29 Javascript Post To Server
Post data to server from javascript on client side. milkyway. Hello everyone, I have a few values and variables that I want to post to a server (without using a SUBMIT button). Is there a way to post data from within javascript - do sockets or connections have to be open for this xhr = new XMLHttpRequest(); var url = "url?email=hey@mail &password=101010"; xhr.open("GET", url, true); xhr.onreadystatechange = function () { if (xhr.1 answer · 4 votes: Sending AJAX request using POST method var xhr = new XMLHttpRequest(); var url = "url"; var data = "email=hey@mail &password=101010"; xhr.open("POST", ...
Ajax Jquery Post List Javascript Objects To Springboot Server
The $.post () method requests data from the server using an HTTP POST request.
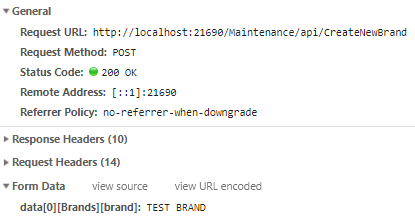
Javascript post to server. By sending asynchronously, the JavaScript does not have to wait for the server response, but can instead: execute other scripts while waiting for server response deal with the response after the response is ready The default value for the async parameter is async = true. Jul 19, 2021 - To send a POST request using vanilla JavaScript, you must use an XMLHttpRequest object to interact with the server and provide the correct Content-Type request header for the POST message body data. In this JavaScript POST request example, the Content-Type: application/json header indicates ... Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
Our client application running inside the web browser will need to issue requests to the REST web server. The browser's Javascript interpreter provides an API for this called XMLHttpRequest, but the actual implementation varies from browser to browser, so we would need to write browser specific code if we wanted to code against this API directly. Send Ajax GET and POST requests Load data asynchronously from the server using GET or POST HTTP requests. Set data type (xml, json, script, text, html) and decode returned data. The following helper function allows sending an Ajax request via GET method - an equivalent to jQuery's $.get (). Find out how to make an HTTP POST request using Node.js ... The V8 JavaScript Engine Run Node.js scripts from the command line ... JavaScript Promises Modern Asynchronous JavaScript with Async and Await The Node.js Event emitter Build an HTTP Server Making HTTP requests with Node.js Make an HTTP POST request using Node.js Get HTTP request body ...
Now, JavaScript has its own built-in way to make API requests. This is the Fetch API, a new standard to make server requests with promises, but includes many other features. In this tutorial, you will create both GET and POST requests using the Fetch API. Prerequisites. To complete this tutorial, you will need the following: Nov 08, 2011 - EDIT: You can set up the AJAX request to post to a server-side script (acting as a proxy) on your own domain, and have that script turn around and post the data to your remote server. ... Not the answer you're looking for? Browse other questions tagged javascript remote-server or ask your own ... May 07, 2020 - JavaScript has great modules and ... from a server side resource. In this article, we are going to look at a few popular ways to make HTTP requests in JavaScript. ... Ajax is the traditional way to make an asynchronous HTTP request. Data can be sent using the HTTP POST method and ...
XMLHttpRequest.send () The XMLHttpRequest method send () sends the request to the server. If the request is asynchronous (which is the default), this method returns as soon as the request is sent and the result is delivered using events. If the request is synchronous, this method doesn't return until the response has arrived. JavaScript basics (see first steps, building blocks, JavaScript objects), the basics of Client-side APIs: Objective: To learn how to fetch data from the server and use it to update the contents of a web page. What is the problem here? Originally page loading on the web was simple — you'd send a request for a website to a server, and as long ... This JavaScript function is very simple, first, we search for the three inputs using 'getElementById', once we have data from these three inputs, we post data to the server, to a handler called 'MailHandler.ashx', the format used is JSON.
Oct 23, 2016 - That might have been because the ... (It only displayed on server that we received a POST.) I've updated the code to actually respond in the POST and that takes care of it. I also included the HTML that I used to test it (which includes your JavaScript code)... 8/9/2009 · this .Page.ClientScript.GetPostBackEventReference (options); returns a JavaScript function that will post back to the server. It is important to note that calling base.Render (writer) isn't strictly necessary. The reason I do so is to render the button on the … Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
JSON is used to send data to and from the server in text format. JSON stands for JavaScript Object Notation. Sending and receiving data between client and server should be as fast as possible. JSON is used by many programming language like perl, php, java etc since it is language independent. The WebSocket protocol, described in the specification RFC 6455 provides a way to exchange data between browser and server via a persistent connection. The data can be passed in both directions as "packets", without breaking the connection and additional HTTP-requests. WebSocket is especially great for services that require continuous data exchange, e.g. online games, real-time trading ... This back and forth happens a bunch of times, and all of this is fully supported in JavaScript! This ability to asynchronously request and process data from a server without requiring a page navigation/reload has a term. That term is Ajax (or AJAX if you want to shout).
16/3/2016 · Web-based applications run smoother if instead of using the traditional form method, they use JavaScript to post data to the server and to update the user interface after posting data: It also makes it easier to keep POST and GET actions separated. SignalR makes it even slicker; it can even update multiple pages at the same time. The method attribute defines how data is sent. The HTTP protocol provides several ways to perform a request; HTML form data can be transmitted via a number of different methods, the most common being the GET method and the POST method. To understand the difference between those two methods, let's step back and examine how HTTP works.Each time you want to reach a resource on the Web, the ... URL is the address of server or using any function on the server side that accept the HTTP-POST. Solution 2: Here using javascript framework to be simple and quick implementation.
