27 Revealing Pattern In Javascript
Nov 06, 2015 - I first learned about the Revealing Module Pattern through Addy Osmani's book Learning JavaScript Design Patterns. Let's take a quick example to show why Revealing Module is great. Let's presume we want an Analytics object. We want to be able to use it throughout our JavaScript to make calls ... Apr 28, 2020 - Use the revealing module pattern in JavaScript to maintain private information using closures while exposing only what you need.
Modular Javascript 3 Revealing Module Pattern Javascript Tutorial
The Revealing Module Pattern in JavaScript You can use the revealing module pattern in JavaScript to maintain private information using closures while exposing only what you need.
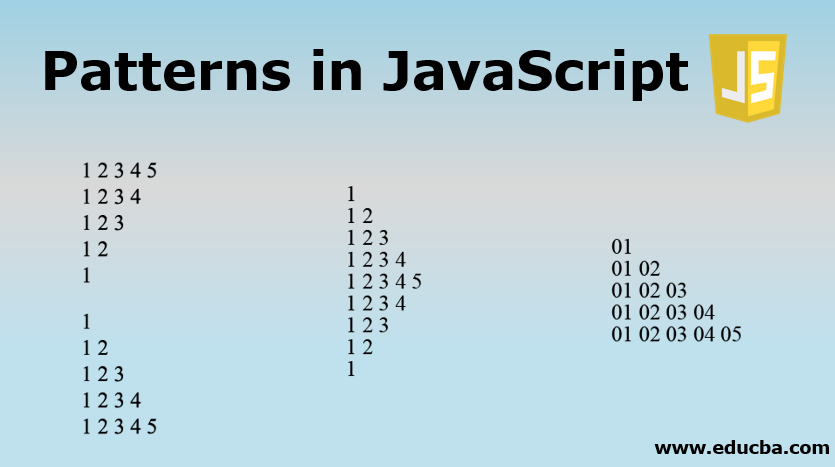
Revealing pattern in javascript. Nov 13, 2011 - I stumbled across this post: JavaScript's Revealing Module Pattern. I would like to use this in my project. Let's imagine I have a function abc and I am calling that function in my main JavaScrip... Aug 10, 2016 - The Revealing Prototype Pattern provides encapsulation with public and private members since it returns an object literal. Since we are returning an object, we will prefix the prototype object with a function. By extending our example above, we can choose what we want to expose in the current ... The Revealing Module Pattern is a variation of that, which allows us to use the same kind of syntax for all our internal constructs, only deferring to object-literal notation at the end, to return...
The Revealing Module pattern is a variant in the Module pattern. The key differences are that all members (private and public) are defined within the closure, the return value is an object literal containing no function definitions, and all references to member data are done through direct references rather than through the returned object. Jun 07, 2016 - Apologies, but something went wrong on our end · Refresh the page, check Medium’s site status, or find something interesting to read May 20, 2020 - Revealing module pattern is a design pattern, which let you organise your javascript code in modules, and gives better code structure. It gives you power to create public/private variables/methods…
The Revealing Module pattern is a variant in the Module pattern. The key differences are that all members (private and public) are defined within the closure, the return value is an object literal containing no function definitions, and all references to member data are done through direct references rather than through the returned object. Using the revealing prototype pattern in JavaScript. January 30, 2016, 6:28 pm Author: James Griffiths When developing applications with JavaScript you quickly realise the need to architect and structure your code in a way that's modular, scalable and efficient. The Revealing Module Pattern Now that we’re a little more familiar with the Module pattern, let’s take a look at a slightly improved version—Christian Heilmann’s Revealing Module pattern. The … - Selection from Learning JavaScript Design Patterns [Book]
As a reminder, the idea behind this series is to create real, practical examples of various JavaScript design patterns based on the book, "Learning JavaScript Design Patterns" by Addy Osmani. (See my review here.) In this blog entry I'll be discussing the Revealing Module pattern. Addy Osmani describes the Revealing Module pattern as: The Module Pattern is great for services and testing/TDD. There are many different variations of the module pattern so for now I will be covering the basics and the Revealing Module Pattern in ES5. Something to note, the next version of JavaScript ES6 has a new specification for asynchronous module loading. Revealing pattern in javascript. New Pluralsight Course On Mvvm Javascript Patterns And Revealing Module Pattern In Javascript Dev Community Revealing Module Pattern In Javascript Dev Community Revealing Module Pattern In Javascript Dev Community Javascript Modules A Beginner S Guide Avoiding The Global Scope With The Revealing Module Pattern ...
To be fair, the revealing module pattern is very similar to the module pattern, but it has a really nice way of exposing the api of a module to it's users or consumers. I think you'll find the revealing module pattern to be a really good balance of how to structure your JavaScript code for readability. The Revealing Prototype Pattern provides encapsulation and facilitates developers to modularize their code instead of having a mess of functions just randomly strewn about a project. As is the goal in JavaScript, this helps us to take variables and functions out of the global scope by making them part of an encapsulated container. This time, we'll be taking a look at a Revealing Module Pattern, frequently used by JavaScript developers. It's a very useful pattern that keeps our codebase clean and prevents malicious JavaScript manipulation from the browser console.
