23 What Is Class Javascript
JavaScript uses prototypal inheritance, which is different from classical inheritance. Classical inheritance is normally the foundation for building classes. For example, one class can extend another class (i.e. build atop). Inheritance is possible in JavaScript, but operates differently under the hood. JavaScript classList is a DOM property of JavaScript that allows for styling the CSS (Cascading Style Sheet) classes of an element. JavaScript classList is a read-only property that returns the names of the CSS classes. It is a property of JavaScript with respect to the other properties of JavaScript that includes style and className.
Javascript Class How To Define And Use Class In Javascript
Sep 05, 2018 - In order to understand the idea behind JS classes we need to understand the constructor functions, prototype and other related concepts. ... Since Javascript is a functional programming language where everything is just a function, in order to have a class like (creating a blueprint for the ...
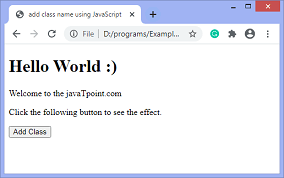
What is class javascript. Java Script is a Dynamic Scripting language used along with HTML. It is an interpreted language that is used to add dynamics content in web pages. There are a number of events that will trigger the execution of JavaScript. The JavaScript client-side mechanism provides many advantages over traditional CGI server-side scripts. 14/8/2012 · Class. In a class-based object-oriented language, in general, state is carried by instances, methods are carried by classes, and inheritance is only of structure and behavior. In ECMAScript, the state and methods are carried by objects, and structure, behavior, and state are all inherited. Babel is a JavaScript compiler. Classes in JavaScript 5. From Mozilla MDN page about classes: JavaScript classes, introduced in ECMAScript 2015, are primarily syntactical sugar over JavaScript's existing prototype-based inheritance. The class syntax does not introduce a new object-oriented inheritance model to JavaScript. The key concept here is prototype-based inheritance.
ECMAScript 2015 (ES6), for the first time, introduced the concept of classes in JavaScript. In an object-oriented programming language, a class is a template for creating objects with properties and methods of the same kind. However, that is not the case in JavaScript. Class inheritance is a way for one class to extend another class. So we can create new functionality on top of the existing. ... In JavaScript, there's a distinction between a constructor function of an inheriting class (so-called "derived constructor") and other functions. 11/5/2021 · A class definition sets enumerable flag to false for all methods in the "prototype". That’s good, because if we for..in over an object, we usually don’t want its class methods. Classes always use strict. All code inside the class construct is automatically in strict mode. Besides, class syntax brings many other features that we’ll explore later.
May 02, 2019 - If you’re new to programming, or javascript you’ve probably heard of objects, and classes. Understanding what a class is can be difficult, you’ll hear words like “this”, “instances”, “objects”… The static keyword defines a static method or property for a class. Neither static methods nor static properties can be called on instances of the class. Instead, they're called on the class itself. Static methods are often utility functions, such as functions to create or clone objects, whereas static properties are useful for caches, fixed-configuration, or any other data you don't need to ... JavaScript ( / ˈdʒɑːvəˌskrɪpt / ), often abbreviated as JS, is a programming language that conforms to the ECMAScript specification. JavaScript is high-level, often just-in-time compiled, and multi-paradigm. It has curly-bracket syntax, dynamic typing, prototype-based object-orientation, and first-class functions .
A JavaScript class is not an object. It is a template for JavaScript objects. Definition and Usage The classList property returns the class name (s) of an element, as a DOMTokenList object. This property is useful to add, remove and toggle CSS classes on an element. The classList property is read-only, however, you can modify it by using the add () and remove () methods. ECMAScript 2015, also known as ES6, introduced JavaScript Classes. JavaScript Classes are templates for JavaScript Objects.
A class is a type of function, but instead of using the keyword ' function ', keyword ' class ' is used to initiate it, and the properties are assigned inside a constructor () method. The constructor () method is called each time the class object is initialized. Example-1 Classes in JavaScript was introduced in the ES6/ECMAScript2015. A class is a type of function with keyword class. Class is executed in strict mode, So the code containing the silent error or mistake throws an error. JavaScript classes can have a constructor method, which is a special method that is called each time when the object is created. A class has a default constructor which is empty but can also be defined within the class method. Unlike in other languages, to declare the constructor, Javascript has a specific keyword called constructor. The parameter(s) given to the constructor are then used to define elements within the ...
27/8/2019 · A class is an extensible program-code-template for creating objects. A javascript class is a type of function and are declared with the class keyword. Classes The concept of classes was introduced in JavaScript in ES6 (ECMA2015). In the object-oriented programming paradigm, a class is a blueprint for creating objects with properties and methods while encapsulating the implementation details from the user. However, the concept of true classes does not exist in JavaScript. Classes JavaScript is different from other object-oriented languages. It is based on constructors and prototypes rather than on classes. For a long time classes were not used in JavaScript.
Dec 13, 2019 - This post familiarizes you with JavaScript classes: how to define a class, initialize the instance, define fields and methods, understand the private and public fields, grasp the static fields and methods. ... The code above defines a class User. The curly braces { } delimit the class body. Note that this syntax is ... Nov 06, 2018 - Object Oriented Programming (OOP) is without question an important advancement in the design and development of software. In fact languages such as C++ and later Java were developed primarily around… Learn JavaScript is the easiest, most interactive way to learn & practice modern JavaScript online. Read short lessons, solve challenges & answer flashcards.
