23 Javascript Async Await Class Method
Await function is used to wait for the promise. It could be used within the async block only. It makes the code wait until the promise returns a result. It only makes the async block wait. const getData = async () => {. var y = await "Hello World"; console.log (y); } console.log (1); ¿Tienes dudas con las Promises y el Async/Await de JavaScript? No te pierdas este video dónde te resuelvo las dudas.Aprende JavaScript desde cero con mi libr...
Why Isn T Async Await Working In A Foreach Cycle Itnext
JavaScript Async. An async function is a function that is declared with the async keyword and allows the await keyword inside it. The async and await keywords allow asynchronous, promise-based behavior to be written more easily and avoid configured promise chains. The async keyword may be used with any of the methods for creating a function.
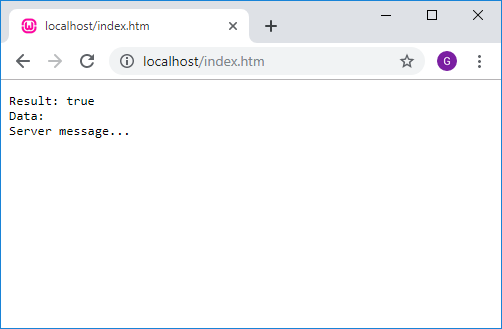
Javascript async await class method. Here are the steps you need to follow for using async/await in React: configure babel. put the async keyword in front of componentDidMount. use await in the function's body. make sure to catch eventual errors. If you use Fetch API in your code be aware that it has some caveats when it comes to handling errors. async is a keyword for the function declaration. await is used during the promise handling. await must be used within an async function, though Chrome now supports "top level" await. async functions return a promise, regardless of what the return value is within the function. async / await and promises are essentially the same under the hood. 7/10/2017 · What I would do is something like this: 1- remove the init call from the constructor. 2- use the class like this: const downloader = new ImageDownloader(); downloader.init() .then(() => downloader.download()); and if you are calling init in an async function you can do: await downloader.init(); const result = await downloader.download();
Using JavaScript's async/await feature solves these potential problems! It also allows for writing much clearer and more concise code, without the need to chain .then () calls, etc. Basically ... 4/3/2017 · class AFactory { static async create() { return new A(await Promise.resolve('fooval')); } } This only makes the params to the constructor to be async, not the constructor itself. An async constructor would pseudo-logic be what @dalu wrote: class A { constructor() { this.foo = await externallib.create(); } } Your point is that the content is not set synchronously when the object is created, which is not required by the question. That's why the question is about using await/async from within a constructor. I've demonstrated how you can invoke as much await/async as you want from a constructor by calling an async function from it.
Examples of async function declaration. The await operator is used to wait for a resolved Promise, that is returned by the async function.In general we can use await with any value. When we use Promise, theawait returns resolved value, if Promise is rejected the await throw exception with rejected value. In case if value is not Promise the await operator returns the same value. Now let's look at how Promises were improved upon by introducing the async/await syntax in Javascript. Javascript Async/Await . Apparently, there was even more room for improvement in Javascript's asynchronous support. With ES 8 (ES 2017), the async/await syntax was introduced to simplify things even more. 16/3/2021 · The keyword await makes JavaScript wait until that promise settles and returns its result. Here’s an example with a promise that resolves in 1 second: async function f() { let promise = new Promise((resolve, reject) => { setTimeout(() => resolve("done!"), 1000) }); let result = await promise; alert( result); } f();
What can ES6 Classes provide, as a pattern of organization, to asynchronous code. Below is an example with ES7 async/await, can an ES6-class have an asynchronous method, or constructor in ES7? Can I do: class Foo { async constructor() { let res = await getHTML(); this.res = res } } Your usage is totally wrong. The created member in your BaseClass class is a field, with type of => Promise<string>, how can a field to be async?. The async keyword must be used with a function, like async function doSomething() { /* ... */ } or async => { /* .... The await keyword can only be used within the body of an async function, like the examples above. If you have experience on ASP.NET MVC then probably you are familiar with async/await keywords in C#. The same thing is now in JavaScript. Before that, Callbacks and Promises are used for asynchronous code. Async/await is built on top of promises and non blocking. The power of Async/await provides asynchronous code look like synchronous code.
Construction is easy! Adopting the functional options pattern for class construction has other benefits, but in particular, it allows for the creation of a class object that may require asynchronous processes.The async function call can be added right into the class instantiation step, without needing a separate init() call or having to modify your established method of class construction. 17/8/2021 · Home › async await class method javascript. 39 Async Await Class Method Javascript Written By Leah J Stevenson. Tuesday, August 17, 2021 Add Comment Edit. Async await class method javascript. Javascript Async Await Serial Parallel And Complex Flow. async function. An async function is a function declared with the async keyword, and the await keyword is permitted within them. The async and await keywords enable asynchronous, promise-based behavior to be written in a cleaner style, avoiding the need to explicitly configure promise chains. Async functions may also be defined as expressions.
