21 How To Use Math Random In Javascript
28/8/2021 · let n1 = Math.floor(Math.random()*10+1) let n2 = Math.floor(Math.random() * 10 + 1) document.getElementById('v1').innerHTML = n1; document.getElementById('v2').innerHTML = n2; var count = 0; var wrong = 0; var score = 0; document.getElementById('v1').value = n1; document.getElementById('v2').value = n2; document.getElementById('restart').style.visibility = "hidden"; … Return a random number between 1 and 100: Math.floor( (Math.random() * 100) + 1); Try it Yourself ». Previous JavaScript Math Object Next .
Recept Doplacilo Adijo Math Random Javascript Between Two
A number generated by a process, whose outcome is unpredictable is called Random Number. In JavaScript, this can be achieved by using Math.random () function. This article describes how to generate a random number using JavaScript.
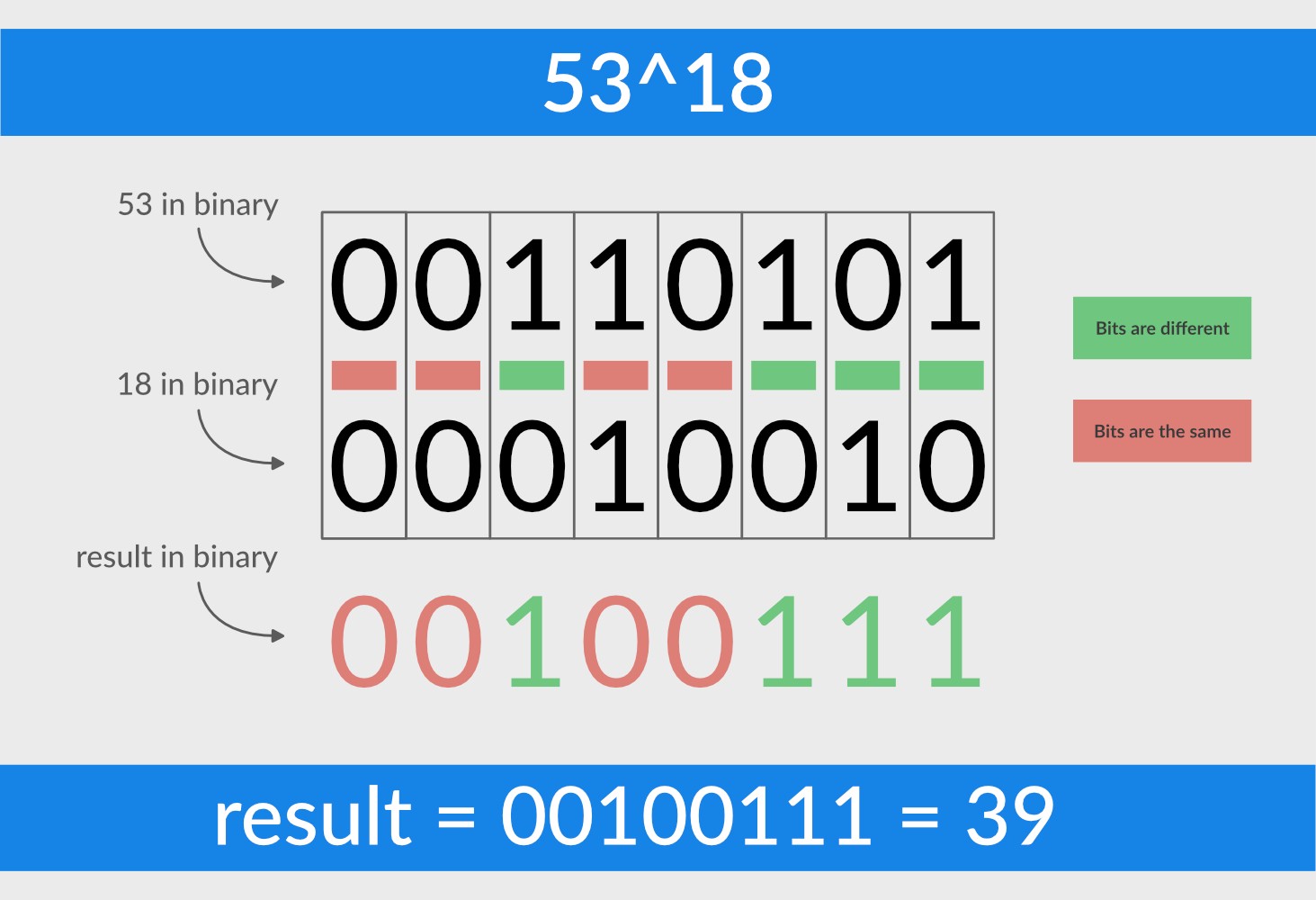
How to use math random in javascript. Program 1: Math.random () can be used to get a random number between two values. The returned value is no lower than min and may possibly be equal to min, and it is also less than and not equal to max. For getting a random number between two values the math.random () function can be executed in the following way: <script type="text/javascript">. The Math object in JavaScript is a built-in object that has properties and methods for performing mathematical calculations. A common use of the Math object is to create a random number using the random () method. const randomValue = Math.random (); But the Math.random () method doesn't actually return a whole number. To create a random number in Javascript, the math.random () function is used. JavaScript math.random () generates a random decimal value between 0 and 1. The function does not take any arguments. To get random numbers higher than 1 simply multiply the result according to the need.
In JavaScript, random () is a function that is used to return a pseudo-random number or random number within a range. Because the random () function is a static function of the Math object, it must be invoked through the placeholder object called Math. There are many ways available to generate a random string in JavaScript. The quickest way is to use the Math.random () method. The Math.random () method returns a random number between 0 (inclusive), and 1 (exclusive). You can convert this random number to a string and then remove the trailing zeros: Math.random () used with Math.floor () can be used to return random integers. There is no such thing as JavaScript integers. We are talking about numbers with no decimals here.
