20 Javascript Catch Error Message
In JavaScript, you have to use an if statement within a single "catch" block: try { execute this block } catch (error) { if (error.name === 'RangeError') { do this } else if (error.name === 'ReferenceError') { do this } else { do this for more generic errors } } Get Weekly Developer Tips. I send out a short email each friday with code snippets, tools, techniques, and interesting stuff from around the web.
Js Try Catch Get Error Message Code Example
JavaScript catches adddlert as an error, and executes the catch code to handle it. JavaScript try and catch The try statement allows you to define a block of code to be tested for errors while it is being executed. The catch statement allows you to define a block of code to be executed, if an error occurs in the try block.
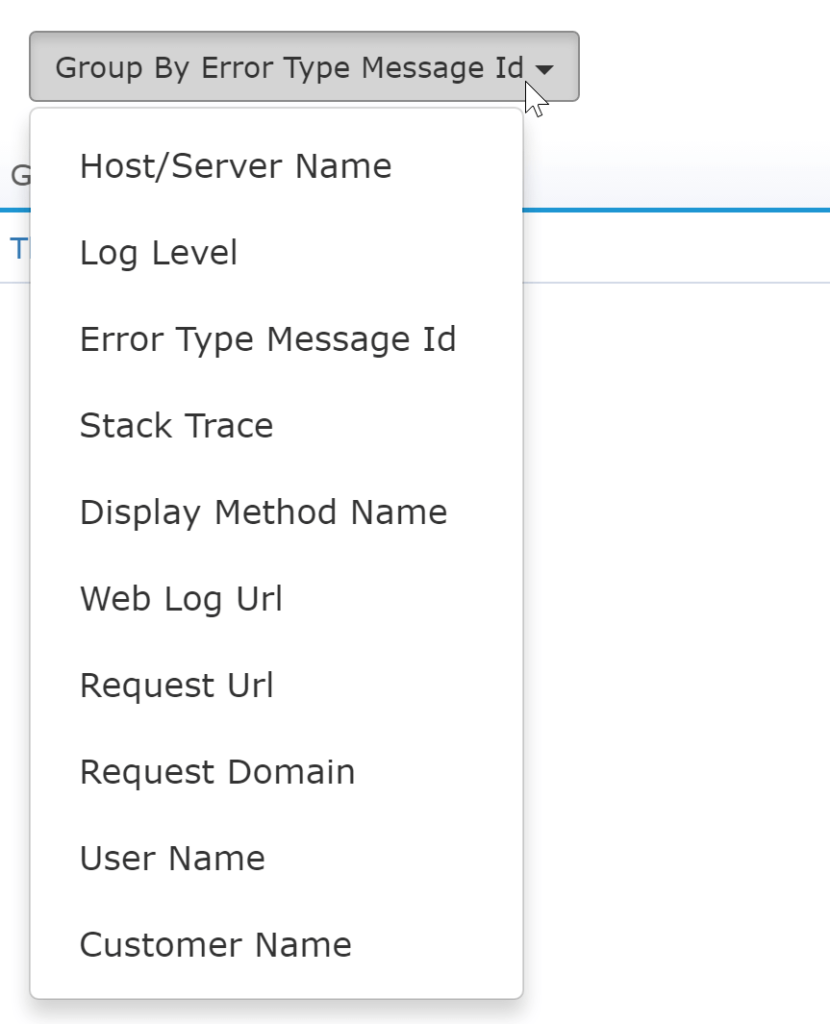
Javascript catch error message. 27/3/2019 · Javascript Throws Block The throw Statement. When any error occurs, JavaScript will stop and generate an error message. The throw statement lets you create your own custom error. Technically you can throw your custom exception (throw an error). The exception can be a JavaScript … Dec 16, 2020 - In this article we will cover what exception handling is, the different types of errors, and the error objects in JavaScript. Jun 07, 2019 - catch: The block that follows this statement will see if the code following the try statement gave an error, and decides what to do with it · With these statements, in JavaScript, you can also add a throw or a finally clause. Let’s see what role they play.
