28 Javascript Count Occurrences Of Character
JavaScript Function to Count String Occurrences. I've been working on a super-awesome-secret node.js project… and I had a need for a high-performance way to read how many lines a file has. Well, I've generalized my code a bit to count the number of occurrences of a substring in a larger string. JavaScript fundamental (ES6 Syntax): Exercise-70 with Solution. Write a JavaScript program to count the occurrences of a value in an array. Use Array.prototype.reduce () to increment a counter each time the specific value is encountered inside the array. This Pen is owned by w3resource on CodePen .
Java Program To Count Duplicate Characters In String Java 8
// program to check the number of occurrence of a character function countString(str, letter) { let count = 0; // looping through the items for (let i = 0; i < str.length; i++) { // check if the character is at that position if (str.charAt(i) == letter) { count += 1; } } return count; } // take input from the user const string = prompt('Enter a string: '); const letterToCheck = prompt('Enter a letter to check: '); //passing parameters and calling the function const …
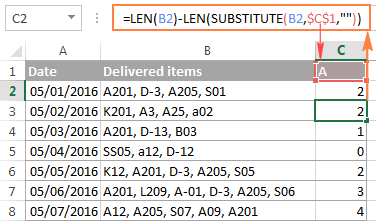
Javascript count occurrences of character. There are many ways to count the number of occurrences of a char in a String in Java. In this quick tutorial, we'll focus on a few examples of how to count characters — first with the core Java library and then with other libraries and frameworks such as Spring and Guava. 1. Find the occurrences of character 'a' in the given string. 2. Find the No. of repetitions which are required to find the 'a' occurrences. 3. Multiply the single string occurrences to the No. of repetitions. 4. If given n is not the multiple of given string size then we will find the 'a' occurrences in the remaining substring. Take an array to store the frequency of each character. If similar character is found, then increment by one otherwise put 1 into that array. Let's say the following is our string −. var sentence = "My name is John Smith"; Following is the JavaScript code to count occurrences −.
Count occurrences of a character in a repeated string in C++; Count the number of occurrences of a string in a VARCHAR field in MySQL? How to Count Occurrences of Each Character in String in Android? Count number of occurrences for each char in a string with JavaScript? C# program to count the occurrences of each character; Java program to ... javascript count number of occurrences in array and store in object; check one occurence array; takes in array and returns count occurrences javascript; using reduce to count multiple string in an array; count number of times each character appears in array javascript; js array find all matches count; how to count instance of an element in aray The stringr package provides a str_count() method which is used to count the number of occurrences of a certain pattern specified as an argument to the function. The pattern may be a single character or a group of characters. Any instances matching to the expression result in the increment of the count.
Given a string and a character, task is to make a function which count occurrence of the given character in the string. Examples: Input : str = "geeksforgeeks" c = 'e' Output : 4 'e' appears four times in str. Input : str = "abccdefgaa" c = 'a' Output : 3 'a' appears three times in str. Recommended: Please try your approach on {IDE} first ... Approach 2: In this approach, we use nested for loop to iterate over string and count for each character in the string. First initialize count with value 0 for ith value of string. Now we iterate over string if ith value matches with the character, increase the count value by 1. Finally, print the value of count. Count characters in JavaScript We can start by transforming the word into an array of characters. We can do that using the split with the remark that it works only with characters taking no more...
I need to count the number of occurrences of a character in a string. For example, suppose my string contains: var mainStr = "str1,str2,str3,str4"; I want to find the count of comma , character, which is 3. And the count of individual strings after the split along comma, which is 4. Java 8 Streams also provide a simple way to count the occurrences of a character in a String. We will first convert the String to an IntStream by using the chars () method. We can also use the codePoints () method instead of chars (). Next, we will use the filter () method with a Lambda expression to filter out all the matching characters. Count of occurrences of a character in a repeated string are: 7 Explanation The number of 's' in str is 2. Length of str is 4. For n=15, str will be fully repeated 3 times (first 12 characters), so count of s in those will be 3*2=6. For the remaining 3 characters (set) s occurs once. So count is 6+1=7
For those that arrived here looking for a generic way to count the number of occurrences of a regex pattern in a string, and don't want it to fail if there are zero occurrences, this code is what you need. ... Detect number of Arabic characters in a String in JavaScript. 0. totalVowels variable is not updating-3. count the number of a specific ... JavaScript exercises, practice and solution: Write a JavaScript function that accepts two arguments, a string and a letter and the function will count the number of occurrences of the specified letter within the string. This post will discuss how to count the total number of occurrences of a character in a string with JavaScript. 1. Using Regex. Regular expressions are often used in JavaScript for matching a text with a pattern. The following code example demonstrates its usage to get the count of the characters in the string.
