23 How To Write Synchronous Code In Javascript
The asynchronous code will be written in three ways: callbacks, promises, and with the async / await keywords. Note: As of this writing, asynchronous programming is no longer done using only callbacks, but learning this obsolete method can provide great context as to why the JavaScript community now uses promises. Jan 22, 2015 - First, this is a very specific case of doing it the wrong way on-purpose to retrofit an asynchronous call into a very synchronous codebase that is many thousands of lines long and time doesn't curr...
Faster Async Functions And Promises V8
How can I write synchronous JavaScript code for PhantomJS? The PhantomJS API uses callbacks to work. It doesn't emit promises that you can use. Of course you could write a wrapper around PhantomJS to support a promise-like API, but that would be overkill.
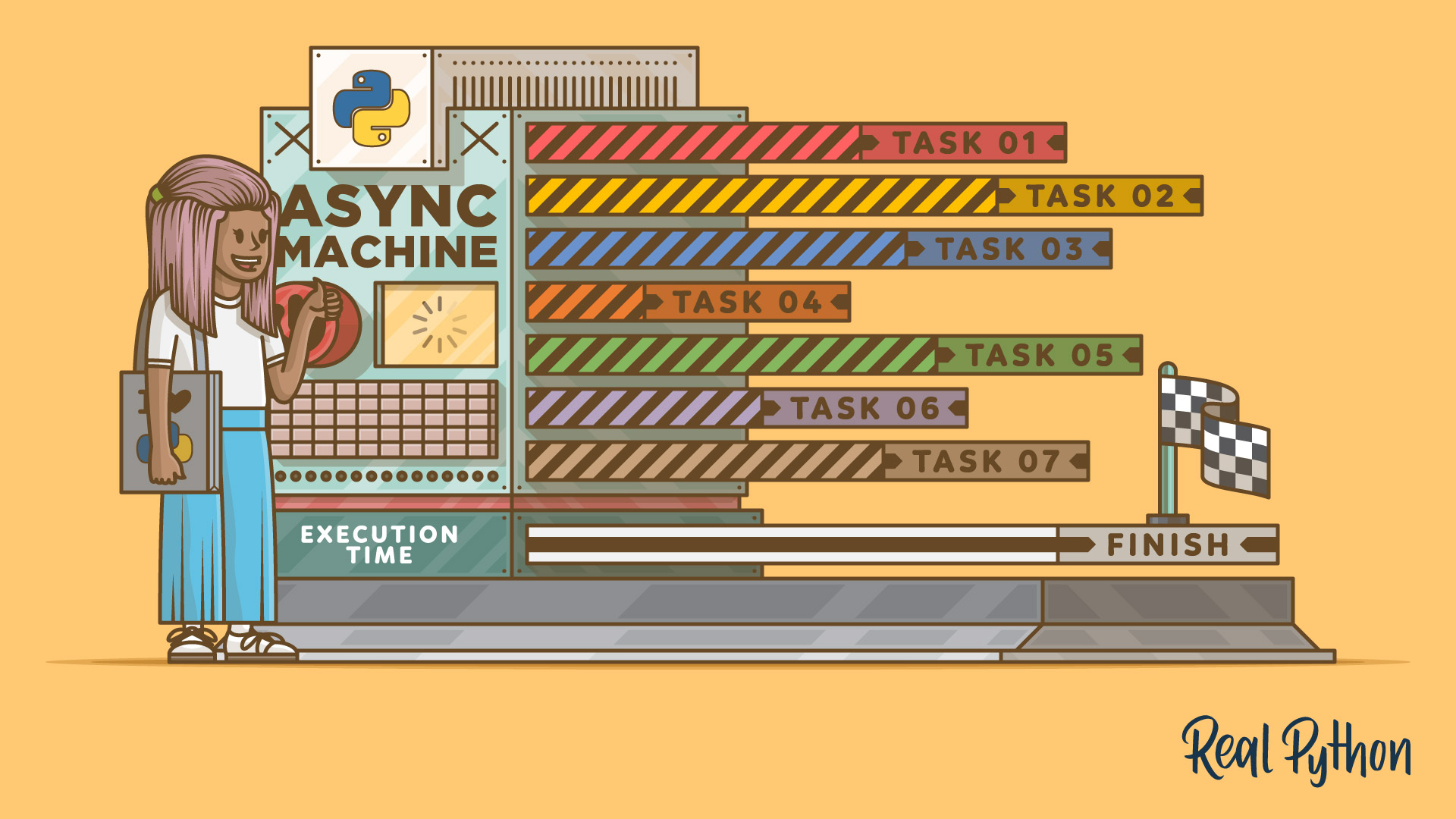
How to write synchronous code in javascript. Jun 28, 2019 - We, the people, like structure. We like categories and descriptions and putting everything we know into tidy little boxes. This is why I found JavaScript so very confusing at first. Is it a scripting… Examples of Synchronous and Asynchronous Code 5:20 with Guil Hernandez This video shows you examples of synchronous and asynchronous JavaScript in the browser. Jan 20, 2020 - Learn Asynchronous calls to Synchronous concepts in JavaScript and convert your Asynchronous calls to Synchronous. You can do it on your own by reading the article until the end.
