26 Create Style Element Javascript
In Web Browser JavaScript it is possible to create tag with built-in DOM API. Quick solution: Screenshot: JavaScript style tag example. There are differ... To style multiple attributes of our button using a style class, we would write clickMeButton.className = 'myStyleClassName' instead. document.body.appendChild(clickMeButton) appends to the body of the document as a child. Let's say we want to modify the label on an HTML button element with the id myButton.
Javascript Codes That Can Add Text And Draw Lines On Images
Compare CSS's simple float: left to Javascript's style.styleFloat = 'left' and style.cssFloat = 'left'. Appending a stylesheet is better for performance when styling 15 or more elements. CSS allows you to leverage pseudo-classes and define styles with the simple a:hover selector instead of both onmouseover and onmouseout event listeners.
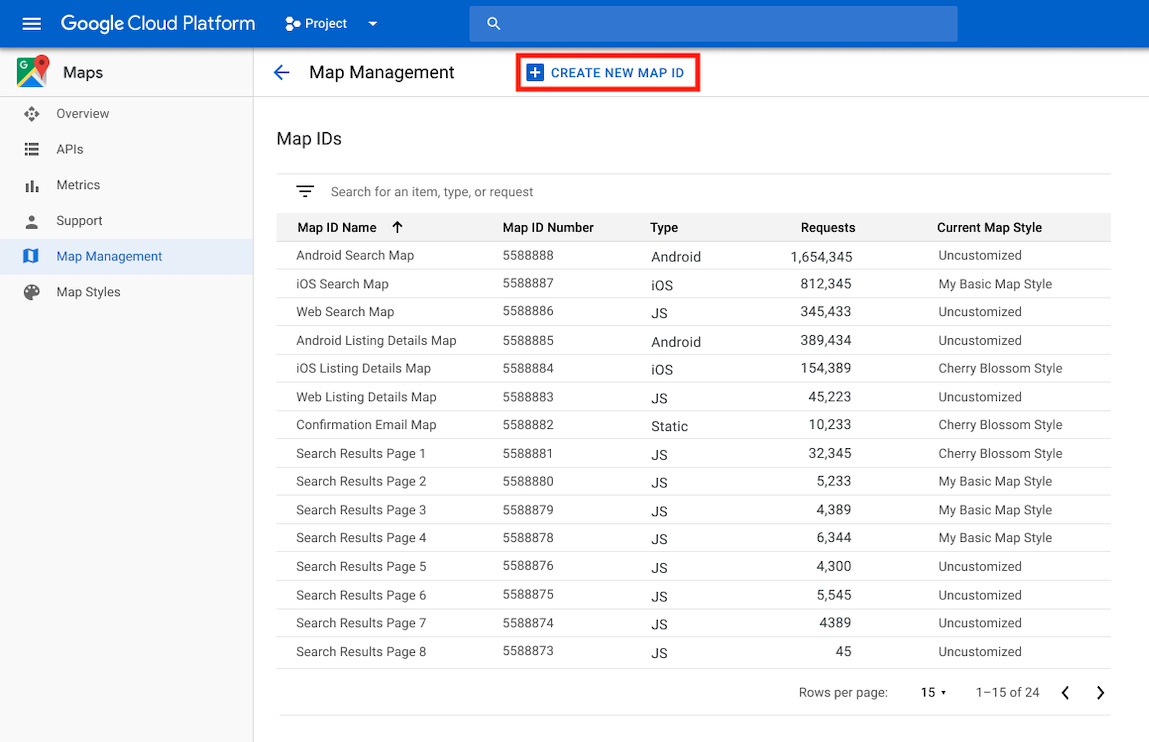
Create style element javascript. 2 weeks ago - It contains CSS, which is applied to the contents of the document containing the <style> element. The <style> element must be included inside the <head> of the document. In general, it is better to put your styles in external stylesheets and apply them using <link> elements. JavaScript HTMLElement.style - To change style of HTML Element programmatically, assign custom style value HTMLElement.style. Example : document.getElementById('idv').style = 'color:#f00;'. Syntax and Try Online Examples are provided to get hands on. Create style element javascript. Inline Css Explained Learn To Add Inline Css Style Back To The Basics How To Generate A Table With Javascript Examine And Edit Css Firefox Developer Tools Mdn Javascript How To Create Dynamically Html Element Create Horizontal Line With Html Amp Css Style Hr Elements In
Nov 19, 2011 - In answer to your first question, ... = createElement(...);, then you do document.body.appendChild(myElement);. ... This won't work in some versions of Internet Explorer. The safer solution is to use yourElement.style.cssText. ... You need to call the appendChild function to append your new element to an existing ... 6. Add ID Attribute To The Image In JavaScript. Adding multiple styles to the image element individually would be tedious. Instead, let's create a new CSS rule inside the style tags or an ... In our previous example, the method called appendChild () was used to append the new element as the last child of the parent element. JavaScript create element action can also be performed with the insertBefore () method, which is used to insert a new element above some selected one. Take a look at the last line of code in the example below ...
