22 How To Check Object Is Null In Javascript
To check for null SPECIFICALLYyou would use this: if (variable === null) This test will ONLYpass for nulland will not pass for "", undefined, false, 0, or NaN. Additionally, I've provided absolute checks for each "false-like" value (one that would return true for !variable). Jul 15, 2017 - Lastly, when you’re ready to ... Check out the Best Courses for Learning Full Stack Web Development ... Null means an empty or non-existent value. Null is assigned, and explicitly means nothing. ... null is also an object. Interestingly, this was actually an error in the original JavaScript ...
Oct 23, 2019 - javascript check if undefined or null or empty string
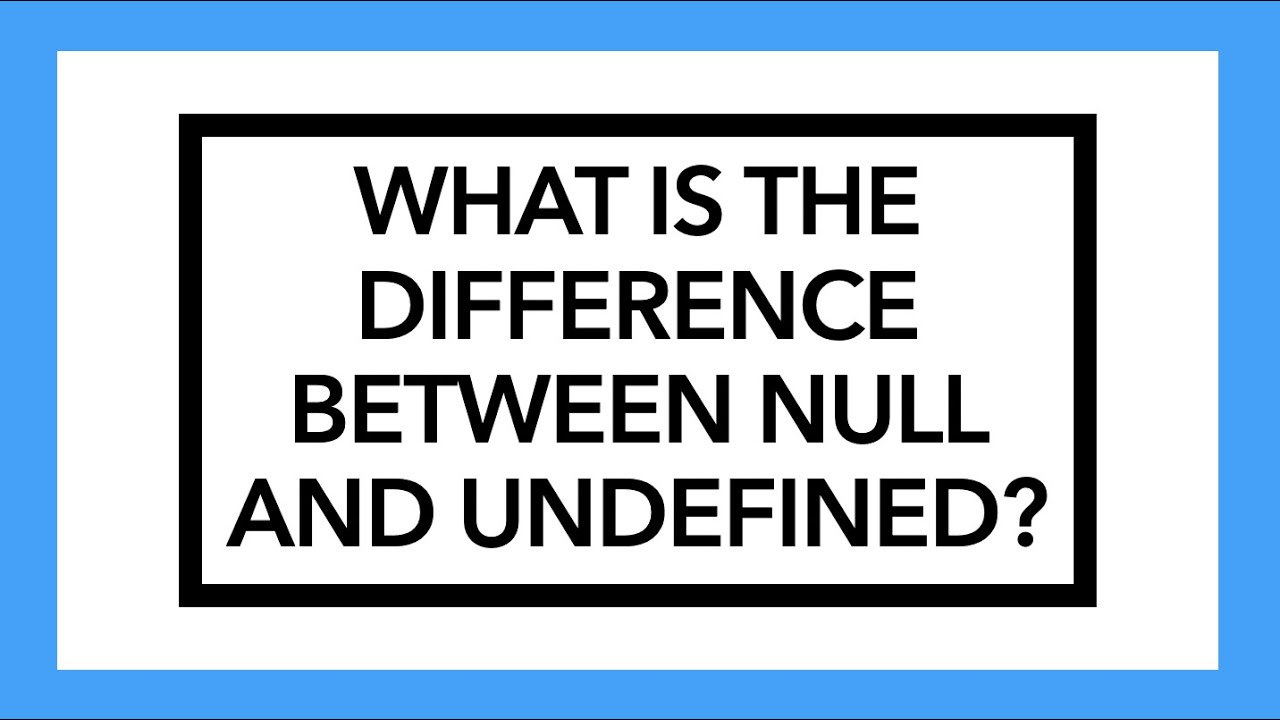
How to check object is null in javascript. follow. grepper; search snippets; faq The value null represents the object value or variable value is don't have any value ("No value"). There is no isNull function in JavaScript script to find without value objects. However you can build your own isNull () function with some logics. Examples of Check null in JavaScript How to Detect null in JavaScript We have seen, detecting null using the typeof operator is confusing. The preferred way to check if something is null is by using the strict equality operator (===). function isNull (input) { return input === null; }
The value null is written with a literal: null.null is not an identifier for a property of the global object, like undefined can be. Instead, null expresses a lack of identification, indicating that a variable points to no object.In APIs, null is often retrieved in a place where an object can be expected but no object is relevant. Nov 02, 2011 - Personal blog of photographer and web developer David Calhoun. So you don't have to use comparison operators like === or !== to check for null values. ... Undefined is also a primitive value in JavaScript. A variable or an object has an undefined value when no value is assigned before using it. So you can say that undefined means lack of value or unknown value.
