30 Javascript Element In Array
JavaScript: Printing array elements. Learn how to print array elements to the console or the web page with JavaScript. Posted on March 15, 2021. When you need to print array elements, you can loop over the array and print each element to the console as follows: javascript: count number of array element occurrences in another array find frequency of elements in an array in n time and 1 space in js stackoverflow occurrence findd in number array
How To Check If Array Includes A Value In Javascript
JS Examples JS HTML DOM JS HTML Input JS HTML Objects JS HTML Events JS Browser JS Editor JS Exercises JS Quiz JS Certificate ... Array iteration methods operate on every array item. ... The forEach() method calls a function (a callback function) once for each array element.
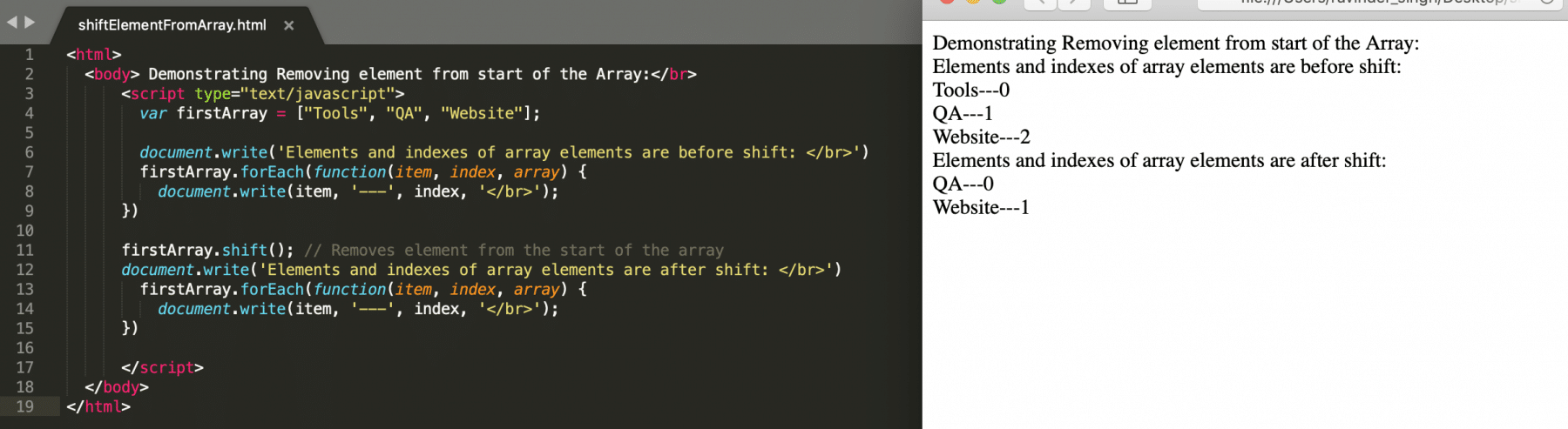
Javascript element in array. javascript has two inbuilt array methods name unshift() and push(), which is used to add elements or items at first or beginning and last or ending of an array in javascript. This tutorial has the purpose to explain how to add an element at the beginning and ending of an array in javascript using these unshift and push js methods. @tokland Maybe the filter will not create an intermediate array. A good optimizing compiler can easily recognize that only the length of the array is used. Maybe none of the current JS compilers are smart enough to do this, but that is not important. Jul 04, 2020 - We actually looping through new Array object in this case! So that's one of the reasons why we need to use for..in carefully, but it's not always the case... ... Since JavaScript elements are saved as standard object properties, it is not advisable to iterate through JavaScript arrays using ...
Removes the first element from an array and returns that element. Array.prototype.slice() Extracts a section of the calling array and returns a new array. Array.prototype.some() Returns true if at least one element in this array satisfies the provided testing function. Array.prototype.sort() Sorts the elements of an array in place and returns ... 13 hours ago - If you don't declare a variable for any of these parameters they just won't be allocated. For example, most uses of the forEach method just use the element, and ignore the index and array. The element is the most important value when iterating, so you should always declare an element parameter. 2 weeks ago - The find method executes the callback function once for each element present in the array until it finds one where callback returns a true value. If such an element is found, find immediately returns the value of that element. Otherwise, find returns undefined. callback is invoked only for ...
JavaScript array can store multiple element of different data types. It is not required to store value of same data type in an array. Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays. But, JavaScript arrays are best described as arrays. Arrays use numbers to access its "elements". In this example, person[0] returns John: 9/7/2021 · There are a couple of different ways to add elements to an array in Javascript: ARRAY.push ("ELEMENT") will append to the end of the array. ARRAY.unshift ("ELEMENT") will append to the start of the array. ARRAY [ARRAY.length] = "ELEMENT" acts just like push, and will append to the end. ARRAYA.concat (ARRAYB) will join two arrays together.