The style property is an object with camelCased styles. Reading and writing to it has the same meaning as modifying individual properties in the "style" attribute. To see how to apply important and other rare stuff - there's a list of methods at MDN. The style.cssText property corresponds to the whole "style" attribute, the full string of ... 6. Add ID Attribute to the Image in JavaScript. Adding multiple styles to the image element individually would be tedious. Instead, let's create a new CSS rule inside the style tags or an external CSS file with an ID selector like below. 3) Creating a script element example. Sometimes, you may want to load a JavaScript file dynamically. To do this, you can use the document.createElement() to create the script element and add it to the document. The following example illustrates how to create a new script element and loads the /lib.js file to the document:
It just creates a hyperlink element with no href, class, title or content. Let's make it a bit more useful: newlink = document.createElement('a'); newlink.setAttribute('class', 'signature'); newlink.setAttribute('href', 'showSignature (xyz)'); The problem with this code is that the href becomes a link to a page called showSignature (xyz) and ... To add inline styles to an element, you follow these steps: First, select the element by using DOM methods such as document.querySelector(). The selected element has the styleproperty that allows you to set the various styles to the element. Then, set the values of the properties of the styleobject. Of course since IE hasn't always supported insertRule, the other method is creating a STYLE element with the proper media attribute, then adding styles to that new stylesheet. This may require juggling multiple STYLE elements, but that's easy enough. I would probably create an object with media queries as indexes, and create/retrieve them that way.
Aug 27, 2011 - How can I use JavaScript to create and style (and append to the page) a div, with content? I know it's possible, but how? To set the style of an element, append a "CSS" property to style and specify a value, like this: element .style.backgroundColor = "red"; // set the background color of an element to red Try it As you can see, the JavaScript syntax for setting CSS properties is slightly different than CSS (backgroundColor instead of background-color). The createElement () method creates an Element Node with the specified name. Tip: After the element is created, use the element.appendChild () or element.insertBefore () method to insert it to the document.
3/3/2020 · The simple way is to just create a new <style> element, add your CSS properties using innerHTML, and append it to the DOM: // create style element const style = document . createElement ( 'style' ) ; // add CSS styles style . innerHTML = ` .btn { color: white; background-color: black; width: 150px; height: 40px; } ` ; // append the style to the DOM in <head> section document . head . … The most straightforward path. Query the element from DOM and change it's inline styles. document.getElementById('target').style.color = 'tomato'; Enter fullscreen mode. Exit fullscreen mode. Short and simple. 2. Global styles. Another option is to create <style> tag, fill it with CSS rules and append the tag to the DOM. To affect many elements, you can do something as follows: In a nutshell, to style elements directly using JavaScript, the first step is to access the element. Our handy querySelector method from earlier is quite helpful here. The second step is just to find the CSS property you care about and give it a value.
To create SVG elements, we need to use the createElementNS () method. The syntax from the MDN docs reads: var element = document.createElementNS(namespaceURI, qualifiedName[, options]); Cool, but what does that mean? 7/2/2009 · This works by injecting a stylenode into the head of the document. This is a similar technique to what is commonly used to lazy-load JavaScript. It works consistently in most modern browsers. appendStyle = function (content) { style = document.createElement('STYLE'); style.type = 'text/css'; style.appendChild(document. JavaScript Create Element. Creating DOM elements using js, adding text to the new element and appending the child to the Html body is not a hard task, it can be done in just a couple of lines of code. Let's see in the next example: var div = document.createElement ('div'); //creating element div.textContent = "Hello, World"; //adding text on ...