17/8/2019 · async keyword makes a method asynchronous, which in turn always returns a promise and allows await to be used. await keyword before a promise makes JavaScript wait until that is resolved/rejected. If the promise is rejected, an exception is generated, otherwise the result is returned. The Async statement is to create async method in JavaScript.The function async is always return a promise.That promise is rejected in the case of uncaught exceptions, otherwise resolved to the return value of the async function. Whats Await in JavaScript. The await is a statement and used to wait for a promise to resolve or reject. What async/await is. async/await is built on top of promises, and it allows us to write asynchronous code better. It is a new way of writing asynchronous code instead of using promises and callbacks. The power of async/await is that it organizes the code making it look cleaner and more "synchronous." There are two particular keywords: async ...
The new methods array. You will need to update the iterate (iteration) method inside of methodIterator class to account for these changes. Check whether or not the function is asynchronous first ... The await expression causes async function execution to pause until a Promise is settled (that is, fulfilled or rejected), and to resume execution of the async function after fulfillment. When resumed, the value of the await expression is that of the fulfilled Promise.. If the Promise is rejected, the await expression throws the rejected value.. If the value of the expression following the ... 1 Answer1. Active Oldest Votes. 20. You dont need function as pointed out by @dfsq in the comments. Then you have to use module.exports or export to exposed your class as a module. masterclass.js. module.exports = class MasterClass { async updateData (a, b) { let [ res1, res2 ] = await Promise.all (call1, call2); return [ res1, res2 ] } } Share.
Async/await is a surprisingly easy syntax to work with promises. It provides an easy interface to read and write promises in a way that makes them appear synchronous. An async/await will always return a Promise. Even if you omit the Promise keyword, the compiler will wrap the function in an immediately resolved Promise. The async keyword. 먼저 비 동기 함수를 async function 으로 만들기 위하여 function ()앞에 async keyword를 추가합니다. async function ()은 await 키워드가 비동기 코드를 호출할 수 있게 해주는 함수 입니다. 브라우저의 JavaScript 콘솔에서 아래와 같이 입력해보세요. : function hello ... Let's have a look how you can modify the above code using async and await in JavaScript. The await keyword works only inside an async function. To catch any errors thrown inside the await method you need to add a try and catch block. The above code looks synchronous and bit more cleaner and easier to read as compared to the earlier piece of code.
ECMAScript 2017 introduced the JavaScript keywords async and await. The following table defines the first browser version with full support for both: Chrome 55. Edge 15. Firefox 52. Safari 11. Opera 42. More recent additions to the JavaScript language are async functions and the await keyword, added in ECMAScript 2017. These features basically act as syntactic sugar on top of promises, making asynchronous code easier to write and to read afterwards. They make async code look more like old-school synchronous code, so they're well worth learning. This article gives you what you need to know. Async on class methods. Class methods can be async. class Example {async asyncMethod {const data = await axios. get ("/some_url_endpoint"); return data }} To call the method we'd do: const exampleClass = new Example (); exampleClass. asyncMethod (). then (//do whatever you want with the result) Await is thenable. We can append a .then() on an ...
Async Constructor Pattern in JavaScript. In this post I'll show three design patterns that deal with async initialization and demonstrate them on a real-world example. This is a little API pattern I came across while building a JS utility library that is using WebAssembly under the hood. Instantiating a WebAssembly instance is an async ... 19/11/2019 · You may have a situation where you have a class and you want to have async methods on it. You can do something like this: class WebPageDownloader { async getWebPage(url) { return await getWebPage(url); } } (async => { const downloader = new WebPageDownloader(); const html = await downloader.getWebPage('https://zaro.io'); console.log('Downloaded', html); })();
Calling Bind On An Async Function Partially Works Stack
What Lies Beneath Async Await In C
Be Careful Of Passing An Async Function As A Parameter In
How To Use Async Await In React Componentdidmount Async
When And How To Use Async Await
Javascript Async Class Method Code Example
Async Await In Swiftui Raywenderlich Com
Await And Async Explained With Diagrams And Examples
Async Constructor Pattern In Javascript Qwtel
Javascript Async Await The Good Part Pitfalls And How To
Getting Started With Async Await In Swiftui Swiftui
Inventory Of Async Await Knowledge In Javascript Minews
Build A Secure Node Js Application With Javascript Async
Understanding Javascript S Async Await
Javascript How To Return Ajax Response From
How To Use Fetch With Async Await
Async Await In Swiftui Raywenderlich Com
Javascript Async And Await A Complete Guide By Harsh
Async Await Beginner Javascript Wes Bos
How To Use Async Await To Properly Link Multiple Functions In
How To Await An Async Event Handler Delegate In Uwp Pspdfkit
0 Response to "23 Javascript Async Await Class Method"
Post a Comment