29 Javascript To Number From String
The toString () method is a built-in method of the JavaScript Number object that allows you to convert any number type value into its string type representation. How to Use the toString Method in JavaScript To use the toString () method, you simply need to call the method on a number value. Examples of JavaScript Convert String to Number. Often times you want to convert a string into a number for various purposes. Lets take a look at some of the examples on how we can achieve this. parseInt() We have a built-in function called parseInt in JavaScript to convert string to number. Here is the example
Use the parseInt() Function to Convert a String to Integer in JavaScript. Another way of dealing with string numbers is using the parseInt() method of JavaScript. parseInt() takes two arguments, one is the string that needs to be converted, and the other one is the radix (means the base). Most numbers that we deal with in our day-to-day life are usually in the base of 10, which signifies a decimal value.
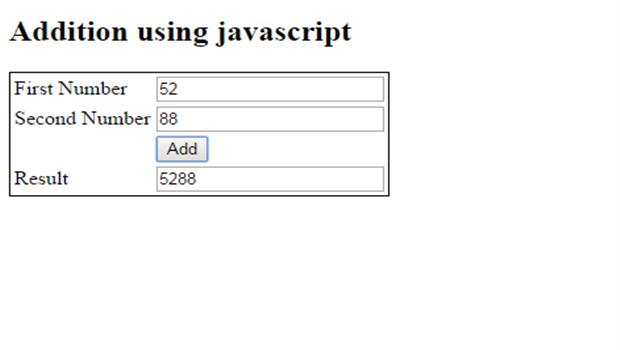
Javascript to number from string. Sep 13, 2020 - There are two main ways to convert a string to a number in javascript. One way is to parse it and the other way is to change its type to a Number. All of the tricks in the other answers (e.g. unary plus) involve implicitly coercing the type of the string to a number. I would like to convert a string like "1 2 3-5" to an array of numbers [1, 2, 3, 4, 5]. Following the robustness principle, the list doesn't need to be separated by ... May 16, 2021. by Rohit. Using the regular expression and match method you can extract phone numbers from strings in JavaScript. Let's take three different patterns of number that you want to search. 10 digits. + sign followed by 10 digits. (xxx)-xxxxxxX format. These patterns are matched with the following regex.
The JavaScript interpreter works from left to right. First 10 + 20 is added because x and y are both numbers. Then 30 + "30" is concatenated because z is a string. Nov 03, 2019 - There are some built-in methods in JavaScript that provide conversion from an number data type to a String, which only differ in performance and readability. There are many ways you can extract or get numbers of a string in JavaScript. One of the simplest ways of extracting numbers from a given string in JavaScript is using Regex or Regular Expressions. Let us assume I have a string value, a name, suffixed with some random numbers. var tName = 'arun_4874';
function justNumbers(string) { var numsStr = string.replace(/[^0-9]/g, ''); return parseInt(numsStr); } var input = "Rs. 6,67,000"; var number = justNumbers(input); console.log(number); // 667000 Share May 13, 2019 - In JavaScript, you can represent a number is an actual number 30, or as a string ‘30’. If you were to use a strict comparison to compare the two, it would fail because they’re two different types of objects. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
4 weeks ago - If no signs are found, the algorithm ... number-parsing on the rest of the string. A value passed as the radix argument is coerced to a Number (if necessary), then if the value is 0, NaN or Infinity (undefined is coerced to NaN), JavaScript assumes the following:... The difference between the String() method and the String method is that the latter does not do any base conversions. How to Convert a Number to a String in JavaScript¶ The template strings can put a number inside a String which is a valid way of parsing an Integer or Float data type: Aug 30, 2016 - I randomized the order because ... similar code from the previous test. Unlike the top answerer, I found that implicit was the worst method. ... Here is simple way to do it: var num = Number(str); in this example str is the variable that contains the string....