Nov 23, 2018 - JavaScript is an oddball of a language with numerous approaches to almost any problem. Did ES6 classes help or just muddy the waters? Find out what veteran JavaScript developer Justen Robertson thinks about OOP in JS. Jul 20, 2021 - The class declaration creates a new class with a given name using prototype-based inheritance. It does have a class keyword as part of its class syntax for creating prototypes—but still no thing called class. JavaScript is not now and has never been a classical OOP language. Speaking of JS in terms of class is only either misleading or a sign of not yet grokking prototypical inheritance ...
JavaScript | Classes. Introduction of new version of JavaScript (ES6) introduced the use of classes instead of functions.Classes are similar to functions.They use class keyword instead of function keyword. They use constructor method to initialise. ES6 Classes formalize the common JavaScript pattern of simulating class-like inheritance hierarchies using functions and prototypes. They are effectively simple sugaring over prototype-based OO, offering a convenient declarative form for class patterns which encourage interoperability. In the other programming languages like Java or C#, a class can have one or more constructors. In JavaScript, a function can have one or more parameters. So, a function with one or more parameters can be used like a constructor where you can pass parameter values at the time or creating an object with new keyword.
The class syntax is not introducing a new object-oriented inheritance model to JavaScript. JavaScript classes provide a much simpler and clearer syntax to create objects and deal with inheritance. -Mozilla Developer Network. Example: Lets use ES6 classes then we will look into traditional way of defining Object and simulate them as classes. In the first implementation, we store action and counter in the environment of the class constructor. An environment is the internal data structure, in which a JavaScript engine stores the parameters and local variables that come into existence whenever a new scope is entered (e.g. via a function ... Classes. Classes are a template for creating objects. They encapsulate data with code to work on that data. Classes in JS are built on prototypes but also have some syntax and semantics that are not shared with ES5 class-like semantics.
May 04, 2018 - Understanding prototypical inheritance is paramount to being an effective JavaScript developer. Being familiar with classes is extremely helpful, as popular JavaScript libraries such as React make frequent use of the class syntax. Creating a Class in JavaScript In programming, a class can be seen as a generalized entity that is used to create a specialized object. For example, in a school environment, a generalized entity (a class) can be students and an object of students can be John Brown. Class Declarations Example: Hoisting. Unlike function declaration, the class declaration is not a part of JavaScript hoisting. So, it is required to declare the class before invoking it. Let's see an example. <script>. //Here, we are invoking the class before declaring it. var e1=new Employee (101,"Martin Roy"); var e2=new Employee (102,"Duke ...
A JavaScript class is a blueprint for creating objects. A class encapsulates data and functions that manipulate data. Unlike other programming languages such as Java and C#, JavaScript classes are syntactic sugar over the prototypal inheritance. In other words, ES6 classes are just special functions. This program will create a JavaScript object that you can easily understand with the help of the diagram below.. Output: In the above program, we defined a class Shape with a constructor() function. We instantiated the class i.e. created an object named shape1.The browser window in the output above shows the text we wanted. What is a Class in JavaScript? Classes in JavaScript are a type of function only, but instead of using the keyword " function ", the keyword " class " is used to declare a class. Its syntax looks like below: class classname { //variables and methods which need to as part of an object }
Dec 12, 2018 - The most popular way to structure any type of software project nowadays is by using classes. In this post # 15, we are going to explore different ways to implementing classes in JavaScript and how we can build class hierarchies. We’ll start by diving into how prototypes work and analyze ways ... A JavaScript class is a type of function. Classes are declared with the class keyword. We will use function expression syntax to initialize a function and class expression syntax to initialize a class. const x = function () {} const y = class {} Aug 06, 2020 - Classes in JavaScript are a special syntax for its prototypical inheritance model that is a comparable inheritance in class-based object oriented languages. Classes are just special functions added to ES6 that are meant to mimic the class keyword from these other languages.
Nov 06, 2019 - Classes in JavaScript are a special syntax for its prototypical inheritance model that is a comparable inheritance in class-based object oriented languages. Classes are just special functions added… Introduction to Abstract Classes in JavaScript JavaScript is an Object-Oriented Language since most of the elements in javascript are objects expect the primitive data types. In Object-Oriented Programming (OOP), the concept of abstraction is to hide the implementational details and showcase essential features of the object to its users.
The Flavors Of Object Oriented Programming In Javascript
Classes And Sub Classes In Javascript
Calling Out From Java To Javascript With Call Back
Get The Closest Element By Selector
Addy Osmani On Twitter Javascript Public Class Fields Are
Ruby Classes Vs Javascript Constructors
Toggle Class Javascript Based Without Jquery Qa With Experts
Javascript Es6 Classes Objects In Programming Languages
Javascript Classes Javascript Codecademy Forums
Making Sense Of Es6 Class Confusion Toptal
Unpacking Javascript Classes Let S Cut To The Chase So
Adding Methods To Our Class How To Object Oriented
Using Static Class Methods To Generate Concrete Instances Of
Example Of Class In Javascript Es6 Learn Web Tutorials
Local Class In Abap Java And Javascript Sap Blogs
Tools Qa How To Use Javascript Classes Class Constructor
An Overview Of Classes In Javascript By Tran Son Hoang
Javascript Es2015 Classes And Prototype Inheritance Part 1
How To Add A Class To An Element Using Javascript Javatpoint
Classes And Objects In Javascript Es6 Intermediate
0 Response to "23 What Is Class Javascript"
Post a Comment