33 Javascript Regular Expression Match
Javascript RegExp¶ Regex or Regular expressions are patterns used for matching the character combinations in strings. Regex are objects in JavaScript. Patterns are used with RegEx exec and test methods, and the match, replace, search, and split methods of String. The test() method executes the search for a match between a regex and a specified ... I want to match a portion of a string using a regular expression and then access that parenthesized substring: var myString = "something format_abc"; // I want "abc" var arr = /(?:^|\s)
A Guide To Javascript Regular Expressions
JavaScript regular expressions automatically use an enhanced regex engine, which provides improved performance and supports all behaviors of standard regular expressions as defined by Mozilla JavaScript. The enhanced regex engine supports using Java syntax in regular expressions. The enhanced regex
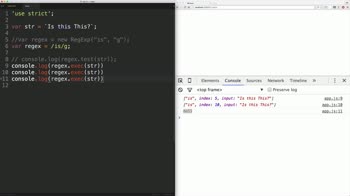
Javascript regular expression match. Description In JavaScript, match () is a string method that is used to find matches based on regular expression matching. Because the match () method is a method of the String object, it must be invoked through a particular instance of the String class. The RegExp object has a number of top level methods, and to test whether a regular expression matches a specific string in Javascript, you can use RegExp.test (). To actually extract information from a string using a regular expression, you can instead use the RegExp.exec () call which provides more information about the match in its result. In the following example, match () is used to find ' Chapter ' followed by 1 or more numeric characters followed by a decimal point and numeric character 0 or more times. The regular expression includes the i flag so that upper/lower case differences will be ignored.
The match () method searches a string for a match against a regular expression, and returns the matches, as an Array object. Read more about regular expressions in our RegExp Tutorial and our RegExp Object Reference. Note: If the regular expression does not include the g modifier (to perform a global search), the match () method will return only ... 22/3/2011 · Regular Expressions. The following is how regular expressions can be used. [abc] – find any character in the brackets a, b or c. [a-z] – find the range of characters within brackets i.e. a to z lowercase. Can also be used with numbers [0-9] [^xyz] – find any character other than the ones specified in the brackets i.e. x,y and z. Definition In JavaScript, a regular expression is simply a type of object that is used to match character combinations in strings. Creating Regex in JS There are two ways to create a regular expression:
Regular expressions allow you to check a string of characters like an e-mail address or password for patterns, to see so if they match the pattern defined by that regular expression and produce actionable information. Creating a Regular Expression There are two ways to create a regular expression in Javascript. Sep 05, 2019 - Parameters: Here the parameter is “regExp” i.e, regular expression which will compare with the given string. Return Value: It will return an array that contain the matches one item for each match or if the match will not found then it will return Null. JavaScript code to show the working ... JavaScript regular expression In JavaScript, we build regular expressions either with slashes // or RegExp object. A pattern is a regular expression that defines the text we are searching for or manipulating. It consists of text literals and metacharacters.
The rule of thumb is that simple regular expressions are simple to read and write, while complex regular expressions can quickly turn into a mess if you don't deeply grasp the basics. How does a Regular Expression look like. In JavaScript, a regular expression is an object, which can be defined in two ways. Jun 13, 2021 - Using a function gives us the ultimate ... the match, has access to outer variables and can do everything. ... If the first argument is a string, it replaces all occurences of the string, while replace replaces only the first occurence. If the first argument is a regular expression without the ... In JavaScript, you can use regular expressions with RegExp () methods: test () and exec (). There are also some string methods that allow you to pass RegEx as its parameter. They are: match (), replace (), search (), and split (). Executes a search for a match in a string and returns an array of information.
The following regular expression flags are available in JavaScript (tbl. 21 provides a compact overview): /g (.global): fundamentally changes how the following methods work. ... How, is explained in §43.6.1 “The flags /g and /y”. In a nutshell, without /g, the methods only consider the first match ... RegExr is an online tool to learn, build, & test Regular Expressions (RegEx / RegExp). Supports JavaScript & PHP/PCRE RegEx. Results update in real-time as you type. Roll over a match or expression for details. 14/8/2013 · The alternation | will first try to match the left subexpression, and when that fails it tries the right one. When the left one includes the right one, it will never match the right one. In your case it will stop at only three groups even if there are more. Try to …
Regular expression tester with syntax highlighting, explanation, cheat sheet for PHP/PCRE, Python, GO, JavaScript, Java. Features a regex quiz & library. regex101: build, test, and debug regex As a quick example, suppose we need to make the expression /Hello/ search case-insensitively, that is, match either 'hello', 'HELLO', 'HeLLO', 'heLlo' and so on.. To do so we'll need to add the flag i in the regular expression as shown below: /Hello/i Similarly, in addition to insensitive searching, if we also wanted to make the search extend to match all occurences of the pattern we would ... 1 week ago - The "g" after the regular expression is an option or flag that performs a global search, looking in the whole string and returning all matches. It is explained in detail below in Advanced Searching With Flags. Why isn't this built into JavaScript? There is a proposal to add such a function ...
Regular expressions are used to perform pattern-matching and "search-and-replace" functions on text. ... For a tutorial about Regular Expressions, read our JavaScript RegExp Tutorial. A JavaScript Regular Expression (or Regex) is a sequence of characters that we can utilize to work effectively with strings. Using this syntax, we can: Dating back to the 1950s, Regular Expressions were formalized as a concept for pattern searching in string processing algorithms. JavaScript's String type has 4 built-in methods: search(), replace(), split(), and match(), all of which accept regular expressions as an argument to help them perform their respective operations. When searching in a given string, you can specify the pattern you're looking for.
