35 How To Reverse An Array In Javascript
Since no one came up with it and to complete the list of ways to reverse an array... array.sort (function () { return 1; }) It's twice as fast as both while-approaches, but other than that, horribly slow. http://jsperf /js-array-reverse-vs-while-loop/53 The reverse () method reverses an array in place. The first array element becomes the last and the last becomes the first. The join () method joins all elements of an array into a string. function reverseString (str) { // Step 1.
How To Reverse An Array In Javascript Preserving The Original
The reverse method transposes the elements of the calling array object in place, mutating the array, and returning a reference to the array. reverse is intentionally generic; this method can be called or applied to objects resembling arrays.
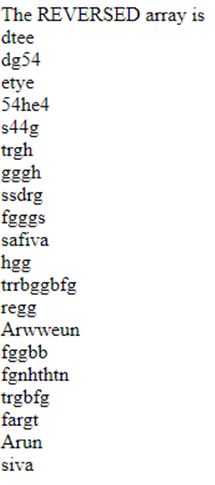
How to reverse an array in javascript. Array reverse () in JavaScript Javascript Web Development Object Oriented Programming The JavaScript Array reverse () function reverses the elements order in an array Following is the code for the array reverse () function − Reverse sum array JavaScript. We are required to write a function, say reverseSum () that takes in two arrays of Numbers, let's say first and second and returns a new array that contains, Sum of first element of first array and last element of second array as first element, 23/4/2019 · We will reverse the array in place without using any extra space. Using Array.prototype.reverse() method let arr = [1, 2, 3, 4, 5]; arr.reverse(); console.log(arr); //[5, 4, 3, 2, 1]
Aug 15, 2020 - A typical JavaScript challenge or interview question has to do with reversing arrays in-place and out-of-place. Specifically, take this problem from Eloquent JavaScript, 2nd Edition: Write two functions reverseArray and reverseArrayInPlace. The first, reverseArray, takes an array as an argument… 7/8/2021 · The easiest way to reverse an array in JavaScript is to use the reverse() function. This function is built-in and is supported in all browsers. The syntax is really easy, you just need to use a dot(.) operator with the array you want to reverse. Making a call to the reverse() function. Let’s see few JavaScript examples to reverse the array. Example 1: Reverse a number Array Aug 15, 2020 - Reversing an array in different ways is one of the most popular questions you can expect to get at a JavaScript interview. In this article, I will present three ways to reverse an array, and using a…
Jul 25, 2020 - The arr.reverse() method is used for in-place reversal of the array. The first element of the array becomes the last element and vice versa. ... Return value: This method returns the reference of the reversed original array. Below examples illustrate the JavaScript Array reverse() method: Jul 19, 2019 - Below are my three most interesting ways to solve the problem of reversing a string in JavaScript. ... Reverse the provided string. You may need to turn the string into an array before you can reverse it. Your result must be a string. How to Reverse an Array in JavaScript with the Spread Operator You can use a combination of the spread operator and the reverse method to reverse an array without changing the original. First, you put the elements returned from the spread operator into a new array by enclosing the spread syntax with square brackets []:
Once to reverse the entire array. Once to reverse from nums[0] to k. Once to reverse from k to the end. And we're done! The runtime complexity here is O(n * 3), since we still need to reverse each element at least once, and we'll be doing that three times. The space complexity here is, again, a constant O(1). Still great! This method reverse an array in place, that means the first element in an array becomes the last element and the last becomes the first. In JavaScript, by using reverse() method we can reverse the order of the elements in an array. The reverse() method is generally called as Array Reverse. EXAMPLE: Reversing the order of the elements in an array. How to reverse the order of a JavaScript array? How to get a decimal portion of a number with JavaScript? How to extract the hostname portion of a URL in JavaScript? JavaScript Array reverse() Array reverse() in JavaScript; Reverse an array in C++; Write a Golang program to reverse an array; Reverse sum array JavaScript; Java program to reverse ...
The JavaScript array reverse () method changes the sequence of elements of the given array and returns the reverse sequence. In other words, the arrays last element becomes first and the first element becomes the last. This method also made the changes in the original array. JavaScript Array reverse() JavaScript Array reverse() is an inbuilt function that reverses the order of the items in an array. The reverse() method will change the original array that is why it is not a pure function. The first array item becomes the last, and the last array element becomes the first due to the array reverse() method in Javascript. How to reverse an array in JavaScript? Here's a Code Recipe to keep around if you need to reverse the order of the elements of an array. You can use the array method, "reverse ()" ⏪ Trying a new segment called #CodeRecipes.
JavaScript Program to Reverse an Array Using Loops. Below is the JavaScript program to reverse an array using loops: Related: An Introduction to the Merge Sort Algorithm // JavaScript program to reverse the elements of an array using loops function reverseArr(arr, size) {for(let i=0, j=size-1; i<(size)/2; i++, j--) Using Array.prototype.reverse()function The standard method to reverse an array in JavaScript is using the reverse()method. This method operates in-place, meaning that the original array is modified, and no reversed copy is created. 1 Aug 27, 2019 - One of the most unconventional approaches that I rarely see in reverse string discussions is using the reduce() method. Once again, it only works for arrays, so first we need to split our string. Then we accumulate our values into an empty string that becomes a reverse of our original string ...
