21 Check Number Regex Javascript
Here, [abc] will match if the string you are trying to match contains any of the a, b or c. You can also specify a range of characters using -inside square brackets. [a-e] is the same as [abcde]. [1-4] is the same as [1234]. [0-39] is the same as [01239]. You can complement (invert) the character set by using caret ^ symbol at the start of a square-bracket. ... Definition and Usage. The [0-9] expression is used to find any character between the brackets. The digits inside the brackets can be any numbers or span of numbers from 0 to 9. Tip: Use the [^0-9] expression to find any character that is NOT a digit.
How To Find Patterns Of Text In Javascript By Anh Dang
The / at both ends mark the start and end of the regex The ^ and $ at the ends is to check the full string than for partial matches \d* looks for multiple occurrences of number charcters You do not need to check for both \d as well as [0-9] as they both do the same - i.e. match numbers.
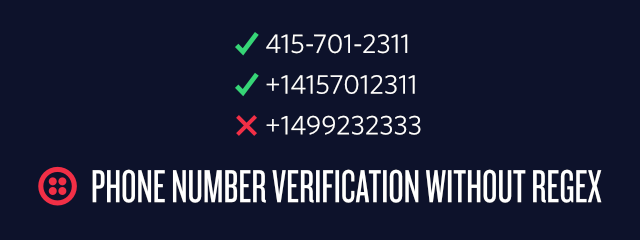
Check number regex javascript. I want to use JavaScript (can be with jQuery) to do some client-side validation to check whether a string matches the regex: ^([a-z0-9]{5,})$ Ideally it would be an expression that returned true or Jul 24, 2020 - Get code examples like "javascript regex for numbers only" instantly right from your google search results with the Grepper Chrome Extension. Check if a string only contains numbers Match elements of a url Match an email address Validate an ip address Match or Validate phone number Match html tag Empty String Match dates (M/D/YY, M/D/YYY, MM/DD/YY, MM/DD/YYYY) Checks the length of number and not starts with 0 Not Allowing Special Characters Match a valid hostname Validate datetime
In Set 1, we have discussed general approach to check whether a string is a valid number or not.In this post, we will discuss regular expression approach to check for a number.. Examples: Input : str = "11.5" Output : true Input : str = "abc" Output : false Input : str = "2e10" Output : true Input : 10e5.4 Output : false Jul 29, 2019 - To match all numbers and letters in JavaScript, we use \w which is equivalent to RegEx \[A-za-z09_]\. To skip all numbers and letters we use \W. This is by far the easiest way I have found to use javascript regex to check phone number format. this particular example checks if it is a 10 digit number. <input name="phone" pattern="^\d {10}$" type="text" size="50"> The input field gets flagged when submit button is clicked if the pattern doesn't match the value, no other css or js required.
Found a tool which allows you to edit regular expression visually: JavaScript Regular Expression Parser & Visualizer. Update: Here's another one with which you can even debugger regexp: Online regex tester and debugger. Update: Another one: RegExr. Update: Regexper and Regex Pal. Use the Regular Expressions to Check Whether a Given String Is a Number or Not in JavaScript. Regular expressions are an object that describes a pattern of characters. These can be used to search the pattern, make changes to them, add, delete, etc. We can use such patterns to check whether a string contains a number or not. For example, Cookbook of JavaScript regular expressions. Addition examples of parsing data with RegExp.
You can write a JavaScript form validation script to check whether the required field (s) in the HTML form contains only letters and numbers. Javascript function to check if a field input contains letters and numbers only Their syntax is cryptic, and the programming interface JavaScript provides for them is clumsy. But they are a powerful tool for inspecting and processing strings. Properly understanding regular expressions will make you a more effective programmer. ... A regular expression is a type of object. It can be either constructed with the RegExp ... When the user presses Enter, than javaScript checks that phone number is valid or not. If the number is valid then alert message will be displayed as 'Phone number is valid'. If the number is invalid, alert message will be displayed as 'Phone number is not valid.