If you try to test the null values using the typeof operator it will not work as expected, because JavaScript return "object" for typeof null instead of "null". This is a long-standing bug in JavaScript, but since lots of codes on the web written around this behavior, and thus fixing it would ... In javascript, we can check if an object is empty or not by using. JSON.stringify. Object.keys (ECMA 5+) Object.entries (ECMA 7+) And if you are using any third party libraries like jquery, lodash, Underscore etc you can use their existing methods for checking javascript empty object. One aspect of JavaScript development that many developers struggle with is dealing with optional values. What are the best strategies to minimize errors caused by values that could be null…
JavaScript provides the typeof operator to check the value data type. The operator returns a string of the value data type. For example, for an object, it will return "object". However, for arrays and null, "object" is returned, and for NaN/Infinity, "number" is returned. null is a primitive value that can be assigned to an object. When you assign null to an object and no other variable is referencing to that object anymore, it will be deleted from the memory permanently. In JavaScript, null is buggy and returns an object type when checked. In JavaScript, one of the everyday tasks while validating data is to ensure that a variable, meant to be string, obtains a valid value. This snippet will guide you in finding the ways of checking whether the string is empty, undefined, or null. Here we go. If you want to check whether the string is empty/null/undefined, use the following code:
Object.keys () Method The Object.keys () method is probably the best way to check if an object is empty because it is supported by almost all browsers including IE9+. It returns an array of a given object's own property names. So we can simply check the length of the array afterward: AFAIK in JAVASCRIPT when a variable is declared but has not assigned value, its type is undefined. so we can check variable even if it would be an object holding some instance in place of value. create a helper method for checking nullity that returns true and use it in your API. Nov 19, 2020 - Because of a historical bug, typeof null in JavaScript returns “object” — so how do you check for null?
4 weeks ago - A protip by kyleross about objects, prototype, javascript, and isempty. if (object != null && typeof(object) == "object") { doSomething(); } The main problem is that if you just check typeof(object) == "object", it will return true if object is null since null's type is "object". However, if you first check that object != null, you can be sure you are having something that is neither undefined nor null. In JavaScript, each variable can hold both object values and primitive values. Therefore, if null means “not an object”, JavaScript also needs an initialization value that means “neither an object nor a primitive value”. That initialization value is undefined.
How to check if an object is empty in JavaScript Find out how to see if a variable is equivalent to an empty object. Published Sep 10, 2019. Say you want to check if a value you have is equal to the empty object, which can be created using the object literal syntax: const emptyObject = {} 30/4/2019 · Method 1: Using the Object.keys(object) method: The required object could be passed to the Object.keys(object) method which will return the keys in the object. The length property is used to the result to check the number of keys. If the length property returns 0 keys, it means that the object is empty. Syntax: Object.keys(object).length === 0. Example: Feb 09, 2017 - You can check for null values in JavaScript. Using null parameters in JavaScript allows programmers to define a variable without assigning a value to it. Null values are used in simple variables like integers and strings, and they are used for objects on a Web page.
null is a special value in JavaScript that represents a missing object. The strict equality operator determines whether a variable is null: variable === null. typoef operator is useful to determine the type of a variable (number, string, boolean). However, typeof is misleading in case of null: typeof null evaluates to 'object'. 18/2/2021 · — Josh Clanton at A Drip of JavaScript O ne way to check for null in JavaScript is to check if a value is loosely equal to null using the double equality == operator: As shown above, null is only loosely equal to itself and undefined, not to the other falsy values shown. It is very easy to check if JavaScript array or object is empty but might need additional checks if you want to also check for null or undefined. Contents hide 1 1.
