27 Javascript Add Class To Dom Element
DOM (Document Object Model) is a way of manipulating the document (HTML document). This article will deal with how to access and set class names to the DOM elements. In DOM, all HTML elements are defined as objects. We will be using Javascript against CSS to manipulate them. Definition and Usage. The appendChild() method appends a node as the last child of a node. Tip: If you want to create a new paragraph, with text, remember to create the text as a Text node which you append to the paragraph, then append the paragraph to the document. You can also use this method to move an element from one element to another (See "More Examples").
Toggle Class Javascript Based Without Jquery Qa With Experts
How to add multiple classes to element in JavaScript, Element.classList modern JavaScript answer on Code to go
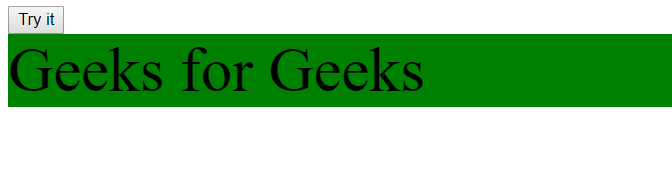
Javascript add class to dom element. Oct 18, 2018 - Implementation detail: classList is not an array, but rather it is a collection of type DOMTokenList. You can’t directly edit classList because it’s a read-only property. You can however use its methods to change the element classes. Download my free JavaScript Beginner's Handbook and check ... You can use any given method to add a class to your element, another way to check for, change or remove them. The className way - Simple way to add a single or multiple classes and remove or change all classes. The classList way - The way to manipulate classes; add, change or remove a single or multiple classes at the same time. Dec 28, 2015 - Checking for or removing a single class when multiple classes are used uses the same approach as the className way. But the classList way is easier to accomplish this and can be used, even if you set it the DOM way. ... If doing a lot of element creations, you can create your own basic ...
Aug 26, 2009 - Before jQuery version 1.12/2.2, the .addClass() method manipulated the className property of the selected elements, not the class attribute. Once the property was changed, it was the browser that updated the attribute accordingly. An implication of this behavior was that this method only worked for documents with HTML DOM ... JavaScript DOM — Add a CSS class to an element August 13, 2020 Atta To add a CSS class to an HTML element, you can use the classList property of the element. JS Classes Class Intro Class Inheritance Class Static ... JavaScript HTML DOM Elements (Nodes) Previous Next Adding and Removing Nodes (HTML Elements) Creating New HTML Elements (Nodes) To add a new element to the HTML DOM, you must create the element (element node) first, and then append it to an existing element. Example
18/4/2020 · Add a Class to an Element. To add a class to an element, you use the classListproperty of the element. Suppose you have an element as follows: <div>Item</div>. Code language:HTML, XML(xml) To add the box class to the <div>element, you use the following: constdiv = document.querySelector('div');div.classList.add('box'); 1 week ago - The className property of the Element interface gets and sets the value of the class attribute of the specified element. To add a new element to the HTML DOM, we have to create it first and then we need to append it to the existing element.. Steps to follow. 1) First, create a div section and add some text to it using <p> tags.. 2) Create an element <p> using document.createElement("p").. 3) Create a text, using document.createTextNode(), so as to insert it in the above-created element("p").
Check if an element has given class. Drag and drop element in a list. Drag and drop table column. Drag and drop table row. Highlight an element when dragging a file over it. Resize columns of a table. Show a custom context menu at clicked position. Next. Append to an element. To add an additional class to an element: To add a class to an element, without removing/affecting existing values, append a space and the new classname, like so: document.getElementById("MyElement").className += " MyClass"; To change all classes for an element: To replace all existing classes with one or more new classes, set the className ... Learn how to add a class name to an element with JavaScript. Add Class. Click the button to add a class to me! Add Class. Step 1) Add HTML: Add a class name to the div element with id="myDIV" (in this example we use a button to add the class). Example. <button onclick="myFunction()">Try it</button>. <div id="myDIV">.
The addEventListener () method attaches an event handler to an element without overwriting existing event handlers. You can add many event handlers to one element. You can add many event handlers of the same type to one element, i.e two "click" events. You can add event listeners to any DOM object not only HTML elements. i.e the window object. Feb 15, 2021 - From there you can easily derive ... to add a new class... just append a space followed by the new class to the element's className property. Knowing this, you can also write a function to remove a class later should the need arise. ... I think it's better to use pure JavaScript, which we can run on the DOM of the ... Element.classList. The Element.classList is a read-only property that returns a live DOMTokenList collection of the class attributes of the element. This can then be used to manipulate the class list. Using classList is a convenient alternative to accessing an element's list of classes as a space-delimited string via element.className.
In my previous articles, we looked at how to add markup as well as plain-text to HTML elements by using vanilla JavaScript. In this article, you'll learn to create and inject a new element to the DOM with JavaScript. To add an event handler to an HTML element, you can use the addEventListener () method of the element object. The addEventListener () method attaches an event handler to the specified HTML element without overwriting the existing event handlers. To attach a click event handler to the above button, you can use the following example: As you can ... Add class names to a DOM element To add a class name to a DOM element, you can use the add () method in the classList property in an element using JavaScript. Let's say you want to add a class name called red to an h1 element.