UnhandledPromiseRejectionWarning: Unhandled promise rejection. This error originated either by throwing inside of an async function without a catch block, or by rejecting a promise which was not handled with .catch(). (rejection id: 6) Download Free Files API. In this article I will explain how to handle errors and exceptions in jQuery AJAX calls and show (display) Custom Exception messages using jQuery Dialog. There are two types of Exceptions which is caught by jQuery. 1. When exception object is in the form of JSON object. 2. If an error occurs, JavaScript terminates the code execution and jumps to the catch block. In the catch block, you can access an error object that contains at least the name of the error and message that explains the error in detail. Different web browsers may add more property to the error object.
The main code is inside the try block. While executing the try block, if any error occurs, it goes to the catch block. The catch block handles the errors as per the catch statements. If no error occurs, the code inside the try block is executed and the catch block is skipped. Quiz: What does this call to the web's new fetch() API do?fetch Learn JavaScript Try, Throw, Catch, and Finally
Syntax Errors Syntax errors, also called parsing errors, occur at compile time in traditional programming languages and at interpret time in JavaScript. For example, the following line causes a syntax error because it is missing a closing parenthesis. <script type = "text/javascript"> <!-- window.print (; //--> </script> 3 weeks ago - If you don't have control over the original errors that are thrown, one option is to catch them and throw new Error objects that have more specific messages. The original error should be passed to the new Error in the constructor option parameter (cause property), as this ensures that the original ... // scripts/badHandler.js function badHandler (fn) {try {return fn ();} catch (e) {} return null;}. This handler receives a fn callback as a parameter. This callback then gets called inside the ...
Tip: When an error occurs, JavaScript will normally stop, and generate an error message. Use the throw statement to create a custom error (throw an exception). If you use throw together with try and catch, you can control program flow and generate custom error messages. A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. The try...catch statementin Javascriptenables web developers handle javascript errorswithin a block of code. While browsing on the web, we frequently get javascript alert messages indicating that a runtime error has occured. And the browser message …
Mar 30, 2021 - So how do we hit send on our error? By throwing. But what does it mean to throw? And what does that mean for our program? Throw really does two things: it stops the program, and it finds a catch to execute. Let’s examine these ideas one at a time. When JavaScript finds a throw keyword, the ... 30/12/2020 · One of the benefits of try/catch is its ability to display your own custom-created error. This is called (throw error). In situations where you don't want this ugly thing that JavaScript displays, you can throw your error (an exception) with the use of the throw statement. This error can be a string, boolean, or object. Exception Handling in JavaScript The try-catch. As with many programming languages, the primary method of dealing with exceptions in JavaScript is the try-catch. In a nutshell, the try-catch is a code block that can be used to deal with thrown exceptions without interrupting program execution.
Where the try block contains the suspected code that can generate errors. If an error occurs then try block throws the exception that is caught by the catch block, in which we can handle it as we want. We can either show a user readable message or we can show the error message in an alert box. Network tab output {"name":["The name field is required."],"parts":["The parts field is required."]} I should be seeing an object that contains JSON form validation as that is the response in my network tab, i seem to get the JS catch output? Aug 18, 2020 - Learn how to deal with errors and exceptions in synchronous and asynchronous JavaScript code.
Jan 07, 2019 - Note that promises without catch-block will not terminate the script, but will give you a less readable message like · (node:7741) UnhandledPromiseRejectionWarning: Unhandled promise rejection (rejection id: 1): Error: something went wrong (node:7741) DeprecationWarning: Unhandled promise ... Aug 01, 2020 - Get code examples like "js try catch get error message" instantly right from your google search results with the Grepper Chrome Extension. If you use the try block, you need to follow it with either the catch statement, the finally block or both. You might think that the finally statement does not serve any purpose, because you can write code just under the try…catch block. The fact is it is not always going to be executed. Examples of that are nested try-blocks.