Aug 21, 2020 - How to determine if a JavaScript array contains a particular value, being a primitive or object. At the implementation level, JavaScript's arrays actually store their elements as standard object properties, using the array index as the property name. The length property is special. It always returns the index of the last element plus one. (In the example below, 'Dusty' is indexed at 30, so cats.length returns 30 + 1). The indexOf () method searches an array for a specified item and returns its position. The search will start at the specified position (at 0 if no start position is specified), and end the search at the end of the array. indexOf () returns -1 if the item is not found.
In JavaScript, you'll often need to iterate through an array collection and execute a callback method for each iteration. And there's a helpful method JS devs typically use to do this: the forEach() method.. The forEach() method calls a specified callback function once for every element it iterates over inside an array. Just like other array iterators such as map and filter, the callback ... Apr 28, 2021 - It contains 4 “elements” inside the array, all strings. And as you can see each element is separated by a comma. This example array holds different makes of cars, and can be referred to with the cars variable. There are a number of ways we can iterate over this array. JavaScript is incredibly ... May 13, 2021 - It is not convenient to use an object here, because it provides no methods to manage the order of elements. We can’t insert a new property “between” the existing ones. Objects are just not meant for such use. There exists a special data structure named Array, to store ordered collections.
Jul 17, 2021 - The arr.sort(fn) method implements a generic sorting algorithm. We don’t need to care how it internally works (an optimized quicksort or Timsort most of the time). It will walk the array, compare its elements using the provided function and reorder them, all we need is to provide the fn which ... push () ¶. The push () method is an in-built JavaScript method that is used to add a number, string, object, array, or any value to the Array. You can use the push () function that adds new items to the end of an array and returns the new length. The new item (s) will be added only at the end of the Array. You can also add multiple elements to ... Firstly, to loop through an array by using the forEach method, you need a callback function (or anonymous function): numbers.forEach (function () { // code }); The function will be executed for every single element of the array. It must take at least one parameter which represents the elements of an array:
A Boolean which is true if the value searchElement is found within the array (or the part of the array indicated by the index fromIndex, if specified). Values of zero are all considered to be equal, regardless of sign. (That is, -0 is considered to be equal to both 0 and +0), but false is not considered to be the same as 0. Here are the different JavaScript functions you can use to add elements to an array: # 1 push - Add an element to the end of the array. #2 unshift - Insert an element at the beginning of the array. #3 spread operator - Adding elements to an array using the new ES6 spread operator. #4 concat - This can be used to append an array to ... JavaScript provides us an alternate array method called lastIndexOf (). As the name suggests, it returns the position of the last occurrence of the items in an array. The lastIndexOf () starts searching the array from the end and stops at the beginning of the array. You can also specify a second parameter to exclude items at the end.
Sep 11, 2020 - Note: If you are seeking the index ... it returns the matching element’s index instead of the element itself. find() is helpful for use cases where you want a single search result value. ... The filter() method returns a new array of all the values in an array that matches the ... The includes() method returns true if an array contains a specified element, otherwise false. ... The numbers in the table specify the first browser version that fully supports the method. An array in JavaScript is a type of global object that is used to store data. ... Here we will assign two variables, one that uses slice() to store the seaCreatures array from the first element until whale, and a second variable to store the elements pufferfish and lobster. To join the two arrays, ...
How to Find the Min/Max Elements in an Array in JavaScript There are multiple methods to find the smallest and largest numbers in a JavaScript array, and the performance of these methods varies based on the number of elements in the array. Let's discuss each of them separately and give the testing results in the end. In the same directory where the JavaScript file is present create the file named code.json. After creating the file to make the work easier add the following code to it: { "notes": [] } In the above code, we create a key called notes which is an array. The Main JavaScript Code. In the upcoming code, we will keep adding elements to the array. The find () method returns the value of the array element that passes a test (provided by a function). The method executes the function once for each element present in the array: If it finds an array element where the function returns a true value, find () returns the value of that array element (and does not check the remaining values)
The JavaScript method toString () converts an array to a string of (comma separated) array values. Array.indexOf and Array.findIndex are similar because they both return the index of the first matching element found in our Array, returning us -1 if it's not found. To check if an element exists, we simply need to check if the returned value is -1 or not.
Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays. But, JavaScript arrays are best described as arrays. Arrays use numbers to access its "elements". In this example, person[0] returns John: The includes () method returns true if an array contains a specified element, otherwise false. includes () is case sensitive. Adding Elements Anywhere in an Array. Now we will discuss a masterstroke method that can be used to add an element anywhere in an array - start, end, middle, and anywhere else in-between. The splice() method adds, removes and replaces elements in an array. It is commonly used for array management.
Sep 11, 2020 - In JavaScript, an array is an ordered list of values. Each value is called an element specified by an index. An JavaScript array has the following characteristics: Adding an element at a given position of the array. Sometimes you need to add an element to a given position in an array. JavaScript doesn't support it out of the box. So we need to create a function to be able to do that. We can add it to the Array prototype so that we can use it directly on the object. Depending on the version of JavaScript you have available, you can use indexOf: Returns the first index at which a given element can be found in the array, or -1 if it is not present.
1 week ago - The for...in loop statement will return all enumerable properties, including those with non–integer names and those that are inherited. Because the order of iteration is implementation-dependent, iterating over an array may not visit elements in a consistent order. If you need the index of the found element in the array, use findIndex (). If you need to find the index of a value, use Array.prototype.indexOf (). (It's similar to findIndex (), but checks each element for equality with the value instead of using a testing function.) Below examples illustrate the JavaScript Array find () method in JavaScript: Example 1: Here the arr.find () method in JavaScript returns the value of the first element in the array that satisfies the provided testing method. <script>. var array = [10, 20, 30, 40, 50]; var found = array.find (function (element) {.
Instead of a delete method, the JavaScript array has a variety of ways you can clean array values. You can remove elements from the end of an array using pop, from the beginning using shift, or from the middle using splice. The JavaScript Array filter method to create a new array with desired items, a more advanced way to remove unwanted elements. 7/7/2020 · Array.splice will modify your original array and return the removed elements so you can do the following: const arr = [1,2,3,4,5]; const index = arr.indexOf(2); const splicedArr = arr.splice(index,1); arr; // [1,3,4,5]; splicedArr; // [2] Enter fullscreen mode. Exit fullscreen mode. 23/9/2020 · The find () method executes this function for each element of array. If the function returns true for any element then that element will be returned by the find () method and it stops checking further for rest of the elements. If no element passed the condition then find () method will return undefined.
Let's assume that a random array has given: let myArray = [[1, 2, 3, 5], [3, 4, 5], [2, 5]]; I want to access each element in array like this: [myArray[0][0], myArray ... In modern browsers which follow the ECMAScript 2016 (ES7) standard, you can use the function Array.prototype.includes, which makes it way more easier to check if an item is present in an array: const array = [1, 2, 3]; const value = 1; const isInArray = array.includes (value); console.log (isInArray); // true. Share. 1 week ago - Neither the length of a JavaScript array nor the types of its elements are fixed. Since an array's length can change at any time, and data can be stored at non-contiguous locations in the array, JavaScript arrays are not guaranteed to be dense; this depends on how the programmer chooses to use them.
Access Random Array Element In Javascript Without Repeating
How To Get Last Array Element In Javascript Example
Delete The Array Elements In Javascript Delete Vs Splice
How To Find Elements In Array In Javascript Js Curious
Dynamic Array In Javascript Using An Array Literal And
How To Check If An Element Is Present In An Array In Javascript
How To Remove Array Duplicates In Es6 By Samantha Ming
Remove Element From Array Javascript First Last Value
How To Remove And Add Elements To A Javascript Array
5 Way To Append Item To Array In Javascript Samanthaming Com
How To Move An Array Element From One Array Position To
Javascript Quiz Array Element Duplicate Count With For Loop
How To Append Element To Array In Javascript Tecadmin
7 Ways To Remove Duplicates From An Array In Javascript By
Javascript Indexof Method Explained With 5 Examples To
6 Ways To Insert Elements To An Array In Javascript
Remove Elements From A Javascript Array Geeksforgeeks
Javascript Array Distinct Ever Wanted To Get Distinct
Remove A Specific Element From Array
How To Remove Array Element From Array In Javascript
The Beginner S Guide To Javascript Array With Examples
Tools Qa Array In Javascript And Common Operations On
Javascript Array Declaration Or Creations Amp Adding Elements
Remove Element From Array In Javascript By Sunil Sandhu
5 Easy Ways To Remove An Element From Javascript Array
How To Remove An Element From An Array In Javascript By
Delete The Array Elements In Javascript Delete Vs Splice
Removing Elements From An Array In Javascript Edureka
0 Response to "30 Javascript Element In Array"
Post a Comment