21 Javascript Hex To Decimal
Read more: How to assign decimal, octal and hexadecimal values to the variables in JavaScript? In such cases, we can convert a decimal value to the hexadecimal string by using the number.toString (16) and hexadecimal string to the decimal by using hex_string.parseInt (16). In both cases, 16 is the base to the number system that says that target ... Python Bootcamp - https://www.codebreakthrough /python-bootcamp💯 FREE Courses (100+ hours) - https://calcur.tech/all-in-ones🐍 Python Course - https://ca...
Convert Hex To Rgb With Javascript
How to Convert Hexadecimal number to decimal in JavaScript? Reviewed by Bhaumik Patel on 7:37 PM Rating: 5 ... How to Convert Hexadecimal number to decimal in Ja...
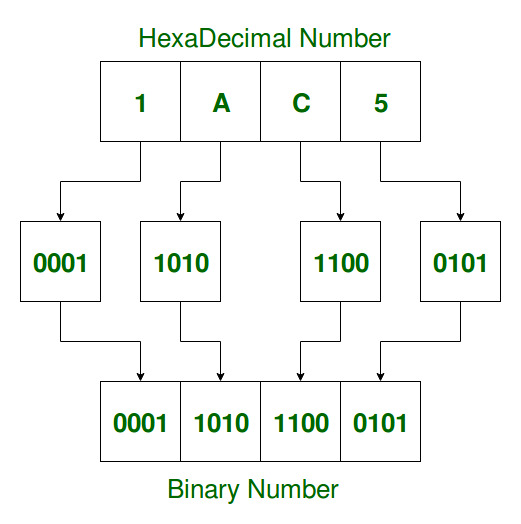
Javascript hex to decimal. By default, JavaScript displays numbers as base 10 decimals. But you can use the toString() method to output numbers from base 2 to base 36. Hexadecimal is base 16. Decimal is base 10. Octal is base 8. Binary is base 2. In this tutorial, we are going to learn about how to convert binary, hexadecimal and octal to decimal numbers in JavaScript. parseInt. In JavaScript, we have the parseInt() method by using that we can make conversions. JavaScript already offers a "native way" of doing it: // our decimal number const nr = 999999999; // convert to hex const hex = nr.toString(16); // back to dec: const decNr = parseInt(hex, 16); However, the example above can only handle numbers till Number.MAX_SAFE_INTEGER => 9007199254740991.
JavaScript Algorithm: Hex to Decimal | by Erica N, Usually this would not be a problem — JavaScript has built-in functions for converting between hex and decimal: parseInt("1234abcd", 16) = As the accepted answer states, the easiest way to convert from decimal to hexadecimal is var hex = dec.toString(16). However, you may prefer to add a ... Get code examples like "How to convert hexadecimal to decimal in JavaScript" instantly right from your google search results with the Grepper Chrome Extension. 4 weeks ago - A value passed as the radix argument ... to NaN), JavaScript assumes the following: If the input string begins with "0x" or "0X" (a zero, followed by lowercase or uppercase X), radix is assumed to be 16 and the rest of the string is parsed as a hexadecimal number. If the input string begins with any other value, the radix is 10 (decimal)...
I'm trying this out for a conversion app I am making, but the hex to decimal is not working as I'd expect it to. I enter: console.log(ConvertBase.hex2bin("13004E00")); // '1001 1000 0000 0010 0111 0000 0000 0' Jan 30, 2021 - As decimal numbers include just 0 to 9, it is a little bit difficult to read big numbers while hexadecimal numbers are base-16 and it is easy to read hexadecimal numbers. Javascript code for Hexadecimal to decimal conversion: Try our decimal to hex online converter tool. Hex to Dec in JavaScript Function parseInt() parses a string argument and returns an integer of the specified radix (the base in mathematical numeral systems).
17/12/2019 · You can easily convert hexa to decimal by javascript and also show its value in the html page by id. You have a well explained below how you can convert hexa decimal to decimal by javascript You can show it in html page by converting id. <script> function hexToDec (hex) { var result = 0, digitValue; hex = hex.toLowerCase (); for (var i = 0; i < hex. Java Convert Hexadecimal to Decimal. We can convert hexadecimal to decimal in java using Integer.parseInt() method or custom logic. Java Hexadecimal to Decimal conversion: Integer.parseInt() The Integer.parseInt() method converts string to int with given redix. The signature of parseInt() method is given below: JavaScript uses a 64-bit floating point representation to store all numbers, even integers. This means that integers larger than 2^53 cannot be represented precisely in JS. This becomes a problem if you need to work with 64-bit numbers, say to convert them between hexadecimal (base 16) and decimal (base 10).