Well, synchronous code in JavaScript will start from the top of a file and execute all the way to the bottom, each line in order until it gets the bottom and it will stop. For example, if a function takes a while to execute or has to wait on something, it freezes everything up in the meanwhile because synchronous code in JavaScript can only do ... Dec 10, 2017 - I am trying to create a program that analyses some data. The data I am collection form the internet with node.js. I'm having a very hard time with the fact that the code is asynchronous, and I'm ge... We discussed synchronous and asynchronous paradigms, and about the importance of writing non-blocking code for your web application. We then took a sneak peek at how asynchronous code works under the hood in Javascript. We also looked at the different APIs that JS has provided to write asynchronous code - callbacks, promises and async/await.
This code snippet demonstrates how to write a sleep() function: View the raw code as a GitHub gist This JavaScript sleep() function works exactly as you might expect, because await causes the synchronous execution of the code to pause until the Promise is resolved. The asynchronous function can be written in Node.js using 'async' preceding the function name. The asynchronous function returns implicit Promise as a result. The async function helps to write promise-based code asynchronously via the event-loop. Async functions will always return a value. Await function can be used inside the asynchronous ... Sep 08, 2015 - I have been under the impression for that JavaScript was always asynchronous. However, I have learned that there are situations where it is not (ie DOM manipulations). Is there a good reference
Synchronous JavaScript Synchronous code in JavaScript will start from the top of a file and execute all the way to the bottom, each line in order until it gets the bottom and it will stop. The only difference is that the syntax of async functions is more similar to synchronous functions than promises, so if you're more accustomed to synchronous code, async functions are the way to ... Introducing asynchronous JavaScript. In this article we briefly recap the problems associated with synchronous JavaScript, and take a first look at some of the different asynchronous techniques you'll encounter, showing how they can help us solve such problems. Basic computer literacy, a reasonable understanding of JavaScript fundamentals. To ...
Oct 09, 2017 - Synchronous operations in JavaScript entails having each step of an operation waits for the previous step to execute completely. This means no matter how long a previous process takes, subsquent process won't kick off until the former is completed. Asynchronous operations, on the other hand, defe Feb 25, 2021 - Since the ECMAScript 2017 (ES8) release and its support adoption by default on Node.js 7.6, you no longer have excuses for not being using one of the hottest ES8 features, which is the async/await… Synchronous Callback. The code execution will block or wait for the event before continuing. Until your event returns a response, your program will not execute any further. So Basically, the callback performs all its work before returning to the call statement. The problem with synchronous callbacks are that they appear to lag.
What is asynchronous code? By design, JavaScript is a synchronous programming language. This means that when code is executed, JavaScript starts at the top of the file and runs through code line by line, until it is done. The result of this design decision is that only one thing can happen at any one time. Synchronous Code Example. To test a synchronous system, write this code in JavaScript: console.log(" I "); console.log(" eat "); console.log(" Ice Cream "); Here's the result in the console: 👇. Asynchronous code example. Let's say it takes two seconds to eat some ice cream. Now, let's test out an asynchronous system. Write the below code in ... Synchronous Execution And The Observer Pattern # As mentioned in the introduction, JavaScript runs the code you write line by line, most of the time. Even in its first years, the language had exceptions to this rule, though they were a few and you might know them already: HTTP Requests, DOM events and time intervals.
This flavor of Javascript allows us to write asynchronous code as if it is synchronous, but in reality of course it is still asynchronous. With this flavor of Javascript you can no longer do two asynchronous tasks at the same time, everything will be executed synchronously. For example if you want to make a request using fetch, but are only ... making asynchronous JS code synchronous. GitHub Gist: instantly share code, notes, and snippets. Write clean, readable synchronous JavaScript code and output to callback-based async code automatically. SyncScript is a compiler that generates readable, compatible JS callback-based code from easy to read synchronous code. The compiled code runs everywhere and on every browser. No libraries or dependencies, just clean code.
More recent additions to the JavaScript language are async functions and the await keyword, added in ECMAScript 2017. These features basically act as syntactic sugar on top of promises, making asynchronous code easier to write and to read afterwards. They make async code look more like old-school synchronous code, so they're well worth learning. This article gives you what you need to know. Code language: CSS (css) ES2017 introduced the async/await keywords that build on top of promises, allowing you to write asynchronous code that looks more like synchronous code and more readable. Technically speaking, the async / await is syntactic sugar for promises.. If a function returns a Promise, you can place the await keyword in front of the function call, like this: Generators are a useful tool for handling asynchrony in your JavaScript applications. They were added to JavaScript with ES2015. When applied correctly they allow us to write asynchronous code that looks synchronous. They also turn one of JavaScripts core paradigms on its head. Contrary to normal functions, generator functions can be paused and resumed at any arbitrary point in time with the ...
