27 Javascript Class Multiple Inheritance
JavaScript class are not pure class like OOPs. JavaScript class does not support multiple inheritance and hybrid inheritance. To implement multiple inheritance, we need to do some JavaScript coding trick. We will build the same example used in above using multiple inheritance concept. The JavaScript inheritance is a mechanism that allows us to create new classes on the basis of already existing classes. It provides flexibility to the child class to reuse the methods and variables of a parent class. The JavaScript extends keyword is used to create a child class on the basis of a parent class.
Multiple Inheritance Java Bytesofgigabytes
In JavaScript, class inheritance is implemented on top of prototypal inheritance, but that does not mean that it does the same thing: JavaScript's class inheritance uses the prototype chain to wire...
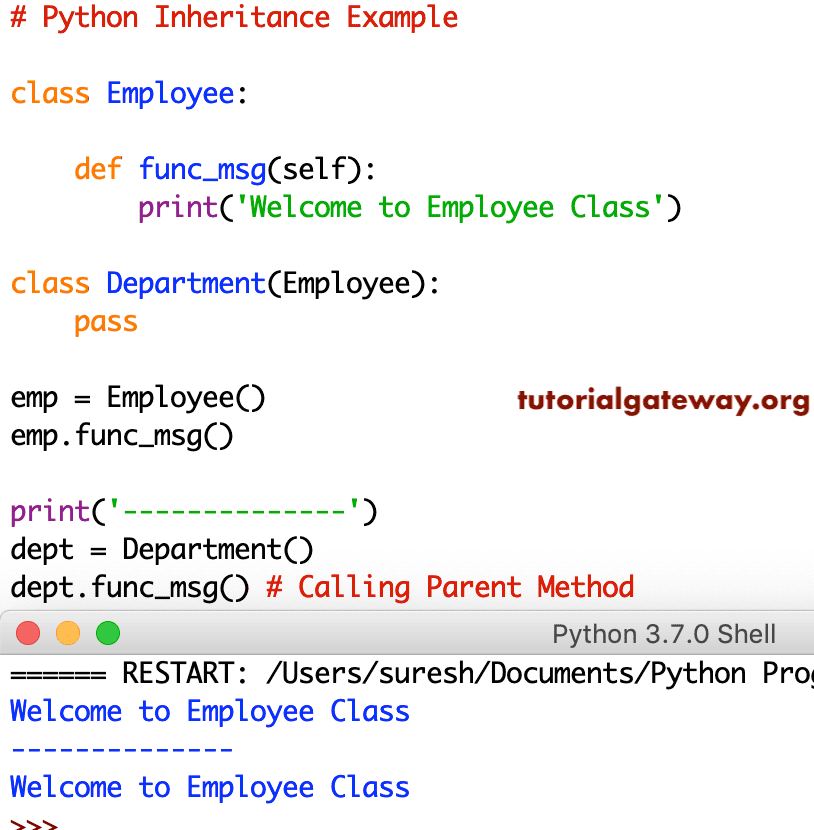
Javascript class multiple inheritance. Multiple inheritance opens a huge can of worms when dealing with JavaScript, which has a linear prototype chain. My solution to the problem was simple: when inheriting from multiple classes, I actually create a new prototype chain made up of clones of every prototype (even the ones deep in) of the constructor you want to inherit from, avoiding ... I've done most of my research on this on BabelJS and on MDN (which has no information at all), but please feel free to tell me if I have not been careful enough in looking around for more information JavaScript: ES6 Multiple Inheritance Class. Phần quan trọng của bài viết. Thật ra từ khi ES6 ra đời thì tôi càng yêu thích lập trình javascipt hơn bất kỳ các ngôn ngữ nào tôi biết. Nhưng chỉ riêng thiếu một thứ của ES6 đó là việc kế thừa nhiều class. Việc đó khiến các devjs ...
Abstract subclasses or mix-ins are templates for classes. An ECMAScript class can only have a single superclass, so multiple inheritance from tooling classes, for example, is not possible. The functionality must be provided by the superclass. JavaScript Class Inheritance Previous Next Class Inheritance. To create a class inheritance, use the extends keyword. A class created with a class inheritance inherits all the methods from another class: Example. Create a class named "Model" which will inherit the methods from the "Car" class: The following table defines the first browser version with full support for Classes in JavaScript: Chrome 49. Edge 12. Firefox 45. Safari 9. Opera 36. Mar, 2016.