Jul 01, 2020 - In as few words as possible, this post will teach you how the JavaScript match method works to retrieve regular expression match(es) from within a string. The string.match () is an inbuilt function in JavaScript used to search a string for a match against any regular expression. If the match is found, then this will return the match as an array. Parameters: Here the parameter is "regExp" (i.e. regular expression) which will compare with the given string. 4/1/2021 · Regular expressions, abbreviated as regex, or sometimes regexp, are one of those concepts that you probably know is really powerful and useful. But they can be daunting, especially for beginning programmers. It doesn't have to be this way. JavaScript includes several helpful methods that make using regular expressions much more
May 14, 2021 - In this article, I’ll explain what RegExp in JavaScript is, its importance, special characters, how to create and write them efficiently, main use-cases, and its different properties and methods. ... Regular expressions are a sequence of characters that are used for matching character ... Regular Expression Tester with highlighting for Javascript and PCRE. Quickly test and debug your regex. Toggle navigation. RegEx Testing From Dan's Tools. ... Top Regular Expressions. Match string not containing string Check if a string only contains numbers ... match ab or cd ... In this tutorial you will learn how regular expressions work, as well as how to use them to perform pattern matching in an efficient way in JavaScript. ... Regular Expressions, commonly known as "regex" or "RegExp", are a specially formatted text strings used to find patterns in text.
Those circumstances are that the regular expression must have the global (g) or sticky (y) option enabled, and the match must happen through the exec method. Again, a less confusing solution would have been to just allow an extra argument to be passed to exec, but confusion is an essential feature of JavaScript... Regular expressions are patterns used to match the character combinations in strings. In JavaScript, regular expressions are also objects. Here, our pattern is a bro and using match () function, and we are checking that pattern against our input string. Global search in regex May 02, 2020 - Use the JavaScript String search() to find the index of the first match based on a regular expression in a string. ... Primitive vs. Reference Values ... The JavaScript Tutorial website helps you learn JavaScript programming from scratch quickly and effectively.
It was added to JavaScript language long after match, as its "new and improved version". Just like match, it looks for matches, but there are 3 differences: It returns not an array, but an iterable object. When the flag g is present, it returns every match as an array with groups. Dec 11, 2017 - I want to use JavaScript (can be with jQuery) to do some client-side validation to check whether a string matches the regex: ^([a-z0-9]{5,})$ Ideally it would be an expression that returned true or Aug 02, 2019 - This is especially important when you need to process a large string and the chance of undesired matches is high. Fortunately, most regular expression flavors provide the lookbehind and lookahead assertions for this purpose. Prior to ES2018, only lookahead assertions were available in JavaScript.
The regular expression property .lastIndex # The regular expression property .lastIndex only has an effect if at least one of the flags /g and /y is used. For regular expression operations that are affected by it, it controls where matching starts..lastIndex and /g # In JavaScript, Regular Expressions also known as regex are patterns used to match, replace, compare character combinations in strings. When we search for some specific data in a text, we can use regular expression to be used as search pattern to describe what you are searching for. 28/3/2012 · var str = "SUM([A2:A10],[B2:B10],[C2:C10],[D2:D10])"; var matches = str.match(/\[[A-Z0-9:]+\]/g); alert(matches); Note, you use the g flag on the regex to get all the matches. You can see it working here: http://jsfiddle /jfriend00/aTrLU/
Regular expressions are an ancient and powerful technique for finding patterns in text. They have been tested by the ages and been found to be so useful that practically every computer language in use today provides support for using regular expressions. Of course this includes the Core JavaScript model. In Core JavaScript, regular expressions are a built-in data type and are employed in ... Sep 02, 2020 - In JavaScript, regular expressions are objects. JavaScript provides the built-in RegExp type that allows you to work with regular expressions effectively. Regular expressions are useful for searching and replacing strings that match a pattern. They have many useful applications. In JavaScript, regular expressions are often used with the two string methods: search () and replace (). The search () method uses an expression to search for a match, and returns the position of the match. The replace () method returns a modified string where the pattern is replaced. Using String search () With a String
Regext To Match Any Substring Longer Than 2 Characters
The Most Surprising Behavior Of Javascript Regular Expression
Making Sense Of Regular Expressions By John Agens Medium
Using Regular Expression In Javascript Codeproject
Javascript Regex Match Check If String Matches Regex
Javascript Regular Expression How To Create Amp Write Them In
Regular Expressions In Javascript Guide To Regular Expressions
How Javascript Works Regular Expressions Regexp By
Javascript And Al The Regular Expressions Example
Javascript Regex Match Example How To Use Js Replace On A
Js Regex Get Word After Certain Characters Stack Overflow
Understanding Regular Expression Matching With Test Match
Javascript Regular Expression How To Create Amp Write Them In
Regular Expression In Javascript
A Practical Guide To Regular Expressions Regex In
Javascript String Match Regex Boolean Code Example
Regular Expression Tutorial Learn How To Use Regular
Email Address Regular Expression That 99 99 Works
Regexr Learn Build Amp Test Regex
A Regular Expression Tester For Nginx And Nginx Plus Nginx
How Javascript Works Regular Expressions Regexp By
Basic Regex In Javascript For Beginners Dev Community
Introduction To The Use Of 10 Regular Expressions In
Get Started With Regular Expressions In Javascript
Javascript Regular Expression To Match A Comma Delimited List
Javascript Regular Expression How To Create Amp Write Them In
Xregexp Extended Javascript Regular Expression Library
Regex In Javascript Egghead Io
Regular Expressions Eloquent Javascript
0 Response to "33 Javascript Regular Expression Match"
Post a Comment