This function checks if it's input is numeric in the classical sense, as one expects a normal number detection function to work. It's a test one can use for HTML form input, for example. It bypasses all the JS folklore, like tipeof (NaN) = number, parseint ('1 Kg') = 1, booleans coerced into numbers, and the like. Try running '/^\d+$/' === '/^d+$/' in your JavaScript console. You need to double-escape when embedding a RegExp in a string: eval('/^\\d+$/') – Mike Samuel Oct 18 '13 at 4:49 ... It doesn't take into account negative number. – Giuseppe Pes May 27 '15 at 11:22 26/2/2020 · To check for all numbers in a field. To get a string contains only numbers (0-9) we use a regular expression (/^ [0-9]+$/) which allows only numbers. Next, the match () method of the string object is used to match the said regular expression against the input value. Here is the complete web document. HTML Code.
JavaScript validation with regular expression: Exercise-2 with Solution. Write a JavaScript program to check a credit card number. Here are some format of some well-known credit cards. American Express :- Starting with 34 or 37, length 15 digits. Visa :- Starting with 4, length 13 or 16 digits. MasterCard :- Starting with 51 through 55, length ... Check number pattern with Javascript regex on the simple website I am building, I am requesting users phone number which is meant to come in either 080-0000-0000 COULD BE 090-0000-0000 OR EVEN 070-0000-0000 OR 080-0000-00000 COULD BE 090-0000-00000 OR EVEN 070-0000-00000 How do I use Javascript Regex test to check pattern, I can do it PHP bu Phone Number validation. The validating phone number is an important point while validating an HTML form. In this page we have discussed how to validate a phone number (in different format) using JavaScript : At first, we validate a phone number of 10 digits with no comma, no spaces, no punctuation and there will be no + sign in front the number.
Regex or Regular expressions are patterns used for matching the character combinations in strings. Regex are objects in JavaScript. Patterns are used with RegEx exec and test methods, and the match, replace, search, and split methods of String. The test () method executes the search for a match between a regex and a specified string. Test String. t1est 23 foo bar 304958 bar. Substitution. Expression Flags. ignore case (i) global (g) multiline (m) extended (x) extra (X) Additionally, area codes and exchanges may not take the form N11 (end with two ones) to avoid confusion with special services except numbers in a non-geographic area code (800, 888, 877, 866, 855, 900) may have a N11 exchange. So, your regex will pass the number (123) 123 4566 even though that is not a valid phone number.
Javascript Check is a string is valid number. Solution 3: RegEx is easy to make subtle ... javascript prompt javascript onclick javascript return javascript for javascript number javascript confirm javascript onchange javascript regular expression javascript if javascript variable javascript timer javascript cookie javascript getelementbyid ... Apr 24, 2018 - Try running '/^\d+$/' === '/^d+$/' in your JavaScript console. You need to double-escape when embedding a RegExp in a string: eval('/^\\d+$/') – Mike Samuel Oct 18 '13 at 4:49 ... It doesn't take into account negative number. – Giuseppe Pes May 27 '15 at 11:22 Get code examples like "js check regex for number" instantly right from your google search results with the Grepper Chrome Extension.
Jun 26, 2020 - Get code examples like "regex to get number from string javascript" instantly right from your google search results with the Grepper Chrome Extension. Detailed example of building a regex to match a number in a given range of numbers Nov 09, 2011 - But realized that it also allows ... characters. Since +100 and -5 are both numbers, isNaN() is not the right way to go. Perhaps a regexp is what I need? Any tips? ... @dewwwald: Some languages implement it differently, but in JavaScript, \d is exactly equivalent to ...
Enter a string: school Enter a letter to check: o 2. In the above example, a regular expression (regex) is used to find the occurrence of a string. const re = new RegExp (letter, 'g'); creates a regular expression. The match () method returns an array containing all the matches. Here, str.match (re); gives ["o", "o"]. 16/10/2019 · How to Check If A String Is a Number (Numeric) using Regex (C++/Javascript) ? October 16, 2019 No Comments c / c++, javascript, regex, string. Validate if a given string can be interpreted as a decimal number. Some examples: “0” => true. ” 0.1 ” => true. “abc” => false. “1 a” => false. “2e10″ => true. The exec () method is a RegExp expression method. It searches a string for a specified pattern, and returns the found text as an object. If no match is found, it returns an empty (null) object. The following example searches a string for the character "e": Example.
