27 Add A New Property To An Object Javascript
1. Using bracket syntax to add new property (Old Way but still powerful) So, this is the way where we can add a new key to the existing object like the way we used to access the array. const key = "Codez" const obj = { 'hello': 'guys', } obj[key] = "Up" console.log(obj) This is a tutorial on how to add new properties to a JavaScript object. In this guide, I will also show you how to dynamically set a new property. Take a look at the following object: //Basic JavaScript object that represents a person. var person = { name: 'Michael Jordan', dob: '1963-02-17', height: 1.98 }
Javascript Engine Fundamentals Shapes And Inline Caches
Date objects, Array objects, and Person objects inherit from Object.prototype. Adding Properties and Methods to Objects Sometimes you want to add new properties (or methods) to all existing objects of a given type.
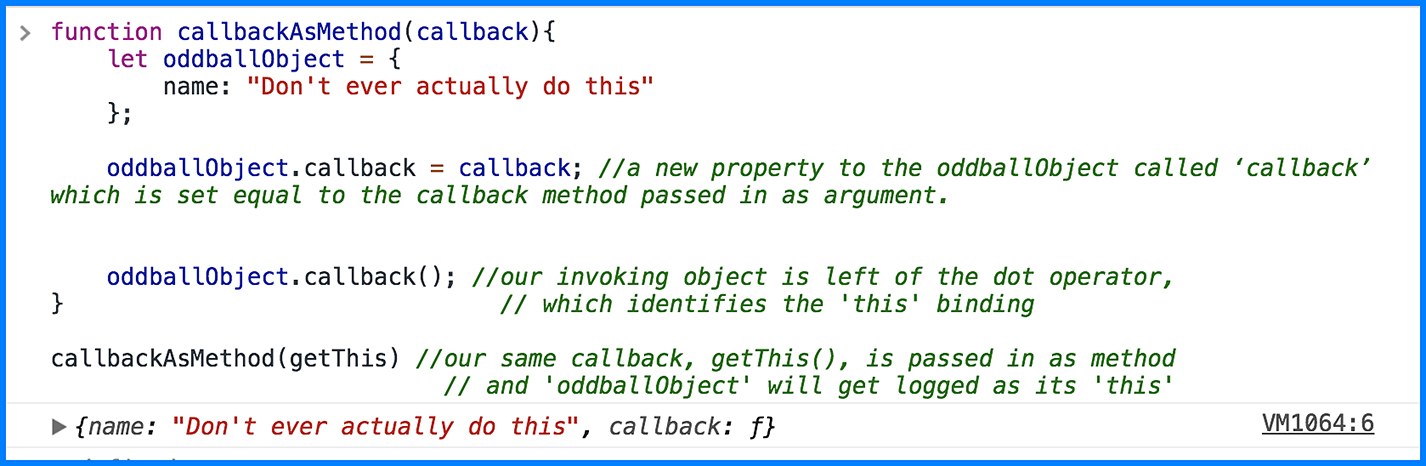
Add a new property to an object javascript. Spread in object literals The Rest/Spread Properties for ECMAScript proposal (ES2018) added spread properties to object literals. It copies own enumerable properties from a provided object onto a new object. Shallow-cloning (excluding prototype) or merging of objects is now possible using a shorter syntax than Object.assign (). The Object.assign () method only copies enumerable and own properties from a source object to a target object. It uses [ [Get]] on the source and [ [Set]] on the target, so it will invoke getters and setters. Therefore it assigns properties, versus copying or defining new properties. JavaScript Program to Add Key/Value Pair to an Object. In this example, you will learn to write a JavaScript program that will add a key/value pair to an object. ... In the above example, we add the new property height to the person object using the dot notation . i.e. person.height = 5.4;. Example 2: Add Key/Value Pair to an Object Using ...
Defining a dynamic property like an Array on the Javascript Object. Let us take the same example as above: var obj = { property1: '', property2: '' }; To create a dynamic property on the object obj we can do: obj['property_name'] = 'some_value'; what this does is, it creates a new property on the object obj which can be accessed as. 27/7/2020 · Javascript add property to Object. To add a new property to Javascript object, define the object name followed by the dot, the name of a new property, an equals sign and the value for the new property. It does not matter if you have to add the property, change the value of the property, or read a value of the property, you have the following choice of syntax. JSON stands for JavaScript Object Notation. A JSON object is really a string that has yet to be turned into the object it represents. To add a property to an existing object in JS you could do the following. object["property"] = value; or . object.property = value;
In order to add a new property to an object, you would assign a new value to a property with the assignment operator (=). For example, we can add a numerical data type to the gimli object as the new age property. Both the dot and bracket notation can be used to add a new object property. gimli.age = 139; 23/3/2021 · Here are the common ways to add properties to an object in Javascript: Use the dot operator – OBJECT.KEY = VALUE; Use the square bracket operator – OBJECT[KEY] = VALUE; Using the spread operator – OBJECT = {...OBJECT, KEY: VALUE, KEY: VALUE}; Finally, the assign function – Object.assign(OBJECT, {KEY: VALUE}); JavaScript Properties. Properties are the values associated with a JavaScript object. A JavaScript object is a collection of unordered properties. Properties can usually be changed, added, and deleted, but some are read only.
