21 Find Object From Array Javascript
The find () method returns the value of the array element that passes a test (provided by a function). The method executes the function once for each element present in the array: If it finds an array element where the function returns a true value, find () returns the value of that array element (and does not check the remaining values) Jul 17, 2021 - Imagine we have an array of objects. How do we find an object with the specific condition?
How To Select A Field Inside An Array Inside An Object In
Find an object in an array by its values - Array.find Let's say we want to find a car that is red. We can use the function Array.find. let car = cars.find (car => car.color === "red");
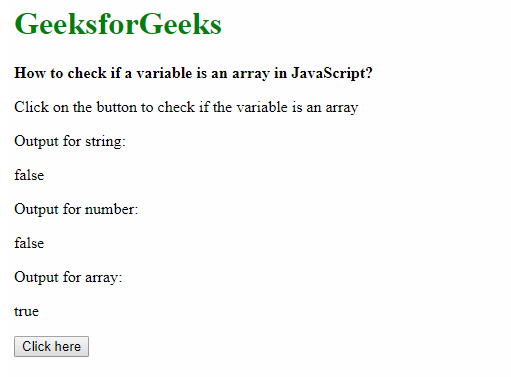
Find object from array javascript. Below examples illustrate the JavaScript Array find () method in JavaScript: Example 1: Here the arr.find () method in JavaScript returns the value of the first element in the array that satisfies the provided testing method. <script>. var array = [10, 20, 30, 40, 50]; var found = array.find (function (element) {. Sep 21, 2016 - Have you ever come across a requirement to find a particular object in a given array of objects? In this post, we will explore various ways to find a particular... The JavaScript Array.find method is a convenient way to find and return the first occurence of an element in an array, under a defined testing function. When you want a single needle from the haystack, reach for find ()! When to Use Array.find
Use filter() on arrays to go through an array and return a new array with the elements that pass the filtering rules. Oct 16, 2018 - Write cleaner and more readable code by making use of modern JavaScript array and object methods. Never touch a for/while loop again! It has a very sweet method on Arrays, .find. So you can find an element like this: array.find( {id: 75} ); You may also pass an object with more properties to it to add another "where-clause". Note that Sugarjs extends native objects, and some people consider this very evil...
How to find an object from an array of objects using the property value in JavaScript? Published January 29, 2021 . To find an object from an array of objects, we can use the filter() method available in the array and then check to see the object property's value matches the value we are looking for in the filter() method. To understand it clearly, The indexOf () method searches an array for a specified item and returns its position. The search will start at the specified position (at 0 if no start position is specified), and end the search at the end of the array. indexOf () returns -1 if the item is not found. If the item is present more than once, the indexOf method returns the ... 8/9/2011 · It has a very sweet method on Arrays, .find. So you can find an element like this: array.find( {id: 75} ); You may also pass an object with more properties to it to add another "where-clause". Note that Sugarjs extends native objects, and some people consider this very evil...
Jul 26, 2021 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. Javascript: Find Object in Array. To find Object in Array in JavaScript, use array.find () method. Javascript array find () function returns the value of the first item in the provided array that satisfies the provided testing function. The find () function returns the value of the first item in an array that pass the test (provided as a function). Get JavaScript object from array of objects by value of property [duplicate] (17 answers) ... I'd have to say though that Å ime Vidas's use of filter seems to be a much cleaner way of looping through an array to find an object (imo). - silverlight513. May 12 '16 at 15:14
Find Object In Array With Certain Property Value In JavaScript July 7, 2020 by Andreas Wik If you have an array of objects and want to extract a single object with a certain property value, e.g. id should be 12811, then find () has got you covered. The only step remaining is to find a correlation for every type of event that was recorded and see whether anything stands out. ... This kind of loop is common in classical JavaScript—going over arrays one element at a time is something that comes up a lot, and to do that you’d run a counter ... Mar 20, 2017 - You may have seen yourself in this situation when coding in JavaScript: you have an array of objects, and you need to find some specific object inside this array based on some property of the object. There are A LOT of ways of achieving this, so I decided to put some of them together in this post to
Array.prototype.findIndex () The findIndex () method returns the index of the first element in the array that satisfies the provided testing function. Otherwise, it returns -1, indicating that no element passed the test. See also the find () method, which returns the value of an array element, instead of its index. Array.find () method runs the callback function on every element present in the array and returns the first matching element. Otherwise, it returns undefined. Find the object from an array by using property 27/12/2019 · JavaScript program to find if an object is in an array or not : Finding out if an object is in an array or not is little bit tricky. indexOf doesn’t work for objects. Either you need to use one loop or you can use any other methods provided in ES6. Loop is not a good option. JavaScript provides a couple of different methods that makes it more easier.