Decimal to HexaDecimal. To convert a JavaScript Number to it's hexadecimal, toString() method is used. toString() method accepts a number parameter, ranging from 2 to 32, which specifies the base to use for representing the numeric values. (234). toString (16) // "ea" (-234). toString (16) // "-ea" Converting a negative number to hexadecimal ... The base can vary from 2 to 36.By default it's 10.. Common use cases for this are: base=16 is used for hex colors, character encodings etc, digits can be 0..9 or A..F.. base=2 is mostly for debugging bitwise operations, digits can be 0 or 1.. base=36 is the maximum, digits can be 0..9 or A..Z.The whole latin alphabet is used to represent a number. A funny, but useful case for 36 is when we ... Bin to Decimal. 100. 100 5391% of 1,586 9,787 wichu 1 Issue Reported. 6 kyu. Words to Hex. 32. 32 1693% of 192 604 jimmyhay. Beta. Convert recurring decimal to fraction.
Convert BigInt Hex to Decimal. There are two ways to go about this: Use the BigInt wrapper (which, much like Number, doesn't use new) Use the literal BigInt syntax (postfixing a number with n) Although you might expect a BigInt.parseInt to complement Number.parseInt (n, 16), that's not the case. Instead you must use base prefixes ( 0x for hex). See the Pen javascript-math-exercise-3 by w3resource (@w3resource) on CodePen. Improve this sample solution and post your code through Disqus. Previous: Write a JavaScript function to convert a binary number to a decimal number. Next: Write a JavaScript function to generate a random integer. The procedure for hex values with alpha should again be similar with the last. We simply detect a 4- or 8-digit value (plus #) then convert the alpha and divide it by 255. To get more precise output but not long decimal numbers for alpha, we can use toFixed(3).
Sometimes, you may need to convert a number from decimal to hexadecimal. It is done with the toString () method. This method takes the parameter, which is the base of the converted string. The base is 16. In this tutorial, we will show you how simple can be converting decimal number to hexadecimal in JavaScript. Convert BigInt Decimal to Hex. There are two ways to go about this: Use the BigInt wrapper (which, much like Number, doesn't use new) Use the literal BigInt syntax (postfixing a number with n) Either way, you'll have to correct for the same types of problems that Number.prototype.toString (base) has always had. Jul 20, 2021 - The toString() method returns a string representing the specified Number object.
Mar 31, 2020 - Get code examples like "convert int to hex javascript" instantly right from your google search results with the Grepper Chrome Extension. How to Convert a Decimal to Binary. To understand how to convert a decimal to base, it's useful to first understand how to convert a decimal to binary. Binary is base 2. We have two different digits to count with, 0 and 1. (Or true and false). To calculate a binary value, we need to divide the decimal by 2 until the quotient is zero. We store ... Most Modbus mastera nowadays can handle 6 digit addressing (4)xxxxx, which tops out at 65,535 registers. hex 0x0E28 could be (3)3625 or (4)3625 or (3)03625 (6 digit) or (4)03625 or some master's 3624 (there's no Modbus police to clean up bad implementations). 0x0E28 could also be a DI or coil register. 2. format.