16/5/2019 · The number from a string in javascript can be extracted into an array of numbers by using the match method. This function takes a regular expression as an argument and extracts the number from the string. Regular expression for extracting a number is (/ (\d+)/). Example 1: This example uses match () function to extract number from string. Learn how to convert a string to a number using JavaScript. This takes care of the decimals as well. Number is a wrapper object that can perform many operations. If we use the constructor (new Number("1234")) it returns us a Number object instead of a number value, so pay attention.Watch out for separators between digits: Aug 28, 2018 - Not the answer you're looking for? Browse other questions tagged javascript regex string numbers numeric or ask your own question. ... Swag is coming back! ... Is everything that has happened, is happening and will happen just a reaction to the action of Big Bang?
Number() The Number() method converts a string to a number.. Sometimes it's an integer. Other times it's a point number. And if you pass in a string with random text in it, you'll get NaN, an acronym for "Not a Number.". As a result of this inconsistency, it's a less safe choice than parseInt() and parseFloat().If you know the format of the number you'd like, use those instead. The Number () function converts the object argument to a number that represents the object's value. If the value cannot be converted to a legal number, NaN is returned. Note: If the parameter is a Date object, the Number () function returns the number of milliseconds since midnight January 1, 1970 UTC. Dec 29, 2018 - There are two main ways to convert a string to a number in javascript. One way is to parse it and the other way is to change its type to a Number. All of the tricks in the other answers (e.g. unary plus) involve implicitly coercing the type of the string to a number.
But, JavaScript has another method for it, known as parseInt () and parseFloat () respectively to parse integer and float values from the string. The numbers should be present at the beginning of the string and before any other character. These functions return numbers parsed till either the string ends or encounters first character. Jul 20, 2021 - The toString() method in Javascript is used with a number and converts the number to a string. It is used to return a string representing the specified Number object. Regular expressions are patterns used to match character combinations in strings. In JavaScript, regular expressions are also objects. These patterns are used with the exec() and test() methods of RegExp, and with the match(), matchAll(), replace(), replaceAll(), search(), and split() methods of String. This chapter describes JavaScript regular expressions.
JavaScript comes with built-in methods to remove all numbers from a string. A way to remove numbers is to replace them with an empty string. A regular expression is a good way to find all numbers in the string and replace them: slice () extracts a part of a string and returns the extracted part in a new string. The method takes 2 parameters: the start position, and the end position (end not included). This example slices out a portion of a string from position 7 to position 12 (13-1): Remember: JavaScript counts positions from zero. First position is 0. Sometimes you might end up with a number that is stored as a string type, which makes it difficult to perform calculations with it. This most commonly happens when data is entered into a form input, and the input type is text.There is a way to solve this problem — passing the string value into the Number() constructor to return a number version of the same value.
Use parseInt () function, which parses a string and returns an integer. The first argument of parseInt must be a string. parseInt will return an integer found in the beginning of the string. Remember, that only the first number in the string will be returned. JavaScript automatically converts primitives to String objects, so that it's possible to use String object methods for primitive strings. In contexts where a method is to be invoked on a primitive string or a property lookup occurs, JavaScript will automatically wrap the string primitive and call the method or perform the property lookup. 11/6/2020 · Learn how to extract one or several numbers from a string with JavaScript. Let’s say you have a string, that includes a number, and you want to extract only the number. No problem, you can use JavaScript’s match () method. Here’s a string value, containing one number (1995) that is assigned to a variable called stringWithOneNumber:
5 days ago - An integer between 2 and 36 that represents the radix (the base in mathematical numeral systems) of the string. If radix is undefined or 0, it is assumed to be 10 except when the number begins with the code unit pairs 0x or 0X, in which case a radix of 16 is assumed. ... An integer parsed from the ... Jun 01, 2016 - Consider the following string in javascript: var string="border-radius:90px 20px 30px 40px"; I want to extract the 4 numbers from that string and store them in an array called numbers.To do that I 5/12/2020 · How to get numeric value from string JavaScript? Simply use a regular expression in the replace function to get numbers from the string in JS. Regular expression for extracting a number is (/ (\d+)/). string.replace (/ [^0-9]/g, '');
The previous two approaches work simply due to the fact that JavaScript tries to assimilate the data-types used in a statement such as addition or multiplication. Using String and Number Objects. Another way of transforming a String to number or a number to String is creating a new String or Number object with the new keyword. Jul 17, 2014 - The radix parameter is used to specify which numeral system to be used, for example, a radix of 16 (hexadecimal) indicates that the number in the string should be parsed from a hexadecimal number to a decimal number. If the radix parameter is omitted, JavaScript assumes the following: Number.prototype.toLocaleString([locales [, options]]) Returns a string with a language sensitive representation of this number. Overrides the Object.prototype.toLocaleString() method. Number.prototype.toPrecision(precision) Returns a string representing the number to a specified precision in fixed-point or exponential notation.
