28 Javascript Remove N From String
The substring () method swaps its two arguments if indexStart is greater than indexEnd , meaning that a string is still returned. The slice () method returns an empty string if this is the case. If either or both of the arguments are negative or NaN, the substring () method treats them as if they were 0 . slice () also treats NaN arguments as 0 ... How to remove all line breaks from a string, (Probably you do not want to remove the newlines, but replace them with other whitespace, for example I found this in JavaScript line breaks: Line breaks in strings vary from platform to platform, but the most common ones are the following: Windows: ...
Remove All Whitespace From A String In Javascript Clue Mediator
May 23, 2019 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
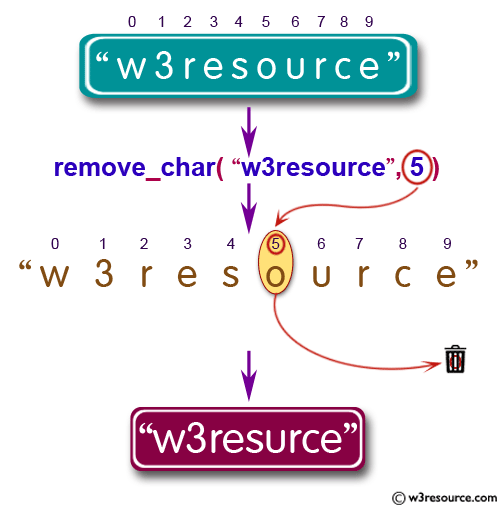
Javascript remove n from string. Javascript replace whitespace with new line, In many real world uses of the javascript code it's probably better to replace the line breaks with a space instead of just removing them entirely. If for instance we To remove newline, space and tab characters from a string, replace them with ... This tells it to replace all instance ... all \n than finally replace all \r. It goes through and removes all types of line breaks from the designated text string. The "gm" at the end of the regex statement signifies that the replacement should take place over many lines (m) and that it should happen more than once (g). The "g" in the javascript replace code ... 17/8/2021 · Javascript remove first N characters from a string using slice () Javascript’s slice (sIndex, eIndex) returns a new string object part of the original string but does not modify it. The sIndex specifies from where the extraction should begin and is the first argument of the method. The index is zero-based, and if this value is negative, it means -> ...
Aug 25, 2020 - how to remove first character from string in javascript ... Error: Node Sass version 5.0.0 is incompatible with ^4.0.0. Learn how to remove line Breaks from text with JavaScript in this tutorial. Javascript string remove all line breaks using split() and join() The split() method in javascript returns an array of substrings formed by splitting a given string. The join() method in javascript joins the elements of the array back into a string. Example:-Remove all line breaks from the string ";\n Javascript \nis a popular \nlanguage \n"
Nov 13, 2020 - In this example,I will learn you how to remove all line breaks from a string using javascript.you can easy and simply remove all line breaks from a string using javascript. Line breaks in strings vary from platform to platform, but the most common ones are the following: Windows: \r\n carriage ... If you wanted to remove say 5 characters from the start, you'd just write substr(5) and you would be left with IsMyString using the above example.. Remove the last character. To remove one character off of the end of a string, we can use the String.slice method. The beautiful thing about slice is it accepts negative numbers, so we don't need to calculate the length like many other ... In this post, we will learn how to remove the last n characters from a string in JavaScript. JavaScript provides slice and substring methods that can be used to remove the last characters from a string. For example, if the string is hello and if we are removing last 3 characters from the string it will print he.
In order to remove all non-numeric characters from a string, replace() function is used. replace() Function: This functiion searches a string for a specific value, or a RegExp, and returns a new string where the replacement is done. Syntax: string.replace( searchVal, newValue ) Parameters: This function accepts two parameters as mentioned above and described below: how to remove newline from string javascript code example · Example 1: javascript remove line breaks from string The second piece of code with the javascript replace method removes all three types of line breaks by using this bit of a regular expression: \r\n|\n|\r. This tells it to replace all instance of the \r\n then replace all \n than finally replace all \r. It goes through and removes all types of line breaks from the designated text string.