Feb 20, 2021 - The style read-only property returns the inline style of an element in the form of a CSSStyleDeclaration object that contains a list of all styles properties for that element with values assigned for the attributes that are defined in the element's inline style attribute. disabled boolean. Applies a disabled state to the Element such that user input is not accepted. Default is false. Create a card Element. JavaScript JavaScript (ESNext) 1. var cardElement = elements.create ('card'); To make your website interactive you must learn to use createElement in JavaScript. This knowledge is a must when creating even the simplest websites.
To define a new HTML element we need the power of JavaScript! The customElements global is used for defining a custom element and teaching the browser about a new tag. Call customElements.define() with the tag name you want to create and a JavaScript class that extends the base HTMLElement. Example - defining a mobile drawer panel, <app-drawer>: Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. You can also apply style on HTML elements to change the visual presentation of HTML documents dynamically using JavaScript. You can set almost all the styles for the elements like, fonts, colors, margins, borders, background images, text alignment, width and height, position, and so on.
21/1/2019 · First, one of the cleanest ways is to add or remove classes from an element, and use classes styling in your CSS. const element = document.querySelector('#my-element') You can use the classList property of an element and its add () and remove () methods: Aug 23, 2017 - Yesterday, we looked at how to get an element’s CSS attributes with vanilla JavaScript. One thing I neglected to mention: getComputedStyle() can only be used to get values, not set them. Today, let’s look at how to set CSS with vanilla JS. Approach 1: Inline Styles The easiest way to set ... The easiest and straightforward way to change CSS styles of an element with JavaScript is by using the DOM style property. All you need to do is just fetch the element from DOM and change its inline styles:
Another way to alter the style of an element is by changing its class attribute. class is a reserved word in JavaScript, so in order to access the element's class, you use element.className. You can append strings to className if you want to add a class to an element, or you could just overwrite className and assign it a whole new class. Therefore, to add specific styles to an element without altering other style values, it is generally preferable to set individual properties on the CSSStyleDeclarationobject. For example, element.style.backgroundColor = "red". A style declaration is reset by setting it to nullor an empty string, e.g., elt.style.color = null. Create an empty image instance using new Image().; Then set its attributes like (src, height, width, alt, title etc). Finally, insert it to the document. Example 2: This example implements the above approach.
tagName A string that specifies the type of element to be created. The nodeName of the created element is initialized with the value of tagName.Don't use qualified names (like "html:a") with this method. When called on an HTML document, createElement() converts tagName to lower case before creating the element. In Firefox, Opera, and Chrome, createElement(null) works like createElement("null"). 27/2/2019 · The document.createElement(‘style’) method is used to create the style element using JavaScript. The steps to create style element are listed below: Steps: Create a style element using the following syntax Syntax: document.createElement('style'); Apply the CSS styles. Append this style element to the head element. Syntax: Creating and get reference to the new stylesheet with Javascript : var element = document.createElement('style'), sheet; // Append style element to head document.head.appendChild(element); // Reference to the stylesheet sheet = element.sheet; Creating and get reference to the new stylesheet with jQuery :
Reduce reflows, organize styles. There's a few ways of styling elements in JavaScript.
Custom Elements Defining New Elements In Html Html5 Rocks
Setting Css Styles Using Javascript Kirupa
Back To The Basics How To Generate A Table With Javascript
How To Modify Attributes Classes And Styles In The Dom
Examine And Edit Css Firefox Developer Tools Mdn
Is There Any Action To Change Element Dimensions Question
How To Allow Style Tag Element Within Your Wordpress Blog For
How To Create Interactive Websites With Javascript By
Change Css Style Of A Class With Javascript But Not In The
Override Important Style Property In Javascript
Javascript Programming Using The Document Object Model
Javascript Set Css Set Css Styles With Javascript Dev
Styling Your Map Maps Javascript Api Google Developers
Adding Css Amp Js And Overriding The Page Load Template Dash
How To Dynamically Create Javascript Elements With Event
How To Make A Css Stylesheet In Notepad Turbofuture
How To Create Html Lists Without Bullets
Javascript Style Attribute How To Implement Css Styles In
How To Create Custom Html Elements Html Javascript
5 Ways To Style React Components In 2020 By Jonathan Saring
How To Build A Random Quote Generator With Javascript And
Setting Css Styles With Javascript Soshace Soshace
How To Style React Components Digitalocean
Javascript Teacher Home Facebook
0 Response to "26 Create Style Element Javascript"
Post a Comment