27 Create New Html Element In Javascript
That was the HTML example. Now let's create the same div with JavaScript (assuming that the styles are in the HTML/CSS already). Creating an element. To create DOM nodes, there are two methods: document.createElement(tag) Creates a new element node with the given tag: Then assign it the newly created element with 2:42. document.createElement followed by a set of parentheses. 2:46. To create a new element, pass document.createElement 2:51. the tag name of the HTML element you want to create as a string, in this case, li. 2:55.
How To Get Html Lt Input Gt Element Values Connected To
As you can see it is even simpler than creating elements!. Create Element with jQuery. As an alternative to vanilla JavaScript, we can use the jQuery library to do the same things as creating, adding, removing DOM elements, etc. var div = $('<div/>').text("Hello, World").appendTo(document.body);
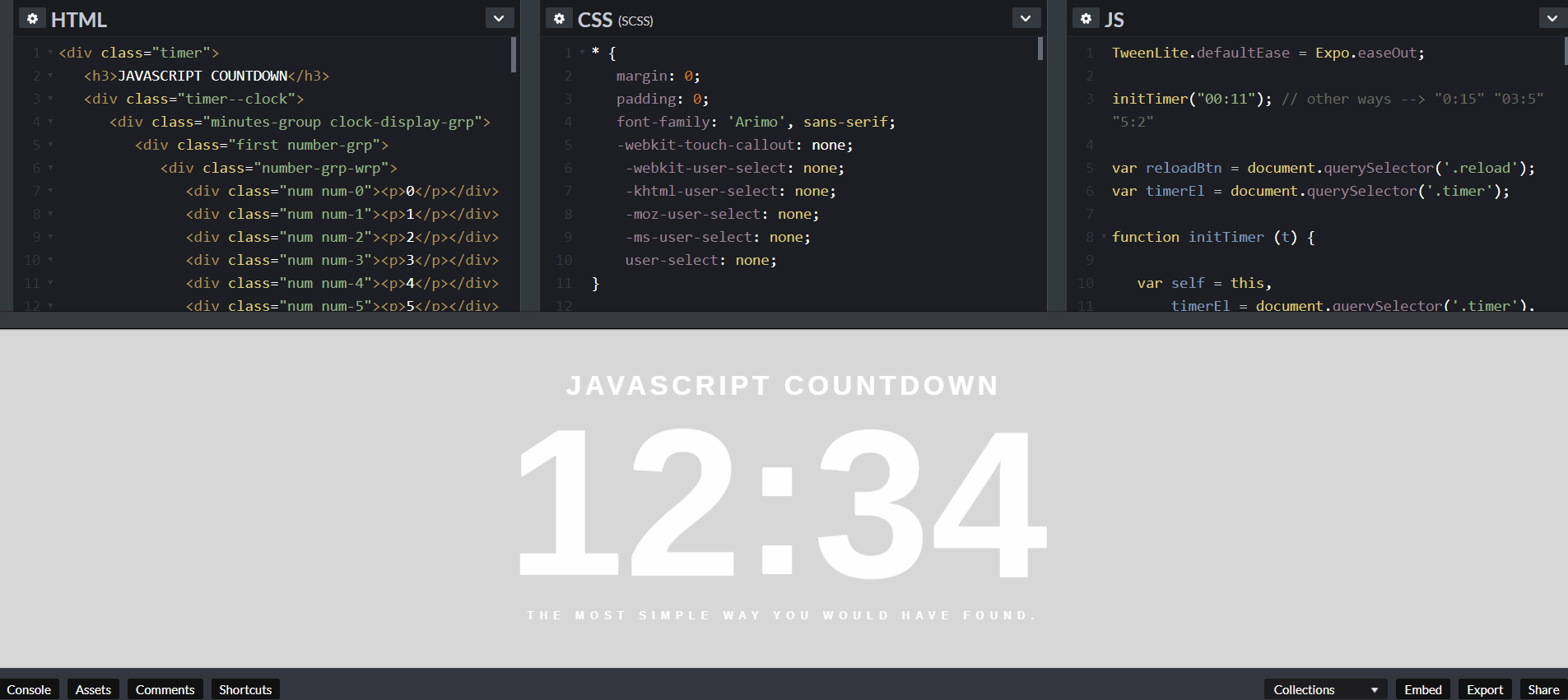
Create new html element in javascript. In this example, we are going to create a list of bookmarks, append bookmarks to parent element and then present it in HTML using plain JavaScript. The starting point is an index.html file that consist of a div element with an ID of bookmarks. The ID is an important and necessary factor in order to locate the element so that we can manipulate it. HTML 5 introduced the <template> element which can be used for this purpose (as now described in the WhatWG spec and MDN docs).. A <template> element is used to declare fragments of HTML that can be utilized in scripts. The element is represented in the DOM as a HTMLTemplateElement which has a .content property of DocumentFragment type, to provide access to the template's contents. In our index.js, we used document.createElement() to dynamically create a new div.Then, we added a dog class to the div we created earlier.. The classList property is used to add, remove, and toggle CSS classes on an element.. Next, we created an image tag with src and alt attributes.. We also created the h2 tag and added text content in it. Additionally, we added a new p element with a class ...
Need to add some content, or update a certain part of the page using Javascript? There are 2 common ways to create and insert HTML elements in Javascript. By directly changing the inner HTML of an element - document.getElementById ("ID").innerHTML = "<p>Text</p>"; By creating and appending a new element. var para = document.createElement ("p"); How to Create Custom Elements The document.registerElement () method is used to create a custom HTML element. This should be passed as the name of your custom element along with an (optional) object that defines the API. In the following example we simply create a new HTML element called <x-treehouse> and then add it to the page. To create a new element with Javascript you use the createElement method of the document object. Like this: var newPara = document.createElement ("p"); In between the round brackets of createElement you type the HTML element you want to create. This needs to go inside of quote marks (single or double). We want to create a new paragraph using ...
