32 Javascript Map Use Object As Key
Apart from the built-in method, it is easy to use an external library like Lodash to help you map over an object's keys. Lodash provides the _.keys method for mapping over an object's keys. There is a slight overhead in terms of performance that you will encounter by using this method, but it is also more succinct. How to Find Element into Object keys Object.Keys () method Uses & Example The JavaScript Object.keys () method returns an array of the given object's property names.The keys may be array index or object named keys.The object.keys return the array of strings that have enumerable properties of passed object.
2 weeks ago - The Map object holds key-value pairs and remembers the original insertion order of the keys. Any value (both objects and primitive values) may be used as either a key or a value.
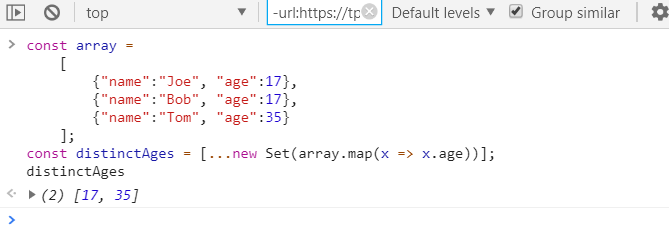
Javascript map use object as key. 1 week ago - The Object.entries() method returns an array of a given object's own enumerable string-keyed property [key, value] pairs. This is the same as iterating with a for...in loop, except that a for...in loop enumerates properties in the prototype chain as well). Following is the code showing Object.keys().map() and Array.map() in JavaScript −Example Live Demo<!DOCTYPE html> Definition and Usage. The map() method creates a new array with the results of calling a function for every array element.. The map() method calls the provided function once for each element in an array, in order.. map() does not execute the function for empty elements. map() does not change the original array.
The map accepts any key type As presented above, if the object's key is not a string or symbol, JavaScript implicitly transforms it into a string. Contrary, the map accepts keys of any type: strings, numbers, boolean, symbols. Moreover, the map preserves the key type. Dec 16, 2019 - Before ES6, to make a dictionary ... the keys and values. This has some problems that can be avoided with maps. An object lets us map strings to values. However, with the pitfalls of JavaScript objects and the existence of the Map constructor, we can finally stop using objects as maps or ... JavaScript's Map object has a handy function, forEach (), which operates similarly to arrays' forEach () function. JavaScript calls the forEach () callback with 3 parameters: the value, the key, and the map itself.
JavaScript Map Object Map in JavaScript is a collection of keyed data elements, just like an Object. But the main difference is that the Map allows keys of any type. A Map object iterates its items in insertion order and using the for…of loop, and it returns an array of [key, value] for each iteration. Maintain a tree of Map objects. Each tree stores: Under an internally-declared Symbol key: The value at that point in the tree (if any). The Symbol guarantees uniqueness, so no user-provided value can overwrite this key.. On all its other keys: all other so-far set next-trees from this tree. For example, on akmap.set(['a', 'b'], true), the internal tree structure would be like— A lightweight introduction into some of array methods in JavaScript, and how work with objects in an iterative manner. Object.keys() Object…
If we'd like to apply them, then we can use Object.entries followed by Object.fromEntries: Use Object.entries (obj) to get an array of key/value pairs from obj. Use array methods on that array, e.g. map, to transform these key/value pairs. Use Object.fromEntries (array) on the resulting array to turn it back into an object. Map object can hold both objects and primitive values as either key or value. When we iterate over the map object it returns the key,value pair in the same order as inserted. JavaScript Map has() method. The JavaScript map has() method indicates whether the Map object contains the specified key. It returns true if the specified key is present, otherwise false. Syntax. The has() method is represented by the following syntax:
Jul 06, 2018 - The internet is a great place to find information, but there is one teeny-tiny problem. You are on a boat in the middle of a deep blue sea. Although most of us do not venture all the way down into… Apr 03, 2017 - Getting value out of maps that are treated like collections is always something I have to remind myself how to do properly. In this post I look at JavaScript object iteration and picking out values from a JavaScript object by property name or index. A Map is a key-value data structure in ES6. A Map object stores each element as Key-value pairs in the format - (key, value), which contains a unique key and value mapped to that key. And due to the uniqueness of each stored key, there is no duplicate pair stored. JavaScript Maps are an object that allows us to store collections in key-value ...
But it produces an "Array" (while I need an Object) of {key : 5}... with the string "key" instead of the name of the key. javascript ecmascript-6 javascript-objects Share Jul 19, 2020 - As a result, we can’t have unique types held as keys on our objects. In a number of ways, the JS object lacks flexibility and does things we wouldn’t expect. But since the addition of ES6 JavaScript, we have a data type called a map that is often glossed over. Dec 02, 2020 - Both of these data structures add additional capabilities to JavaScript and simplify common tasks such as finding the length of a key/value pair collection and removing duplicate items from a data set, respectively. On the other hand, Objects and Arrays have been traditionally used for data ...
Aug 08, 2016 - But .map is generally understood ... in javascript can be an Object, including things with their own very distinct and defined .map interfaces/logic. ... In my opinion first argument of callback should be iterated element itself, not a key. For example i assume it should ... The Object.keys () method was introduced in ES6. It takes the object that you want to iterate over as an argument and returns an array containing all properties names (or keys). You can then use any of the array looping methods, such as forEach (), to iterate through the array and retrieve the value of each property. Here is an example: Everything in JavaScript is an object, and methods are functions attached to these objects..call () allows you to use the context of one object on another. Therefore, you would be copying the context of.map () in an array over to a string.