Unlike the Prototype Pattern which assigns a JavaScript object literal to the prototype, the Revealing Prototype Pattern assigns a function which is immediately invoked as with the Revealing Module Pattern: Calculator.prototype = function () { } (); The complete prototype definition for Calculator is defined using the following syntax. Using the JavaScript Revealing Module Pattern This is the 3rd post in a series on techniques, strategies and patterns for writing JavaScript code.The Prototype Pattern shown in an earlier post works well and is quite efficient, but it's not the only game in town. Revealing Module Pattern in Javascript # javascript # codenewbie # webdev. Patricia Nicole. Patricia Nicole. Patricia Nicole. Follow. playing with web fiery Location Cebu, Philippines work Junior Software Developer Joined May 9, 2021. May 31 ・Updated on Aug 1 ・2 min read [JS #2 WIL Post] ...
The Revealing Module Pattern. This popular pattern focuses on wrapping pieces of JavaScript functionalities into stand alone reusable modules. The pattern relies on the powerful JavaScript support with closures, local variable scope in functions and even the prototype construct in JavaScript. The overall generic structure of the pattern looks ... Similar to Module pattern, the Prototype pattern also has a revealing variation. The Revealing Prototype Pattern provides encapsulation with public and private members since it returns an object literal. Since we are returning an object, we will prefix the prototype object with a function. Nov 05, 2017 - The revealing module pattern is a variation of the module pattern in which we declare ALL methods inside the function private scope, and we return an object with pointers to the methods that we want to make public.
The revealing module pattern lets you define your variables and functions in conventional JavaScript way and gives you the provision to specify the public members at last. Basically, we define all our properties and methods with private module level scope and return an anonymous object with properties as pointers to the private members. Sep 22, 2019 - Using the Revealing Module Pattern in Javascript. GitHub Gist: instantly share code, notes, and snippets. Using the JavaScript Revealing Prototype Pattern. This is the 4th post in a series on techniques, strategies and patterns for writing JavaScript code. In my previous post I discussed the Revealing Module Pattern - one of my favorite JavaScript patterns. If you like the features offered by the Revealing Module Pattern but want to take ...
Jan 15, 2013 - See John Papa's article for more information on the revealing pattern · See http://www.addyosmani /resources/essentialjsdesignpatterns/book/#revealingmodulepatternjavascript for a great reference as well In contrast, with the revealing constructor pattern, we get our nice constructor invariants back. Things like: p instanceof Promise; p.constructor === Promise; Object.getPrototypeOf(p) === Promise.prototype; These are all signs that you're dealing with a well-designed "class" in JavaScript, that will behave as you expect. Apr 21, 2020 - Let’s understand a bit of the theory behind the module pattern and the purpose it serves on implementing this pattern. The Module pattern was originally defined as a way to provide both private and…
Oct 02, 2012 - This is the 3rd post in a series on techniques, strategies and patterns for writing JavaScript code.The Prototype Pattern shown in an earlier post works well and is quite efficient, but it's not the only game in town. One of my favorite overall JavaScript patterns is the Revealing Module Pattern ... Using the JavaScript Revealing Prototype Pattern. This is the 4th post in a series on techniques, strategies and patterns for writing JavaScript code. In my previous post I discussed the Revealing Module Pattern - one of my favorite JavaScript patterns. The only difference is that the revealing module pattern was engineered as a way to ensure that all methods and variables are kept private until they are explicitly exposed; usually through an object literal returned by the closure from which it’s defined. Personally, I like this approach for vanilla JavaScript ...
Revealing Prototype Pattern. Similar to Module pattern, the Prototype pattern also has a revealing variation. The Revealing Prototype Pattern provides encapsulation with public and private members since it returns an object literal. Since we are returning an object, we will prefix the prototype object with a function. By extending our example ... The revealing module pattern allows us to create private variables in a Javascript Module.Here's a writeup on this pattern:http://addyosmani /resources/es... An example of a developer that did this is in recent years is Christian Heilmann, who took the existing Module pattern and made some fundamentally useful changes to it to create the Revealing Module pattern (this is one of the patterns covered later in this book).
The benefits of using the revealing module pattern in JavaScript. Updated on December 10, 2020 Note: this post discusses the "revealing module pattern" that was commonly used in JavaScript prior to widespread adoption of "ES modules" (see also this in-depth post on ES modules). The revealing module pattern provides JavaScript developers with a robust, scalable structure for architecting their classes, clean separation between private and public members, the ability to access global objects locally by reference, is highly readable and self-invoking making this a highly desirable solution for code development ...
Revealing Module Pattern In Javascript By Sander De Bruijn
Revealing Module Pattern In Js Let S Understand Bit Of The
Javascript Modules And Patterns Private Fields Module
Revealing Module Pattern In Js Let S Understand Bit Of The
According To Kiss Javascript Patterns Revealing Module Pattern
Dan Wahlin Revealing Module Pattern Techniques
Javascript Modules A Shot To Figure Out How The Most By
Revealing The Spatial Shifting Pattern Of Covid 19 Pandemic
Patterns For Large Scale Javascript Application Architecture
Javascript Module Systems Even An Old Topic As It Is Today
Javascript Revealing Module Pattern
Using The Revealing Prototype Pattern In Javascript
Namespace And Revealing Module Pattern Trong Javascript
Javascript Frameworks Mindmeister Mind Map
Modular Javascript 3 Revealing Module Pattern Javascript
The Revealing Module Is An Anti Pattern
Javascript Design Patterns Digitalocean
Javascript Revealing Prototype Pattern Vegibit
The Revealing Module Pattern Learning Javascript Design
Prototype Design Pattern In Javascript Digitalocean
Javascript Revealing Module Pattern Pluralsight
Javascript Module Pattern Basics
Patterns In Javascript 3 Amazing Types Of Patterns In
Using The Revealing Module Pattern In Javascript Github
0 Response to "27 Revealing Pattern In Javascript"
Post a Comment