How to Create a New Element Using jQuery Like most jQuery operations, creating an element starts with the dollar function, $ (). This is a shortcut to the core jQuery () function. This function has three distinct purposes, it: Defining a new element. To define a new HTML element we need the power of JavaScript! The customElements global is used for defining a custom element and teaching the browser about a new tag. Call customElements.define() with the tag name you want to create and a JavaScript class that extends the base HTMLElement. Summary: in this tutorial, you will learn how to use the JavaScript document.createElement() to create a new HTML element and attach it to the DOM tree. To create an HTML element, you use the document.createElement() method:
customElements.define("my-element", MyElement); Now for any HTML elements with tag <my-element>, an instance of MyElement is created, and the aforementioned methods are called. We also can document.createElement ('my-element') in JavaScript. Custom element name must contain a hyphen - Browsers parse the HTML document and construct the nodes based on the contents of the HTML file. All nodes are created by the browser for the initial load. However, it is possible to dynamically inject new nodes using the document.createElement() method. Create element dynamically inside document body How to Create a New DOM Element from HTML String. HTML5 introduced the <template> element which can be used for creating a DOM element from HTML string. Watch a video course JavaScript - The Complete Guide (Beginner + Advanced) function htmlToElem(html) { let temp = document .createElement ( 'template' ); html = html.trim (); // Never return a ...
Instead of dealing with the <div> 's children, like other answers, if you know you always want to insert after the <a> element, give it an ID, and then you can insert relative to its siblings: <div id="div"> <a id="div_link">Link</a> <span>text</span> </div>. And then insert your new element directly after that element: When an HTML document is loaded in a browser window, it becomes a Document object. Now we can use the Document object to access to all HTML elements (as node objects) in a page, using any script such as JavaScript. Using Document object, we can add or remove element in the html as we do for xml. Adding a new element to a document is very logical. The little-known Option() constructor can be used to create new HTML option elements. So, depending on how you choose to approach this, you can write some pretty efficient code that side-steps the normally verbose syntax of document.createTextNode(), document.createElement(), and document.appendChild() methods.
