20 How To Use Fetch Javascript
Once you're done, be sure to check your work using the free JSON Lint service. Writing the JavaScript and Fetch API Call. We have our JSON file created. The next step is to write some JavaScript using fetch() to retrieve the contents of our JSON file. Remember earlier I mentioned that fetch accepts one mandatory argument and returns a response. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Tutorials References Exercises Videos NEW Menu . ... The Fetch API interface allows web browser to make HTTP requests to web servers.
Use External Fetch Calls In Office Scripts Office Scripts
XMLHttpRequest () is a JavaScript function that made it possible to fetch data from APIs that returned XML data. XMLHttpRequest gave us the option to fetch XML data from the backend without reloading the entire page. This function has grown from its initial days of being XML only. Now it supports other data formats like JSON and plaintext.
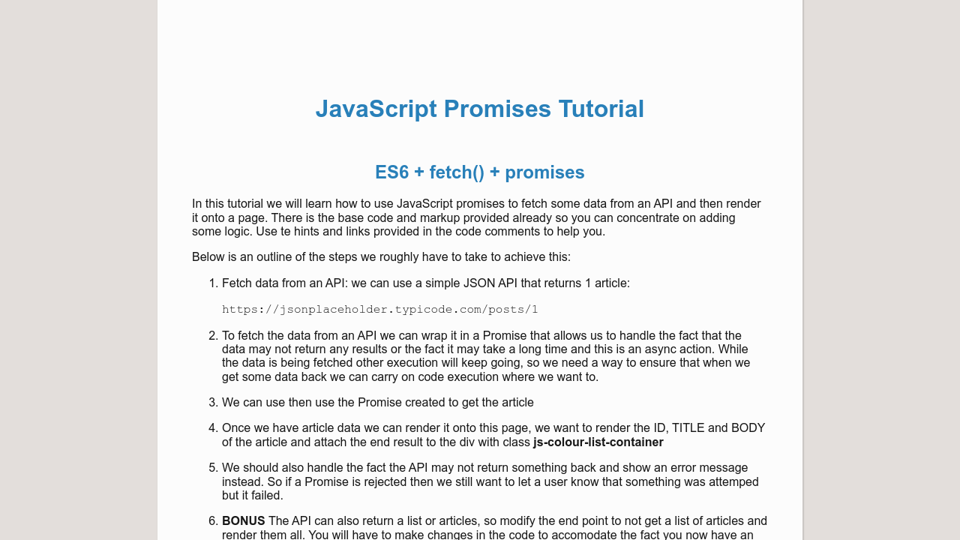
How to use fetch javascript. The Fetch API allows you to asynchronously request for a resource. Use the fetch () method to return a promise that resolves into a Response object. To get the actual data, you call one of the methods of the Response object e.g., text () or json (). These methods resolve into the actual data. How To Use Fetch Request in JavaScript? The Fetch API uses the promise we need to resolve the response object, and for that we use the.then method after the fetch function. It returns the promise that contains response. Using jQuery, you used the cleaner syntax with jQuery.ajax (). Now, JavaScript has its own built-in way to make API requests. This is the Fetch API, a new standard to make server requests with promises, but includes many other features. In this tutorial, you will create both GET and POST requests using the Fetch API.
This led to the creation of technologies that allow web pages to request small chunks of data (such as HTML, XML, JSON, or plain text) and display them only when needed, helping to solve the problem described above.. This is achieved by using APIs like XMLHttpRequest or — more recently — the Fetch API.These technologies allow web pages to directly handle making HTTP requests for specific ... Fetch returns a promise, and you can chain multiple promises, and use the result of the 1st request in the 2nd request, and so on. This example uses the SpaceX APIto get the info of the latest launch, find the rocket's id, and fetch the rocket's info. const url = 'https://api.spacexdata /v4'; The fetch () method is available in the global window scope, with the first parameter being the URL you want to call. By default, the Fetch API makes a GET request. A very simple HTTP request with fetch () would look below: fetch(url).then(res => { }).catch(err => { });
Fetch is a promise based HTTP request API. It's fairly well supported (87%+ https://caniuse /#feat=fetch) and is promise based for ease of use and is a pe... The fetch API is the easiest way to assess resources in the network. It's a tool that allows us to make HTTP requests using different methods such as GET, POST, etc. To start making requests, we use the method fetch () and pass it the required arguments. The fetch method accepts two arguments: I'm using fetch to get data json from an api. Works fine but I have to use it repeatedly for various calls, thus it needs to be synchronous or else I need some way to update the interface when the fetch completes for each component.
