32 Max Value In Object Javascript
The MAX_VALUE property has a value of approximately 1.79E+308, or 2 1024. Values larger than MAX_VALUE are represented as Infinity. Because MAX_VALUE is a static property of Number, you always use it as Number.MAX_VALUE, rather than as a property of a Number object you created. But what if we have array of number and we would like to find min and max value in it. If we send an array to Math.min or Math.max methods we will get NaN const nums = [1, 2, 3] Math.min(nums ...
Javascript Find Max Value In An Array Find Max Value In
Given a hashmap (or a "Dictionary") as a JavaScript object, I'd like to get the Key who has the maximum Value - assuming all Values are integers. In case there's more than one, I don't care which one of them.
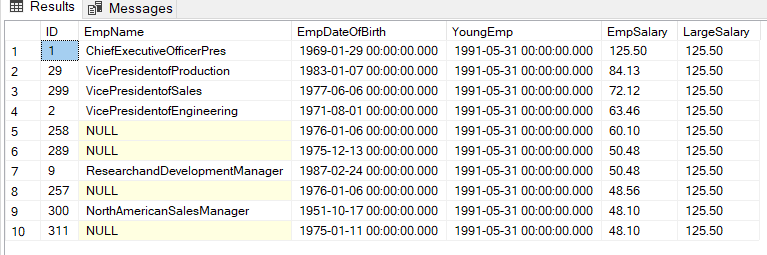
Max value in object javascript. Aug 26, 2020 - We have to write a function that takes in this array and returns the top n element of the array in another array (top means the object that has the highest value for duration). Nov 19, 2020 - The Fastest Way to Find Minimum and Maximum Values in an Array in JavaScript. JavaScript offers several ways to find the smallest and largest numbers in a list, including the built-in Math functions and sorting the…. Maximum value of an attribute in an array of objects can be searched in two ways, one by traversing the array and the other method is by using the Math.max.apply () method. Example 1: In this example, the array is traversed and the required values of the object are compared for each index of the array. var arr = [
Description The MAX_SAFE_INTEGER constant has a value of 9007199254740991 (9,007,199,254,740,991 or ~9 quadrillion). The reasoning behind that number is that JavaScript uses double-precision floating-point format numbers as specified in IEEE 754 and can only safely represent integers between - (2^53 - 1) and 2^53 - 1. Object.values() returns an array whose elements are the enumerable property values found on the object. The ordering of the properties is the same as that given by looping over the property values of the object manually. May 14, 2020 - let max = Math.max(...Object.values(candles)) let high = Object.entries(candles).filter(([i, data]) => max === data) return high ... Install and run react js project...
JavaScript provides 8 mathematical constants that can be accessed as Math properties: Example. Math.E // returns Euler's number. Math.PI // returns PI. Math.SQRT2 // returns the square root of 2. Math.SQRT1_2 // returns the square root of 1/2. Math.LN2 // returns the natural logarithm of 2. Math.LN10 // returns the natural logarithm of 10. There's no way to find the maximum / minimum in the general case without looping through all the n elements (if you go from, 1 to n-1, how do you know whether the element n isn't larger (or smaller) than the current max/min)?. You mentioned that the values change every couple of seconds. The following function uses Function.prototype.apply () to get the maximum of an array. getMaxOfArray ([1, 2, 3]) is equivalent to Math.max (1, 2, 3), but you can use getMaxOfArray () on programmatically constructed arrays. This should only be used for arrays with relatively few elements.
When a JavaScript variable is declared with the keyword " new ", the variable is created as an object: x = new String (); // Declares x as a String object. y = new Number (); // Declares y as a Number object. z = new Boolean (); // Declares z as a Boolean object. Avoid String, Number, and Boolean objects. They complicate your code and slow down ... In JavaScript, max () is a function that is used to return the largest value from the numbers provided as parameters. Because the max () function is a static function of the Math object, it must be invoked through the placeholder object called Math. It should be noted that the maximum Date is not of the same value as the maximum safe integer (Number.MAX_SAFE_INTEGER is 9,007,199,254,740,991).
The JavaScript for/of statement loops through the values of an iterable objects. for/of lets you loop over data structures that are iterable such as Arrays, Strings, Maps, NodeLists, and more. The for/of loop has the following syntax: for ( variable of iterable) {. // code block to be executed. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. How To Find The Maximum Of An Attribute Value In An Array Of JavaScript Objects Finding the maximum value in an array of numbers is easy. Just use Math . max ( ) and pass all the values in the array as arguments to the function call, and you are done.
So we have javascript built-in math max function which can easily find the maximum value from an array. So let's move to our code section. // javascript math max function let max_value = Math.max(1,2,3,4,5) console.log(max_value) // result => 5. As Math.max() the function does not work with an array of number but there is some way to do this. I have an array of objects. I want to find the maximum value of a given numeric value that exists in each of the objects. Suppose the array of objects is called "stat" and the numeric value I want to check is called "member". var stat = [{ member: -1 }, { member: 1 }, { member: 20 }, { member: 5 }]; var toString = Object.prototype.toString; Here is a suggestion in case you have many equal values and not only one maximum: const getMax = object => { return Object.keys(object).filter(x => { return object[x] == Math.max.apply(null, Object.values(object)); }); }; This returns an array, with the keys for all of them with the maximum value, in case there are some that have equal values ...