The Date.parse() method parses a string representation of a date, and returns the number of milliseconds since January 1, 1970, 00:00:00 UTC or NaN if the string is unrecognized or, in some cases, contains illegal date values (e.g. 2015-02-31).. It is not recommended to use Date.parse as until ES5, parsing of strings was entirely implementation dependent. 22/9/2017 · With Regular Expressions, how to get numbers from a String, for example: String myString = "my 2 first gifts were made by my 4 brothers"; myString = myString .replaceAll("\\D+",""); System.out.println("myString : " + myString); the result of myString is "24" you can see an example of this running code here: http://ideone /iOCf5G Use the Number() Function to Check Whether a Given String Is a Number or Not in JavaScript. The Number() function converts the argument to a number representing the object's value. If it fails to convert the value to a number, it returns NaN. We can use it with strings also to check whether a given string is a number or not. For example ...
+myString.replace (/ [^\d.ex-]+/gi, '') strips out all characters that cannot appear in a JavaScript number, and then applies the + operator to it to convert it to a number. If you don't have numbers in hex format or exponential format then you can do without the ex. Apr 28, 2021 - Always specify this parameter to eliminate reader confusion and to guarantee predictable behavior. Different implementations produce different results when a radix is not specified, usually defaulting the value to 10. Return value An integer number parsed from the given string. If the first ... The substr () method extracts parts of a string, beginning at the character at a specified position, and returns a specified number of characters. Tip: To extract characters from the end of the string, use a negative start number. substr () method does not change the original string.
To convert a string to an integer parseInt() function is used in javascript.parseInt() function returns Nan( not a number) when the string doesn't contain number.If a string with a number is sent then only that number will be returned as the output. This function won't accept spaces. If any particular number with spaces is sent then the part of the number that presents before space will be ...
Javascript String To Float Two Methods To Convert String To
The String Data Type In Javascript Dummies
27 String To Number Conversion Javascript Bizanosa
Javascript Function Counts The Number Of Vowels Within A
How To Convert Strings To Numbers Using Javascript
Extract Number From String Javascript Code Example
Adding Two Numbers In Javascript Incorrectly Stack Overflow
Javascript Number To String How To Use Tostring To Convert
How To Compare String And Number In Javascript Code Example
Javascript Check If Variable Is Number Code Example
String And Number Methods In Javascript By Surya Shakti
How To Convert A String To An Integer In Javascript Stack
7 Ways To Convert A String To Number In Javascript Dev
Easily Convert Javascript Strings To Numbers
Javascript Variables And Data Types Number String Boolean
Javascript Type Conversion Number Parseint Parsefloat
Type Conversion Between Node Js And C Alibaba Cloud
How To Convert Integer Array To String Array Using Javascript
What Are The Different Data Types In Javascript Edureka
Convert String To Integer In Javascript Reactgo
Ways To Convert String To Number In Js Time To Hack
Javascript Extract Number From String Regex Amp Replace
How To Convert String To Number In Javascript
Javascript Program To Count The Number Of Vowels In A String
Allow Only Number Or String In Textbox Using Javascript
Javascript Basics For Beginners Javascript Fundamentals
Javascript Extract Phone Number From String Text Regex Read
0 Response to "29 Javascript To Number From String"
Post a Comment