Jul 03, 2019 - Answer No, in JavaScript, a class cannot extend from multiple classes, which is also known as “multiple inheritance”. In JavaScript, objects can only be associated with a single prototype, and extending multiple classes would mean that an object associates with multiple prototypes, which ... Polymorphism (having different functions at multiple levels of an inheritance chain with the same name) may seem like it implies a referential relative link from child back to parent, but it’s still just a result of copy behavior. JavaScript does not automatically create copies (as classes imply) ... In this tutorial, you will learn about JavaScript class inheritance with the help of examples.
Jul 20, 2021 - JavaScript is an object-based language based on prototypes, rather than being class-based. Because of this different basis, it can be less apparent how JavaScript allows you to create hierarchies of objects and to have inheritance of properties and their values. Inheritance is an important concept in object oriented programming. In the classical inheritance, methods from base class get copied into derived class. In JavaScript, inheritance is supported by using prototype object. Some people call it "Prototypal Inheriatance" and some people call it "Behaviour Delegation". If you want to mix multiple classes together, because ES6 classes only support single inheritance, you need to create a chain of classes that contains all the classes you want to mix together. So let's say you want to create a class C that extends both A and B, you could do this: class A {} class B extends A {} class C extends B {} // C ...
Jan 31, 2020 - In JavaScript, there’s no easy way to inherit from multiple classes. We can make our own mixins to inherit from multiple objects. This is made easier in TypeScript by making mixins a standard. With… JavaScript allows you to extend a built-in type such as Array, String, Map, and Set through inheritance. The following Queue class extends the Array reference type. The syntax is much cleaner than the Queue implemented using the constructor/prototype pattern. 22/1/2021 · Create a new class ExtendedClock that inherits from Clock and adds the parameter precision – the number of ms between “ticks”. Should be 1000 (1 second) by default. Your code should be in the file extended-clock.js; Don’t modify the original clock.js. Extend it. Open a sandbox for the task.
multiple inheritance, allowing us to make a class built from the methods of multiple classes. Promiscuous multiple inheritance can be difficult to implement and can potentially suffer from method name collisions. We could implement promiscuous multiple inheritance in JavaScript, but for this example Multiple inheritance occurs when a class inherits from more than one base class. So the class can inherit features from multiple base classes using multiple inheritance. This is an important feature of object oriented programming languages such as C++. A diagram that demonstrates multiple inheritance is given below − 28/5/2017 · // Class for creating multi inheritance. class multi { // Inherit method to create base classes. static inherit(..._bases) { class classes { // The base classes get base() { return _bases; } constructor (..._args) { var index = 0; for (let b of this.base) { let obj = new b(_args[index++]); multi.copy(this, obj); } } } // Copy over properties and methods for (let base of _bases) { multi.copy(classes, base); multi.copy(classes.prototype, base.prototype); } return classes ...
25/4/2015 · in javascript you cant give to a class (constructor function) 2 different prototype object and because inheritance in javascript work with prototype soo you cant do use more than 1 inheritance for one class but you can aggregate and join property of Prototype object and that main property inside a class manually with refactoring that parent classes and next extends that new version and joined class to your target class … Jul 02, 2018 - ITNEXT is a platform for IT developers & software engineers to share knowledge, connect, collaborate, learn and experience next-gen technologies. 21/10/2010 · For this evolutionary exploration, I am going to create three Javascript classes: Human, Monkey, and Ben (that's me!). The Ben class will inherit from both the Human class and the Monkey class, both of which define a swing() and walk() method. Person. swing() walk() Monkey. swing() walk() Ben (Extends Person and Monkey) highFive() walk()
17/9/2019 · JavaScript does not support multiple inheritance. Inheritance of property values occurs at run time by JavaScript searching the prototype chain of an object to find a value. Since every object has a single associated prototype, it cannot dynamically inherit from more than one prototype chain. Javascript extend multiple classes. ES6 Class Multiple inheritance, Inheriting from two classes can be done by creating a parent object as a combination of two parent prototypes. B mixin, will need a wrapper over it to be used const B = (B) => class extends Lets take a look at how inherited props work in js There is a little known feature in TypeScript that allows you to use Mixins to create ... Inheritance with class syntax Above we created a class to represent a person. They have a series of attributes that are common to all people; in this section we'll create our specialized Teacher class, making it inherit from Person using modern class syntax. This is called creating a subclass or subclassing.