19/8/2020 · JavaScript map value to keys (reverse object mapping) We are required to write a function reverseObject () that takes in an object and returns an object where keys are mapped to values. We will approach this by iterating over Object.keys () and pushing key value pair as value key pair in the new object. Introduction to JavaScript Map object Prior to ES6, when you need to map keys to values, you often use an object, because an object allows you to map a key to a value of any type. However, using an object as a map has some side effects: An object always has a default key like the prototype. In custom object as a key in HashMap example, I will show you how to work with user defined objects as keys in Map. To use user defined objects as keys in Map you need to tell Java how to compare user defined objects by overriding the hashCode() and equals() methods. Most of the time you generally use Java API provided classes, for example ...
1/11/2011 · The object keys, after getting stringified, overwrite each other. o[x] and o[y] both are references to the string [object Object].o.x is a true references to key named x of the object o, similarly o.y.. How to use object as key to a value #. There is a way to use objects and keys to values, just not values of a conventional Object.What you need is a WeakMap. So I'm wondering, what's the point of being able to use object keys in `Map` objects if the equality check performed is reference equality? I feel like I'm either missing something obvious here or we have a usability issue on our hands. Benjamin (Also asked in SO http://stackoverflow /q... The Object.keys () method returns an array of a given object's own enumerable property names, iterated in the same order that a normal loop would.
25/11/2020 · Although map [key] also works, e.g. we can set map [key] = 2, this is treating map as a plain JavaScript object, so it implies all corresponding limitations (only string/symbol keys and so on). So we should use map methods: set, get and so on. Map can also use objects as keys. In JavaScript, objects are used to store multiple values as a complex data structure. An object is created with curly braces {…} and a list of properties. A property is a key-value pair where the key must be a string and the value can be of any type.. On the other hand, arrays are an ordered collection that can hold data of any type. In JavaScript, arrays are created with square brackets ... Feb 16, 2017 - Maps are designed as an alternative to using Object literals for storing key/value pairs that require unique keys, and provide very useful methods for iteration. ... Nothing is more fundamental in JavaScript than object literals. Creating a map of sorts is as simple as declaring it in code.
There is one important thing to note about using an Object or Array as a key: the Map is using the reference to the Object to compare equality, not the literal value of the Object. In JavaScript {} === {} returns false, because the two Objects are not the same two Objects, despite having the same (empty) value. Map is a data structure in JavaScript which allows storing of [key, value] pairs where any value can be either used as a key or value. The keys and values in the map collection may be of any type and if a value is added to the map collection using a key which already exists in the collection, then the new value replaces the old value. Aug 17, 2018 - As you can see, even though we are are using two empty objects as keys, we are using the references of those objects in the map. Therefore, Object.is(), who is used for comparing the keys, returns false. Again, notice that the object are not coerced into strings.
Nov 09, 2018 - Regular Object (pay attention to the word ‘regular’ ) in Javascript is dictionary type of data collection — which means it also follows key-value stored concept like Map. Each key in Object — or we normally call it “property” — is also unique and associated with a single value. So, when you pass the key "programmer" to the object, it returns the matching value that is 2. Aside from looking up the value, you may also wish to check whether a given key exists in the object. The object may have only unique keys, and you might want to check if it already exists before adding one. JavaScript map with an array of objects JavaScript map method is used to call a function on each element of an array to create a different array based on the outputs of the function. It creates a new array without modifying the elements of the original array.
Aug 13, 2019 - Among the goodies introduced to JavaScript in ES6, we saw the introduction of Sets and Maps. Unlike ordinary objects and arrays, these are ‘keyed collections’. That means their behaviour is subtly different and — used in the right contexts — they offer considerable performance… Introduction to JavaScript Map Object. The JavaScript map object is a type of collection that allows storing values based on key-value pair terminology. The map object is introduced in the ES6 specification of JavaScript and it allows storing the pairs of keys and respective values in it.
Create An Object From A Map Or Key Value Pairs In Javascript
How To Sort Order Keys In Javascript Objects Geeksforgeeks
Map Vs Object In Javascript Stack Overflow
Map Get Key From Value Javascript Stackoverflow Code Example
How To Check A Key Exists In Javascript Object Geeksforgeeks
This Image Displays The Structure Of The Map Object Left
Javascript Object Convert An Object Into A List Of Pairs
Understanding Map And Set Objects In Javascript Digitalocean
Javascript Map Data Structure With Examples Dot Net Tutorials
Javascript Object Exists Key Design Corral
Javascript Array Distinct Ever Wanted To Get Distinct
Javascript Replacement 6 Key Value Vs Data Develop Paper
Github Pana Two Way Map Js Object Get Value By Key And
Javascript Map Objects Community Help The Observable Forum
Sorting Object Property By Values Stack Overflow
Convert Object Keys According To Table Map Object Code
Using The Map Function In Javascript And React By Jonathan
Es6 Collections Using Map Set Weakmap Weakset Sitepoint
Everything About Javascript Objects By Deepak Gupta
Rewriting Javascript Using Maps Recently I Wrote An Article
Javascript Map Data Structure With Examples Dot Net Tutorials
3 Ways To Add Dynamic Key To Object In Javascript Codez Up
When To Use Map Instead Of Plain Javascript Object
How To Remove A Property From A Javascript Object
Java Hashmap Containskey Object Key And Containsvalue
Javascript Map How To Use The Js Map Function Array Method
How To Merge A List Of Maps By Key In Immutable Js Pluralsight
12 Map Weakmap Es6 Javascript Typescript
Javascript Tracking Key Value Pairs Using Hashmaps By
0 Response to "32 Javascript Map Use Object As Key"
Post a Comment