27 Check If Object Undefined Javascript
In JavaScript, checking if a variable is undefined can be a bit tricky since a null variable can pass a check for undefined if not written properly. As a result, this allows for undefined values to slip through and vice versa. Make sure you use strict equality === to check if a value is equal to undefined. 2 weeks ago - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
How To Check If The Variable Is Undefined
Note the use of a quoted 'undefined' instead of the undefined JavaScript keyword. That is because the typeof operator always returns a string. So how do we check if a property is defined in an object or not?
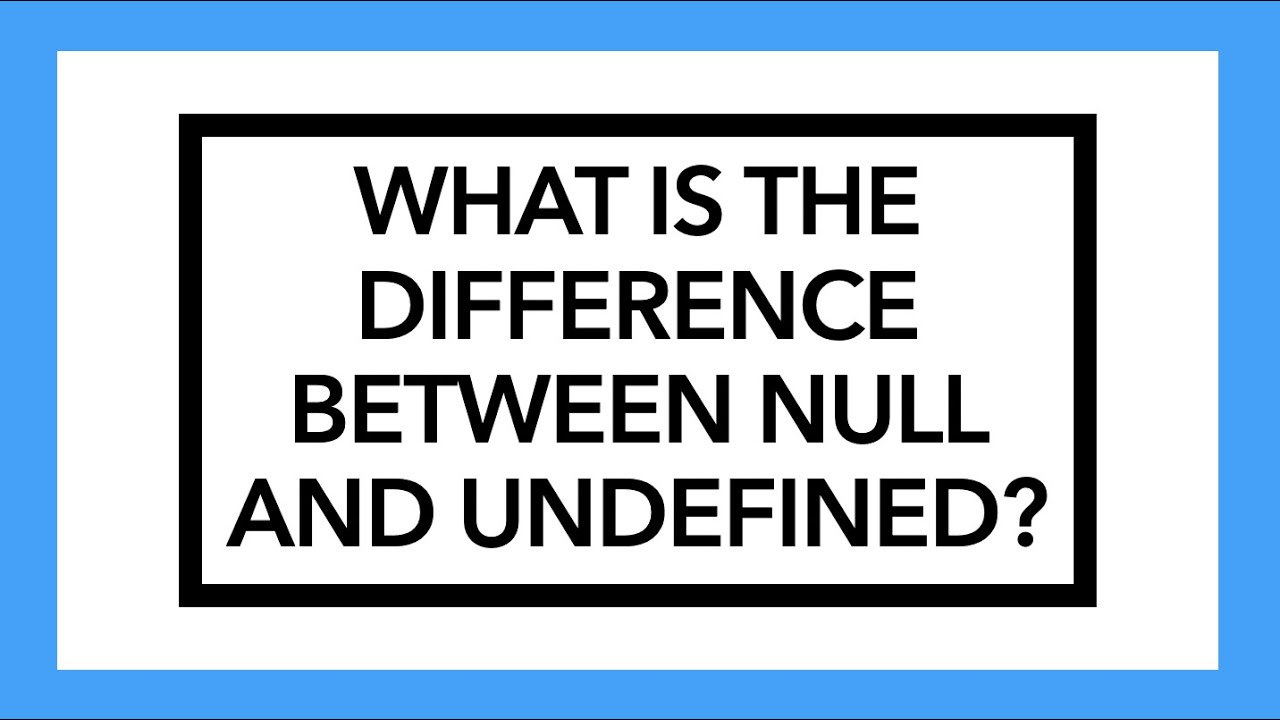
Check if object undefined javascript. You can use the typeof operator in combination with the strict equality operator (===) to check or detect if a JavaScript object property is undefined. Answer: Use the equality operator ( ==) In JavaScript if a variable has been declared, but has not been assigned a value, is automatically assigned the value undefined. Therefore, if you try to display the value of such variable, the word "undefined" will be displayed. Whereas, the null is a special assignment value, which can be assigned to a ... JavaScript provides the typeof operator to check the value data type. The operator returns a string of the value data type. For example, for an object, it will return "object". However, for arrays and null, "object" is returned, and for NaN/Infinity, "number" is returned. It is somehow difficult to check if the value is exactly a real object.