The same replace () method will be used in the below code to remove the special characters but keep the spaces (" ") and dot ("."). The simple way is to replace everything except numbers, alphabets, spaces, and dots. Example:-. Remove special characters from the string "Javascript #*is a #popular language." except spaces and dot. Jul 12, 2016 - I am trying to sanitize a string by stripping out the \n but when I do .replace(/\\\n/g, '') it doesn't seem to catch it. I also Google searched and found: ..in accordance with JavaScript regular expression syntax you need two backslash characters in your regular expression literals such as ... javascript string remove from beginning; javascript drop character by index; remove middle from string typescript; remove a char using index from string javascript; js remove first n chars from string; how to slice the first 3 letters off a string javascript; javascript remove character from middle of string
Remove all Whitespace From a String. JavaScript's string.replace() method supports regular expressions (Regex) to find matches within the string. There's a dedicated Regex to match any whitespace character:\s. Combine this Regex to match all whitespace appearances in the string to ultimately remove them: You need a regexp to replace all occurrences; content = content.replace(/\t/g, ''); (g being the global flag) /^\t+/ restricts to replacing leading tabs only, /^\s+/ includes any leading whitespace which is what you would need for "\t\t var" -> "var" Update. You haven't said how the buffer is received & what type it is, my closest guess although its a strange thing to be receiving; I am trying to sanitize a string by stripping out the \n but when I do .replace(/\\\n/g, '') it doesn't seem to catch it. I also Google searched and found: I also Google searched and found: ..in accordance with JavaScript regular expression syntax you need two backslash characters in your regular expression literals such as /\\/ or /\\/g .
There are three methods to remove the text from a string which are listed below: Method 1: Using replace() method: The replace method can be used to replace the specified string with another string. It takes two parameters, first is the string to be replaced and the second is the string which is replacing from the first string. 13/8/2021 · Javascript remove last N characters from a string using slice () Javascript’s slice (startIndex, endIndex) returns a new String object part of the original string. The slice method will not modify the original string. The startIndex is the first argument that specifies from where the extraction should begin. This post will discuss how to remove the first character from a string in JavaScript. Since strings are immutable in JavaScript, we can't in-place remove characters from it. The idea is to create a new string instead. There are three ways in JavaScript to remove the first character from a string: 1. Using substring() method. The substring ...
9/12/2020 · JavaScript program to remove first n characters from a string: In this post, we will learn how to remove the first n characters from a string in JavaScript. For example, if the string is hello and if we want to remove first two characters from the string, it will give llo. Strings are immutable. Given a string and the task is to remove a character from the given string. Method 1: Using replace() method: The replace method is used to replace a specific character/string with other character/string. It takes two parameters, first is the string to be replaced and the second is the string which is to be replaced with. 16/11/2020 · Removing the first n characters. To remove the first n characters of a string in JavaScript, we can use the built-in slice() method by passing the n as an argument. n is the number of characters we need to remove from a string. Here is an example, that removes the first 3 characters from the following string.
How to remove spaces from a string using JavaScript ? Method 1: Using split () and join () Method: The split () method is used to split a string into multiple sub-strings and return them in the form of an array. A separator can be specified as a parameter so that the string is split whenever that separator is found in the string. Kite is a free autocomplete for Python developers. Code faster with the Kite plugin for your code editor, featuring Line-of-Code Completions and cloudless processing. Using substring() method. JavaScript substring() method retrieves the characters between two indexes and returns a new substring. Two indexes are nothing but startindex and endindex. Let's try to remove the first character from the string using the substring method in the below example.