Check if a string only contains numbers Only letters and numbers Match elements of a url Match an email address Validate an ip address Url Validation Regex | Regular Expression - Taha match whole word Match or Validate phone number nginx test Match html tag Blocking site with unblocked games Find Substring within a string that begins and ends ... RegExr is an online tool to learn, build, & test Regular Expressions (RegEx / RegExp). Sep 21, 2017 - Detailed example of building a regex to match a floating point number as an illustration of a common mistake: making everything optional
When the user presses the "Check" button, the script checks the validity of the number. If the number is valid (matches the character sequence specified by the regular expression), the script shows a message thanking the user and confirming the number. If the number is invalid, the script informs the user that the phone number is not valid. Here we validate various type of password structure through JavaScript codes and regular expression. Check a password between 7 to 16 characters which contain only characters, numeric digit s and underscore and first character must be a letter. Check a password between 6 to 20 characters which contain at least one numeric digit, one uppercase ... The easiest way to validate phone numbers using Javascript would be to use Regular expressions. However there are different formats of Phone numbers depending on the user's preference. Here are some regular expressions to validate phone numbers. Phone number validation using a simple Regular expression
Feb 20, 2021 - If it is a positive number with a positive sign, RegExp() will ignore the positive sign. const str1 = "NaN means not a number. Infinity contains -Infinity and +Infinity in JavaScript.", str2 = "My grandfather is 65 years old and My grandmother is 63 years old.", str3 = "The contract was declared ... Use test() whenever you want to know whether a pattern is found in a string.test() returns a boolean, unlike the String.prototype.search() method (which returns the index of a match, or -1 if not found). To get more information (but with slower execution), use the exec() method. (This is similar to the String.prototype.match() method.) As with exec() (or in combination with it), test() called ... Jul 20, 2021 - The search() method executes a search for a match between a regular expression and this String object.
The above code has an input box and a rectangular div tag. There is, however, a few pieces of AngularJS code that we'll see in a moment. The passwordStrength variable that you see in the ng-style tag holds all the styling information for the strength rectangle. The style information we're going to use is as follows: Still, in some cases, a telephone number can take on a format using various special characters including plus signs and parentheses or take on a wholly different format than another country or region. The goal for phone number validation is to be able to check whether, for example, +1-541-754-3010 or 19-49-89-636-48018 are actual phone numbers. Regular expression tester with syntax highlighting, explanation, cheat sheet for PHP/PCRE, Python, GO, JavaScript, Java. Features a regex quiz & library. regex101: build, test, and debug regex
Javascript function to check whether a field input contains a number with no exponent, mandatory sign (+-), integer, and fraction. To get a string contains a number with no exponent, mandatory integer, fraction, sign (+ -) we use a regular expression / [-+] [0-9]+\. [0-9]+$/ which allows the said format. Next, the match () method of a string ... 26/2/2020 · Write a JavaScript function to check whether a given value is a social security number or not. Sample Solution:-HTML Code: <!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>JavaScript function to check whether a given value is A social security number or not</title> </head> <body> </body> </html> JavaScript Code: 11/2/2021 · To check if a string contains at least one number using regex, you can use the \d regular expression character class in JavaScript. The \d character class is the simplest way to match numbers. // Check if string contain atleast one number 🔥 /\d/.test ( "Hello123World!" ); // true. To get a more in-depth explanation of the process.
Javascript Regular Expression How To Create Amp Write Them In
How To Validate An Email Address In Javascript Stack Overflow
Beginning To Use Regex In Javascript By Cristina Murillo
Regex Google Analytics Amp Google Tag Manager Tutorial
Phone Number Verification Without Regular Expression
Using Regular Expression In Javascript Codeproject
How To Match A String Of Words Using Regex Javascript Stack
A Guide To Javascript Regular Expressions
The Essentials Of Regular Expressions By Sgwethan Towards
Master Javascript Form Validation By Building A Form From Scratch
Javascript Check Valid Domain Name Gmail Code Example
Basic Regex In Javascript For Beginners Dev Community
Javascript Regex Match Check If String Matches Regex Laptrinhx
Java Email Regex Examples Mkyong Com
Introduction To The Use Of 10 Regular Expressions In
Don T Fear The Regex Getting Started On Regular Expressions
8 Regular Expressions You Should Know
How Javascript Works Regular Expressions Regexp By
Javascript Validation With Regular Expression Check Whether
Regex Pattern For Phone Number Javascript Code Example
0 Response to "21 Check Number Regex Javascript"
Post a Comment