29 Create A Function Javascript
The first example uses a regular function, and the second example uses an arrow function. The result shows that the first example returns two different objects (window and button), and the second example returns the window object twice, because the window object is the "owner" of the function. Dec 02, 2018 - There are a few different ways to define a function in JavaScript: A Function Declaration defines a named function. To create a function declaration you use the function keyword followed by the name of the function. When using function declarations, the function definition is hoisted, thus ...
The syntax for creating a function: let func = new Function ([ arg1, arg2,... argN], functionBody); The function is created with the arguments arg1...argN and the given functionBody. It's easier to understand by looking at an example.
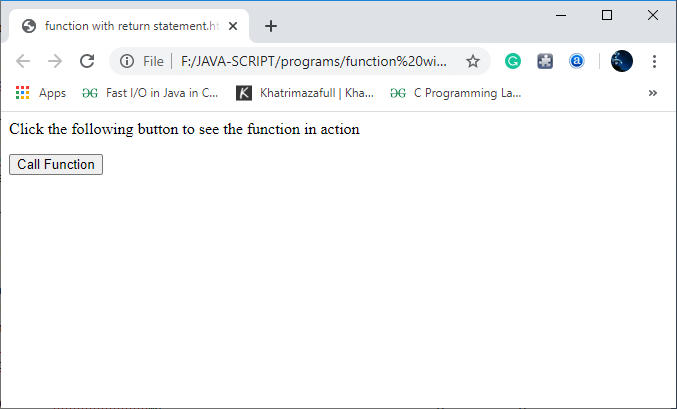
Create a function javascript. A function is a block of code that performs an action or returns a value. Functions are custom code defined by programmers that are reusable, and can therefore make your programs more modular and efficient. In this tutorial, we will learn several ways to define a function, call a function, and use function parameters in JavaScript. 27/8/2021 · JavaScript Return Value; How to Create a Function in JavaScript. Use the keyword function followed by the name of the function. After the function name, open and close parentheses. After parenthesis, open and close curly braces. Within curly braces, write your lines of code. Syntax: function functionname() { lines of code to be executed } Try this yourself: There are many ways in which objects in JavaScript differ from objects in other mainstream programming languages, like Java. I will try to cover that in a another topic. Here, let us only focus on the various ways in which JavaScript allows us to create objects. In JavaScript, think of objects as a collection of 'key:value' pairs.
The second method of creating a JavaScript object is using a constructor function. As opposed to object literals, here, you define an object type without any specific values. Then, you create new object instances and populate each of them with different values. In JavaScript, a constructor function is used to create objects. For example, // constructor function function Person () { this.name = 'John', this.age = 23 } // create an object const person = new Person (); In the above example, function Person () is an object constructor function. To create an object from a constructor function, we use the ... How to create a function from a string in JavaScript ? 11, Oct 19. How to create a function that invokes the method at a given key of an object in JavaScript ? 19, May 21. How to create fullscreen search bar using HTML , CSS and JavaScript ? 17, Mar 21. Object.create( ) In JavaScript.
A JavaScript function is defined with the function keyword, followed by a name, followed by parentheses (). Function names can contain letters, digits, underscores, and dollar signs (same rules as variables). The parentheses may include parameter names separated by commas: (parameter1, parameter2,...) 11/10/2019 · The task is to create a function from the string given in the format of function. Here are few techniques discussed with the help of JavaScript. Approach 1: Use the Function() Constructor to create a function from the string. It accepts any number of arguments(in form of string). Last one should be the body of the function. proto. The object which should be the prototype of the newly-created object. propertiesObject Optional. If specified and not undefined, an object whose enumerable own properties (that is, those properties defined upon itself and not enumerable properties along its prototype chain) specify property descriptors to be added to the newly-created object, with the corresponding property names.
Functions are one of the fundamental building blocks in JavaScript. A function in JavaScript is similar to a procedure—a set of statements that performs a task or calculates a value, but for a procedure to qualify as a function, it should take some input and return an output where there is some obvious relationship between the input and the output. 22/8/2019 · The Function constructor is something that isn't usually talked about in JavaScript circles, but it is a useful mechanism to have that allows you to generate functions dynamically based on a string. Here is a quick example of it in action before we dive into it a bit more. Apr 28, 2020 - We'll look at the four ways of creating a function in JavaScript: as a statement, as an expression, as an arrow function, and using the Function constructor.
A namespace prepends itself to your custom functions to help customers identify your functions as part of your add-in. Uses <ExtensionPoint> and <Resources> elements that are unique to a custom functions manifest. These elements contain the information about the locations of the JavaScript, JSON, and HTML files. Nov 02, 2019 - When calling ok(), JavaScript throws ReferenceError: ok is not defined, because the function declaration is inside a conditional block. The function declaration in conditionals is allowed in non-strict mode, which makes it even more confusing. As a general rule for these situations, when a function should be created ... then returns to the spot in the program where the function was called. 4:20. In fact, in this code, there are built-in JavaScript functions at work, Math.floor, 4:23. Math.random, and alert. 4:28. So when each of those are called, 4:29. the JavaScript engine has to jump into them and execute their code, 4:31.
Instead of declaring and executing the functions in 2 different steps, JavaScript also provides an approach to declare and execute the function immediately. This is also called as IIFE (stands for ... Jul 20, 2021 - Functions are one of the fundamental building blocks in JavaScript. A function in JavaScript is similar to a procedure—a set of statements that performs a task or calculates a value, but for a procedure to qualify as a function, it should take some input and return an output where there is ... In JavaScript, we can make functions that are able to return a value. To create such type of function, we have to use the return statement, but it must be the last statement in the body of the function (or in the definition of the function). Another essential thing to remember is that we can use only one return statement in a function.