Mar 18, 2019 - If your new div will be added to ... can added to the DOM. See the docs for more info. – ziggy wiggy Mar 18 '19 at 15:26 ... This question still needs to be answered. It is not a duplicate of the proposed. This question is looking to add the classname in the same ... In this article, we are discussing how to add a class to an element using JavaScript. In JavaScript, there are some approaches to add a class to an element. We can use the .className property or the .add() method to add a class name to the particular element. Now, let's discuss the approaches to add a class to an element. Using .className property Oct 31, 2019 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
Yesterday, we looked at how to add markup to an element with vanilla JavaScript. As a follow-up, one of the students in my Vanilla JS Slack channel asked me how to inject a new element into the DOM with JavaScript. We can do this using the createElement() method, which let's us, as the name implies, create a new element. This is a fully manipulatable DOM node, and we can add classes and ... Adding a CSS class to an element using JavaScript. Now, let's add the CSS class "newClass" to the DIV element "intro". For the purpose of this example, I have added a delay using the setTimeout() method so that you can see the style changing: //Delay the JS execution by 5 seconds //by using setTimeout setTimeout(function(){ //Add the ... CSS class names can be removed or added using the classList method, or they can modified straight with the className property. Using the…
1 week ago - The getElementsByClassName method of Document interface returns an array-like object of all child elements which have all of the given class name(s). Summary: in this tutorial, you will learn how to use the JavaScript document.createElement() to create a new HTML element and attach it to the DOM tree. To create an HTML element, you use the document.createElement() method: Dec 01, 2019 - How to add class to element in JavaScript, Element.classList.add modern JavaScript answer on Code to go
Sep 15, 2020 - A DOMTokenList representing the contents of the element's class attribute. If the class attribute is not set or empty, it returns an empty DOMTokenList, i.e. a DOMTokenList with the length property equal to 0. The DOMTokenList itself is read-only, although you can modify it using the add() and ... Definition and Usage The classList property returns the class name (s) of an element, as a DOMTokenList object. This property is useful to add, remove and toggle CSS classes on an element. The classList property is read-only, however, you can modify it by using the add () and remove () methods. Note that if you eval the code instead of adding a script element, document.currentScript will not be set properly. If you are making a tool for other people to use, their script code might depend on document.currentScript - Bill Burdick Sep 9 '19 at 16:46
Adding CSS Classes to Elements You can also get or set CSS classes to the HTML elements using the className property. Since, class is a reserved word in JavaScript, so JavaScript uses the className property to refer the value of the HTML class attribute. var el = document.getElementById ('mydiv'); Next, get the className string containing all the class names of the el object variable and split the array into a string filled in with spaces between class names. var a = el.className.split (" "); Finally, check if the class name you want to add already exists in the list of classes on the element. 18/10/2018 · When you have a DOM element reference you can add a new class to it by using the add method: element.classList.add('myclass') You can remove a class using the remove method: element.classList.remove('myclass') Implementation detail: classList is not an array, but rather it is a collection of type DOMTokenList.
Introduction. In the previous tutorial in this series, "How To Make Changes to the DOM," we covered how to create, insert, replace, and remove elements from the Document Object Model (DOM) with built-in methods.By increasing your proficiency in manipulating the DOM, you are better able to utilize JavaScript's interactive capabilities and modify web elements. We can add or remove the class name of a specific HTML element with the help of the classList property. We can use add() and remove() method to add and remove the class name of the HTML element. add(): The add() method will add the new class name of specific HTML element. If the class name already exists then the browser will ignore this method. 2/12/2019 · Javascript Front End Technology Object Oriented Programming. To add a class to a DOM element, you first need to find it using a querySelector like querySelector, getElementById, etc. Then you need to add the class. For example, if you have the following HTML −.
How To Add Class To Select2 Element In V4 0 Newbedev
How To Add And Remove Classes In Vanilla Javascript
Get The Closest Element By Selector
How To Change Element S Class With Javascript Classname And
Adding Html To Ag Grid Column Headers And Cells
How To Add A Css Class To Divi On Scroll With Jquery
How To Modify Attributes Classes And Styles In The Dom
Cheat Sheet For Moving From Jquery To Vanilla Javascript
Jquery Add Remove And Toggle Class Dot Net Tutorials
Manipulate Dom Elements Without Writing Javascript Code
Get Started With Viewing And Changing Css Microsoft Edge
How To Addclass Removeclass Toggleclass In Javascript
Adding Or Removing Css Class In Lightning Web Component
View And Change Css Chrome Developers
How To Createelement In Javascript
Javascript Dom Tutorial 3 Get Elements By Class Or Tag
Addclass Method In Jquery Dotnet Helpers
Add Or Remove Multiple Classes In Javascript For Dom Element
Jquery Add Remove And Toggle Class Dot Net Tutorials
Setting Css Styles With Javascript Soshace Soshace
How To Add Class Just Once In Javascript Stack Overflow
Painting And Rendering Optimization Techniques In Browser By
Chapter 3 Operating On A Jquery Collection Jquery In
How To Add A Class To Dom Element In Javascript Geeksforgeeks
Dynamically Add And Remove Html Elements Using Jquery
How To Add A Class To Dom Element In Javascript Geeksforgeeks
0 Response to "27 Javascript Add Class To Dom Element"
Post a Comment