Yesterday, we looked at how to use the Fetch API with vanilla JS. The article focused on making API calls and working with JSON data. Today, I want to show you how to use fetch() to get HTML instead. The Fetch API returns a stream To recap, the response we get back from fetch() is a ReadableStream. With a typical API request, we use the json() method to get a JSON object from the stream that ... 5/12/2020 · To fetch a user we need: fetch('https://api.github /users/USERNAME'). If the response has status 200, call .json() to read the JS object. Otherwise, if a fetch fails, or the response has non-200 status, we just return null in the resulting array. So here’s the code: 28/5/2020 · fetch() method: The fetch() method is modern and versatile and is very well supported among the modern browsers. It can send network requests to the server and load new information whenever it’s needed, without reloading the browser. Syntax: The fetch() method only has one mandatory argument, which is the URL of the resource you wish to fetch.
The Syntax of Fetch API in JavaScript. We need to call the fetch() method in order to use the Fetch API in our JavaScript code. The fetch() method takes the URL of the API as an argument. We need to define the then() method after the fetch() method: The returning value of the fetch() method is a promise. The Fetch API uses streams. To get our API data as a JSON object, we can use a method native to the Fetch API: json (). We'll call it on our response object, and return its value. We can then work with the actual response JSON in a chained then () method. Now Lets Go Through the 4 Different FETCH Requests You Will Be Making. For the purpose of this blog and simplicity, I created a simple movie database in my db.json file in VSCode and ran it on localhost:3000 using the command json-server --watchdb.json.. This is what my database looks like:
Use the button in the demo to request a random dog image that gets displayed on the page. This uses an event listener to change the image each time the button is clicked.. The Fetch API in older browsers. Browser support shouldn't be a big problem in most cases, but if you still need to support Fetch in older browsers, there are some workarounds and polyfills. Submitting HTML Form Data to the Server Using the Fetch API To submit HTML form data to the server with Fetch API, you need to create and populate a FormData object and pass it to the fetch() request along with the POST method. Fetch fails, as expected. The core concept here is origin - a domain/port/protocol triplet. Cross-origin requests - those sent to another domain (even a subdomain) or protocol or port - require special headers from the remote side. That policy is called "CORS": Cross-Origin Resource Sharing.
In the previous tutorial, we had learned about the fetch method in Javascript and learned how we can use the fetch to make a GET and POST request to the server. Now in this tutorial, we will learn how we can make a PUT request to the server by using fetch in Javascript. The fetch API allows you to make Ajax requests in plain old JavaScript. It's a useful tool for retrieving data and making changes to data on a web server. In this guide, we're going to discuss what the fetch API is and how you can make web requests using the API. We'll talk through two examples: a GET request and a POST request. fetch('http://example /movies.json') .then(response => response.json()) .then(data => console.log( data)); Copy to Clipboard. Here we are fetching a JSON file across the network and printing it to the console. The simplest use of fetch () takes one argument — the path to the resource you want to fetch — and returns a promise containing the ...
Fetch is a Javascript function that accepts two arguments. The first argument is a stringified URL, and the second is an optional argument that will contain information about your request. If you are only looking to retrieve data from an API, you do not need to use the second argument, as Javascript will automatically expect a "GET" request. The fetch () method in JavaScript is used to request to the server and load the information in the webpages. The request can be of any APIs that returns the data of the format JSON or XML. This method returns a promise. To be able to display this data in our HTML file, we first need to fetch the data with JavaScript. We will fetch this data by using the fetch API. We use the fetch API in the following way: fetch (url).then (function (response) { // The JSON data will arrive here }).catch (function (err) { // If an error occured, you will catch it here });
12/5/2019 · Just pass the URL, the path to the resource you want to fetch, to fetch () method: fetch('/js/users.json') .then(response => { }) .catch(err => { }); We pass the path for the resource we want to retrieve as a parameter to fetch (). It returns a promise that passes the response to then () when it is fulfilled. Because fetch () returns a promise, you can simplify the code by using the async/await syntax: response = await fetch (). You've found out how to use fetch () accompanied with async/await to fetch JSON data, handle fetching errors, cancel a request, perform parallel requests.
How To Use Fetch In Javascript To Get Or Post Data
Fetch Api Introduction To Promised Based Data Fetching In
Javascript Fetch Api Tutorial With Js Fetch Post And Header
Consuming Rest Apis In React With Fetch And Axios Smashing
Javascript Fetch Api Cheatsheet Javascript
Javascript Fetch Api Tutorial With Js Fetch Post And Header
Javascript Fundamentals Fetching Data From A Server By
Javascript Fetch Api The Xmlhttprequest Evolution
Using Data In React With The Fetch Api And Axios Css Tricks
How To Use The Javascript Fetch Api To Get Data Marketing
Read Locally Json File Use Fetch Method In React Js By
How To Use Javascript S Fetch Api By Malcolm Staso Medium
A Better Way To Use Fetch Api In Javascript Get My Parking Blog
Fetch Api Introduction To Promised Based Data Fetching In
Web Apis Javascript Fetch Getting Json Data Fun With Apis
How To Use The Javascript Fetch Api To Get Data
0 Response to "20 How To Use Fetch Javascript"
Post a Comment