Creating new HTML Elements - insertBefore() The appendChild() method in the previous example, appended the new element as the last child of the parent. If you don't want that you can use the insertBefore() method: In this tutorial you'll learn how to create HTML elements using JavaScript. In other words, we'll dynamically create HTML elements with JavaScript code. Get... Code language: JavaScript (javascript) How it works: First, select the ul element by its id (menu) using the getElementById() method. Second, create a new list item using the createElement() method. Third, use the insertBefore() method to insert the list item element before the next sibling of the first list item element. Put it all together.
New elements can be dynamically created in JavaScript with the help of createElement () method. The attributes of the created element can be set using the setAttribute () method. The examples given below would demonstrate this approach. Example 1: In this example, a newly created element is added as a child to the parent element. To create a new HTML element using JavaScript, you need to use the createElement() document method and add it to the body of the HTML. Let's say we want to make a paragraph tag with some text content and add it to the body of the HTML. First, Let's create the paragraph tag using the createElement() method. In Javascript, it is possible to programatically create new HTML elements. This lets you add things to the page, given certain conditions. The basics of creating elements # To get started creating elements, we need to define what element it is.
19/7/2019 · To add a new element to the HTML DOM, we have to create it first and then we need to append it to the existing element. Steps to follow. 1) First, create a div section and add some text to it using <p> tags. 2) Create an element <p> using document.createElement("p"). 3) Create a text, using document.createTextNode(), so as to insert it in the above-created element("p"). 4) Using appendChild() try to append the created element, … In an HTML document, the document.createElement () method creates the HTML element specified by tagName, or an HTMLUnknownElement if tagName isn't recognized. Approach 2: Create an empty image instance using new Image (). Then set its attributes like (src, height, width, alt, title etc). Finally, insert it to the document. Example 2: This example implements the above approach. <!DOCTYPE HTML>.
Lines 5 and 6 create a new paragraph element with some simple content, and then lines 8-12 handle inserting the new paragraph into the new document. Line 16 pulls the contentDocument of the frame; this is the document into which we'll be injecting the new content. The next two lines handle importing the contents of our new document into the ... The createElement () method creates an Element Node with the specified name. Tip: After the element is created, use the element.appendChild () or element.insertBefore () method to insert it to the document. First, we give the HTML element a unique id. Then select it with var element = document.getElementById (ID) in Javascript. Finally, take note that innerHTML can be used in two directions. var contents = element.innerHTML will get the current contents of element.
Pure Javascript Building A Real World Application From
Getting Width Amp Height Of An Element In Javascript
Magento 2 Create Html Element With Data Mage Init Attribute
How To Insert Html Element At Certain Point Using Js Jquery
How To Dynamically Create New Elements In Javascript
Methods For Accessing Elements In The Dom File With
Loading Script Files Dynamically
Javascript Dom Document Object Model Guide For Novice
Programmers Sample Guide Dynamically Generate Html Table
Dynamically Add And Remove Html Elements Using Jquery
How To Append Html Code To A Div Using Javascript
Javascript Create Element Javatpoint
Custom Elements Defining New Elements In Html Html5 Rocks
Learn How To Dynamically Create Html Elements With Plain
How To Build A Random Quote Generator With Javascript And
How Can I Create Html Tags Using Javascript Stack Overflow
Inserting Html Elements With Javascript Code Example
Add Javascript To Widgets Elementor Developers
Build A Rich Text Editor With Editor Js By Anurag Kanoria
Html Basics Learn Web Development Mdn
Html For Beginners The Easy Way Start Learning Html Amp Css
Methods For Accessing Elements In The Dom File With
Html Programming With Visual Studio Code
How To Create Your First Login Page With Html Css And
Creating A Javascript Function To Apply Css Rules To A
0 Response to "27 Create New Html Element In Javascript"
Post a Comment