35 For In Range Javascript
A Range object is created without parameters: let range = new Range(); Then we can set the selection boundaries using range.setStart (node, offset) and range.setEnd (node, offset). As you might guess, further we'll use the Range objects for selection, but first let's create few such objects. JavaScript exercises, practice and solution: Write a JavaScript program to check whether two numbers are in range 40..60 or in the range 70..100 inclusive.
Range Slider Uisng Javascript And Css For Changing The
A range represents data in an array or object with a beginning and end value. A lot of languages have built in methods to create ranges, for example to_a in Ruby: ('a'.. 'e').to_a => [ "a", "b", "c", "d", "e"] JavaScript doesn't have a specific built-in method for this, but there are many ways we can build ranges.
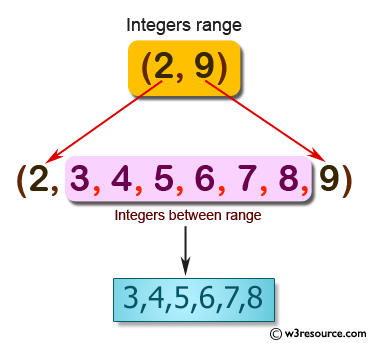
For in range javascript. Gets the first hyperlink in the range, or sets a hyperlink on the range. All hyperlinks in the range are deleted when you set a new hyperlink on the range. Use a '#' to separate the address part from the optional location part. [ API set: WordApi 1.3 ] You can specify a range of characters by using a hyphen, but if the hyphen appears as the first or last character enclosed in the square brackets it is taken as a literal hyphen to be included in the character class as a normal character. For example, [abcd] is the same as [a-d] . They match the "b" in "brisket", and the "c" in "chop". More range retrieval information can be found in object-specific articles. For examples that show how to get a range within a worksheet using the Range object, see Get a range using the Excel JavaScript API. For examples that show how to get ranges from a Table object, see Work with tables using the Excel JavaScript API.
Get used range. The following code sample gets the used range from the worksheet named Sample, loads its address property, and writes a message to the console. The used range is the smallest range that encompasses any cells in the worksheet that have a value or formatting assigned to them. If the entire worksheet is blank, the getUsedRange ... The RangeError object indicates an error when a value is not in the set or range of allowed values.
Range represents a set of one or more contiguous cells such as a cell, a row, a column, block of cells, etc. To learn more about how ranges are used throughout the API, start with Ranges in the Excel JavaScript API. [ API set: ExcelApi 1.1 ] 6/1/2018 · range is a function that basically takes in a starting index and ending index then return a list of all integers from start to end. The most obvious way would be using a for loop. function range ( start , … Step 3) Add JavaScript: Create a dynamic range slider to display the current value, with JavaScript: Example. var slider = document.getElementById("myRange"); var output = document.getElementById("demo"); output.innerHTML = slider.value; // Display the default slider value.
Range The Range interface represents a fragment of a document that can contain nodes and parts of text nodes. A range can be created by using the Document.createRange () method. Range objects can also be retrieved by using the getRangeAt () method of the Selection object or the caretRangeFromPoint () method of the Document object. Square brackets may also contain character ranges. For instance, [a-z] is a character in range from a to z, and [0-5] is a digit from 0 to 5. In the example below we're searching for "x" followed by two digits or letters from A to F: alert("Exception 0xAF".match(/x [0-9A-F] [0-9A-F]/g)); JavaScript const paragraphs = document . querySelectorAll ( 'p' ) ; // Create new range const range = new Range ( ) ; // Start range at second paragraph range . setStartBefore ( paragraphs [ 1 ] ) ; // End range at third paragraph range . setEndAfter ( paragraphs [ 2 ] ) ; // Get window selection const selection = window . getSelection ( ) ; // Add range to window selection selection . addRange ( range ) ;
JavaScript Range is a function that is supported by JavaScript in order to return all the integer and its value from starting to the ending while traversing from start index to the end index. The concept of the range is basically derived from the concept of selection which includes a pair of boundary points with the start range and end range. The default stepping value for range inputs is 1, allowing only integers to be entered, unless the stepping base is not an integer; for example, if you set min to -10 and value to 1.5, then a step of 1 will allow only values such as 1.5, 2.5, 3.5,... in the positive direction and -0.5, -1.5, -2.5,... in the negative direction. 8/10/2010 · OK, in JavaScript we don't have a range() function like PHP, so we need to create the function which is quite easy thing, I write couple of one-line functions for you and separate them for Numbers and Alphabets as below: for Numbers: function numberRange (start, end) { return new Array(end - start).fill().map((d, i) => i + start); } and call it like:
JavaScript does not have a two/three dot notation for creating ranges, however we could use the Ruby examples and create our own. In Ruby, the.to_a and the Array methods are built-in methods for... 22/6/2011 · 59. Here is an option with only a single comparison. // return true if in range, otherwise falsefunction inRange(x, min, max) { return ((x-min)*(x-max) <= 0);}console.log(inRange(5, 1, 10)); // trueconsole.log(inRange(-5, 1, 10)); // falseconsole.log(inRange(20, 1, 10)); // false. Share. Range is something I discovered recently, which again showed me that the possibilities with the Javascript and the DOM are truly endless. As stated on Mozilla's developer site, range ...