catch (catchID) { statements } The catch block specifies an identifier (catchID in the preceding syntax) that holds the value specified by the throw statement. You can use this identifier to get information about the exception that was thrown. JavaScript creates this identifier when the catch block is entered. Try entering a none numeric value (ie: "haha") or a number less than 13 (ie: 11). In both cases, by using throw, control is instantly transferred to catch, with e.message displaying a different message. Technically entering a string or number less than 13 certainly doesn't constitute an exception in JavaScript, though for our purpose here, they should. 5/8/2016 · So the catch(error => ) is actually just catch(response => ) EDIT: I still dont understand why logging the error returns that stack message. I tried logging it like this. And then you can actually see that it is an object. console.log('errorType', typeof error); console.log('error', Object.assign({}, error)); EDIT2:
When a problem arises and is then handled, an "exception is thrown" by the Javascript interpreter. Javascript generates an object containing the details about it, which is what the (error) is... This may or may not be caused by a JavaScript error—either way, don't panic and just reload the page. Then we can move on to checking for JavaScript errors in further detail. Check If JavaScript Is Turned On. We must keep in mind that JavaScript is set differently for each browser. 25/1/2021 · Here it gets an unexpected error, but still shows the same "JSON Error" message. That’s wrong and also makes the code more difficult to debug. To avoid such problems, we can employ the “rethrowing” technique. The rule is simple: Catch should only process errors that it knows and “rethrow” all others.
All in One Software Development Bundle (600+ Courses, 50+ projects) 600+ Online Courses. 50+ projects. 3000+ Hours. Verifiable Certificates. Lifetime Access Tip: When an error occurs, JavaScript will normally stop, and generate an error message. Use the throw statement to create a custom error (throw an exception). If you use throw together with try and catch, you can control program flow and generate custom error messages. Apr 19, 2021 - Languages generally come with a ... error message should help you work out what's going on. More on Node errors. Catch - this is what you do when someone throws something at you, right? You'd probably do it reflexively even if someone threw you one of these... ?! The catch statement in JavaScript lets you handle ...
If any statement within the try -block (or in a function called from within the try -block) throws an exception, control is immediately shifted to the catch -block. If no exception is thrown in the try -block, the catch -block is skipped. The finally -block will always execute after the try -block and catch -block (s) have finished executing. Join Stack Overflow to learn, share knowledge, and build your career. What happens when a regular error occurs and is not caught by try..catch? The script dies with a message in the console. A similar thing happens with unhandled promise rejections. The JavaScript engine tracks such rejections and generates a global error in that case.
Exception handlers enable the programmer to detect errors and then resolve them easily, it helps users who are unware of error messages. Javascript Exception Handling: try/catch Statements Example. Return an error message (we have written "alert" as "adddlert" to deliberately produce an error): try {. adddlert ("Welcome guest!"); } catch(err) {. document.getElementById("demo").innerHTML = err.message; } … Apr 28, 2021 - The try...catch..finally statement specifies a block of code to try along with a response should an error occur. The try statement contains one or more try blocks, and ends with at least one catch and/or a finally clause.
Server responded with a status code not equal to 200, and Javascript code throws an Error object. Promise returned by response.json () may be rejected due to invalid JSON response.
How To Handle Photonjs Errors Issue 600 Prisma Prisma
Introduction To Error Handling In Angular 7 Part 1 Angular
How To Catch Sign Up Error And Display Error With Lock
Javascript Global Event Mechanism Stack Overflow
Node Js Error Handling Best Practices Ship With Confidence
Javascript Error Handling Ten Years Ago At The Beginning Of
Exception Reporting In Power Bi Catch The Error Rows In
Real World Servicenow Exception Handling Developer
5 Ways To Make Http Requests In Node Js Using Async Await
Php Try Catch Basics Amp Advanced Php Exception Handling
Try Catch Finally Throws User Defined Exception In Vb Net
Async Await Without Try Catch In Javascript By Dzmitry
Node Js Tutorial Node Js Errors And Exceptions
Not Able To Get Error Message In Axios Catch Stack Overflow
Print Error Message In Javascript Code Example
Javascript Chapter 15 Debugging Techniques
Node Js 10 0 Fills Some Gaps Polishes A Few Rough Edges
0 Response to "20 Javascript Catch Error Message"
Post a Comment