Jul 20, 2021 - This chapter introduces the concepts, objects and functions used to work with and perform calculations using numbers and dates in JavaScript. This includes using numbers written in various bases including decimal, binary, and hexadecimal, as well as the use of the global Math object to perform ... JavaScript Algorithm: Hex to Decimal | by Erica N, Usually this would not be a problem — JavaScript has built-in functions for converting between hex and decimal: parseInt("1234abcd", 16) = As the accepted answer states, the easiest way to convert from decimal to hexadecimal is var hex = dec.toString(16). However, you may prefer to add a ... Example 1: javascript convert string to 2 decimal var twoPlacedFloat = parseFloat(yourString).toFixed(2) Example 2: javascript convert number to hex yourNumber = par
9/2/2014 · The algorithm itself is quite simple, so no need for a special library, it actually does what you would do on paper, so it is not too inefficient. function hexToDec (s) { var i, j, digits = [0], carry; for (i = 0; i < s.length; i += 1) { carry = parseInt (s.charAt (i), 16); for (j = 0; j < digits.length; j … The hex string "1502BE" is already the hexadecimal representation of the color where the first two-digit pair stands for red, the next for green, and the last for blue. What we need to do is split the string into these RGB pairs "15" for red, "02" for green and "BE" for blue. var aRgbHex = '1502BE'.match (/. {1,2}/g); Jul 17, 2014 - The radix parameter is used to specify which numeral system to be used, for example, a radix of 16 (hexadecimal) indicates that the number in the string should be parsed from a hexadecimal number to a decimal number. If the radix parameter is omitted, JavaScript assumes the following:
Accurate hexadecimal to decimal conversion in JavaScript. Posted in javascript, programming at 4:05 pm by danvk. A problem came up at work yesterday: I was creating a web page that received 64-bit hex numbers from one API. But it needed to pass them off to another API that expected decimal numbers. Get code examples like "convert number to hexadecimal in javascript" instantly right from your google search results with the Grepper Chrome Extension. ff 11. We can convert these numbers back to decimal using the parseInt function. The parseInt function available in JavaScript has the following signature −. parseInt (string, radix); Where, the paramters are the following −. string −The value to parse. If this argument is not a string, then it is converted to one using the ToString method.
Not a member? Sign Up · Demo of converting any decimal value to Hex Javascript provides a conversion method, and directly calls two functions to achieve conversion between the systems. Commonly used hexadecimal conversion: hex to decimal or decimal to hex, binary to decimal, hex, octal, octal to decimal, hex, etc. Then look at how to use javascript to realize these conversion between systems. Nov 07, 2019 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
See the Pen JavaScript Convert Hexadecimal to ASCII format - string-ex-28 by w3resource (@w3resource) on CodePen. Improve this sample solution and post your code through Disqus. Previous: Write a JavaScript function to convert ASCII to Hexadecimal format. Next: Write a JavaScript function to find a word within a string. This solution take on input decimal string, and return hex string. A decimal fractions are supported. Algorithm. split number to sign (s), integer part (i) and fractional part (f) e.g for -123.75 we have s=true, i=123, f=75; integer part to hex: if i='0' stop; get modulo: m=i%16 (in arbitrary precision) convert m to hex digit and put to result ... The parseInt () function parses a string and returns an integer. The radix parameter is used to specify which numeral system to be used, for example, a radix of 16 (hexadecimal) indicates that the number in the string should be parsed from a hexadecimal number to a decimal number. If the radix parameter is omitted, JavaScript assumes the following:
The goal of the function is to return the hex number's decimal equivalent. Examples: let hexString = "1" // 1. let hexString = "a" // 10. let hexString "10" // 16. A way you can solve this algorithm is to use the parseInt () method but with a radix. The parseInt method parses a string and returns an integer but it also accepts a second ...
Nodered How To Covert From Hex Number To Dec Number
Convert Hexadecimal Value String To Ascii Value String
How To Convert Hexadecimal To Decimal And Decimal To Hex
Converting From Decimal To Hexadecimal Representation Video
Numbering System How To Convert Hexadecimal Decimal Value
How To Convert From Decimal To Hexadecimal 15 Steps
Build A Random Hex Color Generator With Vanilla Javascript
Javascript Algorithm Hex To Decimal By Erica N
Coding A Bit Converter In Javascript Convert Between Binary Decimal And Hexadecimal
How To Convert From Decimal To Hexadecimal 15 Steps
Converting A String Type Hex Number To A Decimal Working
Program To Convert Hexadecimal Number To Binary Geeksforgeeks
Convert A Binary Number To Hexadecimal Number Geeksforgeeks
Hexadecimal To Decimal Converter
Hexadecimal To Decimal Vbforums
Javascript Basics Binary To Decimal Conversion Program For Beginners
How To Convert Hexadecimal To Decimal And Decimal To Hex
Hexadecimal To Decimal And Decimal To Hexadecimal Conversion
Python Hex How To Convert Integer To Hexadecimal Number
0 Response to "21 Javascript Hex To Decimal"
Post a Comment