33 Add Remove Value In Array Javascript
The builtin pop and shift methods remove from either end. If you want to remove an element in the middle of an array you can use splice thus function removeElementAtIndex (arr, i) { Array.prototype.splice.call (arr, i, 1); } How to tell whether an element is in an array depends on what you mean by "in". Use Array.filter () to Remove a Specific Element From JavaScript Array Use the Underscore.js Library to Remove a Specific Element From JavaScript Array Use the Lodash Library to Remove a Specific Element From JavaScript Array This tutorial teaches how to remove a specific element from an array in JavaScript.
Numpy Remove Specific Elements In A Numpy Array W3resource
Now we will look at a couple of ways to remove a specific element from an array. First, let's look at Array.splice used in combination with Array.indexOf. Array.splice allows us to remove elements from an Array starting from a specific index. We can provide a second argument to specify how many elements to delete.
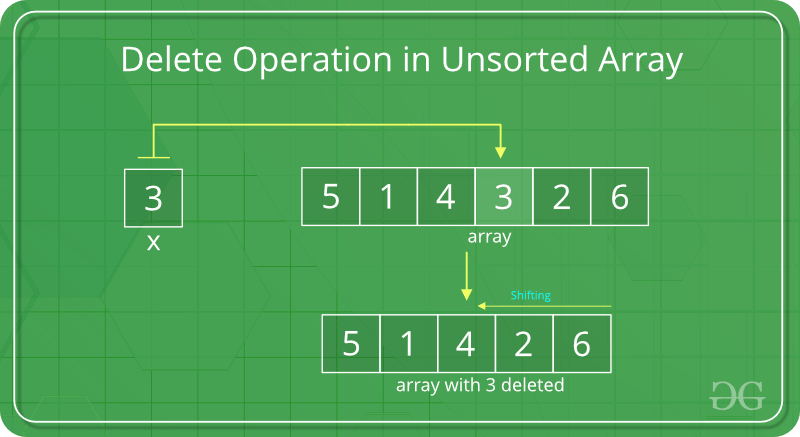
Add remove value in array javascript. Definition and Usage The splice () method adds and/or removes array elements. splice () overwrites the original array. The row parameter then contains the entire friend Object. We want just the value of the state key. The new array will be an array of all states, including duplicates, one for each friend Object in the Array. 2. Add a console.log and type node index in the Terminal window after saving to run the code. // Get an Array containing just the States ... JavaScript Array pop () Method. This method deletes the last element of an array and returns the element. Syntax: array.pop () Return value: It returns the removed array item. An array item can be a string, a number, an array, a boolean, or any other object types that are applicable in an array.
1) Remove duplicates from an array using a Set. A Set is a collection of unique values. To remove duplicates from an array: First, convert an array of duplicates to a Set. The new Set will implicitly remove duplicate elements. Then, convert the set back to an array. The following example uses a Set to remove duplicates from an array: JavaScript offers several ways to add, remove, and replace items in an array - but some of these ways mutate the array, and others are non-mutating; they produce a new array.. Below, I have outlined how to accomplish these three tasks using both mutating and non-mutating practices. This post concludes by showing how to iterate over an array and transform each item using the non-mutating ... Whatever you do, don't use delete to remove an item from an array. JavaScript language specifies that arrays are sparse, i.e., they can have holes in them. Using delete creates these kinds of holes. It removes an item from the array, but it doesn't update the length property. This leaves the array in a funny state that is best avoided.
Remove an Item From an Array by Value Using the splice () Function in JavaScript To remove an item from an array by value, we can use the splice () function in JavaScript. The splice () function adds or removes an item from an array using the index. 25/7/2018 · How to Remove an Element from an Array in JavaScript JavaScript suggests several methods to remove elements from existing Array . You can delete items from the end of an array using pop() , from the beginning using shift() , or from the middle using splice() functions. In the above program, an item is removed from an array using a for loop. Here, The for loop is used to loop through all the elements of an array. While iterating through the elements of the array, if the item to remove does not match with the array element, that element is pushed to newArray. The push() method adds the element to newArray.
It's important to note that splicing an array is an incredibly slow operation and is very difficult or impossible for engines to optimize. If you have performance sensitive uses of collections of items that need to be mutated at arbitrary points then you'll likely want to look into alternative data structure implementations. Javascript array splice () is an inbuilt method that changes the items of an array by removing or replacing the existing elements and/or adding new items. To remove the first object from the array or last object from the array, then use the splice () method. Let's remove the first object from the array. See the following code. Deleting elements using JavaScript Array’s splice() method To delete elements in an array, you pass two arguments into the splice() method as follows: Array .splice(position,num);
The JavaScript array has a variety of ways you can delete array values. There are different methods and techniques you can use to remove elements from JavaScript arrays: pop — Removes from the End... To remove a particular element from an array in JavaScript we'll want to first find the location of the element and then remove it. Finding the location by value can be done with the indexOf() method, which returns the index for the first occurrence of the given value, or -1 if it is not in the array. When you work with arrays, it is easy to remove elements and add new elements. This is what popping and pushing is: ... Since JavaScript arrays are objects, elements can be deleted by using the JavaScript operator delete: ... There are no built-in functions for finding the highest or lowest value in a JavaScript array.