Feb 14, 2016 - Use the 100 answers in this short book to boost your confidence and skills to ace the interviews at your favorite companies like Twitter, Google and Netflix. ... MEET THE NEW JSTIPS BOOK The book to ace the JavaScript Interview. The exec () method is a RegExp expression method. It searches a string for a specified pattern, and returns the found text as an object. If no match is found, it returns an empty (null) object. The following example searches a string for the character "e": Example. Mar 14, 2019 - Spread syntax allows an iterable ... or more key-value pairs (for object literals) are expected. This to me is the cleanest looking solution and I happen to really like it. ... I didn't mean to turn this into an article about functional programming in JavaScript but this is where ...
The Number.MAX_VALUE property belongs to the static Number object. It represents constants for the largest possible positive numbers that JavaScript can work with. The actual value of this constant is 1.7976931348623157 x 10 308. Object Values in JavaScript. A value, on the other hand, can be any data type, including an array, number, or boolean. The values in the above example contain these types: string, integer, boolean, and an array. You can even use a function as a value, in which case it's known as a method. Feb 14, 2018 - Can replace the ternary with a Math.max(acc, shot.amount), along with many other multiple methods. The issue with the reduce function you produced on SO, your reducer accumulator/initial value (0) was placed in the wrong spot. ... OP wanted the object, not the value. With a slight adjustment:
JavaScript: Finding Minimum and Maximum values in an Array of , Given an array of objects and the task is to get the maximum and minimum value from the array of objects. There are a few methods used which Find the object whose property "Y" has the greatest value in an array of objects One way would be to use Array reduce.. const max = data ... May 14, 2020 - Get code examples like "javascript find max value in array of objects" instantly right from your google search results with the Grepper Chrome Extension. The Problem. We have an array of 100,000 objects: [{x: 1, y: 14830}, {x: 2, y: 85055}, {x: 3, y: 03485}, {x: 4, y: 57885}, // ...Y values are randomized. How do we quickly find the minimum and maximum Y values in our array?. The 'Why'. Recently, I published an article on: How I built an Interactive 30-Day Bitcoin Price Graph with React and an API.One of the key ingredients you need to ...
May 23, 2019 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. Description In JavaScript, MAX_VALUE is a static property of the Number object that is used to return the maximum numeric value that can be represented in JavaScript. Because MAX_VALUE is a property of the Number object, it must be invoked through the object called Number. We wish to write a function that takes in such object and a number n (n <= no. of keys in object) and returns an object with n highest key value pairs. Like for n = 2. Output should be −. { tenacity: 97, pace: 96 } Therefore, let's write the code for this function, The complete code for this function will be −.
Javascript find max value in object. JavaScript (/ ˈ dʒ ɑː v ə ˌ s k r ɪ p t /), often abbreviated as JS, is a programming language that conforms to the ECMAScript specification Like for n = 2 User can select an option from the suggestion list I've been using objects as hash tables and I've been getting the highest value from them by Objects.keys(foo).reduce((a,b) => foo[a] > foo[b] ? a : b). However, I've switched to using Maps() . What is the ideal way to iterate through a Map object to get the highest value? Object.values () Method. Object.values () method is used to return an array whose elements are the enumerable property values found on the object. The ordering of the properties is the same as that given by the object manually is a loop is applied to the properties. Object.values () takes the object as an argument of which the enumerable own ...
You can use the Math.max() and ... object, like this: ... <script> var numbers = [1, 5, 2, -7, 13, 4]; var maxValue = Math.max.apply(null, numbers); console.log(maxValue); // Prints: 13 var minValue = Math.min.apply(null, numbers); console.log(minValue); // Prints: -7 </script> See the tutorial on JavaScript borrowing ... May 14, 2020 - Get code examples like "find the highest value in an object array and return the name" instantly right from your google search results with the Grepper Chrome Extension. Use inbuilt method Math apply (), map (), reduce () to get the max value in Array of objects in JavaScript. The Array object lets you store multiple values in a single variable. Ways to get max value in Array of objects:- Using apply () and map () method
Mar 18, 2019 - Spread syntax allows an iterable ... or more key-value pairs (for object literals) are expected. This to me is the cleanest looking solution and I happen to really like it. ... I didn't mean to turn this into an article about functional programming in JavaScript but this is where ... Example 1: object get property with max value javascript var obj = {a: 1, b: 2, undefined: 1}; Object.keys(obj).reduce((a, b) => (obj[a] > obj[b]) ? a : b); Example
Bucket Sort Solution In Javascript
Javascript Finding Minimum And Maximum Values In An Array Of
Find The Min Max Element Of An Array Using Javascript
Finding The Max Value In An Array By Jeff Gosselin Medium
Maximum Range In Projectile Motion Wired
Js Show It Today Interactive Choropleth World Map Using
Intro To Maxmsp 13 Steps With Pictures Instructables
Sql Server Max Aggregate Function
Sql Partition By Clause Overview
Filter An Array For Unique Values In Javascript Dev Community
Javascript Finding Minimum And Maximum Values In An Array Of
How To Use Enums In Typescript Digitalocean
Sequelize Aggregate Functions Min Max Sum Count Etc
How To Find Maximum Value From Arraylist In Java
On A Graph What S The Difference Between Global And Local
Javascript Finding Minimum And Maximum Values In An Array Of
How To Hide Show The Service For Object Generic Object
Sql Server Max Aggregate Function
Find The Min Max Element Of An Array In Javascript Stack
Tutorial The Javascript Mtr Connection Part 2 Cycling 74
Mongodb Data Types 16 Various Data Types In Mongodb Dataflair
Cloudfront Update Configurable Max And Default Ttl Aws
Javascript Get Max Value In Array Of Objects Example Code
Java Program To Find The Largest Number In An Array Edureka
Filter An Array For Unique Values In Javascript Dev Community
Cloudfront Update Configurable Max And Default Ttl Aws
0 Response to "32 Max Value In Object Javascript"
Post a Comment