Count occurrences of each character in a string using Javascript It's common in programming that every developer needs to count each occurrence of a character in a string or sentence in some situation. In this article, we will study all the possible best ways to understand how to count occurrences of each character in a string using Javascript. JavaScript Program to Count the Total Occurrences of a Character in a String. ... You can find the count of total occurrences of a character in a string by other methods like recursion, regular expressions, library functions, etc. The iterative method used in this article is one of the simplest methods to solve this problem. In the above code, the split () method splits the string into an array of strings by separating string at each hello character, so that by subtracting the length with -1 we will the get exact count value.
Javascript: search string in array then count occurrences-2. ... How to replace all occurrences of a string in JavaScript. 7418. How to check whether a string contains a substring in JavaScript? 2026. ... How do you cast Mending on a clockwork character? 7/6/2020 · Q: How to count occurrences of each character in string javascript? For example given string is:-var mainStr = "str1,str2,str3,str4"; Find the count of comma , character, which is 3. And the count of individual strings after the split along with a comma, which is 4. Answer: Use a regular expression Here is a way to count occurrences inside an array of objects. It also places the first array's contents inside a new array to sort the values so that the order in the original array is not disrupted. Then a recursive function is used to go through each element and count the quantity property of each object inside the array.
29/9/2019 · In this tutorial, we will learn how to count the occurrence of a character in a string in Javascript. We will show you how to do this using two different ways. You can save the program in a javascript .js file and verify it by running the file using node. Or, you can create one simple HTML file … get string after character javascript; js fill string with n characters; operator sum string to character javascript; js character certain count; javascript regex match character from a set of characters; how to remove lasr char from string in javascript; javascript count occurrences in string The "g" says to find ALL occurences of the character "a". Otherwise it would only find the first one, and it would never count past the number one. The second flag is "i". It makes the RegEx match all cases of that character. If that flag (i) was not there, the code above would only count 4, because it would skip the uppercase "A" in the string.
In JavaScript, there are several ways to count the occurrences of some text or character in a string. If you need to find the occurrences of a single character, then using the charAt () method inside a loop is the easiest method. Let's look at some examples. March 15, 2014 Javascript Count Character Occurrences Online. This online utility displays the occurrence count of each character and character code contained in the text that you specify. Paste your text in the text box and then press the "Update Counts" button. The text will be searched using Javascript, and every character found in the text will be ...
How To Count String Occurrence In String Javascript
Javascript Count The Occurrence Of A Substring In A String
Count Occurrences Of Each Character In A String Using
Java Count Frequency Of Characters In A String Code Example
Javascript Character Count String Length Eyehunts
Write A Javascript Program To Read A Character String From
Finding All Indexes Of A Specified Character Within A String
How To Find Occurrences Of A Character In A String Using Ruby
How To Write A C Program For Counting Occurences Of
How To Count Characters In Excel Total Or Specific Chars In
How To Read File In Java And Count Total Number Of Characters
Javascript Character Count String Length Eyehunts
Python Program To Find First Occurrence Of A Character In A
Python Count Number Of Occurrences Characters In A String
Java Program To Find The Occurrence Of A Character In A String
Help With Custom Field Js To Count Occurrences Of
C Program To Count Occurrence Of A Word In A Text File
Count Occurrences Of Character In String Java 8 Code Example
Count Character Occurrence Of A String Using Dictionary
How To Count Characters In Excel Total Or Specific Chars In
How To Count Characters Words And Lines In A Text File In
Java Program To Find Maximum Occurring Character In A String
Word Frequency In Javascript Stack Overflow
How To Count The Occurrence Of A Character In A Cell In Excel
How To Count Each Character Occurrence In A Word With
C Java Php Programming Source Code Javascript Count
C Program To Count Occurrence Of Characters In A String C
0 Response to "28 Javascript Count Occurrences Of Character"
Post a Comment