Filter Array Of Objects By Array Of Exclude Property Values
React Front End Developer Resume Example For 2021 Resume
Typescript Compiling With Visual Studio Code
How To Count The Vowels In A String By Brian Salemi Medium
Complex Numbers In Javascript Codedromecodedrome
Connect Jquery File To Nintex Form Nintex Community
How To Check If An Array Includes An Object In Javascript
How To Use Local Host To Run Javascript Files Which Includes
How String Includes Works In Javascript By Deeksha Sharma
Enhancing Nintex Forms With Javascript
A Beginner S Guide To Using Anime Js By Michael Jiang The
How To Use Tinymce In Dark Mode Our Code World
Javascript Array Contains How To Use Array Includes Function
A Quick Introduction To Javascript Array Includes Method
Create A Web Page That Includes A Form Style The Chegg Com
Problem Adding Javascript To A Community Page Salesforce
How To Use The Javascript Includes Function
Javascript String Matching Methods Dev Community
70 480 Exam Dumps You Are Developing An Html Page That
Getting Started With Typescript Includes Introduction To
How To Check If A String Contains A Substring In Javascript
How To Check If A String Contains A Substring In Javascript
Algorithms 101 Includes Vs Indexof In Javascript By
Browser License Key And App Id New Relic Documentation
Xpath Contains Following Sibling Ancestor Amp Selenium And Or
How To Use An Api With Javascript Beginner S Guide
0 Response to "34 How To Use Includes In Javascript"
Post a Comment