16/2/2018 · To check for null values in JavaScript, you need to check the variable value with null. Use the JavaScript strict equality operator to achieve this. Example. You can try to run the following code to check whether the value of the variable is null or not − Sep 29, 2020 - The rect === null evaluates to true because the rect variable is assigned to a null value. On the other hand, the square === null evaluates to false because it’s assigned to an object. To check if a value is not null, you use the strict inequality operator (!==): ... JavaScript null has the ... To check if an object is empty, we can use the keys() method available in the global Object object and then use the length property in the array returned from the method in JavaScript. TL;DR // empty object const emptyObj = {}; // get the number of keys // present in the object // using the Object.keys() method // then use the length property ...
17/8/2020 · To check null in JavaScript, use triple equals operator(===) or Object.is() method. To find the difference between null and undefined, use the triple equality operator or Object.is() method. To loosely check if the variable is null, use a double equality operator(==). Here's a Code Recipe to check if an object is empty or not. For newer browsers, you can use plain vanilla JS and use the new "Object.keys" 🍦 But for older browser support, you can install the Lodash library and use their "isEmpty" method 🤖 const empty = {}; Object.keys(empty).length === 0 && empty.constructor === Object _.isEmpty(empty) If you restrict the question to check if an object exists, typeof o == "object"may be a good idea, except if you don't consider arrays objects, as this will also reported to be the type of objectwhich may leave you a bit confused. Not to mention that typeof nullwill also give you objectwhich is simply wrong.
Nov 01, 2018 - At first glance, null and undefined may seem the same, but they are far from it. This article will explore the differences and similarities between null and undefined in JavaScript. In JavaScript… I'm learing JavaScript. I cannot grasp the idea of an empty object. As I understand, there are situations when I need to check a variable whether it holds an object and has a value. So far, I know ... This will produce the following output −. PS C:\Users\Amit\javascript-code> node demo314.js array1 contains null value array2 does not contains null value object contains null value. AmitDiwan. Published on 26-Oct-2020 11:12:24.
The hasOwnProperty () method returns true if the specified property is a direct property of the object — even if the value is null or undefined. The method returns false if the property is inherited, or has not been declared at all. How to check for null, undefined, empty variables in JavaScript where the variable could be anything like array or object, string, number, Boolean? Hi, coder. So, this is one of the most asked questions that how to check null, undefined, empty variables in JavaScript?
Javascript Null Check How To Check Null Using Object Is
How To Check For An Object In Javascript Object Null Check
7 Tips To Handle Undefined In Javascript
Is Creating Js Object With Object Create Null The Same As
How To Check For Null In Javascript By Dr Derek Austin
How To Check For Null In Javascript By Dr Derek Austin
Why Is Null An Object And What S The Difference Between Null
Understanding Null Safety Dart
How To Check If An Object Is Empty In Javascript
Null Propagation Operator In Javascript
The Top 10 Most Common Mistakes That Node Js Developers Make
How To Check If Object Is Empty In Javascript Samanthaming Com
How Can I Check For An Empty Undefined Null String In
Everything About Javascript Objects By Deepak Gupta
How To Replace A Value If Null Or Undefined In Javascript
How To Check For Null In Javascript By Dr Derek Austin
What Is The Difference Between Null And Undefined In Javascript
How To Check If Json Content Is Null Or Empty Cloudera
How To Check For Null In Javascript By Dr Derek Austin
What Is The Difference Between Null And Undefined In Javascript
How To Check If Object Is Empty In Javascript Samanthaming Com
0 Response to "22 How To Check Object Is Null In Javascript"
Post a Comment