JavaScript has no built-in .length or .isEmpty methods for objects to check if they are empty. So we have to create our own utility method or use 3rd-party libraries like jQuery or Lodash to check if an object has own properties. Get code examples like"how to check if object is undefined in javascript". Write more code and save time using our ready-made code examples. In javascript, we can check if an object is empty or not by using. JSON.stringify. Object.keys (ECMA 5+) Object.entries (ECMA 7+) And if you are using any third party libraries like jquery, lodash, Underscore etc you can use their existing methods for checking javascript empty object.
Previous value: 'ngIf: [object Object]'. Current value: 'ngIf: true'. ... Element implicitly has an 'any' type because expression of type '0' can't be used to index type 'Validator<unknown[]>'. Property '0' does not exist on type 'Validator<unknown[]>' ... Which is not an example of a JavaScript ... In a JavaScript program, the correct way to check if an object property is undefined is to use the typeof operator. typeof returns a string that tells the type of the operand. It is used without parentheses, passing it any value you want to check: Undefined properties, like someExistingObj.someUndefProperty. An undefined property doesn't yield an error and simply returns undefined, which, when converted to a boolean, evaluates to false. So, if you don't care about 0 and false, using if (obj.undefProp) is ok. There's a common idiom based on this fact:
We can use the typeof operator to check if an object property is undefined. The typeof operator returns the string representation of a given object property type. if (typeof obj.age === 'undefined'){ console.log('age property is undefined'); } The explicit approach, when we are checking if a variable is undefined or null separately, should be applied in this case, and my contribution to the topic (27 non-negative answers for now!) is to use void 0 as both short and safe way to perform check for undefined. Here's a Code Recipe to check if an object is empty or not. For newer browsers, you can use plain vanilla JS and use the new "Object.keys" 🍦 But for older browser support, you can install the Lodash library and use their "isEmpty" method 🤖 const empty = {}; Object.keys(empty).length === 0 && empty.constructor === Object _.isEmpty(empty)
In JavaScript you can check if the property of an object is undefined. Based on the outcome you can adjust your flows. Aug 02, 2020 - Because toAppend object can omit first or last properties, it is obligatory to verify whether these properties exist in toAppend. A property accessor evaluates to undefined if the property does not exist. The first temptation to check whether first or last properties are present is to verify ... Jun 21, 2020 - How to check if a property exists in an object in JavaScript by using the hasOwnProperty() method, the in operator, and comparing with undefined.
The undefined is a primitive type in JavaScript. So the undefined is a type. And the undefined type has exactly one value that is undefined. JavaScript uses the undefined value in the following situations. 1) Accessing an uninitialized variable. When you declare a variable and don't initialize it to a value, this variable will have a value ... The easiest way to check if an object property exists but is undefined in JavaScript is to make use of the Object.prototype.hasOwnProperty method which will let you find properties in an object. Here the type of variable is undefined. If you assigned a value(var geeks === undefined ) it will show, if not it will also show undefined but in different meaning. Here the undefined is the typeof undefined. In this, null will display if you assigned null to a variable, null is loosely equals to undefined: But here typeof will show object.
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Nov 01, 2018 - At first glance, null and undefined may seem the same, but they are far from it. This article will explore the differences and similarities between null and undefined in JavaScript. In JavaScript… In JavaScript, a variable can be either defined or not defined, as well as initialized or uninitialized. typeof myVar === 'undefined' evaluates to true if myVar is not defined, but also defined and uninitialized. That's a quick way to determine if a variable is defined.
