34 Javascript Array Remove Last Element
14/10/2020 · To remove the last element of an array, we can use the built-in pop () method in JavaScript. Here is an example: const fruits = ["apple", "banana", "grapes"]; fruits.pop(); console.log(fruits); // ["apple", "banana"] Note: The pop () method also returns the removed element. Similarly, we can also remove the last element of an array by using the ... Nov 19, 2019 - Different ways to remove elements from an array in JavaScript ... Array.pop will delete the last element of an array.
How To Remove Elements From Javascript Array Simple Examples
JavaScript: Remove Element from an Array, Definition and Usage The shift() method removes the first item of an array. Note: This method changes the length of the array. Note: The return value of the shift method is the removed item. Tip: To remove the last item of an array, use the pop() ...
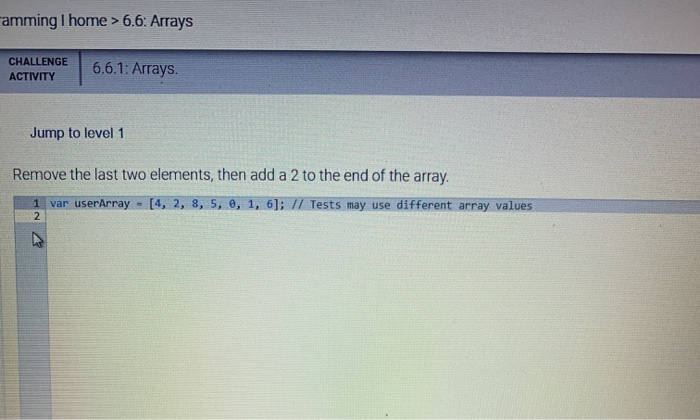
Javascript array remove last element. Remove a particular element on index basis. If you want to remove a specific element from an array from anywhere, you can use the splice() method. Splice can not only remove elements but also replace and add new items. In this article, we only focus on how to remove an element. With splice() you can remove one or more items at once. Define the ... 30/7/2020 · The “pop method” removes the last element of the array, returns that element, and updates the length property. The “pop method” modifies the array on which it is invoked, this means unlike using... The ___________method removes the last element of an array, and returns that element.
17/5/2019 · JavaScript Array pop() Method This method deletes the last element of an array and returns the element. Syntax: array.pop() Return value: It returns the removed array item. An array item can be a string, a number, an array, a boolean, or any other object types that are applicable in an array. To remove the first element of an array, we can use the built-in shift () method in JavaScript. Here is an example: const fruits = ["apple", "banana", "grapes"]; fruits.shift(); console.log(fruits); Note: The shift () method also returns the removed element. Similarly, we can also remove the first element of an array by using the splice ... Hence deleting these items are very easy in JavaScript. 2. Array.prototype.shift () The shift () method removes the first element in an array (that is the item at the index of zero). It also re-orders the remaining elements in the array and decrements the array length by one. Finally, it returns the removed item.
In Javascript, there are two methods in Array.prototype for removing the first element of an array: shift() and splice(). shift() It returns the first element of the array and removes the element ... Posted May 4, 2019. May 15, 2021. by Rohit. JavaScript Array pop method is used to remove the last element from the JS Array and returns that element. This method will modify the Array length by 1. Pop () is one of the methods to use to remove and add four methods. Others 3 methods are shift (), push () and unshift (). The JavaScript shift () method, which is used to remove the first element of the array javascript. The shift method works the same as the pop method, except that it removes the first element of the JavaScript array instead of the last.
The Array pop {pop() method} in JavaScript removes the last element of an array and returns that element. This method changes the length of an array by returning the new array after removing the last element. The Array pop is usually called as pop() method in JavaScript. NOTE: The Array pop returns output as ' undefined ' for an empty array. Method 1: Length property. Simply use Javascript array length property for remove elements from the end in the array. arr.length = 4; You just need to set less value than the current value of array length. So which element index is greater than or equal to the new length will be removed. See below complete example, on click button, will show ... The pop () function is the most straightforward way to achieve this. It removes the last element from an array and returns the deleted element. For example, JavaScript. javascript Copy. var array = [10,11,12,13,14,15,16,17]; array.pop(); console.log(array) Output: JavaScript. javascript Copy.
Instead of a delete method, the JavaScript array has a variety of ways you can clean array values. You can remove elements from the end of an array using pop, from the beginning using shift, or from the middle using splice. The JavaScript Array filter method to create a new array with desired items, a more advanced way to remove unwanted elements. The pop () method removes the last element of an array, and returns that element. Note: This method changes the length of an array. Tip: To remove the first element of an array, use the shift () method. Use the correct Array method to remove the last item of the fruits array.
The pop() method removes the last element of an array, and returns that element. Note: This method changes the length of an array. Tip: To remove the first element of an array, use the shift() method. To remove empty elements from a JavaScript Array, the filter () method can be used, which will return a new array with the elements passing the criteria of the callback function. The filter () method creates an array filled with all array elements that pass a test. To remove null or undefined values do the following: In JavaScript, the Array.splice () method can be used to add, remove, and replace elements from an array. This method modifies the contents of the original array by removing or replacing existing elements and/or adding new elements in place. Array.splice () returns the removed elements (if any) as an array.