The For Loop. The for loop has the following syntax: for ( statement 1; statement 2; statement 3) {. // code block to be executed. } Statement 1 is executed (one time) before the execution of the code block. Statement 2 defines the condition for executing the code block. Statement 3 is executed (every time) after the code block has been executed. In the above markup, I am using oninput attribute to update the labels with the Range values. Note: The JavaScript function ti() is at the end of this blog post. Syntax of HTML5 "oninput" Event Attribute. oninput='someFunction()' The oninput event attribute calls a JavaScript function when you input a value, in our case it's the Range slider ... Underscore.js | _.range () It is used to print the list of elements from the start given as a parameter to the end also a parameter. The start and step parameters are optional. The default value of start is 0 and that of step is 1. In the list formed the start is inclusive and the stop is exclusive. The step parameter can be either positive or ...
Creating a Range Slider. We can create a Range Slider using simple HTML and JavaScript by following the below steps: Step 1:Creating an HTML element. The slider element is defined in this step using the "div" element under which is a input field whose range is defined between 1 and 100. < Is there a function in JavaScript similar to Python's range()? As answered before: no, there's not. But you can make your own. I believe this is an interesting approach for ES6. It works very similar to Python 2.7 range(), but it's much more dynamic. Input Range Object. The Input Range object represents an HTML <input> element with type="range". Note: <input> elements with type="range" are not supported in Internet Explorer 9 and earlier versions. Access an Input Range Object. You can access an <input> element with type="range" by using getElementById():
Definition and Usage. The value property sets or returns the value of the value attribute of a slider control. The value attribute specifies the default value OR the value a user types in (or a value set by a script). In JavaScript, this can be achieved by using Math.random() function. This article describes how to generate a random number using JavaScript. Method 1: Using Math.random() function: The Math.random() function is used to return a floating-point pseudo-random number between range [0,1) , 0 (inclusive) and 1 (exclusive). This random number can ... A Proper Random Function. As you can see from the examples above, it might be a good idea to create a proper random function to use for all random integer purposes. This JavaScript function always returns a random number between min (included) and max (excluded):
Array Number Ranges in JavaScript ES6 Tue, Dec 9, 2014. Warning: Since this post was published, the ECMAScript Technical Committee has removed the comprehensions feature from ES6. This post shows usage of other (supported) features of the language, and has been left unedited. I've spent the last few days really digging into ECMAScript 6. The ...
How To Style Range Input With Css And Javascript For Better
10 Best Range Slider Plugins In Javascript 2021 Update
Javascript Program To Find All Years In A Range With First
Clean Mobile Friendly Range Slider In Javascript
Javascript Basic Check Whether Three Given Integer Values
Coding Grounds Enumerable Range In Javascript
Material Design Style Custom Range Slider Plugin With Jquery
Rangeslider Js Html5 Input Range Slider Element Polyfill
How To Get Values From Html5 Input Type Range Using Javascript
Date Range Picker Javascript Date Range Picker Component
Generating Random Whole Numbers In Javascript In A Specific
Simple Smooth Range Slider In Javascript Sliders Js Css
Value Bubbles For Range Inputs Css Tricks
Creating A Range In Javascript Have You Ever Worked On A
Date Range Date And Time Picker Javascript Component Web
Basic Javascript Use Recursion To Create A Range Of Numbers
Build Jquery Ui Slider Range Slider In Javascript
Javascript Price Range Slider Example Markuptag
Javascript Fundamental Es6 Syntax Initialize An Array
Javascript Scheduler Limit Time Range Selection Min Max
Themeable Range Slider Control In Javascript Jquery Wrunner
Mj Date Range Picker For Vue Js 2 Vue Script
Javascript Range Slider Designs Themes Templates And
Onchange Event Of Daterangepicker Js Predefined Date Range
Value Bubbles For Range Inputs Css Tricks
Github Dangrossman Daterangepicker Javascript Date Range
Javascript Recursion Function Get The Integers In A Range
Javascript Group On An Array Of Objects With Timestamp Range
Genomic Range Query Codility 100 Correct Javascript
Calculate The Numbers Divisible By A Given Number In A Given
What I Learned From Reading Random Number In Range Or How I
Function Range As Range Js Code Example
Add Range Numbers In Array Javascript Code Example
0 Response to "35 For In Range Javascript"
Post a Comment