Mar 12, 2016 - NOTE! it only trims the beginning and end of the string, not line breaks or whitespace in the middle of the string. ... This only removes line breaks from the beginning and end of the string. OP asked how to remove ALL line breaks. Remove newline, space and tab characters from a string in Java. Java 8 Object Oriented Programming Programming. To remove newline, space and tab characters from a string, replace them with empty as shown below. replaceAll ("; [\\n\\t ]", ""); Above, the new line, tab, and space will get replaced with empty, since we have used replaceAll () Nov 16, 2020 - In this tutorial, we are going to learn about how to remove the first n characters of a string in JavaScript. ... Now, we want to remove the first 3 characters vol from the above string.
To remove the last n characters of a string in JavaScript, we can use the built-in slice () method by passing the 0, -n as an arguments. 0 is the start index. -n is the number of characters we need to remove from the end of a string. Here is an example, that removes the last 3 characters from the following string. In JavaScript, the replace method is one of the most frequently used methods to remove a character from a string. Of course, the original purpose of this method is to replace a string with another string, but we can also use it to remove a character from a string by replacing it with an empty string. The slice () method extracts parts of a string and returns the extracted parts in a new string. Use the start and end parameters to specify the part of the string you want to extract. The first character has the position 0, the second has position 1, and so on. Tip: Use a negative number to select from the end of the string.
Javascript remove substring from a string using split () and join () The split () method in javascript divides the string into substrings forming an array, and then this array is returned. The join () method in javascript joins the elements of the array back into a string. In Javascript, there is no remove function for string, but there is substr function. You can use the substr function once or twice to remove characters from string. You can make the following function to remove characters at start index to the end of string, just like the c# method first overload String.Remove (int startIndex): JS string class provides a replace method that can be used to replace a substring from a given string with an empty string. This can be used to remove text from either or both ends of the string. Syntax
Method 1 - substring function. Use the JavaScript substring () function to remove the last character from a string in JavaScript. with the using javascript trim string functions to string slice. This function String returns the part of the string between the start part as well as end indexes, or to the end of the string. Syntax: how to remove first character from string in javascript ... Error: Node Sass version 5.0.0 is incompatible with ^4.0.0. Sep 07, 2020 - JavaScript Problems— Remove Line Breaks, Reversing Strings, Node ES6 Modules ... Like any kind of apps, there are difficult issues to solve when we write JavaScript apps.
23/5/2019 · Regular Expression: This method uses regular expressions to detect and replace newlines in the string. It is fed into replace function along with string to replace with, which in our case is an empty string. String.replace( regex / substr, replace with) The regular expression to cover all types of newlines is /\r\n|\n|\r/gm
Javascript Algorithm Remove The Time By Erica N Level Up
Javascript Remove Line Breaks From String Code Example
Python How To Print Without Newline
Python Program To Replace Single Or Multiple Character
Solution Delete Operation For Two Strings Dev Community
Jquery Replace Newline With Br Example Convert New Lines To
Javascript Remove Character From String Code Example
Remove New Line From String Js Code Example
Remove R N With Newline In Json String Stack Overflow
How To Remove Spaces From A String Using Javascript Stack
Javascript Basic Remove A Character At The Specified
Python Program To Remove First Occurrence Of A Character In A
Remove First N Elements From Array Javascript Example Code
How To Remove Array Duplicates In Es6 By Samantha Ming
Remove First Occurrence Of Character In String Javascript
Understanding Regex With Notepad Dr Haider M Al Khateeb
Notepad Simple Trick To Replace Multiple Spaces With
Column Row Edit Change Character Delete Knime
String Remove Last Characters Revit Dynamo
Python Program To Remove Spaces From String
How To Replace White Space Inside A String In Javascript
How To Manipulate A Part Of String Split Trim Substring
Remove Last Character From A String In Javascript Speedysense
How To Manipulate A Part Of String Split Trim Substring
Insert Remove Splice And Replace Elements With Array Splice
Javascript Chop Slice Trim Off Last Character In String
0 Response to "28 Javascript Remove N From String"
Post a Comment