JavaScript provides us an alternate array method called lastIndexOf (). As the name suggests, it returns the position of the last occurrence of the items in an array. The lastIndexOf () starts searching the array from the end and stops at the beginning of the array. You can also specify a second parameter to exclude items at the end. 8 Ways to Remove Duplicate Array Values in JavaScript. 1. De-Dupe Arrays with Set Objects. Set objects objects are my go-to when de-duplicating arrays. Try out this example in your browser console, spoiler - Set objects work great for primitive types, but may not support use cases requiring the de-duplication of objects in an array. An integer indicating the number of elements in the array to remove from start. If deleteCount is omitted, or if its value is equal to or larger than array.length - start (that is, if it is equal to or greater than the number of elements left in the array, starting at start ), then all the elements from start to the end of the array will be ...
There are many cases when it needs to remove some unused values from the Array which no longer needed within the program. Inbuilt functions are available in JavaScript to do this. Some functions auto-arrange the Array index after deletion and some just delete the value and not rearrange the index. For that purpose, I decided to write this article about some of the best methods we can use to add and remove elements in arrays in Javascript. In this article we'll be covering push , pop , shift , unshift , concat , splice , slice and also on how to use the ES6 spread operator for javascript arrays. Instead of a delete method, the JavaScript array has a variety of ways you can clean array values. You can remove elements from the end of an array using pop, from the beginning using shift, or from the middle using splice. The JavaScript Array filter method to create a new array with desired items, a more advanced way to remove unwanted elements.
filter() calls a provided callback function once for each element in an array, and constructs a new array of all the values for which callback returns a value that coerces to true. callback is invoked only for indexes of the array which have assigned values; it is not invoked for indexes which have been deleted or which have never been assigned ... 3/6/2020 · In JavaScript, the Array.splice () method can be used to add, remove, and replace elements from an array. This method modifies the contents of the original array by removing or replacing existing elements and/or adding new elements in place. Array.splice () returns the removed elements (if any) as an array. 5 Way to Append Item to Array in JavaScript. Here are 5 ways to add an item to the end of an array. push, splice, and length will mutate the original array. Whereas concat and spread will not and will instead return a new array. Which is the best depends on your use case 👍.
push () ¶. The push () method is an in-built JavaScript method that is used to add a number, string, object, array, or any value to the Array. You can use the push () function that adds new items to the end of an array and returns the new length. The new item (s) will be added only at the end of the Array. You can also add multiple elements to ... Let's know first that what's Javascript filter method. Javascipt filter is a array method used to filter an array based on condition and it return a new array. Array.filter (function (elem, index, arr) { }) Array.filter ( (elem, index, arr)=> { }) We use a filter method to remove duplicate values from an array. You can also use $.grep () function to remove value from array. Of course, this function is depended on JQuery. So before use this function you need to add JQuery lib into your project. I always use this function to get a difference between the two arrays just like array_diff () a PHP function.
In the above example, we accessed the array at position 1 of the nestedArray variable, then the item at position 0 in the inner array. Adding an Item to an Array. In our seaCreatures variable we had five items, which consisted of the indices from 0 to 4. If we want to add a new item to the array, we can assign a value to the next index.
Dynamically Add And Remove Html Elements Using Jquery
Some Powerful Things You Can Do With The Array Splice Method
Adding And Removing Items From A Powershell Array Jonathan
How To Remove Duplicate Objects From An Array In Javascript
Add Remove Multiple Dynamic Input Fields Template Driven
9 Ways To Remove Elements From A Javascript Array
Removing Empty Or Undefined Elements From An Array Stack
Removing Elements From An Array In Javascript Edureka
How To Delete An Item From An Array In React Vegibit
How Can I Remove A Specific Item From An Array Stack Overflow
Python Arrays Create Update Remove Index And Slice
Remove Object From An Array Of Objects In Javascript
Remove Object From An Array Of Objects In Javascript
Search Insert And Delete In An Unsorted Array Geeksforgeeks
Delete The Array Elements In Javascript Delete Vs Splice
C Program To Delete An Element In An Array
Javascript Array Splice Delete Insert And Replace
Java67 How To Remove A Number From An Integer Array In Java
Manipulating Javascript Arrays Removing Keys By Adrian
Hacks For Creating Javascript Arrays
Pop Push Shift And Unshift Array Methods In Javascript
Python List Append How To Add An Element To An Array
Typescript Remove Item From Array Learn The Examples And
How To Remove A Property From A Javascript Object
Remove Element From Array Using Slice Stack Overflow
Program To Remove Duplicate Elements In An Array C Programs
Javascript Array Splice Delete Insert And Replace
The Firebase Blog Better Arrays In Cloud Firestore
Javascript Unique Array How To Use Array Filter Method
Some Powerful Things You Can Do With The Array Splice Method
0 Response to "33 Add Remove Value In Array Javascript"
Post a Comment