Algorithmic random number generation can't exactly be random, per se; which is why they're more aptly called pseudo-random number generators (PRNGs). If you're using math and formulae to create a sequence of numbers, random though they might seem, those numbers will eventually repeat and reveal a non-random pattern. JavaScript Random: Main Tips. When returning a random number from 0 (inclusive) to 1 (exclusive), we use Math.random JavaScript method. It is a very good idea to create a proper function for Math.random JavaScript method to be used for all random integer purposes. Ability to generate random numbers is especially handy when creating games. The JavaScript random number method returns a random number from 0 (inclusive) up to but not including 1 (exclusive). In order to get numbers from other ranges (e.g 0-100), you can multiply the result by a specific number. At the run time, the seed is selected automatically. The user can not choose or reset the seed.
Math.random().toString(36).substr(2, 5), because.substring(7)causes it to be longer than 5 characters. JavaScript makes it really easy to generate and use pseudo-random numbers. By using the built-in Math.random() function, you are able to achieve just that.Ma... Random number in JavaScript - writing the code Using Math.random() function. We will use the Math.random() function, which will give us a random number in JavaScript. This function will give us a number from 0 to 1, for example 0.345, 0.87695, 0.1, 0, 0.11111,....
This is a tutorial for programming BEGINNERS.This tutorial will show you how to use JavaScript: Math.random(). We will use Math.random to display images at ... The JavaScript Math.random () method is an excellent built-in method for producing random numbers. When Math.random () is executed, it returns a random number that can be anywhere between 0 and 1. The 0 is included and 1 is excluded. Generating a random floating point number between 0 and 1 In order to generate completely random colors, we'll pass random numbers within a fixed range to the three HSL values. We can do this using Math.random and Math.floor. Math.random generates random numbers between 0 and 1. We can multiply these numbers by our specified range and use Math.floor to round up to the nearest whole number.