We see that our original array remains the same and reversed array is assigned to a new variable. Array.slice() and Array.reverse() You can also chain slice() method with reverse() method to make a new copy of reversed array. In JavaScript it has many type of reverse functions reverse array and reverse arrayInPlace these two functions will use in the different sequential in script.The reverse array it takes the array as an parameter and it produces a new array for the same elements in the descending order and the second method called reverseArrayInPlace is used for whatever we have achieved in the reverse method ... Javascript array reverse () method reverses the element of an array. The first array element becomes the last and the last becomes the first.
11/8/2021 · You could use a loop and reverse the array manually, but as we will see, in JavaScript there are much faster ways to achieve our goal: To start, let's start from the following array which we'll call 'letters': const letters = ['a', 'b', 'c', 'd','e']; How to reverse the array itself JavaScript Array reverse () Javascript Web Development Front End Technology Object Oriented Programming. The reverse () method of JavaScript is used to reverse the array elements. The syntax is as follows −. array.reverse () Let us now implement the reverse () method in JavaScript −. Apr 25, 2017 - Why won't this function reverseArrayInPlace work? I want to do simply what the function says - reverse the order of elements so that the results end up in the same array arr. I am choosing to do th...
Javascript has a reverse() method that you can call in an array. var a = [3,5,7,8]; a.reverse(); // 8 7 5 3 Not sure if that's what you mean by 'libraries you can't use', I'm guessing something to do with practice. ... I've made some test of solutions that not only reverse array but also makes its copy. Here is test code. Reverse an array using JavaScript. Syntax, examples & different methods. Jul 20, 2020 - I had the need to reverse a JavaScript array, and here is what I did. Given an array list: const list = [1, 2, 3, 4, 5] The easiest and most intuitive way is to call the reverse() method of an array. This method alters the original array, so I can declare list as a const, because I don’t ...
array.pop () removes the popped element from the array, reducing its size by one. Once you're at i === 4, your break condition no longer evaluates to true and the loop ends. Something theoretically so simple can become a problem if you are new to JavaScript and didn't know the default behavior of the inherited reverse method of an array. In JavaScript is pretty easy to reverse the current order of the items of an array in JavaScript using the mentioned method: Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
Nov 26, 2019 - We can reverse an array by both modifying the source array or without modifying the source array. We will look at different ways to do each. 2. Using the map method with the unshift array method… Explanation. In the first line the array is defined. Then with the size variable, we will store the length of the array. After that, we traverse the loop in reverse order, which is starting with "size-1" index, which is 4 in our case and we are iterating till the 0th index. Inside the loop, we print or work on our element. 1. JavaScript Built-in Methods. The best way to reverse a string is by using three different JavaScript built-in methods: split (), reverse () and join (). split () - It splits a string into an array of substrings using a separator, and returns the new array. reverse () - This method reverses the order the elements in an array.
Reverse an array in Java. Reversing an array in java can be done in three simple methods. (i) Take input the size of the array and the elements of the array. (ii) Consider a function reverse which takes the parameters-the array (say arr) and the size of the array (say n). (iii) Inside the function, a new array (with the array size of the first ...
How To Sort And Reverse An Array In Javascript
Javascript Array Reverse How To Reverse Array Items
How To Reverse An Array In Javascript Samanthaming Com
Reverse The Order Of An Array In Vue Js Javascript Renat
Salt Array Of Js Algorithm 3 Aes Block In This Block We
Javascript Three Ways To Reverse An Array By Bahay Gulle
Javascript Tutorial 19 Reverse An Array
Vue Js Reverse Array Tutorial Example Tuts Make
Javascript Three Ways To Reverse An Array By Bahay Gulle
Javascript Coding Interview Question 1 Reverse An Array
Javascript Basic Reverse The Elements Of A Given Array Of
Reverse An Array In Groups Of Given Size Geeksforgeeks
Reverse Array Method Javascript Tutorial
How To Reverse A String In Javascript By James Kerrane
Javascript Reverse Array Tutorial With Example Js Code
Print Array Elements In Reverse Order Javascript Code Example
Javascript Reverse Array Tutorial With Example Js Code
Reverse Array Javascript Tutorial 2021
Five Ways To Reverse An Array In Javascript By Javascript
The Javascript Array Handbook Js Array Methods Explained
Dynamic Array In Javascript Using An Array Literal And
Is There A Way To Use Map On An Array In Reverse Order With
Reverse Array Travarsal Using Javascript Stack Overflow
20 Javascript Array Methods Dev Community
How To Reverse Arrays In Javascript Without Using Reverse
Reverse Array Java Example Examples Java Code Geeks 2021
Get The Last Item In An Array Stack Overflow
5 Working With Arrays And Loops Javascript Cookbook Book
How To Reverse Arrays In Javascript Without Using Reverse
Javascript Reverse Array How Does Javascript Reverse Array
More Javascript Array Tips Removing Falsy Values Random
0 Response to "35 How To Reverse An Array In Javascript"
Post a Comment