18/2/2019 · let abc = 0; Function_A(2, function(result) { abc = result; //should by 4 });//Get the results of Function A function Function_A(myVar, callback) { callback(myVar * 2); } Share Improve this answer Considerations for JavaScript functions. When you work with JavaScript functions, be aware of the considerations in the following sections. Choose single-vCPU App Service plans. When you create a function app that uses the App Service plan, we recommend that you select a single-vCPU plan rather than a plan with multiple vCPUs. A function is a parametric block of code defined once and called multiple times later. In JavaScript a function is composed and influenced by many components: JavaScript code that forms the function body The list of parameters
Jun 21, 2021 - We’ve already seen examples of built-in functions, like alert(message), prompt(message, default) and confirm(question). But we can create functions of our own as well. ... To create a function we can use a function declaration. The functions of JavaScript The function is a way to create a block of code that can be reused in JavaScript programs again and again by simply calling the function. Function in JavaScript may take one or more parameters and may also return value. A JS function code will execute only when it is called. JavaScript functions have both properties and methods. The arguments.length property returns the number of arguments received when the function was invoked: ... A function defined as the property of an object, is called a method to the object. A function designed to create new objects, is called ...
4 days ago - Functions are very important and useful in any programming language as they make the code reusable A function is a block of code which will be executed only if it is called. If you have a few l 3. Write a JavaScript function that generates all combinations of a string. Go to the editor. Example string : 'dog'. Expected Output : d,do,dog,o,og,g. Click me to see the solution. 4. Write a JavaScript function that returns a passed string with letters in alphabetical order. Go to the editor. Four Ways to Create a Function in JavaScript Four Ways to Create a Function in JavaScript We'll look at the four ways of creating a function in JavaScript: as a statement, as an expression, as an arrow function, and using the Function constructor. "I wish undefined was a function in JavaScript."
// to create a jQuery function, you basically just extend the jQuery prototype // (using the fn alias) $.fn.myfunction = function () { // blah }; Inside that function, the this variable corresponds to the jQuery wrapped set you called your function on. We just learned that function objects are copied by reference. However, when modifying the actual function body, things are a bit different because this will cause a new function object to be created. In the next example the original function body is changed and JavaScript will create a new function object. Normally, a function defined would not be accessible to all places of a page. Say a function is mentioned using "function checkCookie()" on a external file loaded first on a page. This function cannot be called by the JavaScript file loaded last. So you have to make it a Global function that can be called from anywhere on a page.
JavaScript Functions . JavaScript provides functions similar to most of the scripting and programming languages. In JavaScript, a function allows you to define a block of code, give it a name and then execute it as many times as you want. A JavaScript function can be defined using function keyword. 30/6/2021 · A Function is a block of statements that is designed to perform a particular task. //defining a function function test(){ console.log ("Hi from Function"); } //calling the function test () There are four ways a function can be defined in JavaScript. They are as follows: Function Declaration. Function Expression. Arrow Function. It works like creating a new function, but as functions are objects in JavaScript, you create an object. When an object is created using the constructor, it inherits all its properties and methods: Example Copy
JavaScript - Functions, A function is a group of reusable code which can be called anywhere in your program. This eliminates the need of writing the same code again and again. It helps Bindings declared with let and const are in fact local to the block that they are declared in, so if you create one of those inside of a loop, the code before and after the loop cannot “see” it. In pre-2015 JavaScript, only functions created new scopes, so old-style bindings, created with ... The Function () constructor expects any number of string arguments. The last argument is the body of the function - it can contain arbitrary JavaScript statements, separated from each other by semicolons. Notice that the Function () constructor is not passed any argument that specifies a name for the function it creates.
Javascript Functions Concept To Ease Your Web Development
Using Method Chaining With The Revealing Module Pattern In
Creating A Javascript Function To Apply Css Rules To A
How To Write A Function In Javascript Javatpoint
Using A User Defined Function Javascript For Lora Networks
Basic Javascript Write Reusable Javascript With Functions
Three Ways To Create A Javascript Class Learn Web Tutorials
Learn How To Get Current Date Amp Time In Javascript
Javascript Functions Understanding The Basics By Brandon
Javascript Reports Documentation
Create A Selection Sort Function In Javascript
Javascript Fundamental Es6 Syntax Create A Function That
Javascript Hello World How To Write Your First Program In
Javascript Functions Akanksha S Blog
Here Are The New Built In Methods And Functions In Javascript
Four Ways To Create A Function In Javascript
Javascript Functions Javascript Tutorial
Getting To Know Javascript Callbacks Create Callback
Create A Function In Javascript
Javascript Create Class And Call Function Within The Class
Exploring Javascript S Eval Capabilities And Closure Scoping
Javascript Objects How To Create Amp Access An Object In
Suitescript 2 1 Delivers Modern Javascript Syntax For Server
How To Create A Custom Callback In Javascript Geeksforgeeks
Three Ways To Create A Javascript Class Learn Web Tutorials
Snowflake User Defined Functions Syntax And Examples
Object Create In Javascript The Object Create Method Is One
Serverless Node Js Code With Azure Functions Azure
0 Response to "29 Create A Function Javascript"
Post a Comment