JavaScript variables start with the value of undefined if they are not given a value when they are declared (or initialized) using var, let, or const. Variables that have not been declared usually... Even if the property name exists (but has undefined value), hero.name !== undefined evaluates to false: which incorrectly indicates a missing property.. 4. Summary. There are mainly 3 ways to check if the property exists. The first way is to invoke object.hasOwnProperty(propName).The method returns true if the propName exists inside object, and false otherwise. The in operator is another way to check the presence of a property in an object in JavaScript. It returns true if the property exists in an object. Otherwise, it returns false. Let us use the in operator to look for the cake property in the food object:
check undefined object javascript one liner set to emtpy; check type javascript; how to check if array is empty or not in javascript; query selector has clas; classList has class; javascript hasclass; convert empty string to boolean javascript; javascript check if boolean is undefined; isset js; Nov 26, 2019 - Previous value: 'ngIf: [object Object]'. Current value: 'ngIf: true'. ... Element implicitly has an 'any' type because expression of type '0' can't be used to index type 'Validator<unknown[]>'. Property '0' does not exist on type 'Validator<unknown[]>' ... Which is not an example of a JavaScript ... Aug 28, 2020 - Method 1: Using the typeof operator The typeof operator returns the type of the variable on which it is called as a string. The return string for any object that does not exist is “undefined”. This can be used to check if an object exists or not, as a non-existing object will always return ...
JavaScript check undefined. To check undefined in JavaScript, use (===) triple equals operator. In modern browsers, you can compare the variable directly to undefined using the triple equals operator. There are also two more ways to check if the variable is undefined or not in JavaScript, but those ways are not recommended. In versions of JavaScript prior to ECMAScript 5, the property named "undefined" on the global object was writeable, and therefore a simple check foo === undefinedmight behave unexpectedly if it had accidentally been redefined. In modern JavaScript, the property is read-only. In JavaScript, one of the everyday tasks while validating data is to ensure that a variable, meant to be string, obtains a valid value. This snippet will guide you in finding the ways of checking whether the string is empty, undefined, or null. Here we go. If you want to check whether the string is empty/null/undefined, use the following code:
Jul 20, 2021 - JavaScript is a statically scoped language, so knowing if a variable is declared can be read by seeing whether it is declared in an enclosing context. The global scope is bound to the global object, so checking the existence of a variable in the global context can be done by checking the existence ... May 20, 2020 - Previous value: 'ngIf: [object Object]'. Current value: 'ngIf: true'. ... Element implicitly has an 'any' type because expression of type '0' can't be used to index type 'Validator<unknown[]>'. Property '0' does not exist on type 'Validator<unknown[]>' ... Which is not an example of a JavaScript ... Checking if a key exists in a javascript object - Checking for undefined-ness is not an accurate way of testing whether a key exists.
If you are still concerned, there are two ways to check if a value is undefined even if the global undefined has been overwritten. You can use the void operator to obtain the value of undefined. This will work even if the global window.undefined value has been over-written: if (name === void (0)) {...} The typeof operator for undefined value returns undefined. Hence, you can check the undefined value using typeof operator. Also, null values are checked using the === operator. Note: We cannot use the typeof operator for null as it returns object.
Why Is Null An Object And What S The Difference Between Null
Javascript Undefined Code Example
Undefined Vs Null Find Out The Top 8 Most Awesome Differences
Object Key Undefined Stack Overflow
Uncaught Typeerror Cannot Read Property Of Undefined
How To Check If A Javascript Array Is Empty Or Not With Length
How To Check If A Javascript Object Property Is Undefined
Js Difference Between Null And Undefined By Ejiro Edwin
What Is The Difference Between Null And Undefined In Javascript
Top 10 Javascript Errors From 1000 Projects And How To
Understanding Null And Undefined In Javascript By Chidume
Is There A Standard Function To Check For Null Undefined Or
4 Ways To Check If The Property Exists In Javascript Object
4 Ways To Check If The Property Exists In Javascript Object
How Not To Sort An Array In Javascript Phil Nash
How To Check If A Javascript Array Is Empty Or Not With Length
Checking If An Array Contains A Value In Javascript
Javascript Lesson 4 Null And Undefined Type In Javascript
How To Check If A Variable In Javascript Is Undefined Or Null
7 Tips To Handle Undefined In Javascript
How To Check If An Object Has A Property Properly In
Understanding Null Undefined And Nan By Kuba Michalski
How To Check For An Undefined Or Null Variable In Javascript
How To Check If A Javascript Promise Has Been Fulfilled
Everything About Javascript Objects By Deepak Gupta
Use Typeof Operator To Check If A Variable Is A Primitive
0 Response to "27 Check If Object Undefined Javascript"
Post a Comment