When an object or class inherits the characteristics and features form more than one parent class, then this type of inheritance is known as multiple inheritance. Thus, a multiple inheritance acquires the properties from more than one parent class. TypeScript does not support multiple inheritance. JavaScript does not support multiple inheritance, but mixins can be implemented by copying methods into prototype. We can use mixins as a way to augment a class by adding multiple behaviors, like event-handling as we have seen above. Mixins may become a point of conflict if they accidentally overwrite existing class methods. Inheritance with JavaScript prototypes. November 22, 2019 6 min read 1897. TL;DR: In this post, we will look at prototypes and how to use them for inheritance in JavaScript. We will also see how the prototypical approach is different from class-based inheritance.
In JavaScript, one class inherits other classes using the " extends " keyword. The child class will have access to all the methods of the parent class, and you can invoke all the methods of the parent class using the child class object. So, JavaScript uses a similar concept for creating an inheritance by chaining multiple instances to prototypes and creating nested prototypes. For example, the object shown in the above image is ... Classes in JavaScript do not actually offer additional functionality, and are often described as providing "syntactical sugar" over prototypes and inheritance in that they offer a cleaner and more elegant syntax.
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. JavaScript is a prototype-based language, meaning object properties and methods can be shared through generalized objects that have the ability to be cloned and extended. This is known as prototypical inheritance and differs from class inheritance. Aug 07, 2015 - If you want to continue to use ... for classes that inherit from several things, so that you could just inherit from each mixin, and have everything be great. Unfortunately, it would be quite jarring to change the inheritance model now, so JavaScript does not implement multiple inheritance ...
To bring the traditional classes to JavaScript, ES2015 standard introduces the class syntax: a syntactic sugar over the prototypal inheritance. This post familiarizes you with JavaScript classes: how to define a class, initialize the instance, define fields and methods, understand the private and public fields, grasp the static fields and methods. Jul 26, 2021 - JavaScript is a bit confusing for developers experienced in class-based languages (like Java or C++), as it is dynamic and does not provide a class implementation per se (the class keyword is introduced in ES2015, but is syntactical sugar, JavaScript remains prototype-based). Dec 07, 2015 - Original post: Multiple inheritance [edit, not proper inheritance of type, but of properties; mixins] in Javascript is pretty straightforward if you use constructed prototypes rather than generic-object ones. Here are two parent classes to inherit from:
Feb 22, 2015 - In JavaScript, specifically the React (the apple of God’s eye), we can use Mixins when create a new class. In fact, is such a great pattern to archive Multiple Inheritance. So I made this kind of example on how you can reach this using ES6 and npm’s mixin library. Multiple Inheritance with Class Factories Another approach the JavaScript community has been experimenting with is generating classes on demand that extend a variable superclass. Feb 21, 2019 - Future Studio provides on-demand learning & wants you to become a better Android (Retrofit, Gson, Glide, Picasso) and Node.js/hapi developer!
Pdf A Comparative Study On The Effect Of Multiple
Javascript Efficient Solution For Multi Inheritance Stack
Node Js Extend Multiple Classes Multi Inheritance
Js To Cs Conversion Problem With Multiple Class Files Unity
Java 8 Multiple Inheritance Conflict Resolution Rules And
Details Of The Object Model Javascript Mdn
Difference Between Single And Multiple Inheritance In C
Multiple Inheritance In Php Geeksforgeeks
Inheritance In Java Oops Learn All Types With Example
Advanced Programming Behnam Hatami Fall 2017 Agenda Inheritance
Multiple Inheritance In Java Java Tutorials Dream In Code
Understanding And Using Prototypical Inheritance In Javascript
How To Connect Objects With Each Other In Different
Python Multiple Inheritance Explained With Examples
Why Does Java C Etc Doesn T Support Multiple Inheritance
Implement Multiple Inheritance In C
Inheritance Versus Composition How To Choose Infoworld
Java Inheritance Types Extends Class With Examples Eyehunts
Inheritance In Java Object Oriented Programming Concepts
Python Multiple Inheritance What Is It And How To Use It
Java Program To Implement Multiple Inheritance
Python Multiple Inheritance What Is It And How To Use It
Multiple Inheritance In Java With Example Computer Notes
0 Response to "27 Javascript Class Multiple Inheritance"
Post a Comment