JavaScript is single-threaded and synchronous language. The code is executed in order one at a time. But Javascript may appear to be asynchronous in some situations. Dec 19, 2019 - Engineer | Lover of dogs, books, and learning | Dos XX can be most interesting man in the world, I'm happy being the luckiest. | I write about what I learn @ code-comments ... I often find myself looking up patterns for converting synchronous code into async variants in Javascript. Jul 27, 2013 - An introduction to asynchronous JavaScript, part of a series on JavaScript for Beginners.
Sep 25, 2019 - JavaScript is synchronous. This means that it will execute your code block by order after hoisting. Before the code executes, var and function declarations are “hoisted” to the top of their scope. The second way to write asynchronous JavaScript code are Promises. Promises are a newer feature, introduced to JavaScript with the ES6 specification. They provide a very easy way to deal with asynchronous JavaScript code. This one reason why many JavaScript developers, if not almost all, started using them instead of callbacks. ES7's Async + Await allows the developer to write more concise asynchronous code. It is just a piece of syntactical sugar for a developer's JavaScript. An added bit of coding utility if you will. When implemented correctly, it can do the following: Make code more readable. Better describe when a asynchronous action begins + ends.
Mar 30, 2017 - JavaScript’s first-class treatment of functions is what primarily allows us to create synchronization patterns for asynchronous tasks. Synchronization is necessary because often times the result of an operation depends on the result of an asynchronous task. We write the code in a way that ... Synchronous JavaScript. Synchronous JavaScript as the name implies, means in a sequence, or an order. Here, every function or program is done in a sequence, each waiting for the first function to execute before it executes the next, synchronous code goes from top to bottom. To better understand synchronous JavaScript, let's look at the code ... Dec 02, 2018 - We, the people, like structure. We like categories and descriptions and putting everything we know into tidy little boxes. This is why I found JavaScript so confusing at first. Is it a scripting or a…
The behavior of JavaScript is asynchronous, so there is a concept of promises to handle such asynchronous behavior. Because of this asynchronous behavior, it continues its work and does not wait for anything during execution. Async/await functions help us to write the code in a synchronous manner. How to use sleep function in JavaScript? Async/await is a new way of writing asynchronous code in JavaScript. Before this, we used callbacks and promises for asynchronous code. It allows us to write a synchronous-looking code that is easier to maintain and understand. Async/await is non-blocking, built on top of promises and can't be used in plain callbacks. Javascript is synchronous "by default". Meaning, the next line of code cannot run until the current one has finished. The next function cannot run until the current one has completed. But blocks of code run in parallel when it comes to asynchronous; Asynchronous functions run independently.
Synchronous JavaScript: As the name suggests synchronous means to be in a sequence, i.e. every statement of the code gets executed one by one. So, basically a statement has to wait for the earlier statement to get executed. Let us understand this with the help of an example. Write "Synchronous" Node.js Code with ES6 Generators Nov 21, 2015. One of the most amazing things about ES6 (EcmaScript 2015) is the introduction of generator functions.These are special functions that may be paused at any time as they wait for an async operation to complete by utilizing the yield expression, and are resumed as soon as that operation completes. Tip: To check if a JavaScript file is part of JavaScript project, just open the file in VS Code and run the JavaScript: Go to Project Configuration command. This command opens the jsconfig.json that references the JavaScript file. A notification is shown if the file is not part of any jsconfig.json project.
Nov 08, 2018 - Synchronous and asynchronous are confusing concepts in JavaScript, especially for beginners. Two or more things are synchronous when they happen at the Jun 11, 2019 - JavaScript is synchronous by default and is single threaded. This means that code cannot create new threads and it will execute your code… Synchronous and asynchronous execution in Javascript By default, the code execution in JavaScript is synchronous. This means that each operation blocks the following until it's done. Therefore, operations which typically take longer (e.g. network requests) will block any further execution.
Javascript Promises Tutorial How To Write Asynchronous Code
Java14 Synchronous Httpclient Example Overview And Simple
An Alternative Way To Use Async Await Without Try Catch
How Javascript Works Exceptions Best Practices For
Callback Vs Promises Vs Async Await Loginradius Engineering
Deeply Understanding Javascript Async And Await With Examples
Asynchronous Adventures With Node Js By Priyesh Saraswat
Cleaner Code With Async Await Tutorial Khalil Stemmler
Javascript Goes Asynchronous And It S Awesome Sitepoint
How To Deal With Asynchronous Code In Javascript By Tahere
Javascript Asynchronous Tutorial
Callback Hell Bmc Software Blogs
The Difference Between Asynchronous And Multi Threading
Wtf Is Synchronous And Asynchronous By Skrew Everything
Synchronous Code Vs Asynchronous Code In Node Js
Combining Asynchronous And Synchronous Programming Software
Javascript Async Await Tutorial Learn Callbacks Promises
Getting Started With Async Features In Python Real Python
Using Javascript Promises In Keylines Part 1 Cambridge
Javascript Engines How Do They Even Work From Call Stack To
Asynchronous Javascript From Callback Hell To Async And
Write Asynchronous Code In A Breeze With Async And Await
0 Response to "23 How To Write Synchronous Code In Javascript"
Post a Comment