Lots of Ways to Use Math.random () in JavaScript. Math.random () is an API in JavaScript. It is a function that gives you a random number. The number returned will be between 0 (inclusive, as in, it's possible for an actual 0 to be returned) and 1 (exclusive, as in, it's not possible for an actual 1 to be returned). Answer: It's easy and useful to generate random decimals or whole numbers. See below steps to do get the whole number:-Use Math.random() to generate a random decimal. Multiply that random decimal by 100 or any number. Use another function, Math.floor() to round the number down to its nearest whole number. To generate a random whole number, you can use the following Math methods along with Math.random (): Math.ceil () — Rounds a number upwards to the nearest integer Math.floor () — Rounds a number downwards to the nearest integer Math.round () — Rounds a number to the nearest integer
1. Get a random number between two numbers including min and max numbers. By the use of the below method, you will get the random number between two numbers including min and max values. function getRandomNumber(min, max) { return Math.floor(Math.random() * (max - min + 1) + min); } getRandomNumber(0, 50); Copy. Also, your randomness doesn't become more random by adding arithmetic with static values into the value like you did. You should replace it with 4. You also should use something like Math.ceil () (since you're ignoring the 0 value, which is also probably not a good idea), not toFixed () which returns a string. 30/11/2020 · Math.random() is an API in JavaScript. It is a function that gives you a random number. The number returned will be between 0 (inclusive, as in, it’s possible for an actual 0 to be returned) and 1 (exclusive, as in, it’s not possible for an actual 1 to be returned). Math.random(); // returns a random number lower than 1
Math object provides various properties and methods. Random is one of the methods of math objects in javascript. We can use the random function to get a random number between 0 (inclusive) and 1 (exclusive). The result we get is a floating-point number which can include 0, but not 1. The Math.random() function returns a floating-point, pseudo-random number in the range 0 to less than 1 (inclusive of 0, but not 1) with approximately uniform distribution over that range — which you can then scale to your desired range. Javascript Math.random () is an inbuilt method that returns a random number between 0 (inclusive) and 1 (exclusive). The implementation selects the initial seed to a random number generation algorithm; it cannot be chosen or reset by the user.
Pick a Random Value From an Array Using the Math.random () Function in JavaScript We can pick a value from a given array by using its index in JavaScript. To pick a random value from a given array, we need to generate a random index in the range of 0 to the length of the array. Lack of compartmentalization. Before ES6, the Math.random() definition didn't specify that distinct pages had to produce distinct sequences of values.. This allowed an attacker to generate some random numbers, determine the state of the PNRG, redirect the user to a vulnerable application (which would use Math.random() for sensitive things) and predict which number Math.random() was going to ... We can use Math.random() to generate a number between 0-1(inclusive of 0, but not 1) randomly. Then multiply the random number with the length of the array. floor to result to make it whole number .
The answer is what you originally assumed, and that is by using the Math.random () method. This will create a random number, from 0 to the length of the array. In this case, since the array has 4 elements inside of it, the last element is at an index of 3. So randomFoodIndex will create a random number from 0 to 3.
How To Generate 3 Random Whole Numbers Within A Given Range
Lots Of Ways To Use Math Random In Javascript Css Tricks
The Problem Of A Parseint Function Found By Javascript Random
Random Number In Javascript Practical Example
How Does Javascript S Math Random Generate Random Numbers
Javascript Math Random Summarized By Plex Page Content
Generate A Random Number Between Two Numbers In Javascript
Adding Math Floor Math Random 6000 To Wait Field Using
How Can I Generate Random Variable For Testing Issue 769
Javascript Random Number Between 1 And 10 Design Corral
Javascript Math Random Normal Distribution Gaussian Bell
The Objects In Javascript Chance Or Math Random Introduction To Javascript Programming Htmlboy
Javascript Fundamental Es6 Syntax Generate A Random
Js Random Number Between 1 And 100 Code Example
Javascript Wichmann Hill Random Number Generator James D
Math Random Does Not Generate Random Numbers Issue 1310
This Make Function Math Random In My Whole React App Return
Why Does This Random Distribution Look Asymmetric Stack
0 Response to "21 How To Use Math Random In Javascript"
Post a Comment