Learn how to get the last element from the array in javascript. There are multiple ways through which we can get the last element from the array in javascript. Using pop() We can use the pop() of array which removes and returns the last element of the array. 12/2/2018 · How to remove last array element in JavaScript and return it? Javascript Web Development Front End Technology To remove last array element in JavaScript, use the pop () method. JavaScript array pop () method removes the last element from an array and returns that element. Oct 04, 2020 - array.pop(); //returns popped element //example var fruits = ["Banana", "Orange", "Apple", "Mango"]; fruits.pop(); // fruits= ["Banana", "Orange", "Apple"];
We can remove the last element from an array using the array_pop () function. This function takes an array as its input and returns the last array element, which is being removed. It returns NULL if the array is empty. After removing the last element from the array then the array index is modified, and the length of the array is reduced by one. The array.splice () method is used to add or remove items from an array. This method takes in 3 parameters, the index where the element's id is to be inserted or removed, the number of items to be deleted and the new items which are to be inserted. The ___________method removes the last element of an array, and returns that element.
Sep 29, 2020 - array.pop(); //returns popped element //example var fruits = ["Banana", "Orange", "Apple", "Mango"]; fruits.pop(); // fruits= ["Banana", "Orange", "Apple"]; Use the correct Array method to remove the last item of the fruits array. While push pushes an element to the end of the array, 0:20. pop pops the item off the end, and shift is like the opposite of unshift. 0:23. It removes the first element from an array. 0:28. Let's revisit the shoppingList array and run the pop method on it. 0:31. The last item, coffee, gets removed by pop. 0:35.
So, slice (-1) in your case extracts the last one array element in the sequence and that is 2 (as we have already seen in the above demo). Now, let's talk about the end parameter in the slice () method syntax here. It is again a zero-based index at which extraction from an array ends. So, lets say we have a array like:- The last element has the index of length-1. Since the length field just returns the saved value of elements - this is a fast, efficient and cheap operation. The best way to get the last element of a JavaScript array/list is: var lastElement = myList [myList.length - 1 ]; console .log (lastElement); This results in: 26/8/2020 · Remove the Last Element From Array. pop() removes the last element of an array and returns the removed element. cars.pop(); console.log(cars); Output ["Toyota ", "Volkswagen", "BMW"] Great!!! Try to remove the first and last by our yourself. Keep learning. Thanks for reading!
Remove an Element from an Array in JavaScript. JavaScript recommends some methods to remove elements from the specific Array. You can remove items from the beginning using shift (), from the end of an array using pop (), or from the middle using splice () functions. Let's address them one by one with their examples. If you want to remove last element of the array then the pop method is useful, it will return that element and update the length property. The pop method modifies the array on which it is invoked, This means unlike using delete the last element is removed completely and the array length reduced. Jul 20, 2021 - The pop() method removes the last element from an array and returns that element. This method changes the length of the array.
25/7/2018 · The Array.prototype.shift() method is used to remove the last element from the array and returns that element: let myArray = [ "1" , "2" , "3" , "4" ]; console .log(myArray.shift()); The pop() and shift() methods change the length of the array. Apr 28, 2021 - This post will discuss how to remove the last element from an array in JavaScript... The standard way to remove the last element from an array is with the `pop()` method. This method works by modifying the original array. 16/10/2020 · Now, we need to remove the first element apple, the last element grapes from the above array. Using the shift () and pop () methods To remove the first and last element from an array, we can use the shift () and pop () methods in JavaScript. Here is an example:
Please turn on JavaScript in your browser and refresh the page to view its content Removing an element from the end of an array The Array.pop () method can be used to remove an element from the end of an array. This method removes the last element and returns it: push () / pop () — add/remove elements from the end of the array unshift () / shift () — add/remove elements from the beginning of the array concat () — returns a new array comprised of this array joined with other array (s) and/or value (s) Found a problem with this page?
All You Need To Know About Javascript Arrays With Example
How To Remove The Last Element Of An Array In C Quora
Delete The Array Elements In Javascript Delete Vs Splice
Java Exercises Remove A Specific Element From An Array
How To Remove Element From An Array In Javascript Codevscolor
Javascript Remove Element From An Array
Remove An Element From An Array In Javscript Delete Element
Insert Remove Splice And Replace Elements With Array Splice
How To Remove Last Element From Array Javascript
Javascript Removes The Last Element From An Array Example Code
Remove Selected Element From Array Javascript Code Example
Get The Last Item In An Array Stack Overflow
Java Exercises Remove A Specific Element From An Array
Remove Last Node Of The Linked List Geeksforgeeks
Remove Elements From Lists Python List Remove Method
Remove Comma From Last Element Of An Array Javascript Javascript Tutorial Javascript Course
Javascript Array Remove An Element Javascript Remove Element
Js How To Remove Item From Array Code Example
How To Remove Commas From Array In Javascript
The Best Way To Remove The First Element Of An Array In
Get The Last Item In An Array Javascript
Remove Element From Array Javascript First Last Value
Javascript Remove Object From Array
Pop Push Shift And Unshift Array Methods In Javascript
Javascript Remove The Last Item From An Array Geeksforgeeks
Python Print List After Removing Element At Given Index
Javascript Remove Last Element From Array Without Mutation
Remove A Specific Element From Array
Javascript Array Push And Pop Method
Removing Elements From An Array In Javascript Edureka
How Can I Remove Elements From Javascript Arrays O Reilly
Java Remove Theast Two Elements Then Add A 2 To The Chegg Com
Javascript Remove First Element From Array
0 Response to "34 Javascript Array Remove Last Element"
Post a Comment