Sep 27, 2017 - Learn how to find elements in an Array with new ES6 methods Mar 10, 2021 - In the above code, we have used the Javascript indexOf method. So, this is how to search value in an array in javascript. Find the object in the JavaScript array. JavaScript Array find () In this tutorial, we will learn about the JavaScript Array find () method with the help of examples. The find () method returns the value of the first array element that satisfies the provided test function.
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Apr 03, 2017 - Getting value out of maps that are treated like collections is always something I have to remind myself how to do properly. In this post I look at JavaScript object iteration and picking out values from a JavaScript object by property name or index. Find object by id in an array of JavaScript objects. 12. How to find value in json. 41. Which web browsers natively support Array.forEach() 2. Compare and Array to an array of objects and return a part of the matched objects. 1. Search a value inside complex object. 2.
The Array.prototype.findIndex () method returns an index in the array if an element in the array satisfies the provided testing function; otherwise, it will return -1, which indicates that no element passed the test. It executes the callback function once for every index in the array until it finds the one where callback returns true. The Object.fromEntries () method takes a list of key-value pairs and returns a new object whose properties are given by those entries. Method 2 — Using Array.find() The Array.find() method takes a callback function as parameter and executes that function once for each element present in the array, until it finds one where the function returns a true value. If the element is found it returns the value of the element, otherwise undefined is returned.
Search Objects in an Array Using the find() Method in JavaScript. The find() method is an alternate way of finding objects and their elements from an array in JavaScript. The find() is an ES6 method. This method works similar to the forEach() loop, and accessing the elements inside the object is similar to what we have seen before. The find () method returns the first value in the array, if an element in the array satisfies the provided testing function. Otherwise undefined is returned. If you want to find its index instead, use findIndex (): 2 You can use the JavaScript some() method to find out if a JavaScript array contains an object.
Aug 31, 2020 - The length property of the JavaScript object is used to find out the size of that object. This property is used with many objects like JavaScript string, JavaScript array, etc. In the case of strings, this property returns the number of characters present in the string. where a is the array of objects. What this does is map each of the objects in the array to a date created with the value of MeasureDate. This mapped array is then applied to the Math.max function to get the latest date and the result is converted to a date. Mar 27, 2018 - When trying to find items in more complex arrays, though, it’s super helpful. For example, imagine an API that returns a JSON file with an array of objects, like this:
The findIndex () method returns the index of the first array element that passes a test (provided by a function). The method executes the function once for each element present in the array: The find () method returns the value of the first element in the provided array that satisfies the provided testing function. If no values satisfy the testing function, undefined is returned. If you need the index of the found element in the array, use findIndex (). If you need to find the index of a value, use Array.prototype.indexOf (). Extract Data from Arrays and Objects in JavaScript. In this blog, we are going to explore how to extract data from arrays and objects in javaScript using destructuring. JavaScript destructuring assignment is another feature deployed as a part of the ECMAScript release in 2015.
Object.values() returns an array whose elements are the enumerable property values found on the object. The ordering of the properties is the same as that given by looping over the property values of the object manually. Using a mapping such as this would allow for much faster reconciliation (and replacement) of items from the allItems array with items in the newItems array: function replaceItemsOnId (items, replacement) { /* Create a map where the key is the id of replacement items. This map will speed up the reconciling process in the subsequent "map" stage ... Javascript queries related to "find object in array by property" js array.find es6; how to find an object in an array based on an object property
Arrays are Objects. Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays. But, JavaScript arrays are best described as arrays. Arrays use numbers to access its "elements". In this example, person [0] returns John:
Find All Objects In Array Javascript
4 Ways To Convert String To Character Array In Javascript
How To Inspect A Javascript Object
Filter Vs Find Javascript Array Methods
Data Binding Revolutions With Object Observe Html5 Rocks
Find Object Inside Array Of Object Base On Id Javascript Code
Lodash Find Object In Array Lodash Find String In Array
Get Object From Array Based On Property Using Lodash Find
Using Scope Watchcollection To Watch Functions In
Array Vs Set Vs Map Vs Object Built In Objects In Js Es6
How To Check If A Variable Is An Array In Javascript
Javascript Lesson 26 Nested Array Object In Javascript
Javascript Merge Array Of Objects By Key Es6 Reactgo
Javascript Array Distinct Ever Wanted To Get Distinct
Methods For Deep Cloning Objects In Javascript Logrocket Blog
Check If An Array Is Empty Or Not In Javascript Geeksforgeeks
How To Remove Duplicate Objects From An Array In Javascript
6 Use Case Of Spread With Array In Javascript Samanthaming Com
5 Ways To Convert Array Of Objects To Object In Javascript
0 Response to "21 Find Object From Array Javascript"
Post a Comment