To add a new key-value pair to an object, the simplest and popular way is to use the dot notation: foods. custard = '🍮'; console.log( foods); Alternatively, you could also use the square bracket notation to add a new item: foods ['cake'] = '🍰'; console.log( foods); As you can see above, when you add a new item to an object, it usually ... JavaScript is designed on a simple object-based paradigm. An object is a collection of properties, and a property is an association between a name (or key) and a value. A property's value can be a function, in which case the property is known as a method. In addition to objects that are predefined in the browser, you can define your own objects. This chapter describes how to use objects ... The native JavaScript object has no push () method. To add new items to a plain object use this syntax: element [ yourKey ] = yourValue; On the other hand you could define element as an array using
Add a new property to JavaScript object. This video covers 2 fundamental approaches of adding new property to a JavaScript Object. The first one is without modifying the existing object and second approach is by changing the object itself. Read more about assign() method on JavaScript Object … There are two ways to add new properties to an object: var obj = {key1: value1, key2: value2 }; Using dot notation: obj. key3 = "value3"; Using square bracket notation: obj ["key3"] = "value3"; The first form is used when you know the name of the property. The second form is used when the name of the property is dynamically determined. Like in ... You can also add a method to an object literal. For example, the getName() method below takes two properties of the user001 object (firstName and lastName) and returns the user's full name.The this keyword refers to the current object of which properties the method is calling.
Adding a property to an object with ES5 JavaScript The basic functionality is given in JavaScript itself. You can extend properties by calling object.thingYouWantToCall where thingYouWantToCall is the property. The name: values pairs in JavaScript objects are called properties. We can add the property to JavaScript object using a variable as the name by using dot notation or bracket notation. Below example illustrate explain two different approaches: Example 1: In this example, we will be using dot notation. Sometimes we want to add dynamic properties to an object in JavaScript. For instance, we expect to get Properties names from a user inputting. But we can't use them directly as the object's properties traditionally. See the sample code below: var weapons = { sords: true, guns: false, shield: true } var pro = 'arrows'; weapons.pro … Continue reading "How to add dynamic properties to an ...
Adding a property to an object constructor is different from adding a property to a normal object.If we want to add a property we have to add it in the constructor itself rather than outside the constructor whereas we can add anywhere in a normal object.. Example-1. In the following example, a property is added as it is in the case of a normal object. ... Add New Properties to a JavaScript Object You can add new properties to existing JavaScript objects the same way you would modify them. Here's how we would add a bark property to ourDog: addition: It can add new objects by simply giving values to those new objects. deletion: It uses delete keyword, to delete a property from an object. Example 1: Shows adding a new property to the existing object.
In JavaScript, we can add new properties in an object when we defined the object, and also even if the object is already declared we can still add new properties in an object by using dot notation (.) or square bracket notation ([]) with new property/key name followed by assignment operator with property value. This would result in the same person object as before with the properties firstName and lastName.Also, here the last properties are overwriting the first properties, but we still have a problem of applying the object returned in the && expression into the object because the current version assumes that lastName is just a key and has a static value.. We can pass an object to be destructured ... The static method Object.defineProperty () defines a new property directly on an object, or modifies an existing property on an object, and returns the object.
var data = { 'Property1': 1 }; // Two methods of adding a new property [ key (Property4), value (4) ] to the // existing object (data) data['Property2'] = 2; // bracket method data.Property3 = 3; // dot method console.log(data); // { Property1: 1, Property2: 2, Property3: 3 } // But if 'key' of a property is unknown and will be found / calculated // dynamically then use only [bracket] method not a dot method var key; … proto. The object which should be the prototype of the newly-created object. propertiesObject Optional. If specified and not undefined, an object whose enumerable own properties (that is, those properties defined upon itself and not enumerable properties along its prototype chain) specify property descriptors to be added to the newly-created object, with the corresponding property names.
2 Ways To Remove A Property From An Object In Javascript
Add Read Only Immutable Property To A Javascript Object
Java Properties File How To Read Config Properties Values In
Vipul Kelkar Sharepoint Office 365 Azure Index Web
How To Add A New Property To An Existing Object Dynamically
How To Remove Property From Javascript Object Tecadmin
How To Use Object Destructuring In Javascript
How To Get All Property Values Of A Javascript Object
A Deeper Look At Objects In Javascript
How To Merge Two Objects In Javascript
Understanding Classes And Objects
Everything About Javascript Objects By Deepak Gupta
Data Structures Objects And Arrays Eloquent Javascript
How To Use Object Destructuring In Javascript
Mastering This In Javascript Callbacks And Bind Apply
How To Add An Object To An Array In Javascript Geeksforgeeks
How To Find Unique Values By Property In An Array Of Objects
Javascript Array Of Objects Tutorial How To Create Update
Diving Deeper In Javascripts Objects By Arfat Salman Bits
How To Get The Length Of An Object In Javascript
How Can I Add A Key Value Pair To A Javascript Object
Javascript Array Push Cannot Add Property 0 Object Is Not
3 Ways To Add Dynamic Key To Object In Javascript Codez Up
0 Response to "27 Add A New Property To An Object Javascript"
Post a Comment