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Pass arrays of data to the server (while still ignoring the return results). ... Alert the results from requesting test.php (HTML or XML, depending on what was returned). Alert the results from requesting test.php with an additional payload of data (HTML or XML, depending on what was returned). Post ... GET is less secure compared to POST because data sent is part of the URL. Never use GET when sending passwords or other sensitive information! POST is a little safer than GET because the parameters are not stored in browser history or in web server logs. Visibility. Data is visible to everyone in the URL.
20/10/2020 · From javascript to server. I have this code which allows you to upload an image onto the canvas and mark out segments on the canvas. When the canvas is double clicked, the coordinates of the segments would be added to an array. Use JSON.stringify() to convert the JavaScript object into a JSON string. Send the URL-encoded JSON string to the server as part of the HTTP Request. This can be done using the HEAD, GET, or POST method by assigning the JSON string to a variable. It can also be sent as raw text using the POST ... AJAX is the only way that allows communicating client-side with the server-side. It is easier to send AJAX requests using JavaScript libraries or frameworks. But it is also good to know how to send AJAX request with plain Javascript. Use the XMLHttpRequest object to communicate with the server.
[JavaScript/AJAX Code] To post HTML form data to the server in URL-encoded format using JavaScript/AJAX, you need to make an HTTP POST request to the server and provide the HTML form data in the body of the JavaScript/AJAX POST message. You also need to specify the data type using the Content-Type: application/x-www-form-urlencoded request header. Using Fetch to Post Data. The Fetch API is not limited to GET requests only. You can make all other types of requests (POST, PUT, DELETE, etc.) with custom request headers and post data. Here is an example of a POST request: Sending Form Data via JavaScript. Right now, clicking submit on the form doesn't go anywhere. Since we don't have an action that leads to a URL, the form will just post to itself by default. Since index.html is an html file, not a PHP file, no form processing can happen on this page. Instead, we'll send the form to PHP through JavaScript.
The first step is to attach an event handler the <form> to capture the submit event: const form = document.querySelector('#signup-form'); form.addEventListener('submit', () => { }); The next step is to create and send an actual POST request. If you are already familiar with jQuery, sending a POST request is quite similar to the $.post () method. The jQuery post () method sends asynchronous http POST request to the server to submit the data to the server and get the response. Syntax: $.post (url, [data], [callback], [type]); Parameter Description: url: request url from which you want to submit & retrieve the data. data: json data to be sent to the server with request as a form data. However, always use POST requests when: A cached file is not an option (update a file or database on the server). Sending a large amount of data to the server (POST has no size limitations). Sending user input (which can contain unknown characters), POST is more robust and secure than GET.
Building an HTTP request by hand can be overwhelming. Fortunately, the XMLHttpRequest specification provides a newer, simpler way to handle form data requests with the FormData object.. The FormData object can be used to build form data for transmission, or to get the data within a form element to manage how it's sent. Note that FormData objects are "write only", which means you can change ... Sep 26, 2019 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. 22/7/2014 · You can use AJAX to post data to a server without any direct UI interaction. I will break down a simple jQuery example below: $.ajax ( { type: "POST", url: url, data: data, success: success, }); $.ajax Is a method offered by the jQuery framework to make AJAX requests simple and …
var xhr = new XMLHttpRequest(); xhr.open("POST", yourUrl, true); xhr. ... There is an easy method to wrap your data and send it to server as if you were ...12 answers · 202 votes: You can send it and insert the data to the body: var xhr = new XMLHttpRequest(); xhr.open("POST", ...
How To Send A Post And Get Request In Javascript Using No
React Js Image Preview And Upload To Server Class Component
Web Apps Client Side Rendering Ssr Pre Rendering Toptal
Javascript Fetch Post To Express Server Fails The Server
Upload Files Using Multer On Server In Nodejs And Expressjs
File Uploading In React Js Geeksforgeeks
Sending Data To Web Using Nodejs Via Serial Port Embedds
Introduction To The Server Side Learn Web Development Mdn
Ajax Jquery Post List Javascript Objects To Springboot Server
A Guide To Using Php To Download Onedrive Files Selected By
Sending Form Data Learn Web Development Mdn
Belajar Html Javascript Python Dll Dm Me Shell Backdoor
Here Are The Most Popular Ways To Make An Http Request In
Why The Request From The Javascript Client To The Java Server
How To Wait Until The Previous Post Request Is Completed In
Javascript Complete Ajax Tutorial Get Amp Post Request In
Send Draft Js Data To A Server And Fetch It With React Hooks
Http Post Request Nodejs Code Example
Ajax Jquery Post List Javascript Objects To Springboot Server
Post Data And Redirect To External Web Server With Curl In
Simple Custom Post Request Datatables Forums
Here Are The Most Popular Ways To Make An Http Request In
Sending Form Data Learn Web Development Mdn
The Journey To Isomorphic Rendering Performance Javascript
Form Submission Using Javascript Ajax And Php Bootstrapfriendly
Unsure Why My Javascript Fetch Call Is Failing When The
0 Response to "29 Javascript Post To Server"
Post a Comment